MVC 모델
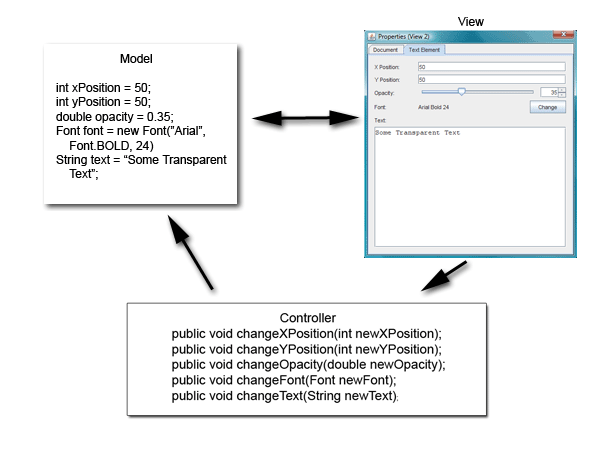
- 소프트웨어 설계에서 세 가지 구성 요소인 모델(Model), 뷰(View), 컨트롤러(Controller)를 이용한 설계 방식
구성요소
Model 모델
- 소프트웨어 내 데이터
- The model represents data and the rules that govern access to and updates of this data. In enterprise software, a model often serves as a software approximation of a real-world process. (Oracle)
- 데이터 및 데이터 접근과 업데이트에 관한 규칙을 나타냄
- 기업형 소프트웨어에서 주로 현실에서의 과정을 소프트웨어적으로 비슷하게 풀어낸 것
- Model represents an object or JAVA POJO carrying data. It can also have logic to update controller if its data changes. (tutorialspoint)
- 객체 즉 데이터를 갖고 있는 POJO(Plain Old Java Object, 오래된 방식의 간단한 자바 오브젝트. 비종속적)를 나타냄
- 데이터가 바뀌면 컨트롤러를 업데이트할 수 있는 로직을 가지고 있음.
View 뷰
- 사용자에게 보이는 화면 내용=UI
- The view renders the contents of a model. It specifies exactly how the model data should be presented. If the model data changes, the view must update its presentation as needed. This can be achieved by using a push model, in which the view registers itself with the model for change notifications, or a pull model, in which the view is responsible for calling the model when it needs to retrieve the most current data. (Oracle)
- 모델의 컨텐츠를 보여주는 역할.
- 모델의 데이터가 바뀌면 뷰의 모습도 함께 바뀌어야함
- push model, pull model?
- View represents the visualization of the data that model contains. (tutorialspoint)
- 모델이 가진 데이터를 시각적으로 보여주는 역할
Controller 컨트롤러
- 모델과 뷰의 상호 작용을 관리
- The controller translates the user's interactions with the view into actions that the model will perform. In a stand-alone GUI client, user interactions could be button clicks or menu selections, whereas in an enterprise web application, they appear as GET and POST HTTP requests. Depending on the context, a controller may also select a new view -- for example, a web page of results -- to present back to the user. (Oracle)
- 독립적 GUI 클라이언트: 버튼을 클릭하거나 메뉴를 고르는 것
- 기업형 웹 어플리케이션: GET, POST HTTP 리퀘스트
- 상황에 따라 컨트롤러는 새로운 뷰를 만들 수 있다
- Controller acts on both model and view. It controls the data flow into model object and updates the view whenever data changes. It keeps view and model separate. (tutorialspoint)
순서
- 사용자의 입력이 컨트롤러로 들어옴
- 컨트롤러는 모델에서 데이터를 불러옴
- 해당 데이터를 뷰를 통해 화면으로 출력함
특징
- 모델, 뷰, 컨트롤러가 기능별로 독립적으로 분리
- 여러 명의 개발자가 동시에 각각의 기능 개발 가능
- 일부 기능이 추가될 경우도 기존의 구성요소를 재사용 가능 (코드 재사용성 향상)
예시
- 웹 애플리케이션에 쉽게 적용할 수 있는 라이브러리 예시
- AngularJS (앵귤러자바스크립트)
- EmberJS (엠버자바스크립트)
- JavaScriptMVC
- BackboneJS
변형 형태
- MVC 유형에서 뷰와 모델은 정확히 분리되지만, 컨트롤러가 뷰의 구성에 상당 부분 관여하는 경우가 있어 각각의 독립성이 다소 떨어지는 문제가 발생할 수 있다.
- 이를 해결하기 위해 MVP와 MVVM 등장!
