5주차 강의 복습 및 정리
1. 데이터 타입 확인
typeof
을 원하는 것 앞에 작성 시 테이터를 확인할 수 있음. 단, object, null, array 모두 "object"로 출력함
- 변수: let, const --> 데이터를 적을 때 전역인지 지역인지 확인인지 항상 확인!
2. 연산자
- 산술(더하기 빼기):
1 + 3
- 할당:
let a = 2
- 논리:
const a = 1 === 1
/ and 연산자 a && b
/ or 연산자 a || b
/ not 연산자 !a
- 삼항
const a = 1 < 2
if (a) {
console.log(true);
} else {
console.log(false);
}
console.log(a ? true : false);
3. 조건문
3-1. if...else
if (a) {
console.log(true);
} else if {
console.log(false);
} else {
console.log('what');
}
3-2. switch
switch (a) {
case 0:
console.log(0)
break;
case 2:
console.log(0)
break;
case 4:
console.log(0)
break;
default:
console.log('what')
}
4. 반복문 for
const ulEl = document.querySelector('ul')
for (let i = 0; i < 10; i += 1) {
const li = document.createElement('li')
li.textContent = `list-${i + 1}`
if ((i + 1 ) % 2 === 0) {
li.addEventListener('click', function () {
console.log(li.textContent);
})}
ulEl.appendChild(li)
}
5. 함수
let x = 3;
ley y = 4;
function adding1() {
console.log(x + y);
}
adding1();
let adding2 = function (x, y) {
console.log(x + y)
}
adding2(x, y)
5-1. 호이스팅
const a = 7;
double();
function double() {
console.log(a * 2);
}
5-2.화살표 함수
const double = function (x, y) {
return x * y;
}
const doubelArrow = (x, y) => x * y;
const a = 7;
function double() {
console.log(a * 2);
}
double();
(function () {
console.log(a * 2);
})();
((function () {
console.log(a * 2);
}));
5-4. 타이머 함수
const timer = setTimeout(() => {
console.log('heropy');
}, 3000);
const h1El = document.querySelector('hi');
h1El.addEventListener('click', () => {
clearTimeout(timer)
})
const timer2 = setInterval(() => {
console.log('heropy');
}, 3000);
const h1El2 = document.querySelector('hi');
h1El.addEventListener('click', () => {
clearInterval(timer)
})
5-5. 콜백 함수
function timeout(cb) {
setTimeout(() => {
console.log('hi');
cb();
}, 3000);
}
timeout(() => {
console.log('Done!');
});
6. 클래스: 객체를 생성하기 위한 템플릿(공장)
6-1. 생성자 함수(prototype)
function User (first, last) {
this.firstName = first;
this.lastName = last;
}
User.prototype.getFullName = function () {
return `${this.firstName} ${this.lastName}`
}
const heropy = new User('heropy', 'park');
const amy = new User('amy', 'park');
const neo = new User('neo', 'park');
console.log(heropy);
console.log(amy);
console.log(neo);
- 객체를 생성하는 함수이며 다수의 객체를 만들 때 사용
- 인스턴스를 찍어내는 공장이라고 이해함 -> new로 인해서 계속 새로운 객체를 생산 가능
6-2. this
const heropy = {
name: 'hi',
normal: function () {
console.log(this.name);
},
arrow: () => {
console.log(this.name);
}
}
heropy.normal();
heropy.arrow();
const amy = {
name: 'amy',
normal: heropy.normal,
arrow: heropy.arrow
}
amy.normal();
amy.arrow();
const timer = {
greet: 'hi',
timeout: function () {
setTimeout(() => {
console.log(this.greet);
}, 2000)
}
}
timer.timeout()
6-3. ES6 Classes 정의
class User {
constructor(first, last) {
this.firstName = first;
this.lastName = last;
}
getFullName() {
return `${this.firstName} ${this.lastName}`
}
}
const heropy = new User('heropy', 'park');
const amy = new User('amy', 'park');
const neo = new User('neo', 'park');
console.log(heropy);
console.log(amy.getFullName());
console.log(neo.getFullName());
6-4. 상속(확장)
class Vehicle {
constructor(name, wheel) {
this.name = name;
this.wheel = wheel;
}
}
const myVehicle = new Vehicle('bent', '2');
console.log(myVehicle);
class Bicycle extends Vehicle {
constructor(name , wheel) {
super(name, wheel)
}
}
const myBicycle = new Bicycle('ekek', 2);
const sonBicycle = new Bicycle('eqe', 2);
console.log(myBicycle);
console.log(sonBicycle);
class Car extends Vehicle {
constructor(name, wheel, licenese) {
