import java.util.Random;
import java.util.*;
class RockPaperScissors {
private final static String ROCK = "바위";
private final static String PAPER = "보";
private final static String SCISSORS = "가위";
private final static String[] ROCK_PAPER_SCISSORS = {ROCK, PAPER, SCISSORS};
private final static String COMMA = ", ";
private final static String STR_SITUATION_OK = "군요. 알겠습니다.\n";
private final static String STR_SITUATION_ASK = "니까?(Y/N)\n";
private final static String STR_SITUATION_PARDON = "...? 다시 한번 말씀해주시겠습";
private final static String STR_SITUATION_NEWLINE = "\n";
private final static String STR_SITUATION_BR = "\n\n";
private final static String STR_SITUATION_SPACE = " ";
private final static String STR_SITUATION_ESC = "니까?(x로 탈출): ";
private static String strSituationOutOfRPS = SCISSORS.concat(STR_SITUATION_SPACE).concat(ROCK).concat(STR_SITUATION_SPACE).concat(PAPER).concat(" 셋 중 ");
private static List<String> rockList = new ArrayList<String>();
private static List<String> paperList = new ArrayList<String>();
private static List<String> scissorsList = new ArrayList<String>();
private static int scoreScissors;
private static int scoreRock;
private static int scorePaper;
private static int score;
private static boolean firstGame = true;
private static void game() {
if (firstGame) {
rockList.add(ROCK);
paperList.add(PAPER);
scissorsList.add(SCISSORS);
firstGame = false;
};
Scanner scanner = new Scanner(System.in, "Cp949");
StringBuilder print = new StringBuilder();
String hand = new String();
Random random = new Random();
int randomIndex;
String handRandom = new String();
boolean keepGoing = true;
rps:
while(keepGoing) {
print.append(SCISSORS);
print.append(COMMA);
print.append(ROCK);
print.append(COMMA);
print.append(PAPER);
print.append(" 중 하나를 입력하세요.");
print.append(STR_SITUATION_NEWLINE);
System.out.print(print);
print.setLength(0);
run:
while(true) {
randomIndex = random.nextInt(3);
handRandom = ROCK_PAPER_SCISSORS[randomIndex];
switch (handRandom) {
case SCISSORS: {
scoreScissors = 1;
scoreRock = 2;
scorePaper = 0;
break;
}
case ROCK: {
scoreScissors = 0;
scoreRock = 1;
scorePaper = 2;
break;
}
case PAPER: {
scoreScissors = 2;
scoreRock = 0;
scorePaper = 1;
break;
}
default: {
System.out.println("err1: handRandom 의 값이 ".concat(handRandom).concat(" 입니다."));
return;
}
};
String input = (scanner.nextLine().replaceAll("\\s+","")).toLowerCase();
int rockListSize = rockList.size();
for (int i = 0; i < rockListSize; i++) {
if (input.equals(rockList.get(i))) {
hand = ROCK;
break run;
};
};
int paperListSize = paperList.size();
for (int i = 0; i < paperListSize; i++) {
if (input.equals(paperList.get(i))) {
hand = PAPER;
break run;
};
};
int scissorsListSize = scissorsList.size();
for (int i = 0; i < scissorsListSize; i++) {
if (input.equals(scissorsList.get(i))) {
hand = SCISSORS;
break run;
