1. Req
import { Controller, Get, Req } from '@nestjs/common';
import { Request } from 'express';
import { AppService } from './app.service';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
getHello(@Req() req: Request): string {
console.log(req);
return 'hello';
}
}
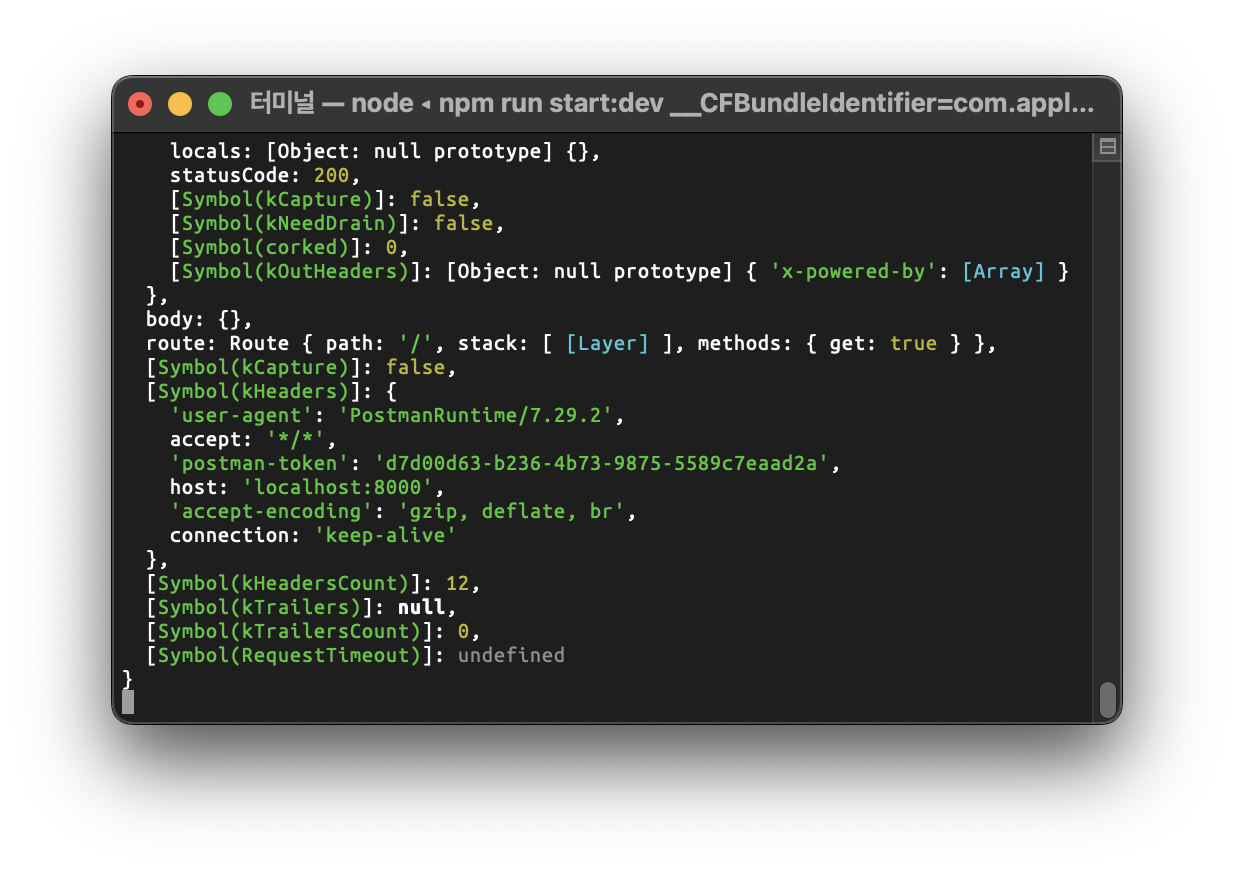
2. Body
import { Body, Controller, Get } from '@nestjs/common';
import { AppService } from './app.service';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
