프로젝트 개요
- EmployeeServiceImpl 클래스는 EmployeeService 인터페이스를 구현
- EmployeeServiceImpl 클래스에서는 EmployeeDAO 객체를 생성하고 DAO 클래스의 메서드를 호출하여 데이터베이스와 상호 작용
- 비즈니스 로직은 EmployeeServiceImpl 클래스에서 구현되며, EmployeeDAO 클래스는 데이터베이스와의 상호 작용을 담당
담당 기능 구현
EmployeeService
package controller;
import java.util.List;
import org.apache.ibatis.session.SqlSession;
import dto.UserDTO;
import exception.AccountRequestNotFoundException;
import exception.AllCustomerInfocheckFailException;
import exception.CustomerAccountApprovalException;
import exception.CustomerEnrolFailException;
import exception.CustomerInfocheckFailException;
import exception.DuplicateCustomerException;
public interface EmployeeService {
List<UserDTO> getAccountRequests(String isTemporary) throws AccountRequestNotFoundException, AllCustomerInfocheckFailException;
int registerCustomer(SqlSession session, UserDTO user) throws CustomerEnrolFailException, DuplicateCustomerException;
void approveCustomer(String user_id) throws CustomerAccountApprovalException;
UserDTO getCustomerById(String user_id) throws CustomerInfocheckFailException;
List<UserDTO> getAllCustomers() throws AllCustomerInfocheckFailException;
int rejectAccountRequest(SqlSession session, String user_id);
}
EmployeeServiceImpl
package controller;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import dao.EmployeeDAO;
import dto.UserDTO;
import exception.AccountRequestNotFoundException;
import exception.AllCustomerInfocheckFailException;
import exception.CustomerAccountApprovalException;
import exception.CustomerAccountRejectionException;
import exception.CustomerEnrolFailException;
import exception.CustomerInfocheckFailException;
import exception.DuplicateCustomerException;
public class EmployeeServiceImpl implements EmployeeService {
static SqlSessionFactory sqlSessionFactory;
static {
String resource = "mybatis/Configuration.xml";
InputStream inputStream = null;
try {
inputStream = Resources.getResourceAsStream(resource);
} catch (IOException e) {
e.printStackTrace();
}
sqlSessionFactory =
new SqlSessionFactoryBuilder().build(inputStream);
}
@Override
public List<UserDTO> getAccountRequests(String isTemporary) throws AccountRequestNotFoundException {
try (SqlSession session = sqlSessionFactory.openSession()) {
EmployeeDAO dao = new EmployeeDAO();
List<UserDTO> userList = dao.getAccountRequests(session, isTemporary);
if (userList == null || userList.isEmpty()) {
throw new AccountRequestNotFoundException("계좌 생성 요구 조회 실패: 조회된 데이터가 없습니다.");
}
return userList;
}
}
@Override
public int registerCustomer(SqlSession session, UserDTO user) throws CustomerEnrolFailException, DuplicateCustomerException {
try {
int count = session.selectOne("mybatis.EmployeeMapper.isDuplicatedCustomer", user.getUserId());
if (count > 0) {
throw new DuplicateCustomerException("이미 등록된 고객입니다.");
}
int n = session.insert("mybatis.EmployeeMapper.registerCustomer", user);
if (n != 1) {
throw new CustomerEnrolFailException("고객 등록에 실패했습니다.");
}
return n;
} catch (DuplicateCustomerException e) {
throw e;
} catch (Exception e) {
throw new CustomerEnrolFailException("고객 등록에 실패했습니다.");
}
}
@Override
public void approveCustomer(String user_id) throws CustomerAccountApprovalException {
try (SqlSession session = sqlSessionFactory.openSession()) {
EmployeeDAO dao = new EmployeeDAO();
int count = dao.approveCustomer(session, user_id);
if (count != 1) {
throw new CustomerAccountApprovalException("고객 계정 승인 실패: 대포통장 의심 거래자입니다. 다시 확인하세요. ");
}
session.commit();
}
}
@Override
public int rejectAccountRequest(SqlSession session, String user_id) {
int result = 0;
try {
int count = session.selectOne("mybatis.EmployeeMapper.getAccountRequests", user_id);
