📌 홀짝게임
코드
**import sys
from PyQt5.QtWidgets import *
from PyQt5 import uic
from dask.array.random import randint
form_class = uic.loadUiType("myQt05.ui")[0]
class WindowClass(QMainWindow, form_class) :
def __init__(self) :
super().__init__()
self.setupUi(self)
self.pb.clicked.connect(self.oddEven)
self.qle1.returnPressed.connect(self.oddEven)
def oddEven(self) :
User = self.qle1.text()
com = self.comRand()
if(User == com):
result = "User 승리"
else:
result = "Com 승리"
self.qle2.setText(com)
self.qle3.setText(result)
def comRand(self):
com = ""
rnd = randint(0,2)
if(rnd == 0):
com = "짝"
else:
com = "홀"
return com
if __name__ == "__main__" :
app = QApplication(sys.argv)
myWindow = WindowClass()
myWindow.show()
app.exec_()**
설명
**
self.pb.clicked.connect(self.oddEven)
self.qle1.returnPressed.connect(self.oddEven)**
**def comRand(self):
com = ""
rnd = randint(0,1+1)
if(rnd == 0):
com = "짝"
else:
com = "홀"
return com**
- 랜덤 함수를 이용해 컴퓨터의 홀 짝을 생성하는 함수
- 홀 짝 판별 함수에 호출되어 사용된다.
- randInt(start,end+1) : start부터 end 사이의 랜덤한 정수를 반환하는 함수
- randInt(0,2) : 0 ~ 1까지 랜덤 정수를 반환함
- 직관성을 높이기 위해 randInt(0,1+1)로 표현
**
def oddEven(self) :
User = self.qle1.text()
com = self.comRand()
if(User == com):
result = "User 승리"
else:
result = "Com 승리"
qle2, qle3에 출력
self.qle2.setText(com)
self.qle3.setText(result)**
실행 결과
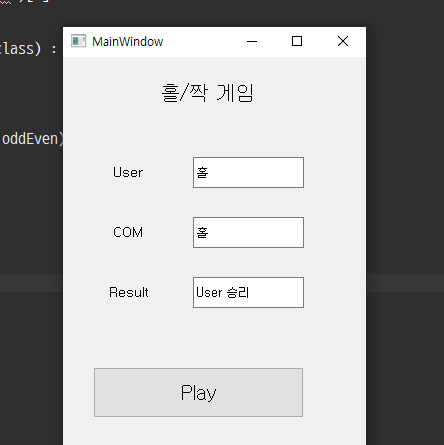
📌 로또 번호 생성
코드
import sys
from PyQt5.QtWidgets import *
from PyQt5 import uic
import secrets
form_class = uic.loadUiType("myQt06.ui")[0]
class WindowClass(QMainWindow, form_class):
def __init__(self):
super().__init__()
self.setupUi(self)
self.pb.clicked.connect(self.createRotto)
def createRotto(self):
num = []
for i in range(1, 46):
num.append(i)
rotto = []
for i in range(0, 7):
rotto.append(secrets.choice(num))
rottoSet = list(set(rotto))
rottoSet.sort()
self.lbl1.setText(str(rottoSet[0]))
self.lbl2.setText(str(rottoSet[1]))
self.lbl3.setText(str(rottoSet[2]))
self.lbl4.setText(str(rottoSet[3]))
self.lbl5.setText(str(rottoSet[4]))
self.lbl6.setText(str(rottoSet[5]))
num.clear()
rotto.clear()
rottoSet.clear()
if __name__ == "__main__":
app = QApplication(sys.argv)
myWindow = WindowClass()
myWindow.show()
app.exec_()
설명
def createRotto(self):
num = []
for i in range(1, 46):
num.append(i)
rotto = []
for i in range(0, 7):
rotto.append(secrets.choice(num))
rottoSet = list(set(rotto))
rottoSet.sort()