getHello(@Body() body: any): string {
console.log(body);
return 'hello';
}
}
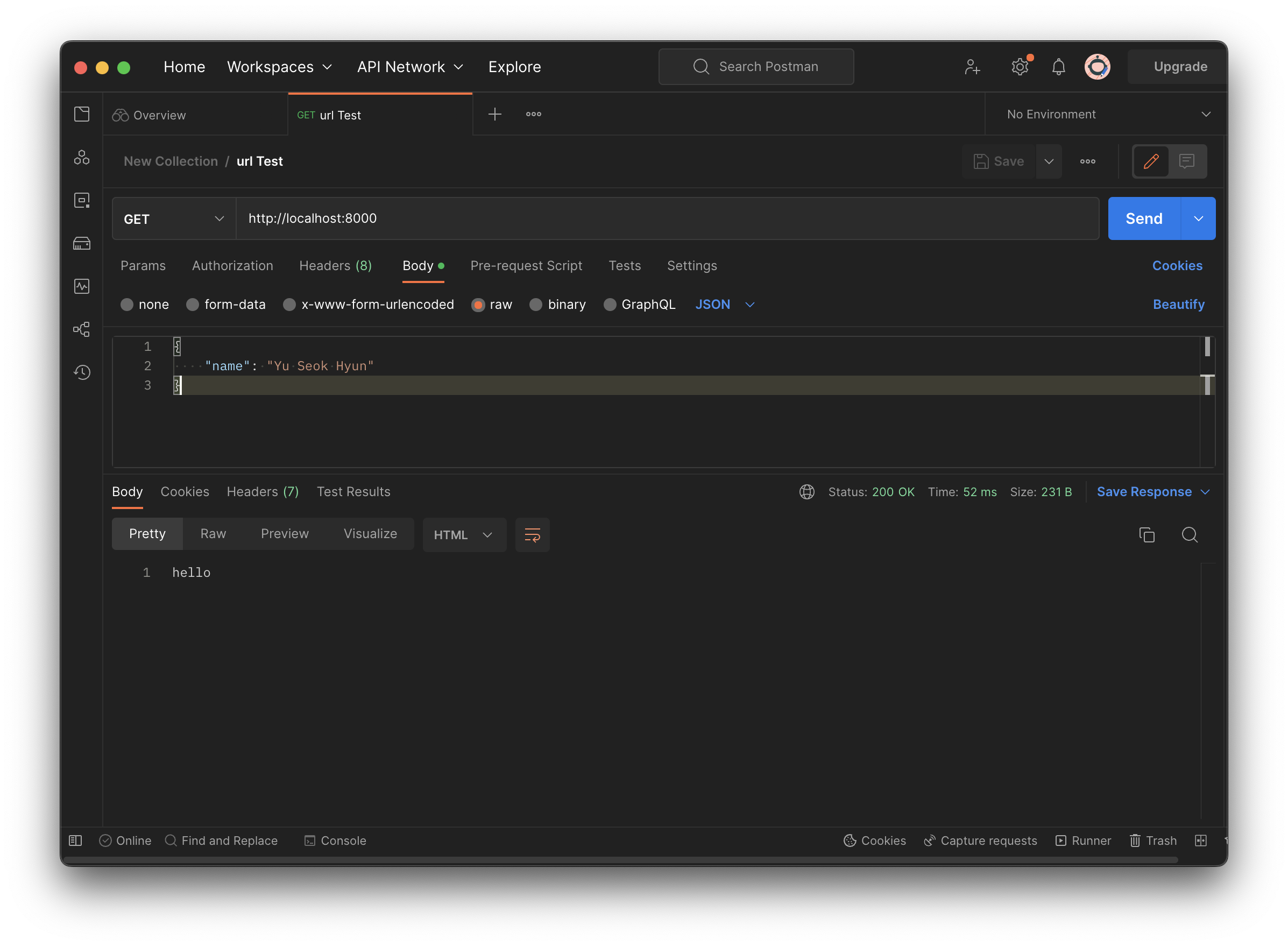
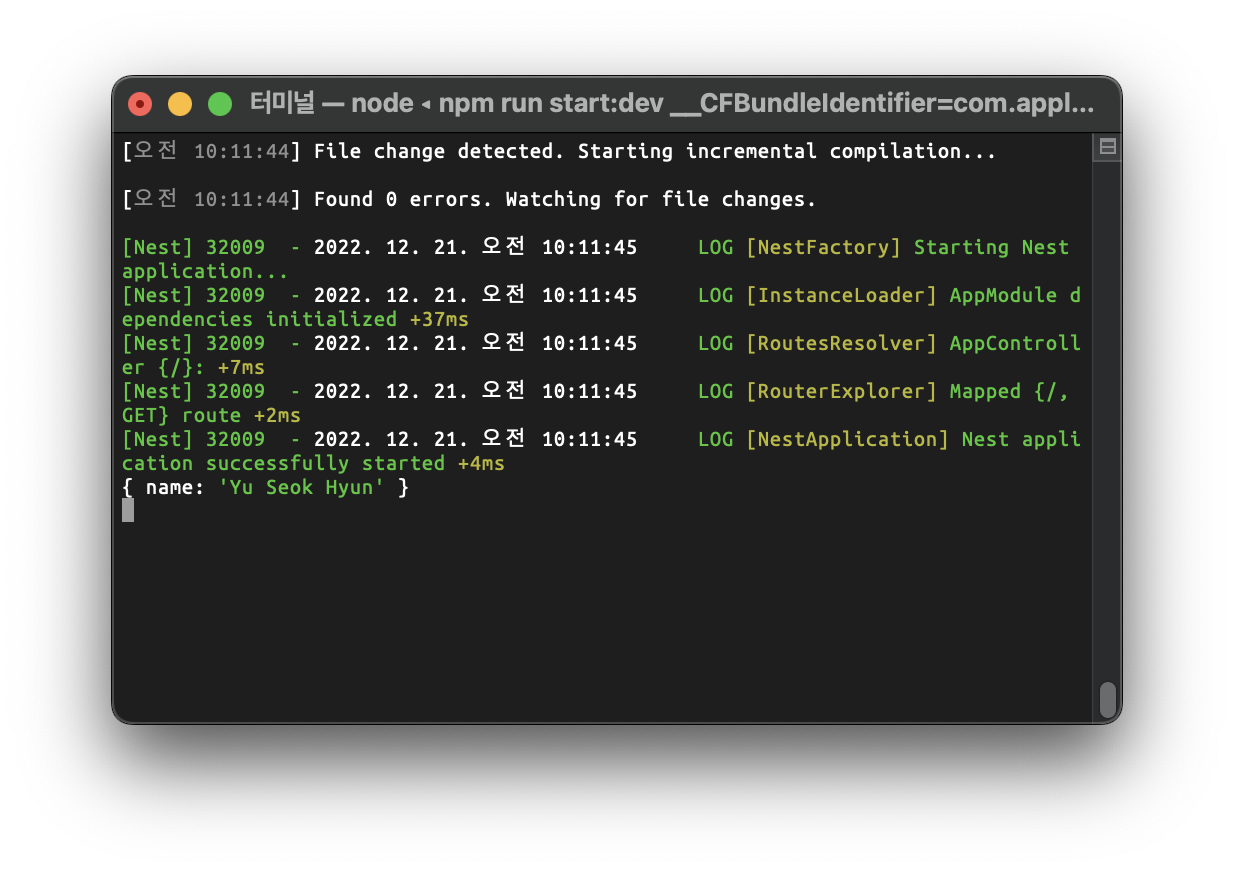
3. Param
import { Controller, Get, Param } from '@nestjs/common';