super(name, wheel);
this.licenese = licenese;
}
}
const myCar = new Car('bentz', 4, true);
const sonCar = new Car('kia', 4, false);
console.log(myCar);
console.log(sonCar);
7. JS 데이터
7-1. 문자열(string)
- 문자 데이터 ("", '')
- MDN 참조하여 필요한 함수 탐색
- 쓰레기통 아이콘이면 더 이상 사용X
- 실험실 아이콘이면 시험 중
7-2. 숫자와 수학
- 숫자 데이터와
Math
활용하여 연산 기능 구현
- MDN 참조하여 필요한 함수 탐색 가능
7-3. 배열(array)
const obj = [element1, element2, element3];
- 배열의 원본을 수정시키는 것과 아닌 것이 있으니 이를 잘 구별하여 사용 필요
7-4. 객체(object)
let hihi = {
name: 'hi',
age: 17
}
const hihi = new Hi (first, last) {
this.firstName = first;
this.lastName = last;
}
7-4-1. prototye(유튜브-코딩애플 참조)
Array.prototype.함수 = function() {};
some.함수
- 이런 prototype이 아닌 클래스에도 메서드를 만들 수 있으며 정적(static) 메서드라고 함
7-5. 구조 분해 할당 (destructing assignment)
const user = {
name: "hi",
age: 85,
email: 'abc@gmail.com'
}
const { name: naming, age, email, address = 'Korea' } = user;
console.log(address);
console.log(naming);
const fruits = ['Apple', 'Banana', 'Cherry'];
const [a, b, c, d] = fruits;
console.log(a, b, c, d);
const [, e] = fruits;
console.log(e);
7-6. 전개 연산자(spread)
const fruits = ['Apple', 'Banana', 'Cherry', 'Orange'];
console.log(fruits);
console.log(...fruits);
function toObject(a, b, ...c) {
return {
a,
b,
c,
}
}
console.log(toObject(...fruits));
7-7. 불변성(immutability)
let a = 1;
let b = 2;
console.log(a, b, a == b);
b = a;
console.log(a, b, a == b);
b = 7;
console.log(a, b, a == b);
let c = 1;
console.log(b, c, b == c);
let a = { k: 1 };
let b = { k: 1 };
console.log(a, b, a == b);
a.k = 7;
b = a;
console.log(a, b, a == b);
a.k = 2;
console.log(a, b, a == b);
let c = b;
console.log(a, b, c, a == c);
a.k = 9;
console.log(a, b, c, a == c);
7-8. 얕은 복사(shallow)와 깊은 복사(deep)
import _ from 'lodash'
const user = {
name: 'hi',
age: 85,
emmails: ['abc@gmail.com']
}
const copyUser = _.cloneDeep(user);
console.log(copyUser === user);
user.age = 32;
console.log('user', user);
console.log('copyUser', copyUser);
user.emmails.push('neo@gmail.com');
console.log(user.emmails === copyUser.emmails);
8. JS 데이터 실습
8-1. 가져오기 내보내기 (improt, export)
import _ from 'lodash';
export default function getType(data) {
return Object.prototype.toString.call(data).slice(2, 8);
}
export function random () {
return Math.floor(Math.random() * 10);
}
export const user = {
name: 'hi',
age: 12
}
8-2. lodash
import _ from 'lodash';
const userA = [
{ userId: '1', name: 'A'},
{ userId: '2', name: 'B'}
]
const userB = [
{ userId: 1, name: 'A'},
{ userId: 3, name: 'C'},
]
const userC = userA.concat(userB);
_.uniqBy(userC, userId);
const userD = _.unionBy(userA, userB, 'userID');
import _ from 'lodash';
const users = [
{ userId: '1', name: 'A'},
{ userId: '2', name: 'B'},
{ userId: '3', name: 'C'},
{ userId: '4', name: 'D'},
{ userId: '5', name: 'E'},
]
const foundUser = _.find(users, {name: 'A'});
const foundIndex = _.findIndex(users, { name: 'C'})
_.remove(users, { user: 'A' })
console.log(users)
8-3. JSON(JavaScript Object Notation)
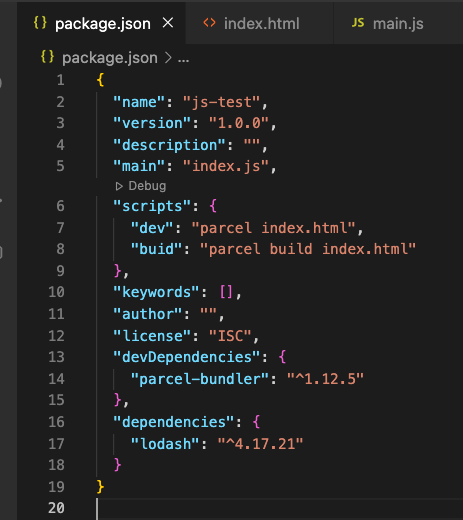
- 웹 어플리케이션에서 데이터를 전송할 때 일반적으로 사용
- 문자 데이터지만 import하였을 때 객체로 인식됨 → JSON.parse를 통해서 객체로 전환하여 사용
- 다시 JSON으로 사용하려면 JSON.strigfy
8-4. Storage
- 브라우저에 값을 저장
- local storage: domain 주소(사이트)에 종속, 데이터가 만료되지 않음, 반영구적으로 사용
- seesion storage: 페이지 세션이 끝날 때 사라짐
const user = {
name: 'hi',
age: 12,
emails: [
'this@gmail.com',
'that@gmail.com'
]
}
const str = localStorage.getItem('user');
const obj = JSON.parse(str);
obj.age = 22;
localStorage.setItem('user', JSON.stringify(obj));
8-5. OMDb(Open Movie Database) API
- query string을 활용하여 요청함 ->
{주소}http://www.omdbapi.com/?속성=값&속성=값&속성=값
- 요청하여 받은 값은 json형태로 받음!