};
};
print.append(input);
print.append("...? 그건 ");
print.append(strSituationOutOfRPS);
print.append("하나입");
print.append(STR_SITUATION_ASK);
System.out.print(print);
print.setLength(0);
boolean answerIsInRPS = yesOrNo();
if (answerIsInRPS) {
print.append(strSituationOutOfRPS);
print.append("어떤 겁니까?: ");
System.out.print(print);
print.setLength(0);
while(true) {
String answer = (scanner.nextLine().replaceAll("\\s+","")).toLowerCase();
switch(answer) {
case SCISSORS: {
print.append(SCISSORS);
print.append(STR_SITUATION_OK);
scissorsList.add(input);
hand = SCISSORS;
break;
}
case ROCK: {
print.append(ROCK);
print.append(STR_SITUATION_OK);
rockList.add(input);
hand = ROCK;
break;
}
case PAPER: {
print.append(PAPER);
print.append(STR_SITUATION_OK);
paperList.add(input);
hand = PAPER;
break;
}
case "x": {
print.append(STR_SITUATION_NEWLINE);
continue rps;
}
default : {
print.append(answer);
print.append(STR_SITUATION_PARDON);
print.append(STR_SITUATION_ESC);
}
};
System.out.print(print);
print.setLength(0);
if (hand.length() != 0) {
break;
};
};
break run;
} else {
print.append(strSituationOutOfRPS);
print.append("하나를 입력해주시길 바랍니다: ");
System.out.print(print);
print.setLength(0);
};
};
switch(hand) {
case ROCK: {
score = scoreRock;
break;
}
case PAPER: {
score = scorePaper;
break;
}
case SCISSORS: {
score = scoreScissors;
break;
}
default: {
score = -1;
}
};
print.append(handRandom);
print.append(STR_SITUATION_NEWLINE);
switch(score) {
case 0: {
print.append("졌습니다.");
break;
}
case 1: {
print.append("비겼습니다.");
break;
}
case 2: {
print.append("이겼습니다.");
break;
}
default: {
System.out.println("err2: score 의 값이 ".concat(String.valueOf(score)).concat(" 입니다."));
return;
}
};
print.append(STR_SITUATION_NEWLINE);
print.append("계속하시겠습");
print.append(STR_SITUATION_ASK);
System.out.print(print);
print.setLength(0);
keepGoing = yesOrNo();
hand = "";
};
print.append("프로그램을 종료합니다.");
print.append(STR_SITUATION_BR);
System.out.print(print);
print.setLength(0);
}
private static boolean yesOrNo() {
Scanner scanner = new Scanner(System.in, "Cp949");
StringBuilder print = new StringBuilder();
boolean yn = false;
boolean asking = true;
while (asking) {
String answer = (scanner.nextLine().replaceAll("\\s+","")).toLowerCase();
switch (answer) {
case "네": case "예": case "yes": case "y": case "ㅇ": case "ㅇㅇ": case "어": case "어어": case "응": case "그래": {
asking = false;
yn = true;
break;
}
case "아니오": case "아니요": case "아뇨": case "아닙니다": case "no": case "n": case "ㄴ": case "ㄴㄴ": case "아니": {
asking = false;
yn = false;
break;
}
default: {
print.append(STR_SITUATION_NEWLINE);
print.append(answer);
print.append(STR_SITUATION_PARDON);
print.append(STR_SITUATION_ASK);
System.out.print(print);
print.setLength(0);
}
};
};
return yn;
}
public static void main(String[] args) {
game();
}
}
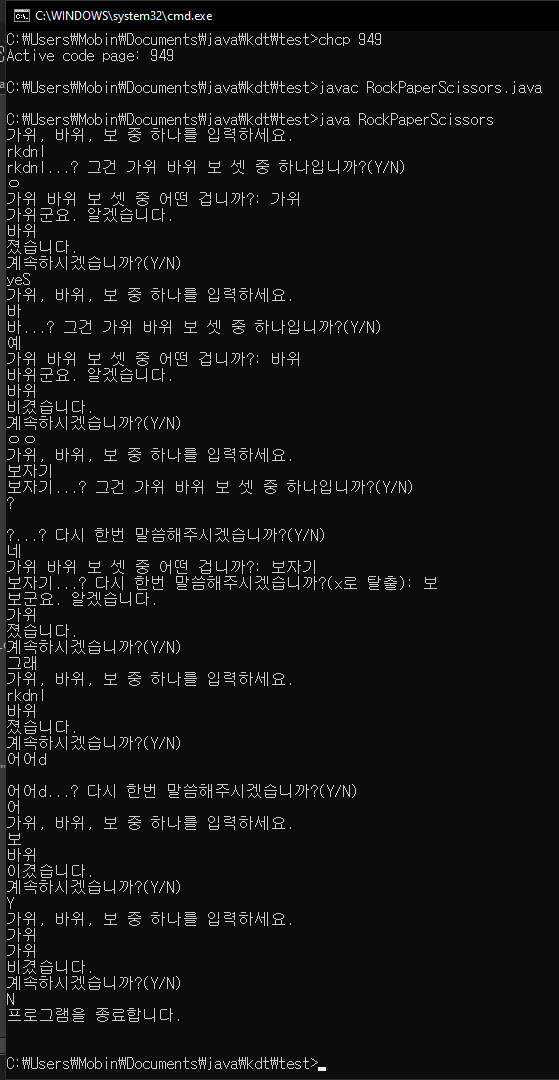