if (count != 1) {
throw new AccountRequestNotFoundException("해당 계좌 생성 요청이 존재하지 않습니다.");
}
result = session.delete("mybatis.EmployeeMapper.deleteAccountRequest", user_id);
session.commit();
System.out.println("계좌 생성 요청이 거절되었습니다.");
} catch (AccountRequestNotFoundException e) {
System.out.println(e.getMessage());
} catch (Exception e) {
System.out.println("계좌 생성 요청 거절 중 예외가 발생했습니다: " + e.getMessage());
}
return result;
}
@Override
public UserDTO getCustomerById(String user_id) throws CustomerInfocheckFailException {
try (SqlSession session = sqlSessionFactory.openSession()) {
EmployeeDAO dao = new EmployeeDAO();
UserDTO user = dao.getCustomerById(session, user_id);
if (user == null) {
throw new CustomerInfocheckFailException("고객 정보 조회 실패: 해당 ID의 고객이 존재하지 않습니다.");
}
return user;
}
}
@Override
public List<UserDTO> getAllCustomers() throws AllCustomerInfocheckFailException {
try (SqlSession session = sqlSessionFactory.openSession()) {
EmployeeDAO dao = new EmployeeDAO();
List<UserDTO> userList = dao.getAllCustomers(session);
if (userList == null || userList.isEmpty()) {
throw new AllCustomerInfocheckFailException("모든 고객 정보 조회 실패: 조회된 데이터가 없습니다.");
}
return userList;
}
}
}
EmployeeDAO
package dao;
import java.util.List;
import org.apache.ibatis.session.SqlSession;
import dto.UserDTO;
import exception.AccountRequestNotFoundException;
import exception.CustomerEnrolFailException;
import exception.DuplicateCustomerException;
public class EmployeeDAO {
public List<UserDTO> getAccountRequests(SqlSession session, String isTemporary) {
List<UserDTO> userList = null;
if ("1".equals(isTemporary)) {
userList = session.selectList("mybatis.EmployeeMapper.getAccountRequests", true);
} else {
userList = session.selectList("mybatis.EmployeeMapper.getAccountRequests", false);
}
return userList;
}
public int registerCustomer(SqlSession session, UserDTO user) throws CustomerEnrolFailException, DuplicateCustomerException {
try {
int count = session.selectOne("mybatis.EmployeeMapper.isDuplicatedCustomer", user.getUserId());
if (count > 0) {
throw new DuplicateCustomerException("이미 등록된 고객입니다.");
}
int n = session.insert("mybatis.EmployeeMapper.registerCustomer", user);
if (n != 1) {
throw new CustomerEnrolFailException("고객 등록에 실패했습니다.");
}
return n;
} catch (DuplicateCustomerException e) {
throw e;
} catch (Exception e) {
throw new CustomerEnrolFailException("고객 등록에 실패했습니다.");
}
}
public int approveCustomer(SqlSession session, String user_id) {
int count = session.update("mybatis.EmployeeMapper.approveCustomer", user_id);
return count;
}
public int rejectAccountRequest(SqlSession session, String user_id){
int result = 0;
try {
int count = session.selectOne("mybatis.EmployeeMapper.getAccountRequests", user_id);
if (count != 1) {
throw new AccountRequestNotFoundException("해당 계좌 생성 요청이 존재하지 않습니다.");
}
result = session.delete("mybatis.EmployeeMapper.deleteAccountRequest", user_id);
session.commit();
System.out.println("계좌 생성 요청이 거절되었습니다.");
} catch (AccountRequestNotFoundException e) {
System.out.println(e.getMessage());
} catch (Exception e) {
System.out.println("계좌 생성 요청 거절 중 예외가 발생했습니다: " + e.getMessage());
}
return result;
}
public UserDTO getCustomerById(SqlSession session, String user_id) {
UserDTO user = null;
user = session.selectOne("mybatis.EmployeeMapper.getCustomerById", user_id);
return user;
}
public List<UserDTO> getAllCustomers(SqlSession session) {
List<UserDTO> userList = null;
userList = session.selectList("mybatis.EmployeeMapper.getAllCustomers");
return userList;
}
}
EmployeeMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="mybatis.EmployeeMapper">
