ch 14-45~49 collect(), Collectors
collect()와 Collectors
- collect()는 Collector를 매개변수로 하는 스트림의 최종연산
Object collect(Collector collector) // Collector를 구현한 클래스의 객체를 매개변수로
Object collect(Supplier supplier, BiConsumer accumulator, BiConsumer combiner) // 잘 안쓰임
- reduce() = 전체 리듀싱
- collect() = 그룹별 리듀싱
- Collector는 수집(collect)에 필요한 메서드를 정의해 놓은 인터페이스

- Collectors클래스는 다양한 기능의 컬렉터(Collector를 구현한 클래스)를 제공
* 변환 - mapping(), toList(), toSet(), toMap(), toCollection(), ...
* 통계 - counting(), summinInt(), averageInt(), maxBy(), minBy(), summarizingIng(), ...
* 문자열 결합 - joining()
* 리듀싱 - reducing
* 그룹화와 분할 - groupingBy(), partitioningBy(), collectingAndThen()
- collect() : 최종연산
- collector() : 인터페이스
- Collectors : 클래스
스트림을 컬렉션, 배열로 변환
- 스트림을 컬렉션으로 변환 - toList(), toSet(), toMap(), toCollection()

- 스트림을 배열로 변환 - toArray()
Student[] stuNames = studentStream.toArray(Student[]::new); // OK
Student[] stuNames = studentStream.toArray(); // 에러 (매개변수 있는 것)
- Student[]stuNames=(Student[])studentStream.toArray();//OK 자동 형변환이 안된다는 뜻
Object[] stuNames = studentStream.toArray(); // OK. (매개변수 없는 것)
스트림의 통계 - counting(), summingInt()
- 스트림의 통계정보 제공 - counting(), summingInt(), maxBy(), minBy(),...
long count = stuStream.count();
long count = stuStream.collect(counting()); // Collectors.counting()
long totalScore = stuStream.mapToInt(Student::getTotalScore).sum(); // IntStream의 sum()
long totalScore = stuStream.collect(summingInt(Student::getTotalScore));
OptionalInt topScore = studentStream.mapToInt(Student::getTotalScore).max()
Optional<Student> topStudent = stuStream
.max(Comparator.comparingInt(Student::getTotalScore));
Optional<Student> topStudent = stuStream
.collect(maxBy(Comparator.comparingInt(Student::getTotalScore));
스트림을 리듀싱 - reducing()
Collector reducing(BinaryOperator<T> op)
Collector reducing(T identity, BinaryOperator<T> op)
Collector reducing(U identity, Fuction<T,U> mapper, BinaryOperator<U> op) // map+reduce
- reduce()와 하는 일이 똑같다.
- reduce() = 전체 리듀싱
- reducing() = 그룹별 리듀싱
IntStream intStream = new Random().ints(1,46).distinct().limit(6);
OptionalInt max = intStream.reduce(Integer::max); // 전체 리듀싱
Optional<Integer> max = intStream.boxed().collect(reducing(Integer::max); // 그룹별 리듀싱 가능
long sum = intStream.reduce(0, (a,b) -> a + b);
long sum = intStream.boxed().collect(reducint(0, (a,b) -> a + b);
int grandTotal = stuStream.map(Student::getTotalScore).reduce(0, Integer::sum);
int grandTotal = stuStream.collect(reducing(0, Student::getTotalScore, Integer::sum));
- 문자열 스트림의 요소를 모두 연결 - joining()
String studentNames = stuStream.map(Student::getName).collect(joining());
String studentNames = stuStream.map(Student::getName).collect(joining(",")); // 구분자
String studentNames = stuStream.map(Student::getName).collect(joining(",", "[", "]"));
String studentInfo = stuStream.collect(joining(","); // Student의 toString()으로 결합
ch 14-50~55 스트림의 그룹화와 분할
스트림의 그룹화와 분할
- partioningBy()는 스트림을 2분할한다.
Collector partitioningBy(Predicate predicate)
Collector partitioningBy(Predicate predicate, Collector downstream)
- groupingBy()는 스트림을 n분할한다.
