2021.04.12
달력만들기
- 년, 월
- 해당 월의 1일 무슨 요일?
- 월의 마지막 일?
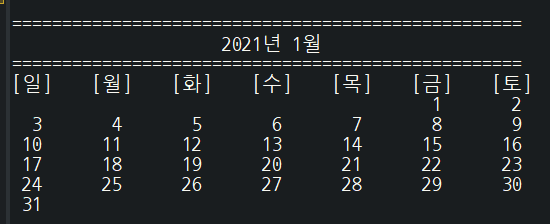
public class Ex23_Calendar {
public static void main(String[] args) throws NumberFormatException, IOException {
int year = 0, month = -1;
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.println("달력만들기");
System.out.print("년 : ");
year = Integer.parseInt(reader.readLine());
System.out.print("월 : ");
month = Integer.parseInt(reader.readLine());
output(year,month);
}
public static void output(int year, int month) {
int lastDay = 0;
int day_of_week = 0;
lastDay = getLastDay(year, month);
day_of_week = getDayOfWeek(year, month);
System.out.println();
System.out.println("===================================================");
System.out.printf(" %d년 %d월\n", year, month);
System.out.println("===================================================");
System.out.println("[일]\t[월]\t[화]\t[수]\t[목]\t[금]\t[토]");
for(int i=0;i<day_of_week;i++) {
System.out.print("\t");
}
for (int i=1; i<=lastDay; i++) {
System.out.printf("%3d\t", i);
if (i % 7 == (7 - day_of_week)) {
System.out.println();
}
}
}
public static int getDayOfWeek(int year, int month) {
int totalDays = 0;
for (int i=1; i<year; i++) {
if(isLeafYear(i)) {
totalDays+= 366;
} else {
totalDays+= 365;
}
}
for(int i=1; i<month; i++) {
totalDays += getLastDay(year, i);
}
totalDays++;
return totalDays % 7;
}
public static int getLastDay(int year, int month) {
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
return 31;
case 4:
case 6:
case 9:
case 11:
return 30;
case 2:
return isLeafYear(year) ? 29 : 28;
}
return 0;
}
public static boolean isLeafYear(int year) {
if (year % 4 == 0) {
if (year % 100 == 0) {
if (year % 400 == 0) {
return true;
} else {
return false;
}
} else {
return true;
}
} else {
return false;
}
}
}
반복문
중첩 for문
for(int i=0; i<10; i++) {
for(int j=0; j<10; j++) {
System.out.printf("i: %d, j: %d\n", i, j);
}
}
for(int i=0; i<100; i++) {
System.out.printf("i: %d\n", i);
}
- 우리 주변에 2중 for문의 루프 변수 변화값을 볼 수 있는 사례
for (int i=0; i<24; i++) {
for (int j=0; j<60; j++) {
System.out.printf("%02d:%02d\n", i, j);
}
for(int i=1; i<=6; i++) {
for(int j=1; j<=30; j++) {
System.out.printf("%d강의실 %d번 학생\n", i, j);
}
}
for(int i=1; i<=15; i++) {
for(int j=1; j<=5; j ++) {
System.out.printf("%d층 %d호 ",i, j);
}
}
for (int i = 0; i < 10; i++) {
for (int j = 0; j<10; j++) {
for (int k = 0; k < 10; k++) {
System.out.println("실행문");
}
}
}
for (int i=0; i<24; i++) {
for (int j=0; j<60; j++) {
for(int k=0; k<60; k++) {
System.out.printf("%02d:%02d:%02d\n", i, j, k);
}
}
}
for (int dan=2; dan<10; dan++) {
for (int i=1; i<10; i++) {
System.out.printf("%d x %d = %2d\n", dan, i, dan * i);
}
System.out.println();
}
for (int i=0; i<10; i++) {
for(int j=0; j<10; j++) {
if(i == 5) {
break;
}
System.out.printf("i: %d, j: %d\n", i, j);
}
}
for(int i=0; i<10; i++) {
for(int j=0; j<10; j++) {
System.out.print("*");
}
System.out.println();
}
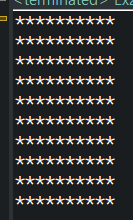
for(int i=0; i<5; i++) {
for(int j=0; j<=i; j++) {
System.out.print("*");
}
System.out.println();
}
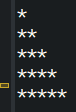
for(int i=0; i<5; i++) {
for(int j=i; j<5; j++) {
System.out.print("*");
}
System.out.println();
}
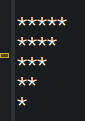
for(int i=0; i<5; i++) {
for(int j=0; j<(4-i); j++) {
System.out.print(" ");
}
for(int j=0; j<=i; j++) {
System.out.print("*");
}
System.out.println();
}
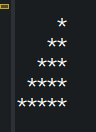
while
While(조건식){
실행문;
}
for(int i=1; i<=10; i++) {
System.out.println(i);
}
System.out.println();
int n = 1;
while(n<=10) {
System.out.println(n);
n++;
}
int sum = 0;
for(int i=0; ; i++) {
sum+=i;
if(sum>=1000) {
System.out.println(i);
break;
}
}
System.out.println(sum);
sum = 0;
n = 1;
while(true) {
sum += n;
if(sum >= 1000) {
break;
}
n++;
}
System.out.println(sum);
sum = 0;
n = 1;
while(sum < 1000) {
sum += n;
n++;
}
System.out.println(sum);
sum = 0;
for(int i=0; sum<=1000; i++) {
sum+=i;
}
System.out.println(sum);
do while
while (조건식){
실행코드;
}
do {
실행코드;
} while (조건식);
int n = 20;
do {
System.out.println(n);
n++;
} while (n <= 10);
문자열, String
- 문자열과 관련된 여러가지 기능을 자바가 제공 (★★★★★)
문자열의 길이
- 문자열을 구성하는 문자의 개수(글자 수)
- int length()
String txt = "";
txt = "ABCDEF";
System.out.println(txt.length());
System.out.println("ABCDEF".length());
txt = "123 홍길동 !@#";
System.out.println(txt.length());
System.out.println((int)' ');
System.out.println((int)' ');
System.out.println((int)'\t');
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.print("문장 입력 : ");
txt = reader.readLine();
System.out.printf("입력한 문장은 총 %d개의 문자로 구성되어있습니다.\n", txt.length());
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.print("이름 입력: ");
String name = reader.readLine();
if(name.length() >= 2 && name.length() <= 5) {
System.out.println("환영합니다.");
} else {
System.out.println("이름은 2~5자 이내로 입력하세요.");
}
String name ="";
while (true) {
System.out.println("이름 : ");
name = reader.readLine();
if(name.length() >= 2 && name.length() <= 5) {
break;
} else {
System.out.println("이름은 2~5자 이내로 입력하세요.");
}
}
System.out.println("회원가입을 진행합니다.");
문자열 추출 - char charAt(int index)
- 원하는 위치의 문자를 추출
- char charAt(int index)
- index : 추출하고자 하는 문자의 위치(첨자, index, 서수)
- 서수를 0부터 센다. > Zero-based Index (자바)
String txt = "홍길동";
char c = txt.charAt(3);
System.out.println(c);
for(int i=1; i<=10; i++) {
}
for (int i=0; i<10; i++) {
}
for(int i=0; i<txt.length(); i++) {
System.out.println(txt.charAt(i));
}
String id = "test";
for (int i = 0; i < id.length(); i++) {
char c = id.charAt(i);
int code = (int) c;
if (code < 97 || code > 122) {
System.out.println("잘못된 문자가 있습니다.");
break;
}
}
System.out.println("종료");