Quiz 1.
interface Product {
id: number;
name: string;
price: number;
seller: {
id: number;
name: string;
company: string;
};
}
function getSellersFromProducts(products: any): any {
return products.map((product) => product.seller);
}
import { Equal, Expect } from "@type-challenges/utils";
type TestCases = [
Expect<Equal<Parameters<typeof getSellersFromProducts>[0], Product[]>>,
Expect<Equal<ReturnType<typeof getSellersFromProducts>, Product["seller"][]>>
];
βοΈλ΅μ(νλ¦Ό)
function getSellersFromProducts(products: Product[]): Product["seller"] {
return products.map((product) => product.seller);
}
μ λ΅
function getSellersFromProducts(products: Product[]): Product["seller"][] {
return products.map((product) => product.seller);
}
ν΄μ€
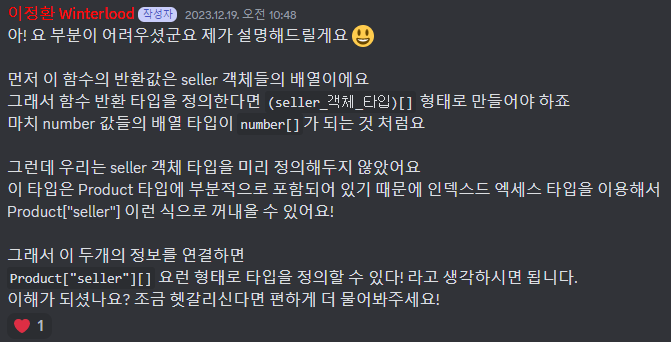
Quiz 2.
interface Product {
id: number;
name: string;
price: number;
seller: {
id: number;
name: string;
company: string;
};
}
type PartialProduct = any;
type ReadonlyProduct = any;
type ReadonlyPartialProduct = any;
import { Equal, Expect, NotEqual } from "@type-challenges/utils";
type TestCases = [
Expect<Equal<PartialProduct, Partial<Product>>>,
Expect<Equal<ReadonlyProduct, Readonly<Product>>>,
Expect<Equal<ReadonlyPartialProduct, Readonly<Partial<Product>>>>
];
βοΈλ΅μ
type PartialProduct = {
[key in keyof Product]?: Product[key];
};
type ReadonlyProduct = {
readonly [key in keyof Product]: Product[key];
};
type ReadonlyPartialProduct = {
readonly [key in keyof Product]?: Product[key];
};
μ½λλ§ν¬
Quiz 3.
type Score = any;
import { Equal, Expect, ExpectExtends, ExpectFalse } from "@type-challenges/utils";
const tc1 = "19-0";
const tc2 = "5-11";
const tc3 = "9-1";
const tc4 = "2-8";
const tc5 = "7-2";
type TestCases = [
ExpectFalse<ExpectExtends<Score, typeof tc1>>,
ExpectFalse<ExpectExtends<Score, typeof tc2>>,
Expect<ExpectExtends<Score, typeof tc3>>,
Expect<ExpectExtends<Score, typeof tc4>>,
Expect<ExpectExtends<Score, typeof tc5>>
];
βοΈλ΅μ
type Score = `${Int}-${Int}`;
type Int = '1'|'2'|'3'|'4'|'5'|'6'|'7'|'8'|'9'|'10';
μ½λλ§ν¬