조건문(if문, if~else문, elif문)
- 조건문을 이용해서 프로그램의 실행을 분기한다.
1. 교통 과속 위반 프로그램을 만들어보자
carSpeed = int(input('속도 입력: '))
limitSpeed = 50
if carSpeed > 50:
print('안전속도 위반! 과태료 50,000원 부과 대상!')
if carSpeed <= 50:
print('안전속도 준수!')
2. 문자 메시지 길이에 따라 문자 요금이 결정되는 프로그램을 만들어보자
message = input('메시지 입력: ')
lenMessage = len(message)
msgPrice = 50
if lenMessage <= 50:
msgPrice = 50
print('SMS 발송!')
else:
msgPrice = 100
print('MMS 발송!')
print('메시지 길이: {}'.format(lenMessage))
print('메시지 발송요금: {}'.format(msgPrice))
3. 국어, 영어, 수학, 과학, 국사 점수를 입력하면 총점을 비롯한 각종 데이터가 출력되는 프로그램을 만들어보자
- 과목별 점수를 입력하면 총점, 평균, 편차를 출력한다.
- 평균은 다음과 같다.
(국어: 85, 영어:82, 수학: 89, 과학: 75, 국사: 94)
- 각종 편자 데이터는 막대그래프로 시각화한다.
korAvg = 85; engAvg = 82; matAvg = 89
sciAvg = 75; hisAvg = 94
totalAvg = korAvg + engAvg + matAvg + sciAvg + hisAvg
avgAvg = int(totalAvg / 5)
korScore = int(input('국어점수: '))
engScore = int(input('영어점수: '))
matScore = int(input('수학점수: '))
sciScore = int(input('과학점수: '))
hisScore = int(input('국사점수: '))
totalScore = korScore + engScore + matScore + sciScore + hisScore
avgScore = int(totalScore / 5)
korGap = korScore - korAvg
engGap = engScore - engAvg
matGap = matScore - matAvg
sciGap = sciScore - sciAvg
hisGap = hisScore - hisAvg
totalGap = totalScore - totalAvg
avgGap = avgScore - avgAvg
print('-' * 70)
print('총점: {}({}), 평균: {}({})'.format(totalScore, totalGap, avgScore, avgGap))
print('국어: {}({}), 영어: {}({}), 수학: {}({}), 과학: {}({}), 국사: {}({})'.format(
korScore, korGap, engScore, engGap, matScore, matGap, sciScore, sciGap, hisScore, hisGap
))
print('-' * 70)
#그래프
str = '+' if korGap > 0 else '-'
print('국어 편차: {}({})'.format(str * abs(korGap), korGap)) #abs = 절대값
str = '+' if engGap > 0 else '-'
print('영어 편차: {}({})'.format(str * abs(engGap), engGap)) #abs = 절대값
str = '+' if matGap > 0 else '-'
print('수학 편차: {}({})'.format(str * abs(matGap), matGap)) #abs = 절대값
str = '+' if sciGap > 0 else '-'
print('과학 편차: {}({})'.format(str * abs(sciGap), sciGap)) #abs = 절대값
str = '+' if hisGap > 0 else '-'
print('국사 편차: {}({})'.format(str * abs(hisGap), hisGap)) #abs = 절대값
str = '+' if totalGap > 0 else '-'
print('총점 편차: {}({})'.format(str * abs(totalGap), totalGap)) #abs = 절대값
str = '+' if avgGap > 0 else '-'
print('평균 편차: {}({})'.format(str * abs(avgGap), avgGap)) #abs = 절대값
print('-' * 70)
-->
국어점수: 95
영어점수: 81
수학점수: 100
과학점수: 51
국사점수: 67
----------------------------------------------------------------------
총점: 394(-31), 평균: 78(-7)
국어: 95(10), 영어: 81(-1), 수학: 100(11), 과학: 51(-24), 국사: 67(-27)
----------------------------------------------------------------------
국어 편차: ++++++++++(10)
영어 편차: -(-1)
수학 편차: +++++++++++(11)
과학 편차: ------------------------(-24)
국사 편차: ---------------------------(-27)
총점 편차: -------------------------------(-31)
평균 편차: -------(-7)
----------------------------------------------------------------------
4. 난수를 이용해서 홀/짝 게임을 만들어보자
import random
comNum = random.randint(1, 2)
useselect = int(input('홀/짝 선택: 1. 홀 \t 2. 짝'))
if comNum == 1 and useselect == 1:
print('빙고! 홀수!')
elif comNum == 2 and useselect == 2:
print('빙고! 짝수!')
elif comNum == 1 and useselect == 2:
print('실패! 홀수!')
elif comNum == 2 and useselect == 1:
print('실패! 홀수!')
