simplevector.h
#ifndef SIMPLE_VECTOR
#define SIMPLE_VECTOR
#include <algorithm>
#include <initializer_list>
#include <stdexcept>
template <typename T>
class SimpleVector {
private:
T* data;
int currentSize;
int currentCapacity;
protected:
void resize(int newCapacity);
public:
//기본 생성자
SimpleVector() : currentSize(0), currentCapacity(10), data(new T[10]) {}
// 매개변수 생성자
SimpleVector(int capacity) : currentSize(0), currentCapacity(capacity), data(new T[capacity]) {}
// 복사 생성자
SimpleVector(const SimpleVector<T>& other)
: currentCapacity(other.currentCapacity),
currentSize(other.currentSize),
data(new T[other.currentCapacity]) {
for (int i = 0; i < currentSize; ++i) {
data[i] = other.data[i];
}
}
// 이니셜라이저_리스트 생성자 { }
SimpleVector(std::initializer_list<T> list) : currentSize(list.size()), currentCapacity(list.size()), data(new T[list.size()]) {
int i = 0;
for (const auto& val : list){
data[i++] = val;
}
}
// ~소멸자
~SimpleVector() {
delete[] data;
}
// 멤버함수 선언
void push_back(const T& value);
void pop_back();
size_t size() const; // 어짜피 양수만 나오므로
size_t capacity() const; // size_t가 적합
void sortData();
void sortDescending();
// 연산자 [] 오버로딩
T& operator[](int index) {
if (index >= currentSize || index < 0) {
throw std::out_of_range("Index out of range");
}
return data[index];
}
const T& operator[](int index) const {
if (index >= currentSize || index < 0) {
throw std::out_of_range("Index out of range");
}
return data[index];
}
};
// vector의 reserve 처럼 구현
template <typename T>
void SimpleVector<T>::resize(int newCapacity) {
if (newCapacity > currentCapacity){
T* newData = new T[newCapacity];
for (int i = 0; i < currentSize; ++i) {
newData[i] = data[i];
}
delete[] data;
data = newData;
currentCapacity = newCapacity;
}
}
// push_back, resize() 멤버함수를 통한 구현
/*
template <typename T>
void SimpleVector<T>::push_back(const T& value) {
if (currentSize >= currentCapacity) {
resize((currentCapacity == 0) ? 1 : (currentCapacity + 5));
}
data[currentSize++] = value;
}
*/
// push_back
template <typename T>
void SimpleVector<T>::push_back(const T& value) {
if (currentSize >= currentCapacity) {
int newcapacity = (currentCapacity == 0) ? 1 : (currentCapacity + 5);
T* newdata = new T[newcapacity];
for (int i = 0; i < currentSize; i++) {
newdata[i] = data[i];
}
delete[] data;
data = newdata;
currentCapacity = newcapacity;
}
data[currentSize] = value;
currentSize++;
}
// pop_back 원소 아예 제거하는 버전
/*
template <typename T>
void SimpleVector<T>::pop_back() {
int newSize = currentSize - 1;
T* newdata = new T[currentCapacity];
for (int i = 0; i < newSize; i++) {
newdata[i] = data[i];
}
delete[] data;
data = newdata;
currentSize = newSize;
}
*/
// pop_back 배열 새로 안하고 size만 조절
template <typename T>
void SimpleVector<T>::pop_back() {
if (currentSize > 0) {
currentSize--;
}
}
// size
template <typename T>
size_t SimpleVector<T>::size () const {
return currentSize;
}
// capacity
template <typename T>
size_t SimpleVector<T>::capacity() const {
return currentCapacity;
}
// 정렬(오름차순)
template <typename T>
void SimpleVector<T>::sortData() {
std::sort(data, data + currentSize); // data(data[0]) <= x < data+currnetSize(data[currentSize])
}
// 정렬(내림차순)
template <typename T>
void SimpleVector<T>::sortDescending() {
std::sort(data, data + currentSize, std::greater<T>());
}
#endif
main.cpp
#include "simplevector.h"
#include <iostream>
#include <string>
using std::cin;
using std::string;
using std::cout;
using std::endl;
// 객체 정보 출력 함수 이것 때문에 연산자[] 오버로딩.
// size(), capacity() 작동 확인도 가능
template <typename T>
void printinfo(SimpleVector<T>& obj) {
cout << "size : " << obj.size() << endl
<< "capacity : " << obj.capacity() << endl;
for (int i = 0; i < obj.size() ; i++) {
cout << obj[i] << " ";
}
}
void seperateline() {
cout << endl;
for (int i = 0; i < 30; i++) {
cout << '-';
}
cout << endl;
}
int main() {
cout << "기본, 매개변수 생성자 작동 확인" << endl;
SimpleVector<int> vec1; // 기본 생성자 호출
cout << "capacity of vec1 : " << vec1.capacity() << endl; // 용량 확인
SimpleVector<int> vec2(20); // 매개변수 생성자 호출
cout << "capacity of vec1 : " << vec2.capacity() << endl; // 용량 확인
seperateline();
cout << "이니셜라이저, 복사 생성자 작동 확인" << endl;
SimpleVector<int> vec3 = { 5, 4, 2, 6, 7 ,9 ,1 ,3 ,8 ,10 }; // 이니셜라이저 생성자 호출
SimpleVector<int> vec4 = vec3; // 복사 생성자 호출;
printinfo(vec4);
seperateline();
cout << "push_back 동작 확인" << endl;
vec1.push_back(10); // push_back
vec1.push_back(20);
vec1.push_back(30);
vec1.push_back(40);
printinfo(vec1);
seperateline();
cout << "push_back 배열크기 증가 확인" << endl;
vec3.push_back(11); // push_back 배열 크기 5증가
printinfo(vec3);
seperateline();
cout << "pop_back 동작 확인" << endl;
vec1.pop_back(); // pop_back
vec1.pop_back();
printinfo(vec1);
seperateline();
cout << "정렬 확인(오름차순)" << endl;
vec3.sortData();
printinfo(vec3);
seperateline();
cout << "정렬 확인(내림차순)" << endl;
vec3.sortDescending();
printinfo(vec3);
seperateline();
// 여러가지 자료형 확인
SimpleVector<float> vecfloat = { 1.0, 12.4, 43.23, 12.8, 7.3 };
printinfo(vecfloat);
seperateline();
SimpleVector<string> vecstring = { "안녕", "하세요,", "Hello", "World"};
printinfo(vecstring);
seperateline();
return 0;
}
결과창
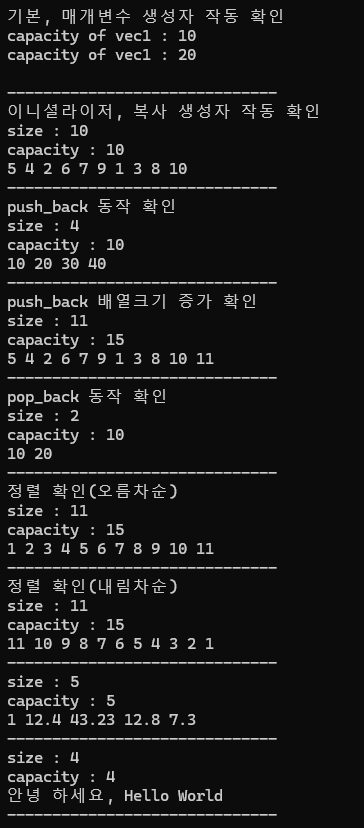