import { AppService } from './app.service';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get('/:id')
getHello(@Param() param: any): string {
console.log(param);
return 'hello';
}
}
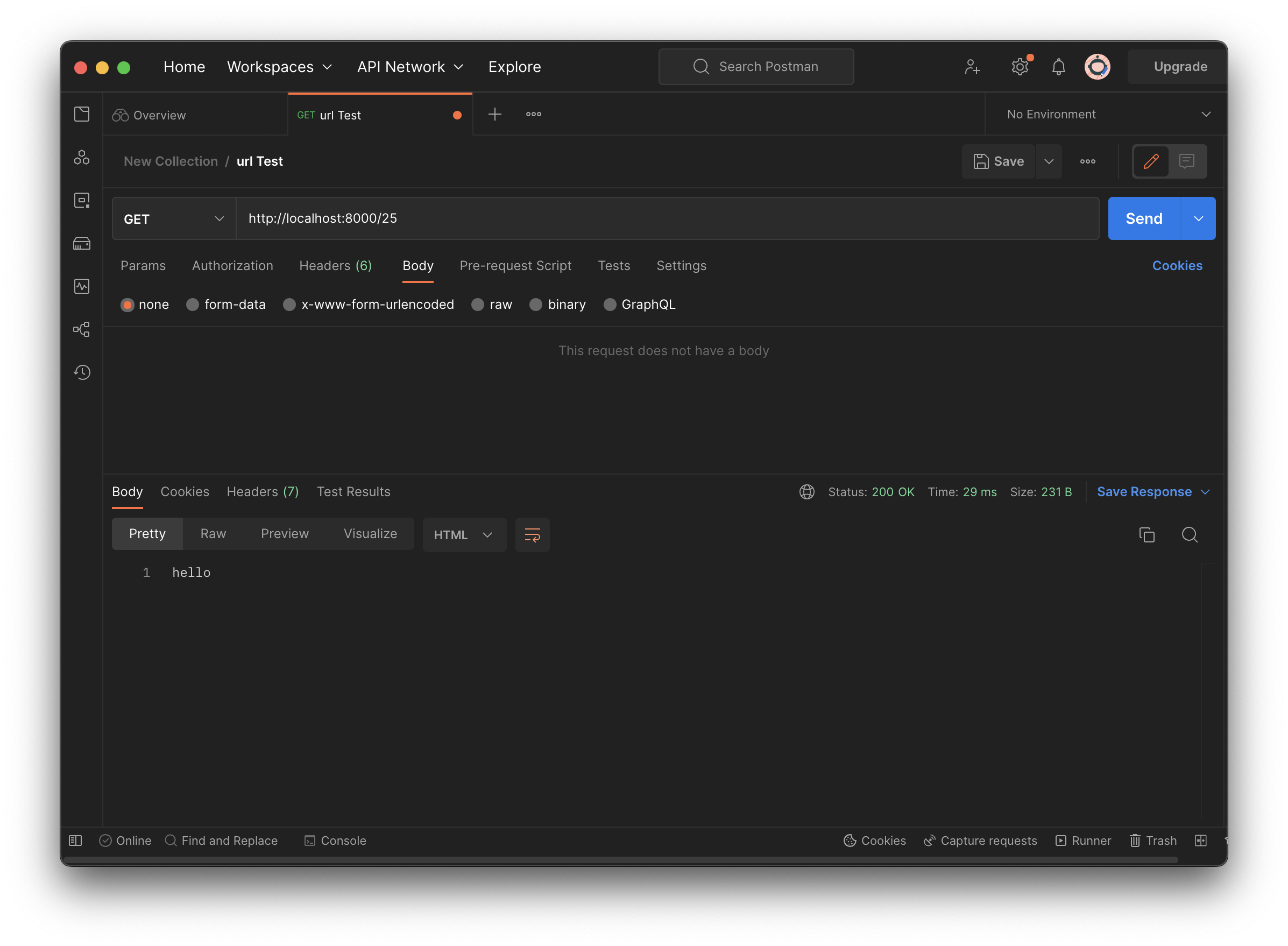
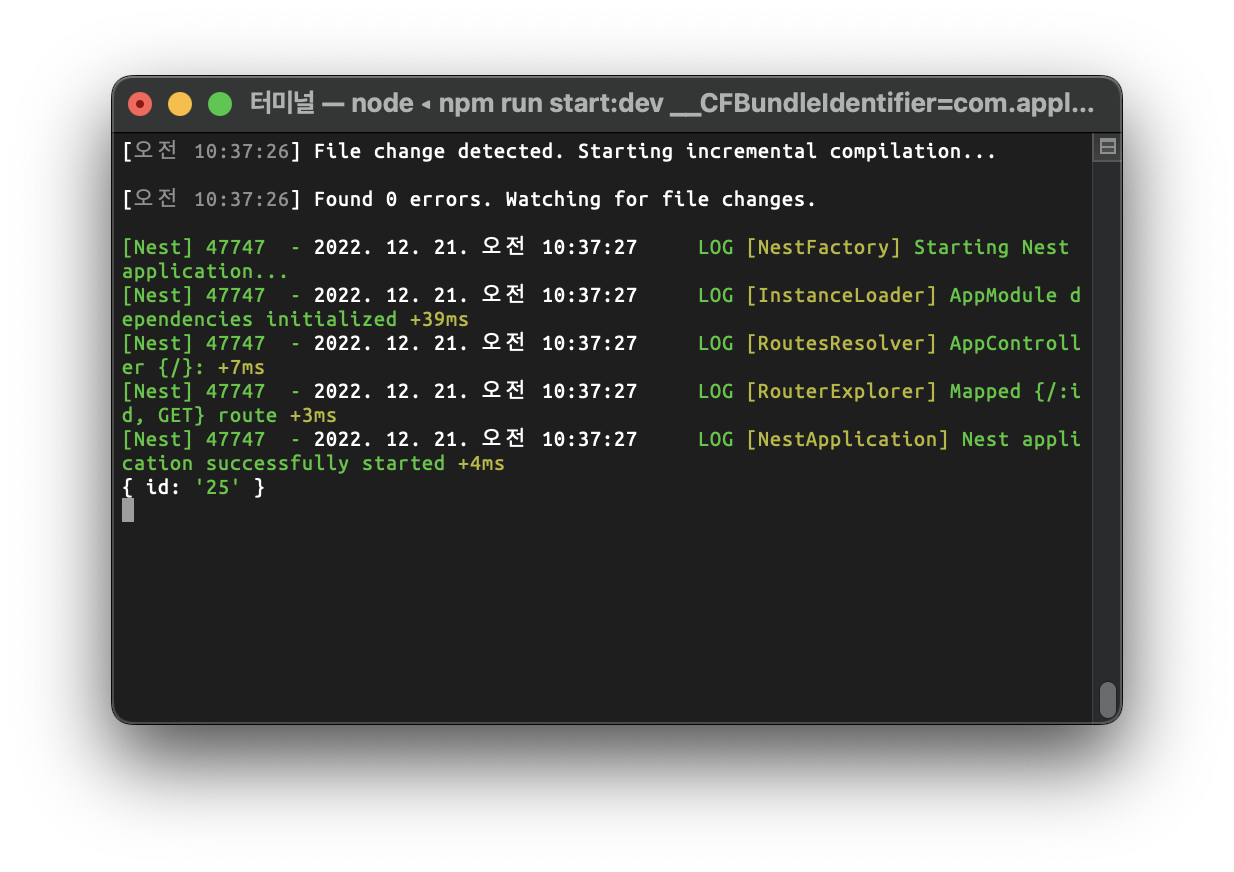