5. 난수를 이용해서 가위, 바위, 보 게임을 만들어보자
import random
comNum = random.randint(1, 3)
userNum = int(input('가위, 바위, 보 선택: 1. 가위 \t 2. 바위 \t 3. 보'))
if (comNum == 1 and userNum == 2) or \
(comNum == 2 and userNum == 3) or \
(comNum == 3 and userNum == 1):
print('컴퓨터: 패, 유저: 승')
elif comNum == userNum:
print('무승부')
else:
print('컴퓨터: 승, 유저: 패')
print('컴퓨터: {}, 유저: {}'.format(comNum, userNum))
-->
가위, 바위, 보 선택: 1. 가위 2. 바위 3. 보3
컴퓨터: 패, 유저: 승
컴퓨터: 2, 유저: 3
6. 아래 요금표를 참고해서 상수도 요금 계산기를 만들어보자
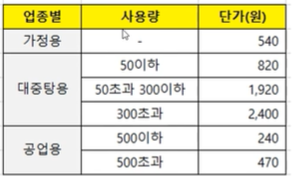
part = int(input('업종 선택(1. 가정용 \t 2. 대중탕용 \t 3. 공업용)'))
userWater = int(input('사용량 입력: '))
unitPrice = 0
if part == 1:
unitPrice = 540
elif part == 2:
if userWater <= 50:
unitPrice = 820
elif userWater > 50 and userWater <= 300:
unitPrice = 1920
elif userWater > 300:
unitPrice = 2400
elif part == 3:
if userWater <= 500:
unitPrice = 240
else:
unitPrice = 470
print('=' * 30)
print('상수도 요금표')
print('-' * 30)
print('사용량 \t : \t 요금')
userPrice = userWater * unitPrice
print('{} \t : \t {}원'.format(userWater, format(userPrice, ',')))
-->
업종 선택(1. 가정용 2. 대중탕용 3. 공업용)3
사용량 입력: 900
==============================
상수도 요금표
------------------------------
사용량 : 요금
900 : 423,000원
7. 미세먼지 비상저감조치로 차량 운행제한 프로그램을 다음 내용에 맞게 만들어보자
- 미세먼지 측정수치가 150이하면 차량 5부제 실시
- 미세먼지 측정수치가 150을 초과하면 차량 2부제 실시
- 차량 2부제를 실시하더라도 영업용차량은 5부제 실시
- 미세먼지 수치, 차량종류, 차량번호를 입력하면 운행가능여부 출력
import datetime
today = datetime.datetime.today()
day = today.day
limitDust = 150
dustNum = int(input('미세먼지 수치 입력: '))
carType = int(input('차량종류선택: 1. 승용차 2. 영업용차'))
carNumber = int(input('차량번호입력: '))
print('-' * 30)
print(today)
print('-' * 30)
if dustNum > limitDust and carType == 1:
if (day % 2) == (carNumber % 2): # 2부제는 짝수 홀수
print('차량 2부제 적용')
print('차량 2부제로 금일 운행제한 대상 차량입니다.')
else:
print('금일 운행 가능합니다.')
if dustNum > limitDust and carType == 2:
if (day % 5) == (carNumber % 5): #2부제는 짝수 홀수
print('차량 5부제 적용')
print('차량 5부제로 금일 운행제한 대상 차량입니다.')
else:
print('금일 운행 가능합니다.')
if dustNum <= limitDust:
if (day % 5) == (carNumber % 5): #2부제는 짝수 홀수
print('차량 5부제 적용')
print('차량 5부제로 금일 운행제한 대상 차량입니다.')
else:
print('금일 운행 가능합니다.')
-->
미세먼지 수치 입력: 155
차량종류선택: 1. 승용차 2. 영업용차1
차량번호입력: 7468
------------------------------
2023-10-18 16:05:10.870226
------------------------------
차량 2부제 적용
차량 2부제로 금일 운행제한 대상 차량입니다.
8. pc에서 난수를 발생하면 사용자가 맞추는 게임을 만들어보자
- pc가 난수(1~1000)를 발생하면 사용자는 숫자(정수)를 입력한다
- 사용자가 난수를 맞추면 게임이 종료된다
- 만약 못 맞추게 되면 난수와 사용자 숫자의 크고 작음을 출력한 후 사용자에게 다시 기회를 준다
- 최종적으로 시도한 횟수를 출력한다
import random
rNum = random.randint(1, 1000)
tryCount = 0
gameFlag = True
while gameFlag:
tryCount += 1
pNum = int(input('1에서 1000까지의 정수입력: '))
if rNum == pNum:
print('빙고!')
gameFlag = False
else:
if rNum > pNum:
print('난수가 크다!')
else:
print('난수가 작다!')
print('난수: {}, 시도횟수: {}'.format(rNum, tryCount))
9. 실내온도를 입력하면 스마트에어컨 상태가 자동으로 설정되는 프로그램을 만들어보자
innerTemp = int(input('실내 온도 입력: '))
if innerTemp <= 18:
print('에어컨: OFF')
elif innerTemp > 18 and innerTemp <= 22:
print('에어컨: 매우 약')
elif innerTemp > 22 and innerTemp <= 24:
print('에어컨: 약')
elif innerTemp > 24 and innerTemp <= 26:
print('에어컨: 중')
elif innerTemp > 26 and innerTemp <= 28:
print('에어컨: 강')
elif innerTemp > 28:
print('에어컨: 매우 강')