self.lbl1.setText(str(rottoSet[0]))
self.lbl2.setText(str(rottoSet[1]))
self.lbl3.setText(str(rottoSet[2]))
self.lbl4.setText(str(rottoSet[3]))
self.lbl5.setText(str(rottoSet[4]))
self.lbl6.setText(str(rottoSet[5]))
num.clear()
rotto.clear()
rottoSet.clear()
- 리스트
- num = [] :리스트를 생성
- num.append(0) : 리스트 0번째에 0 이 담김
- num.sort() : 오름차순으로 정렬
- num[0] : 0번째 데이터
- num.clear() : 리스트 비우기
실행 결과
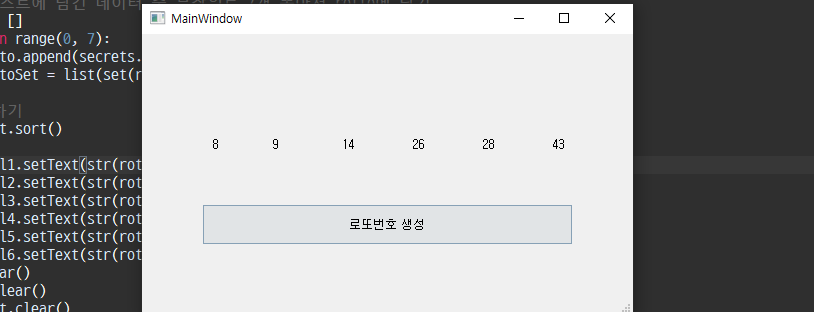
📌 가위바위보
코드
import sys
from PyQt5.QtWidgets import *
from PyQt5 import uic
import secrets
form_class = uic.loadUiType("myQt07.ui")[0]
class WindowClass(QMainWindow, form_class):
def __init__(self):
super().__init__()
self.setupUi(self)
self.pb.clicked.connect(self.rsp)
self.qle1.returnPressed.connect(self.rsp)
def rsp(self) :
user = self.qle1.text()
comList = ["가위", "바위", "보"]
com = secrets.choice(comList)
if(user == com):
result = "비김"
elif(user == "가위" and com == "보"
or user == "바위" and com == "가위"
or user == "보" and com == "바위"):
result = "User 승리"
else:
result = "COM 승리"
self.qle2.setText(com)
self.qle3.setText(result)
if __name__ == "__main__":
app = QApplication(sys.argv)
myWindow = WindowClass()
myWindow.show()
app.exec_()
설명
import sys
from PyQt5.QtWidgets import *
from PyQt5 import uic
import secrets
form_class = uic.loadUiType("myQt07.ui")[0]
class WindowClass(QMainWindow, form_class):
def __init__(self):
super().__init__()
self.setupUi(self)
self.pb.clicked.connect(self.rsp)
self.qle1.returnPressed.connect(self.rsp)
def rsp(self) :
user = self.qle1.text()
comList = ["가위", "바위", "보"]
com = secrets.choice(comList)
if(user == com):
result = "비김"
elif(user == "가위" and com == "보"
or user == "바위" and com == "가위"
or user == "보" and com == "바위"):
result = "User 승리"
else:
result = "COM 승리"
self.qle2.setText(com)
self.qle3.setText(result)
if __name__ == "__main__":
app = QApplication(sys.argv)
myWindow = WindowClass()
myWindow.show()
app.exec_()
설명
def rsp(self) :
user = self.qle1.text()
comList = ["가위", "바위", "보"]
com = secrets.choice(comList)
if(user == com):
result = "비김"
elif(user == "가위" and com == "보"
or user == "바위" and com == "가위"
or user == "보" and com == "바위"):
result = "User 승리"
else:
result = "COM 승리"
self.qle2.setText(com)
self.qle3.setText(result)
- secrets.choice(데이터 집합) : 데이터 집합 안에서 무작위 데이터 1개를 리턴한다.
- comList에 “가위”, “바위”, “보”를 담고 secrets.choice(comList)를 하면 셋 중 한개 값 반환
실행 결과