import { Controller, Get, Param } from '@nestjs/common';
import { AppService } from './app.service';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get('/:id')
getHello(@Param('id') id: string): string {
console.log(id);
return 'hello';
}
}
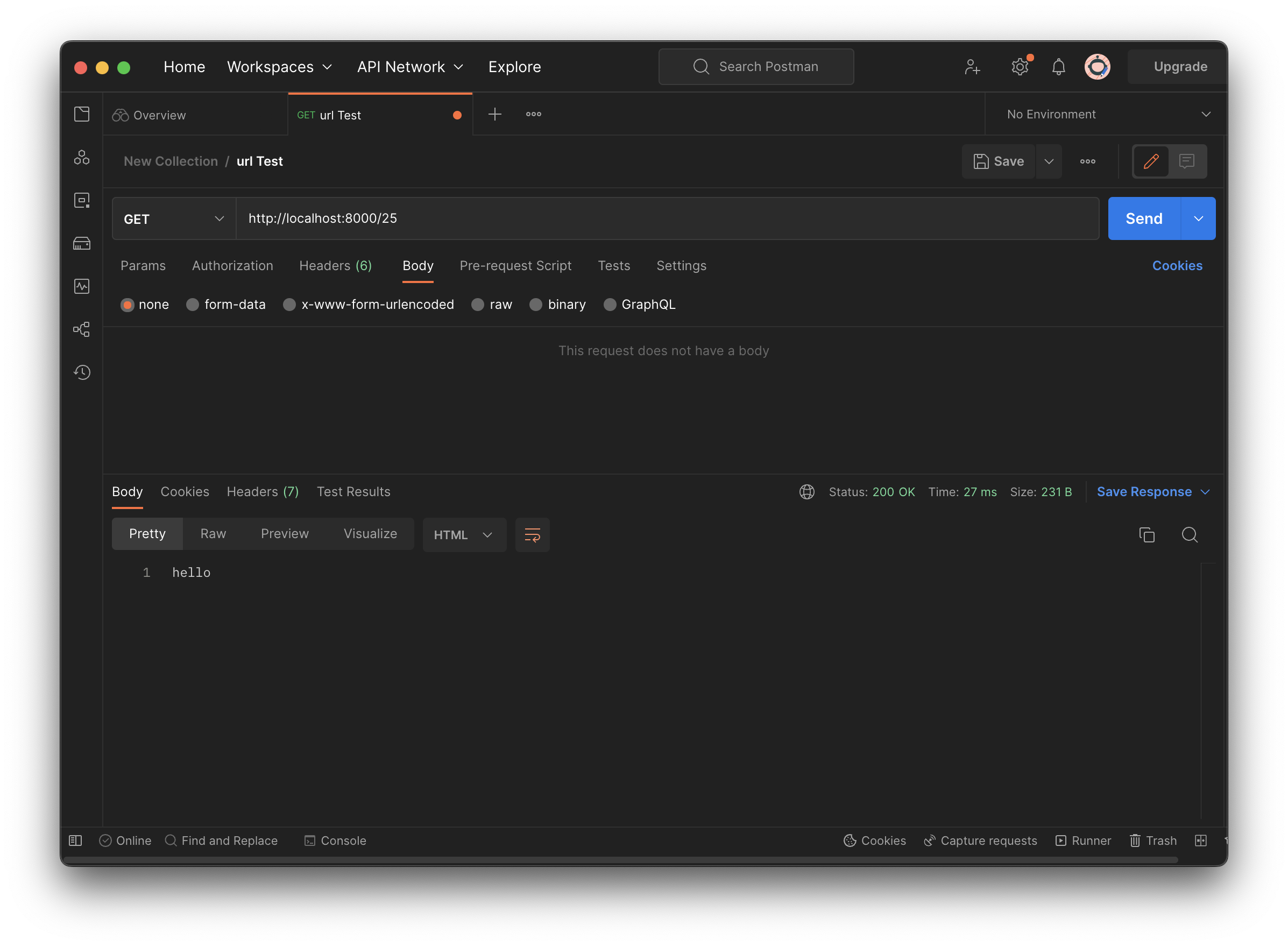
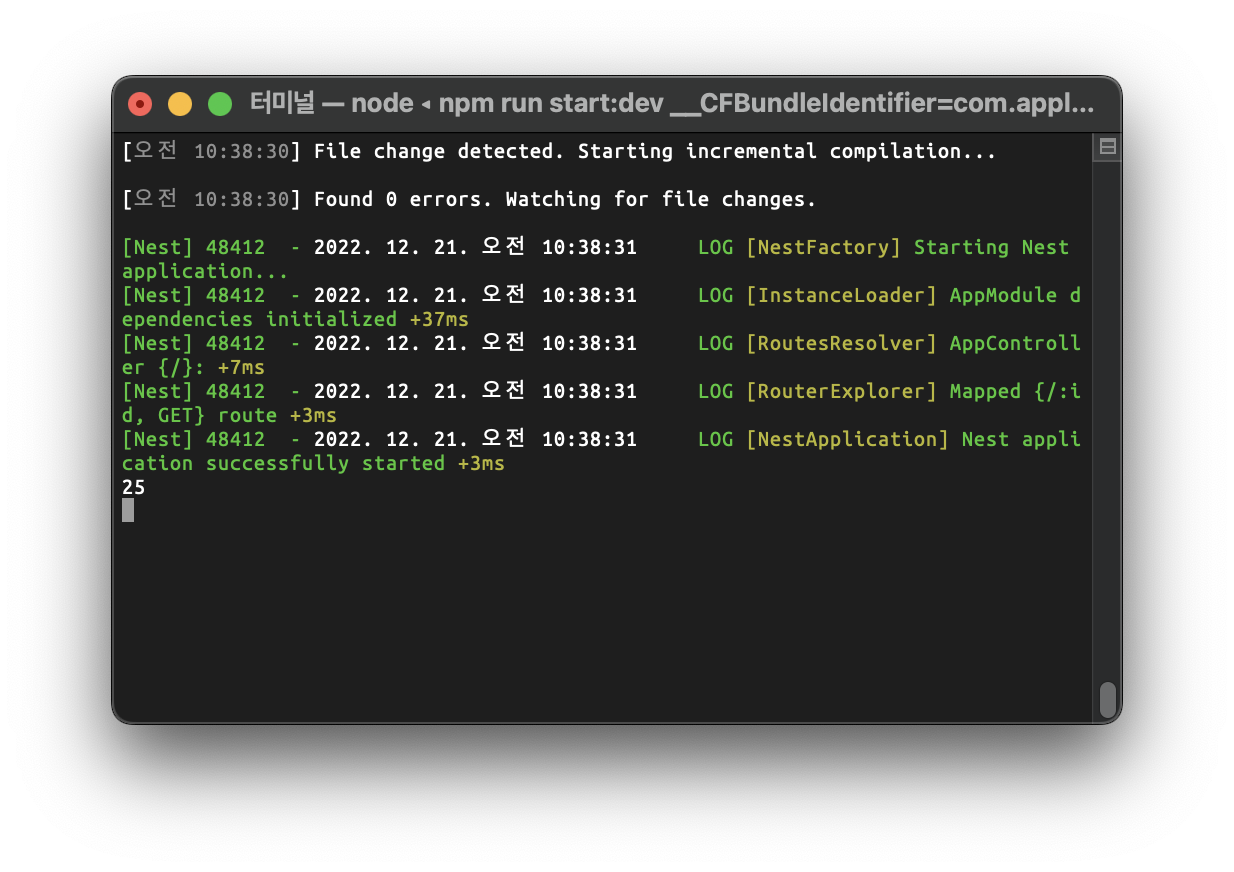
4. Query
import { Controller, Get, Query } from '@nestjs/common';
import { AppService } from './app.service';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