<!-- 계좌 생성 요구 조회 -->
<select id="getAccountRequests" resultMap="accountRequestResult">
SELECT *
FROM account
WHERE is_temporary = #{isTemporary}
</select>
<!-- 결과를 매핑하는 resultMap 정의 -->
<!-- resultMap은 SQL 쿼리 결과를 자바 객체로 매핑하기 위한 설정 -->
<!-- DB에서 조회한 데이터를 자동으로 Java 객체로 변환 -->
<!-- property는 임의의 변수명 (테이블 컬럼명과 무관) -->
<resultMap id="accountRequestResult" type="mybatis.EmployeeMapper.UserDTO">
<id property="id" column="id"/>
<result property="userId" column="user_id"/>
<!-- is_temporary 컬럼의 값을 isTemporary 변수에 매핑 -->
<result property="isTemporary" column="is_temporary"/>
<result property="createDate" column="create_date"/>
</resultMap>
<!-- 고객 등록 -->
<!-- <insert> 태그를 이용해 등록할 고객의 정보를 bank_user 테이블에 삽입 -->
<!-- type 값은 'Customer'로 고정 -->
<!-- user_key는 sequence를 이용해 새로운 고유한 값을 자동으로 생성 -->
<insert id="registerCustomer" parameterType="mybatis.EmployeeMapper.UserDTO">
INSERT INTO bank_user(
user_key,
user_id,
user_password,
type,
name,
birth_day)
VALUES (
bank_user_sequence.NEXTVAL,
#{user_id},
#{user_password},
'Customer',
#{name},
#{birth_day})
</insert>
<!-- 고객 id 중복 확인 -->
<select id="isDuplicatedCustomer" parameterType="String" resultType="int">
SELECT COUNT(*) FROM customer WHERE user_id = #{userId}
</select>
<!-- 고객 승인 -->
<update id="approveCustomer" parameterType="int">
UPDATE bank_user
SET is_temporary = 0
WHERE user_key = #{userKey}
</update>
<!-- 고객 승인 거절 -->
<delete id="deleteAccountRequest" parameterType="String">
DELETE FROM account_request WHERE user_id = #{user_id}
</delete>
<!-- 고객 정보 열람 -->
<select id="getCustomerById" parameterType="String" resultType="mybatis.EmployeeMapper.UserDTO">
SELECT *
FROM bank_user
WHERE user_id = #{user_id}
</select>
<!-- 전체 회원 정보 조회 -->
<select id="getAllCustomers" resultMap="CustomerResult">
SELECT *
FROM bank_user
</select>
<resultMap id="CustomerResult" type="mybatis.EmployeeMapper.CustomerResult">
<id property="user_id" column="user_id"/>
<result property="user_key" column="user_key"/>
<result property="name" column="name"/>
<result property="birth_day" column="birth_day"/>
</resultMap>
</mapper>
- 직원(Employee) 이 로그인하면 보이는 뷰 수정
package view;
import java.util.List;
import java.util.Scanner;
import org.apache.ibatis.session.SqlSession;
import controller.EmployeeServiceImpl;
import controller.ManagerServiceImpl;
import controller.UserATM_Impl;
import controller.UserJoin_Impl;
import dto.AccountDTO;
import dto.UserDTO;
import exception.AccountRequestNotFoundException;
import exception.AllCustomerInfocheckFailException;
import exception.CustomerAccountApprovalException;
import exception.CustomerAccountRejectionException;
import exception.CustomerEnrolFailException;
import exception.CustomerInfocheckFailException;
import exception.DeleteEmployeeFailException;
import exception.EmployeeCreationFailException;
import exception.HandOverManagerException;
public class Menu {
public static Scanner scan = new Scanner(System.in);
public static UserATM_Impl userImpl = new UserATM_Impl();
public static UserJoin_Impl userJoin = new UserJoin_Impl();
private static UserDTO loginedUser;
private static List<AccountDTO> login_User_account_list;
private static Menu menu = new Menu();
public static Menu getInstance() {
if(menu == null) {
menu = new Menu();
}
return menu;
}
public void init() {
loginMenu();
}
public void loginMenu() {
while(true) {
System.out.println("");
System.out.println("\t┏━━━* Daou_Bank ATM ━━━━┓");
System.out.println("\t┃ ┃");
System.out.println("\t┗━━━━━━━━━━━━━━━━━━━━━━━┛");
System.out.println("\t ┃ ┃");
System.out.println("\t ┃ ━━━━━━━━━━━━━━━━ *");
System.out.println("\t ┃ 1) 로그인");
System.out.println("\t ┃ 2) 회원가입");
