선택자 필터
- 기본 선택자보다 더 정밀한 선택이 필요한 경우 필터와 선택 메서드를 활용
- 형식: "선택자:필터"
- 사용 방법
- 1) 선택자로 기준 엘리먼트를 먼저 찾는다
- 2) 필터는 이 엘리먼트 주변의 다른 엘리먼트나 선택된 집합 중의 일부를 더 정밀하게 선택하는 역할을 한다
기본 필터
종류
:first
:last
:even
:odd
- :eq(index)
- 선택요소 중 주어진 index에 일치하는 index를 갖는 요소
:gt(index)
$("li:gt(3))
▶ $("li").slice(4)
:lt(index)
$("li:lt(4))
▶ $("li").slice(0,3)
- :not(selector)
- 괄호의 selector와 일치하는 모든 요소를 제외
자식 필터
- 요소를 자식으로 갖는 부모의 그룹 안에서 해당 자식을 찾는다
$("td:first-child")
- td를 자식으로 갖는 tr(부모)그룹에서 첫번째 자식
종류
- :first-child
- :last-child
- :nth-child(index/even/odd/equation)
- :only-child
- 자신이 부모요소의 유일한 자식요소인 것과 일치
예제: 201008Ex02
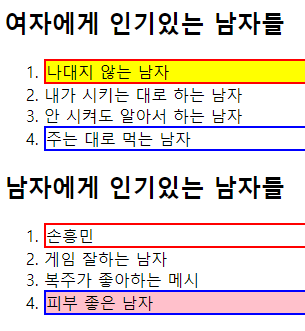
- first(), last() : 모든 요소 통틀어서 첫번째 (딱1개)
- :fisrt-child, :last-child : 각 부모별 요소중 첫번째 애들 (여러개 될 수 있음)
js
$("#ex1dv li").first().css("background-color","yellow");
$("#ex1dv li:first-child").css("border","2px solid red");
$("#ex1dv li").last().css("background-color","pink");
$("#ex1dv li:last-child").css("border","2px solid blue");
html
<h2>여자에게 인기있는 남자들</h2>
<ol>
<li>나대지 않는 남자</li>
<li>내가 시키는 대로 하는 남자</li>
<li>안 시켜도 알아서 하는 남자</li>
<li>주는 대로 먹는 남자</li>
</ol>
내용 필터
종류
- :contains(text)
- 지정한 text를 포함하는 모든 요소를 반환(대소문자 구분)
-$("p:contains(jQuery)").css(...);
-$("body *:contains(jQuery)").css(...);
-$("body :contains(jQuery)").css(...);
:has(selector)
- .has(selector)
- For better performance in modern browsers, use
$( "your-pure-css-selector" ).has( selector/DOMElement )
instead.
- 지정한 selector를 포함하고 있는 모든 요소 반환
-$("div:has(p, span)").css(...);
- p, span 모두 있어야하는게 아닌 하나라도 있으면 됨
- :empty
- 자식요소도 없고 텍스트도 없는 모든 요소 반환
- :parent
- 자식요소 또는 텍스트를 갖고 있는 모든 요소를 반환
- To obtain the parents or ancestors of an existing jQuery set, see the
.parent()
and .parents()
methods.
예제: 201012Ex03
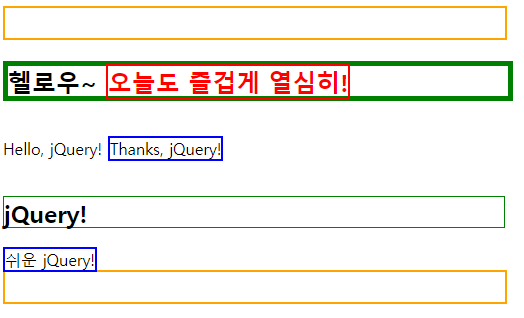
js
<script>
$(document).ready(function() {
$("span:contains(jQuery)").css("border","2px solid blue");
$("span:not(:contains(jQuery))".css("border","2px solid red");
$("div").has("span").css("border","5px solid green");
$("div:empty").css("border","2px solid orange");
$("div span").css("color","red");
$("div:parent").css("font-weight","bold").css("font-size","1.5em");
});
</script>
<style>
div {
width: 500px;
height: 30px;
border: 1px solid green;
}
</style>
html
<body>
<div></div><br>
<div>헬로우~
<span>오늘도 즐겁게 열심히!</span>
</div><br>
<p>Hello, jQuery!