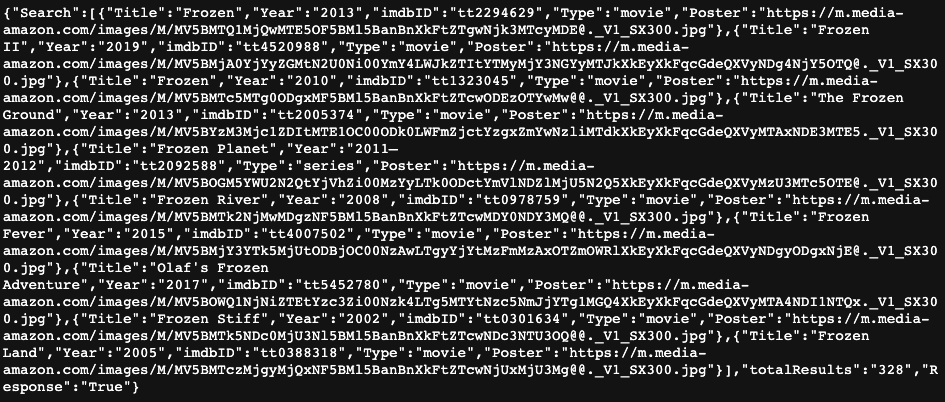
- axios 패키지를 이용하며 구조를 이해하고 다음 코드 선언 시 아래 사진과 같이 나옴
import axios from "axios";
function fetchMovies() {
axios
.get('https://www.omdbapi.com/?apikey=7035c60c&s=frozen')
.then((res) => {
console.log(res);
const h1El = document.querySelector('h1');
const imgEl = document.querySelector('img');
h1El.textContent = res.data.Search[0].Title;
imgEl.src = res.data.Search[0].Poster;
});
}
fetchMovies();
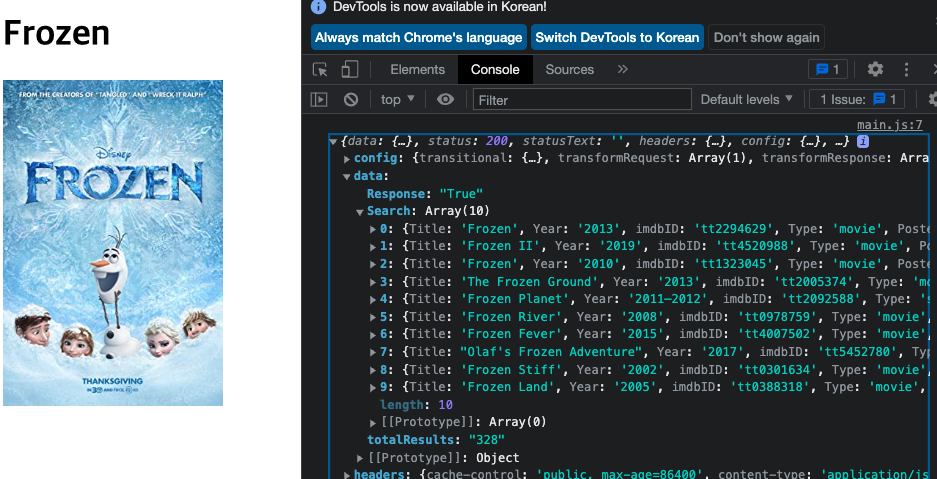
주간회고
- 이제 슬슬 강의만으로 이해하기 부족한 부분이 많다. 강의가 원론적인 의미로 이야기하는 부분이 많아서 이해하지 못하는 부분은 스스로 찾고 정리해아 함 -> 이 과정에서 개인적으로 느꼈을 때 "코딩애플"과 "생활코딩" 이 두분이 가장 이해하기 쉽게 설명해주는 듯
- 나중에 이런 것이 있었지...하면서 기억할 수 있게 자세하게 기억하기 보다는 전체적으로 느낌을 가지고 필요할 때 찾아볼 수 있는 기억력을 키울 수 있게 노력하자