System.out.println("\t ┃ 3) 관리자 로그인(임시)");
System.out.println("\t ┃ 0) 종료하기");
System.out.println("\t ┃ ");
System.out.print("\t ┃ 메뉴 입력 : ");
String menu = scan.next();
System.out.println("\t ┃ ━━━━━━━━━━━━━━━━ ┃");
System.out.println("\t ┃ *");
System.out.println("\t ┗━━━━━━━━━━━━━━━━━━━┛\n");
System.out.println("");
switch(menu) {
case ("1"):
userJoin.userLogin(loginedUser,login_User_account_list);
break;
case ("2"):
userJoin.userSignup();
break;
case ("3"):
loginedUser = new UserDTO(
1, "bizyoung93", "123123", "Manager", "김현영", "1993/03/29");
EmployeeView();
break;
case ("0"):
System.out.println("Good Bye *");
System.exit(0);return;
default:
System.out.println("다시 입력해주세요 :)");
}
}
}
public void userView(UserDTO userdto, List<AccountDTO> account_list) {
loginedUser = userdto;
login_User_account_list = account_list;
while(true) {
System.out.println("");
System.out.println("\t┏━━━* Daou_Bank ATM ━━━━┓");
System.out.println("\t┃ ┃");
System.out.println("\t┗━━━━━━━━━━━━━━━━━━━━━━━┛");
System.out.println("\t ┃ ┃");
System.out.println("\t ┃ ━━━━━━━━━━━━━━━━ *");
System.out.println("\t ┃ 1) 계좌조회");
System.out.println("\t ┃ 2) 입금 ");
System.out.println("\t ┃ 3) 출금 ");
System.out.println("\t ┃ 4) 계좌이체");
System.out.println("\t ┃ 5) 통장정리");
System.out.println("\t ┃ 6) 계좌 개설 요청");
System.out.println("\t ┃ 7) 마이 페이지");
System.out.println("\t ┃ 0) 로그아웃");
System.out.println("\t ┃ ");
System.out.print("\t ┃ 메뉴 입력 : ");
String menu = scan.next();
System.out.println("\t ┃ ━━━━━━━━━━━━━━━━ ┃");
System.out.println("\t ┃ *");
System.out.println("\t ┗━━━━━━━━━━━━━━━━━━━┛\n");
System.out.println("");
switch(menu) {
case ("1"):
userImpl.userBalance();
break;
case ("2"):
userImpl.userDeposit();
break;
case ("3"):
userImpl.userWithdraw();
break;
case ("4"):
userImpl.userTransfer();
break;
case ("5"):
userImpl.userHistory();
break;
case ("6"):
userImpl.createAccount(loginedUser.getUser_key());
break;
case ("7"):
userImpl.showInfo(loginedUser,login_User_account_list);
break;
case ("0"):
System.out.println("로그아웃 합니다.");
loginedUser = null;
login_User_account_list = null;
loginMenu();
break;
default:
System.out.println("없는 메뉴를 선택하셨습니다");
userView(loginedUser,login_User_account_list);
}
}
}
public void EmployeeView() {
while(true) {
System.out.println("");
System.out.println("\t┏━━━* Daou_Bank ATM ━━━━┓");
System.out.println("\t┃ ┃");
System.out.println("\t┗━━━━━━━━━━━━━━━━━━━━━━━┛");
System.out.println("\t ┃ ┃");
System.out.println("\t ┃ ━━━━━━━━━━━━━━━━ *");
System.out.println("\t ┃ 1) 계좌 생성 요구 조회");
System.out.println("\t ┃ 2) 고객 등록");
System.out.println("\t ┃ 3) 고객 정보 열람");
System.out.println("\t ┃ 4) 고객 계좌 생성 승인");
System.out.println("\t ┃ 5) 고객 계좌 생성 거절");
System.out.println("\t ┃ 6) 전체 회원 정보 조회");
if (loginedUser.getType() == "Manager") {
System.out.println("\t ┃ 7) 직원 등록");
System.out.println("\t ┃ 8) 관리자 권한 인계");
System.out.println("\t ┃ 9) 직원 삭제");
}
System.out.println("\t ┃ 0) 로그아웃");
System.out.println("\t ┃ ");
System.out.print("\t ┃ 메뉴 입력 : ");
int menu = scan.nextInt();
System.out.println("\t ┃ ━━━━━━━━━━━━━━━━ ┃");
System.out.println("\t ┃ *");
System.out.println("\t ┗━━━━━━━━━━━━━━━━━━━┛\n");
System.out.println("");
if (menu == 1) {
System.out.println("계좌 생성 요구 조회를 선택하셨습니다.");
EmployeeServiceImpl service = new EmployeeServiceImpl();
String isTemporary = scan.nextLine();
try {
boolean isTemp = "1".equals(isTemporary) ? true : false;
service.getAccountRequests(isTemp ? "1" : "0");
} catch (AccountRequestNotFoundException e) {
System.out.println(e.getMessage());
} catch (Exception e) {
System.out.println("잘못된 입력값입니다. 1 또는 0을 입력해주세요.");
}
} else if (menu == 2) {