getHello(@Query() query: any): string {
console.log(query);
return 'hello';
}
}
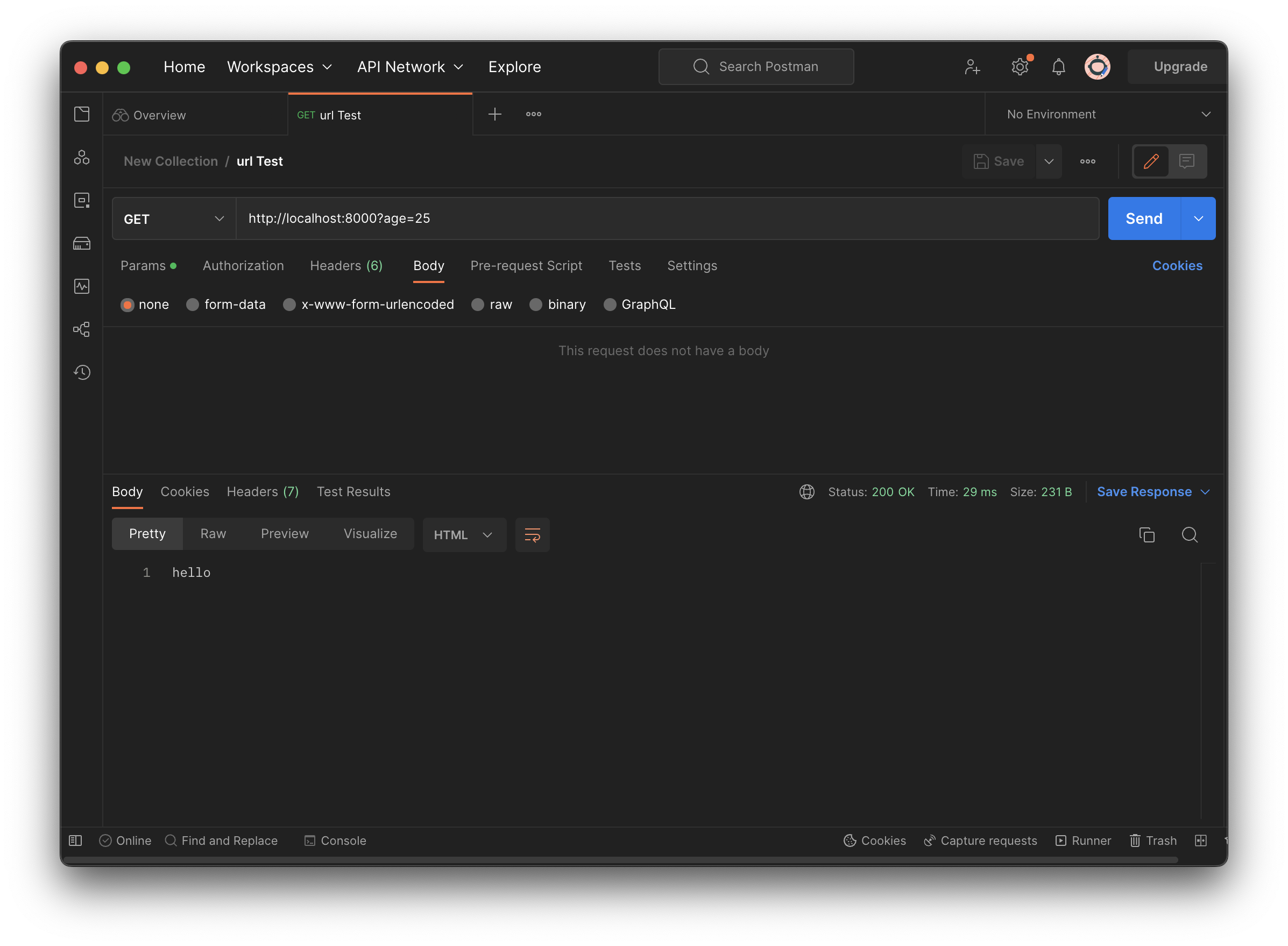
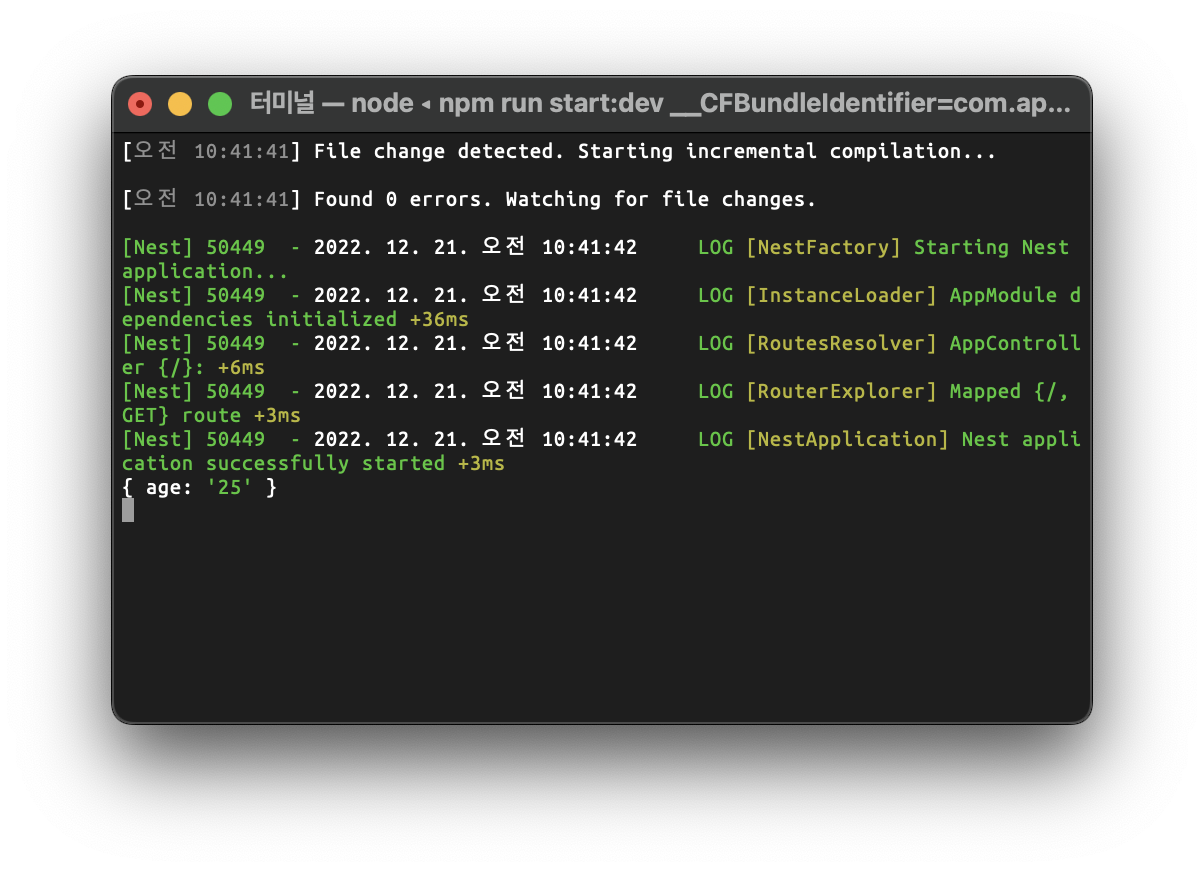
import { Controller, Get, Query } from '@nestjs/common';