MVP (Model-View-Presenter)
- 컨트롤러의 뷰 구성기능은 모두 뷰 자체에 맡기고 프리젠터(presenter)가 모델과 뷰 사이의 인터페이스 역할만을 하도록 하는 것
MVVM (Model-View-ViewModel)
- 데이터 바인딩 기반으로 뷰가 뷰모델과 인터페이스하도록 제한을 두는 것
구현
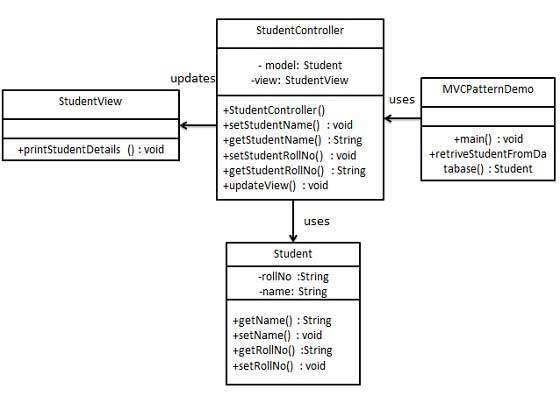
1단계: Create Model.
Student.java
public class Student {
private String rollNo;
private String name;
public String getRollNo() {
return rollNo;
}
public void setRollNo(String rollNo) {
this.rollNo = rollNo;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
2단계: Create View.
StudentView.java
public class StudentView {
public void printStudentDetails(String studentName, String studentRollNo){
System.out.println("Student: ");
System.out.println("Name: " + studentName);
System.out.println("Roll No: " + studentRollNo);
}
}
3단계: Create Controller.
StudentController.java
public class StudentController {
private Student model;
private StudentView view;
public StudentController(Student model, StudentView view){
this.model = model;
this.view = view;
}
public void setStudentName(String name){
model.setName(name);
}
public String getStudentName(){
return model.getName();
}
public void setStudentRollNo(String rollNo){
model.setRollNo(rollNo);
}
public String getStudentRollNo(){
return model.getRollNo();
}
public void updateView(){
view.printStudentDetails(model.getName(), model.getRollNo());
}
}
4단계: Use the StudentController methods to demonstrate MVC design pattern usage.
MVCPatternDemo.java
public class MVCPatternDemo {
public static void main(String[] args) {
Student model = retriveStudentFromDatabase();
StudentView view = new StudentView();
StudentController controller = new StudentController(model, view);
controller.updateView();
controller.setStudentName("John");
controller.updateView();
}
private static Student retriveStudentFromDatabase(){
Student student = new Student();
student.setName("Robert");
student.setRollNo("10");
return student;
}
}
5단계: Verity the output.
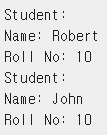
MVC 설명 출처
싱글톤 패턴
- Singleton
- 정확히 하나의 요소만 갖는 집합
- 단독 개체, 독신자
- Singleton Pattern
- 객체의 생성과 관련된 패턴
- 특정 클래스의 객체가 오직 한 개만 존재하도록 보장
- 클래스의 객체를 하나로 제한
- one of the simplest design patterns in Java
- comes under creational pattern as this pattern provides one of the best ways to create an object
- involves a single class which is resposible to create an object while making sure that only single object gets created.
- This class provides a way to access its only object which can be accessed directly without need to instantiate the object of the class. (이 클래스는 인스턴스화를 하지않고도 객체에 접근할 수 있는 경로를 제공해준다.)
예시
- 프린터 드라이버
- 여러 컴퓨터에서 프린터 한대를 공유하는 경우
- 하나를 끝내고 다음 작업을 하는 것이 아닌 동시에 섞여 나오면 안될 것임.
- Singleton 패턴: 여러 클라이언트(컴퓨터)가 동일 객체(공유 프린터)를 사용하지만 한 개의 객체(프린트 명령을 받은 출력물)가 유일하도록 상위 객체가 보장하도록 하는 것
- 동일한 자원이나 데이터를 처리하는 객체가 불필요하게 여러 개 만들어질 필요가 없는 경우에 주로 사용
구현
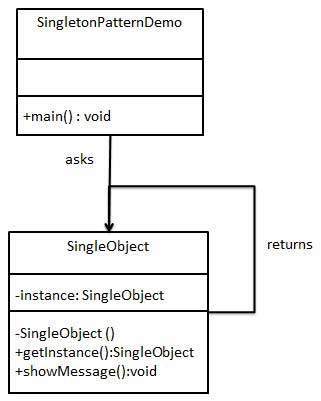
1단계: Create a Singleton Class.
SingleObject.java
public class SingleObject {
private static SingleObject instance = new SingleObject();
private SingleObject(){}
public static SingleObject getInstance(){
return instance;
}
public void showMessage(){
System.out.println("Hello World!");
}
}
2단계: Get the only object from the singleton class.
SingletonPatternDemo.java
public class SingletonPatternDemo {
public static void main(String[] args) {
SingleObject object = SingleObject.getInstance();
object.showMessage();
}
}
3단계: Verify the output.
- Hello World! 라고 출력창에 뜰 것임
싱글톤패턴 설명 출처