System.out.println("고객 등록을 선택하셨습니다.");
EmployeeServiceImpl service = new EmployeeServiceImpl();
String user_id = scan.nextLine();
UserDTO user = new UserDTO(user_id);
SqlSession sqlSession = MybatisUtil.getSqlSessionFactory().openSession();
try {
service.registerCustomer(sqlSession, user);
} catch (CustomerEnrolFailException e) {
System.out.println(e.getMessage());
}
} else if (menu == 3) {
System.out.println("고객 정보 열람을 선택하셨습니다.");
EmployeeServiceImpl service = new EmployeeServiceImpl();
String user_id = scan.nextLine();
try {
service.getCustomerById(user_id);
} catch (CustomerInfocheckFailException e) {
System.out.println(e.getMessage());
}
} else if (menu == 4) {
System.out.println("고객 계좌 생성 승인을 선택하셨습니다.");
EmployeeServiceImpl service = new EmployeeServiceImpl();
String user_id = scan.nextLine();
try {
service.approveCustomer(user_id);
} catch (CustomerAccountApprovalException e) {
System.out.println(e.getMessage());
}
}
else if (menu == 5) {
System.out.println("고객 계좌 생성 거절을 선택하셨습니다.");
String user_id = scan.nextLine();
EmployeeServiceImpl service = new EmployeeServiceImpl();
SqlSession sqlSession = MybatisUtil.getSqlSessionFactory().openSession();
try {
service.rejectAccountRequest(sqlSession, user_id);
} catch (CustomerAccountRejectionException e) {
System.out.println(e.getMessage());
}
} else if (menu == 6) {
System.out.println("전체 회원 정보 조회을 선택하셨습니다.");
EmployeeServiceImpl service = new EmployeeServiceImpl();
try {
service.getAllCustomers();
} catch (AllCustomerInfocheckFailException e) {
System.out.println(e.getMessage());
}
} else if (menu == 7) {
System.out.println("직원 등록을 선택하셨습니다.");
ManagerServiceImpl service = new ManagerServiceImpl();
try {
service.registerEmployee(user);
} catch (EmployeeCreationFailException e) {
System.out.println(e.getMessage());
}
System.out.println("직원 아이디를 입력해주세요");
String user_id = scan.next();
System.out.println("직원의 비밀번호를 입력해주세요");
String user_password = scan.next();
System.out.println("직원의 비밀번호 확인을 입력해주세요");
String user_password_confirm = scan.next();
System.out.println("직원의 이름을 입력해주세요");
String user_name = scan.next();
System.out.println("직원의 생일을 입력해주세요");
String user_birth_day = scan.next();
UserDTO user = new UserDTO(-1, user_id, user_password, "Employee", user_name, user_birth_day);
try {
service.registerEmployee(user);
} catch (EmployeeCreationFailException e) {
System.out.println(e.getMessage());
}
} else if (menu == 8) {
System.out.println("관리자 권한 인계를 선택하셨습니다.");
ManagerServiceImpl service = new ManagerServiceImpl();
System.out.println("인계하시려는 직원 아이디를 입력해주세요");
String targetEmployee = scan.next();
try {
service.handOverManager(loginedUser, targetEmployee);
} catch (HandOverManagerException e) {
System.out.println(e.getMessage());
}
} else if (menu == 9) {
System.out.println("직원 삭제를 선택하셨습니다.");
ManagerServiceImpl service = new ManagerServiceImpl();
System.out.println("삭제하시려는 직원 아이디를 입력해주세요");
String targetEmployee = scan.next();
try {
service.deleteEmployee(targetEmployee);
} catch (DeleteEmployeeFailException e) {
System.out.println(e.getMessage());
}
} else if (menu == 0) {
System.out.println("로그아웃을 선택하셨습니다.");
loginedUser = null;
login_User_account_list = null;
loginMenu();
return;
} else {
System.out.println("없는 메뉴를 선택하셨습니다. 다시 입력해 주세요");
}
}
}
}
예외 처리
- Exception 패키지에 아래의 클래스 파일 작성
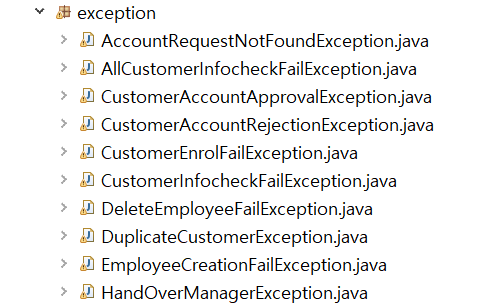
import exception.AccountRequestNotFoundException;
import exception.AllCustomerInfocheckFailException;
import exception.CustomerAccountApprovalException;
import exception.CustomerEnrolFailException;
import exception.CustomerInfocheckFailException;
import exception.DuplicateCustomerException;
Appendix