Collector groupingBy(Function classfier)
Collector groupingBy(Function classfier, Collector downsream)
Collector groupingBy(Function classfier, Supplier mapFactory, Collector downstream)
스트림의 분할 - partitioningBy()
Collector partitioningBy(Predicate predicate)
Collector partitioningBy(Predicate predicate, Collector downstream)
Map<Boolean, List<Student>> stuBySex = stuStream
.collect(partitioningBy(Student::isMale)); // 학생들을 성별로 분할
List<Student> maleStudent = stuBySex.get(true); // Map에서 남학생 목록을 얻는다.
List<Student> femaleStudent = stuBySex.get(false); // Map에서 여학생 목록을 얻는다.
Map<Boolean, Long> stuNumBySex = stuStream
.collect(partitioningBy(Student::isMale, counting()); // 분할 + 통계
System.out.println("남학생 수 :"+stuNumBySex.get(true)); // 남학생 수 : 8
System.out.println("여학생 수 :"+stuNumBySex.get(false)); // 여학생 수 : 10
Map<Boolean, Optional<Student>> topScoreBySex = stuStream // 분할 + 통계
.collect(partitioningBy(Student::isMale, maxBy(comparingInt(Student::getScore))));
System.out.println("남학생 1등 :"+topScoreBySex.get(true)); // 남학생 1등 : Optional[[나자바,남, 1, 1, 300]]
System.out.println("여학생 1등 :"+topScoreBySex.get(false)); // 여학생 1등 : Optional[[김지미,여, 1, 1, 250]]
Map<Boolean, Map<Boolean, List<Student>>> failedStuBySex = stuStream // 다중 분할
.collect(partitioningBy(Student::isMale, // 1.성별로 분할(남/녀)
partitioningBy(s -> s.getScore() < 150)); // 2. 성적으로 분할(불합격/합격)
List<Student> failedMaleStu = failedStuBySex.get(true).get(true);
List<Student> failedFemaleStu = failedStuBySex.get(false).get(true);
스트림의 그룹화 - groupingBy()
Collector groupingBy(Function classfier)
Collector groupingBy(Function classfier, Collector downstream)
Collector groupingBy(Function classfier, Supplier mapFactory, Collector downstream)
Map<Integer, List<Student>> stuByBan = stuStream // 학생을 반별로 그룹화
.collect(groupingBy(Student::getBan, toList())); // toList()생략가능
Map<Integer, Map<Integer, List<Student>>> stuByHakAndBan = stuStream // 다중그룹화
.collect(groupingBy(Student::getHak, // 1. 학년별 그룹화
groupingBy(Student::getBan) // 2. 반별 그룹화
));
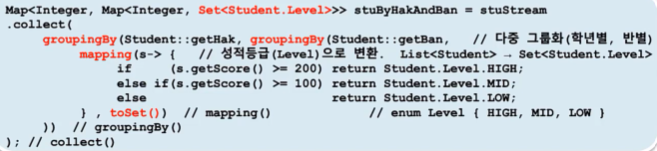
스트림의 변환(1/2)
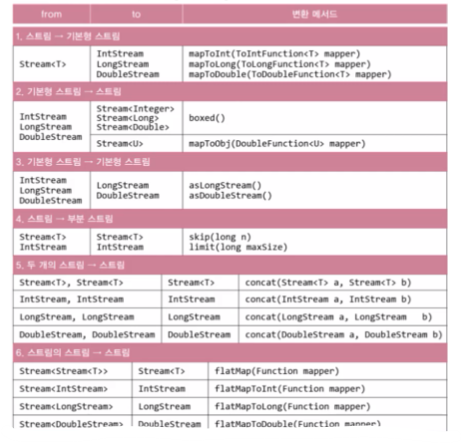
스트림의 변환(2/2)
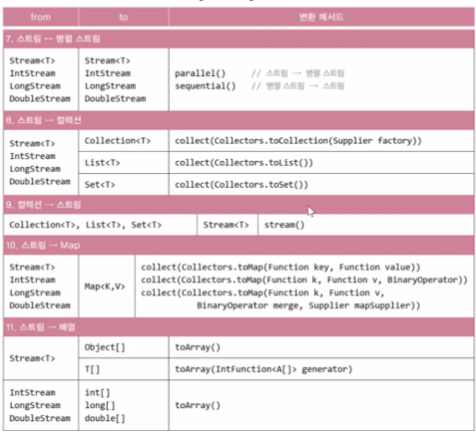