<span>Thanks, jQuery!</span>
</p><br>
<div>jQuery!</div><br>
<span>쉬운 jQuery!</span><br>
<div></div><br>
</body>
가시성 상태 필터
종류
- :hidden
.filter(":hidden")
- 숨겨진 요소를 선택
$("p:hidden").show("5000");
- 대상: 숨겨진 요소의 유형(작동하는 요소 유형)
- display: none
- 폼 요소중 type='hidden'
- width와 height가 0으로 설정
- 부모 요소가 hidden
- 작동 안할 때:
visibility: hidden
opacity: 0
- :visible
- 보이는 요소를 선택
- 대상: 숨겨진 요소가 아닌 것
예제1 201012Ex04
js
$("#ex1dv button").click(function() {
if($(this).html().indexOf("hidden") > -1) {
$("#ex1dv :hidden").each(function() {
alret($(this).html());
});
} else {
$("#ex1dv :visible").each(function() {
alret($(this).html());
});
}
});
html
<div id="ex1dv">
<h3>예제1 - 가시성 필터1</h3>
<p>보이는 문단</p>
<p style="visibility: hidden;">숨겨진 문단</p>
<a style="display: none">히든 링크</a>
<input type="hidden" value="히든 밸류">
<p style="display: none;">자리를 차지 하지 못한 문단</p>
<p style="opacity: 0;">투명한 문단</p>
<hr />
<button id="hidden">hidden요소</button> <button id="visible">visible요소</button>
</div>
예제2 201012Ex04
js
$("#form2 :button").click(function() {
if($(this).val()=="hidden요소"){
var str = "";
$("#form2 :hidden").each(function() {
str += "태그 : " + $(this).prop("tagName") +
" / 유형 : " + this.type +
" / name : " + $(this).attr("name") +
" / value : " + $(this).val() +
" / html : " + $(this).html() + "\n";
});
alert(str);
} else {
var str = "";
$("#form2 :visible").each(function() {
str += "태그 : " + $(this).prop("tagName") +
" / 유형 : " + this.type +
" / name : " + $(this).attr("name") +
" / value : " + $(this).val() +
" / html : " + $(this).html() + "\n";
});
alert(str);
}
});
html
<div id="ex2dv">
<h3>예제2 - 가시성 필터2</h3>
<form id="form2">
<input type="hidden" name="userAge" value="33"/>
<input type="hidden" name="userCity" value="대전"/>
ID : <input type="text" name="userID" value="hong"/>
<span style="display:none">ID가 중복됩니다</span><br>
이름 : <input type="text" name="userName" value="홍길동"/>
<span style="visibility:hidden">이름을 입력하세요</span><br>
성별 : <input type="radio" name="gender" value="남" id="male"/><label for="male">남자</label>
<input type="radio" name="gender" value="여" id="female"/><label for="female">여자</label><br>
<input type="button" value="hidden요소"/>
<input type="button" value="visible요소"/>
</form>
</div>
종류
- :enabled
- :disabled
- :selected
- :checked
예제 201012Ex05
js
$("#ex2dv :button").click(function() {
var str = "";
$(":checkbox:checked","#form2").each(function() {
str += $(this).val() + ", ";
});
str = str.substr(0,str.length-2);
$("#result").html(str);
});
html
<div id="ex2dv">
<h3>예제2 - checked</h3>
<form action="" id="form2">
취미 :
<input type="checkbox" value="여행"/>여행
<input type="checkbox" value="장기"/>장기
<input type="checkbox" value="바둑"/>바둑
<input type="checkbox" value="독서"/>독서
<input type="checkbox" value="낚시"/>낚시
<br><br>
<input type="button" value="전송" />
<br><br>
선택 결과 : <span id="result"></span>
</form>
</div>
기타 필터