1. with 와 in 문법에 대하여 설명하시오.
function print(p) {
document.write(p);
}
function println(p) {
print(p);
print("<br>");
}
let object = {};
object.property1 = 1;
object.property2 = 2;
object.property3 = 3;
with (object) {
println(property1);
println(property2);
println(property3);
}
for (let x in object) {
println(object[x]);
}
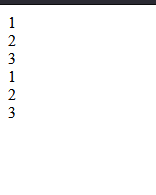
2.아래를 프로그래밍 하시오.
var circle = new Circle();
circle.setRaius(10);
circle.getArea();
var rec = new Rectangle();
rec.setWidth(10);
rec.setHeight(10);
rec.getArea();
function print(p) {
document.write(p);
}
function println(p) {
print(p);
print("<br>");
}
function Circle() {}
Circle.prototype.radius = 0;
Circle.prototype.setRadius = function (radius) {
this.radius = radius;
};
Circle.prototype.getArea = function () {
println(Math.PI * Math.pow(this.radius, 2));
};
function Rectangle() {}
Rectangle.prototype.width = 0;
Rectangle.prototype.height = 0;
Rectangle.prototype.setWidth = function (width) {
this.width = width;
};
Rectangle.prototype.setHeight = function (height) {
this.height = height;
};
Rectangle.prototype.getArea = function () {
println(this.width * this.height);
};
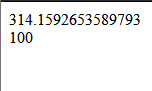
3. prototype 에 대하여 설명하시오.
function print(p) {
document.write(p);
}
function println(p) {
print(p);
print("<br>");
}
function Constructor() {}
Constructor.prototype.property = 1;
Constructor.prototype.setProperty = function (property) {
this.property = property;
};
Constructor.prototype.getProperty = function () {
return this.property;
};
let c = new Constructor();
println(c.getProperty());
c.setProperty(3);
println(c.property);
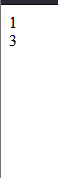
4. 아래 코드 실행시 처음과 끝에 실행되는 구문에 대하여 설명하시오?
function User(name) {
this.name = name;
this.isAdmin = false;
}
let user = new User("보라");
5. 생성자 함수에 대하여 설명하시오.
function print(p) {
document.write(p);
}
function println(p) {
print(p);
print("<br>");
}
function Constructor(p1, p2, p3) {
this.p1 = p1;
this.p2 = p2;
this.p3 = p3;
}
let c = new Constructor(1, 2, 3);
with (c) {
println(p1);
println(p2);
println(p3);
}
