๐ Map ํน์ง
key=value
์์ผ๋ก ์ด๋ฃจ์ด์ ธ ์๋ค.
- ์์๊ฐ ์๋ค.
- ์ค๋ณต๋ key๋ฅผ ์ ์ฅํ ์ ์๋ค.
- ์ธ๋ฑ์ค๊ฐ ์๋ค.
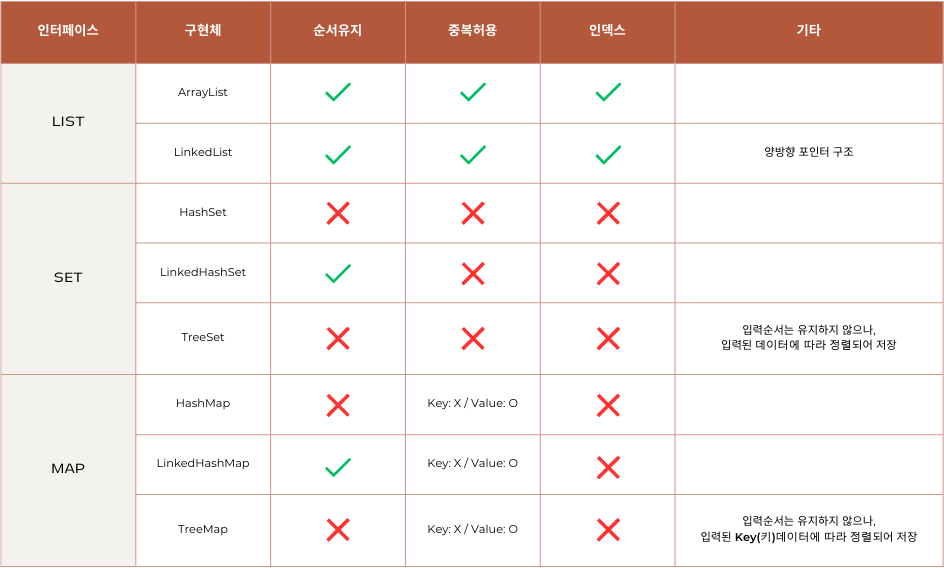
๐ Map ์์ฑ
- ๋น Map ๋ง๋๋ ๋ฐฉ๋ฒ: new HashMap()
- ์ด๊ธฐ ์์๋ฅผ ํฌํจํ Map ๋ง๋๋ ๋ฐฉ๋ฒ: new HashMap() {{ put(key1, value1); put(key2, value2); ... }}
package lesson10;
import java.util.HashMap;
import java.util.Map;
public class Ex01 {
public static void main(String[] args) {
Map<String, Integer> map1 = new HashMap<>();
System.out.println(map1);
Map<String, Integer> map2 = new HashMap<>() {{
put("a", 1);
put("b", 2);
}};
System.out.println(map2);
}
}
๐ ์ถ๊ฐ/์ญ์ /์์ /์ฝ๊ธฐ
| List | Set | Map |
---|
์ถ๊ฐ | add(), addAll() | add(), addAll() | put(), putAll() |
์ญ์ | remove(), removeAll(), clear() | remove(), removeAll(), clear() | remove(), keySet().removeAll(), values().removeAll(), clear() |
์์ | set() | ์์ ์ญ์ ํ ์ถ๊ฐ | ๋์ผํ key๋ก ๋ค์ ์ถ๊ฐ |
์ฝ๊ธฐ | get() | ๋ฐ๋ณต๋ฌธ(Iterator, ํฅ์๋ for๋ฌธ) ์ฌ์ฉ | get() |
- ์ถ๊ฐ: put(), putAll()
- ์ญ์ : remove(),
keySet().removeAll()
, values().removeAll()
, clear()
- ์์ : ๋์ผํ key๋ก ๋ค์ ์ถ๊ฐ
- ์ฝ๊ธฐ: get()
keySet(), values() ์ฃผ์ํ ์
- map.keySet()์์ ์ญ์ ํ๋ฉด ์๋ณธ์์๋ ์ญ์ ๋๋ค.
- map.values()์์ ์ญ์ ํ๋ฉด ์๋ณธ์์๋ ์ญ์ ๋๋ค.
package lesson10;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class Ex01 {
public static void main(String[] args) {
Map<String, Integer> map1 = new HashMap<>();
Map<String, Integer> map2 = new HashMap<>() {{
put("a", 1);
put("b", 2);
put("c", 2);
}};
map1.put("A", 1);
map1.put("B", 2);
map1.put("C", 3);
map1.put("D", 3);
map1.put("E", 5);
map1.put("F", 6);
System.out.println(map1);
map1.putAll(map2);
System.out.println(map1);
map1.remove("a");
System.out.println(map1);
map1.remove("B", 2);
System.out.println(map1);
map1.keySet().removeAll(List.of("A", "E"));
System.out.println(map1);
map1.values().removeAll(List.of(3, 6));
System.out.println(map1);
map1.clear();
System.out.println(map1);
System.out.println(map2);
map2.put("b", 20);
System.out.println(map2);
int value = map2.get("a");
System.out.println(value);
}
}
๐ Map ์ธํฐํ์ด์ค ๋ฉ์๋
๋ฉ์๋๋ช
| ์ค๋ช
| ๋ฐํ๊ฐ |
---|
keySet() | key๋ค์ ๋ชจ์์ Set ์๋ฃํ์ผ๋ก ๋ฐํ | Set |
values() | ๊ฐ๋ค์ ๋ชจ์์ Collection ์๋ฃํ์ผ๋ก ๋ฐํ | Collection |
containsKey(๊ฐ) | key๊ฐ ์๋์ง ํ์ธ | boolean |
containsValue(๊ฐ) | value๊ฐ ์๋์ง ํ์ธ | boolean |
isEmpty() | Map์ด ๋น์ด์๋์ง ํ์ธ | boolean |
size() | Map์ ํฌ๊ธฐ | int |
toString() | Map์ ๋ชจ๋ ์์๋ฅผ {ํค=๊ฐ, ํค=๊ฐ...}์ ๋ฌธ์์ด ํํ๋ก ๋ฐํ | String |