import { AppService } from './app.service';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
getHello(@Query('age') age: string): string {
console.log(age);
return 'hello';
}
}
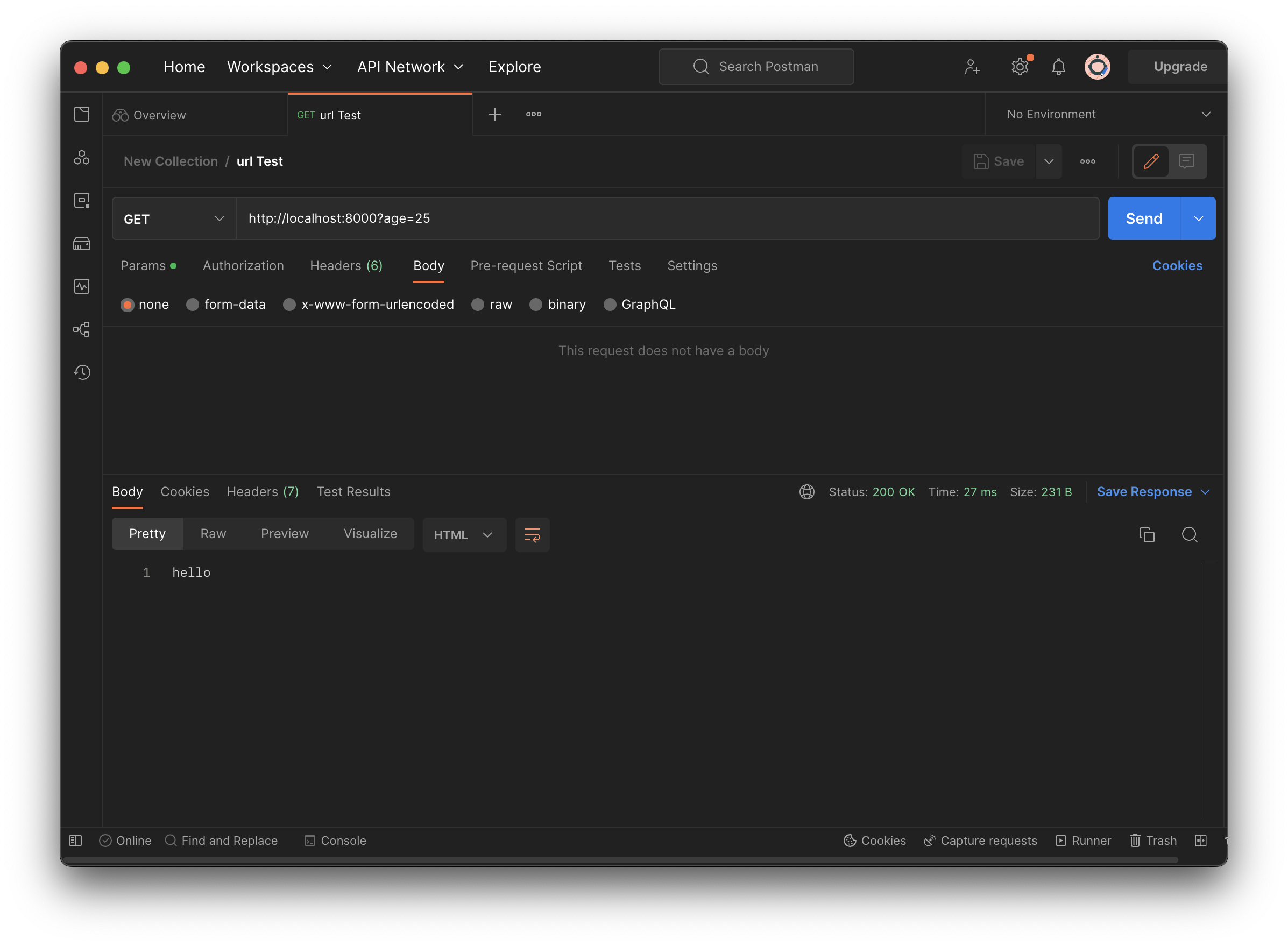
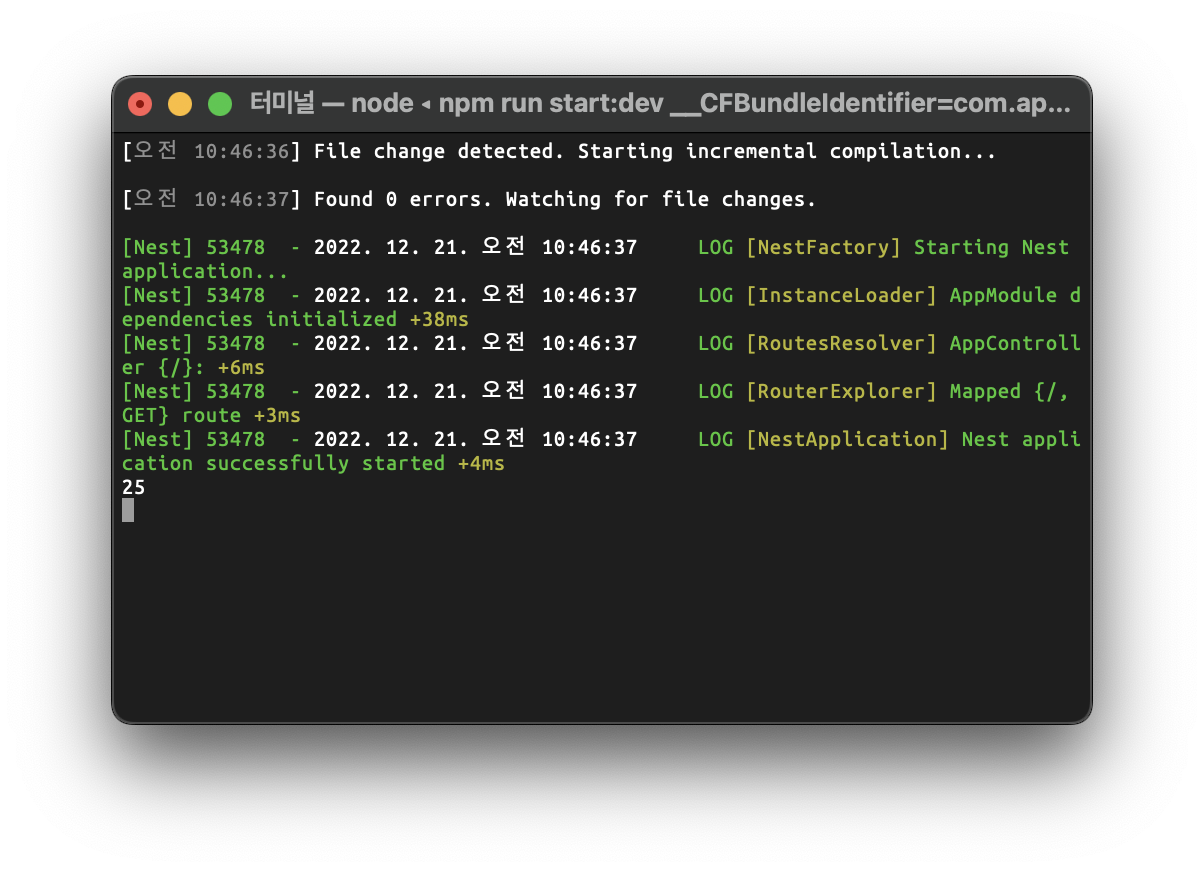