ch 14-17~22 스트림 만들기
스트림 만들기 - 컬렉션
- Collection인터페이스의 stream()으로 컬렉션을 스트림으로 변환
Stream<E> stream() // Collection인터페이스의 메서드
List<Integer> list = Arrays.asList(1,2,3,4,5);
Stream<Integer> intStream = list.stream(); // list를 스트림으로 변환
// 스트림의 모든 요소를 출력
intStream.forEach(System.out::print); // 12345
intStream.forEach(System.out::print); // 에러. 스트림이 이미 닫혔다.
스트림 만들기 - 배열
Stream<T> Stream.of(T... values) // 가변 인자
Stream<T> Stream.of(T[])
Stream<T> Arrays.stream(T[])
Stream<T> Arrays.stream(T[] array, int startInclusive, int endExclusive)
Strem<String> strStream = Stream.of("a","b","c"); // 가변 인자
Strem<String> strStream = Stream.of(new String[]{"a","b","c"});
Strem<String> strStream = Arrays.stream(new String[]{"a","b","c"});
Strem<String> strStream = Arrays.stream(new String[]{"a","b","c"}, 0, 3);
IntStream IntStream.of(int...values) // Stream이 아니라 IntStream
IntStream IntStream.of(int[])
IntStream Arrays.stream(int[])
IntStreamArrays.stream(int[] array, int startInclusive, int endExclusive)
스트림 만들기 - 임의의 수
IntStreamintStream = new Random().ints(); // 무한 스트림
intStream.limit(5).forEach(System.out::println); // 5개의 요소만 출력한다.
IntStream intStream = new Random().ints(5); // 크기가 5인 난수 스트림을 반환
Integer.MIN_VALUE <= ints() <= Integer.MAX_VALUE
Long.MIN_VALUE <= longs() <= Long.MAX_VALUE
0.0 <= doubles() < 1.0
- 지정된 범위의 난수를 요소로 갖는 스트림을 생성하는 메서드(Random클래스)
IntStream ints(int begin, int end) // 무한 스트림
LongStream longs(long begin, long end)
DoubleStream doubles(double begin, double end)
IntStream ints(long streamSize, int begin, int end) // 유한 스트림
LongStream longs(long StreamSize, long begin, long end)
DoubleStream doubles(long streamSize, double begin, double end)
스트림 만들기 - 특정 범위의 정수
- 특정 범위의 정수를 요소로 갖는 스트림 생성하기(IntStream, LongStream)
IntStream IntStream.range(int begin, int end)
IntStream IntStream.rangeClosed(int begin, int end)
IntStream intStream = IntStream.range(1, 5); // 1,2,3,4
IntStream intStream = IntStream.rangeClosed(1, 5); // 1,2,3,4,5
스트림 만들기 - 람다식 iterate(), generate()
static <T> Stream<T> iterate(T seed, UnaryOperator<T> f) // 이전 요소에 종속적
static <T> Stream<T> generate(Supplier<T> s) // 이전 요소에 독립적
- iterate()는 이전 요소를 seed로 해서 다음 요소를 계산한다.
Stream<Integer> evenStream = Stream.iterate(0, n->n+2); // 0, 2, 4, 6, ...
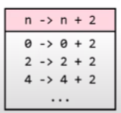
- generate()는 seed를 사용하지 않는다.
Stream<Double> randomStream = Stream.generate(Math::random);
Stream<Integer> oneStream = Stream.generate(()->1);
스트림 만들기 - 파일과 빈 스트림
Stream<Path> Files.list(Path dir) // Path는 파일 또는 디렉토리
Stream<String> Files.lines(Path path)
Stream<String> Files.lines(Path path, Charset cs)
Stream<String> lines() // BufferedReader클래스의 메서드
비어있는 스트림 생성하기
Stream emptyStream = Stream.empty(); // empty()는 빈 스트림을 생성해서 반환한다.
long count = emptyStream.count(); // count의 값은 0
ch 14-23~25 스트림의 연산
스트림의 연산
- 스트림이 제공하는 기능 - 중간 연산과 최종 연산
- 중간 연산 - 연산결과가 스트림인 연산. 반복적으로 적용가능
- 최종 연산 - 연산결과가 스트림이 아닌 연산. 단 한번만 적용가능(스트림의 요소를 소모)


- 중간 연산은 n번 가능하며, 연산결과가 Stream의 값을 갖기 때문에 이어서 사용할 수 있다.
- 최종 연산은 1번 가능하며, 각 Stream 연산자에 따라 값이 다르다. 최종 연산 후에 스트림이 닫힌다.
스트림의 연산 - 중간 연산
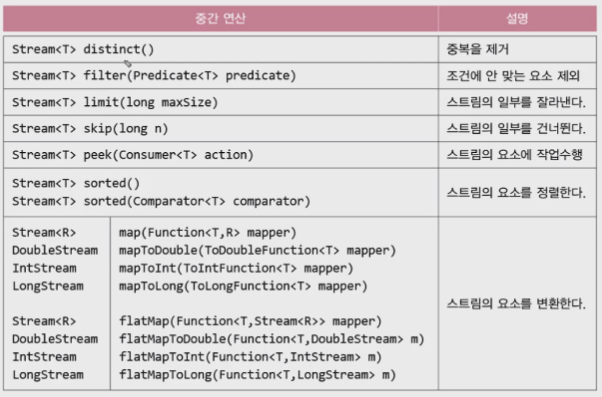
스트림의 연산 - 최종 연산
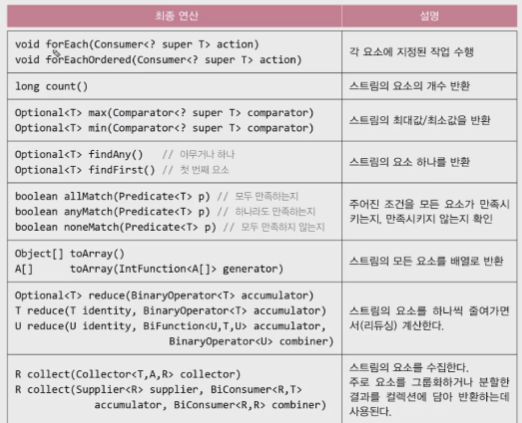
- Comparator = 정렬 기준
- findAny() = 병렬
- findFirst() = 직렬
- Optional = 래퍼클래스 (작업 결과가 null이더라도 객체에 담아서 준다.)
- reduce() = 스트림의 요소를 하나씩 줄여가며 계산 Ex) sum
- collect() = reduce()를 이용해서 그룹작업을 한다.