2021.04.26
ArrayList - Q24번문제 풀이
public class Ex64_ArrayList {
public static void main(String[] args) {
MyList list = new MyList();
System.out.println(list);
list.add(100);
System.out.println(list);
list.add(200);
System.out.println(list);
list.add(300);
System.out.println(list);
list.add(400);
System.out.println(list);
list.add(500);
System.out.println(list);
list.add(600);
list.add(700);
list.add(800);
list.add(900);
System.out.println(list);
System.out.println("list.get(0):"+ list.get(0));
System.out.println("list.get(0):"+ list.get(3));
System.out.println("list.get(0):"+ list.get(5));
System.out.println("size : "+ list.size());
list.set(0, 1000);
System.out.println(list);
list.set(8, 9000);
System.out.println(list);
System.out.println(list.size());
list.remove(4);
System.out.println(list);
list.add(1);
System.out.println(list);
list.add(5,5);
System.out.println(list);
list.add(5,5);
list.add(5,5);
list.add(5,5);
list.add(5,5);
list.add(5,5);
list.add(5,5);
list.add(5,5);
System.out.println(list);
list.add(5, 5);
System.out.println(list);
list.clear();
System.out.println(list);
list.add(100);
list.add(200);
System.out.println(list.size());
for (int i=0; i<list.size(); i++) {
System.out.println(list.get(i));
}
System.out.println();
System.out.println();
System.out.println();
System.out.println(list);
list.trimToSize();
System.out.println(list);
}
}
class MyList {
private int[] list;
private int index;
public MyList() {
this.list = new int[4];
this.index = 0;
}
public void add(int n) {
doubling();
this.list[this.index] = n;
this.index++;
}
private void doubling() {
if(this.index >= this.list.length) {
int[] temp = new int[this.list.length * 2];
for(int i=0; i<this.list.length; i++) {
temp[i] = this.list[i];
}
this.list = temp;
}
}
@Override
public String toString() {
String temp = "[";
for(int n: this.list) {
temp += String.format("%4d,", n);
}
temp = temp.substring(0,temp.length()-1);
temp += "]";
return String.format("length: %d\nindex: %d\n%s\n"
, this.list.length
, this.index
, temp);
}
public int get(int index) {
if(index>=0 && index < this.index) {
return this.list[index];
} else {
throw new IndexOutOfBoundsException();
}
}
public int size() {
return this.index;
}
public void set (int index,int n) {
if(index>=0 && index < this.index) {
this.list[index] = n;
} else {
throw new IndexOutOfBoundsException();
}
}
public void remove(int index) {
if (index >= 0 && index < this.index) {
for (int i=index; i<this.index-1; i++) {
this.list[i] = this.list[i+1];
}
this.index--;
} else {
throw new IndexOutOfBoundsException();
}
}
public void add(int index, int n) {
if(index >= 0 && index < this.index) {
doubling();
for(int i=this.index-1; i>=index; i--) {
this.list[i] = this.list[i];
}
this.list[index] = n;
this.index++;
} else {
throw new IndexOutOfBoundsException();
}
}
public int indexOf(int n) {
for(int i=0; i<this.index; i++) {
if(this.list[i] == n) {
return i;
}
}
return -1;
}
public int lastIndexOf(int n) {
for(int i=this.index-1; i>=0; i--) {
if(this.list[i] == n) {
return i;
}
}
return -1;
}
public void clear () {
this.index = 0;
}
public boolean contains(int n) {
for (int i=0; i<this.index; i++) {
if (this.list[i] == n) {
return true;
}
}
return false;
}
public void trimToSize() {
int[] temp = new int[this.index];
for (int i=0; i<temp.length; i++) {
temp[i] = this.list[i];
}
this.list = temp;
}
public boolean isEmpty() {
return this.index == 0 ? true : false;
}
}
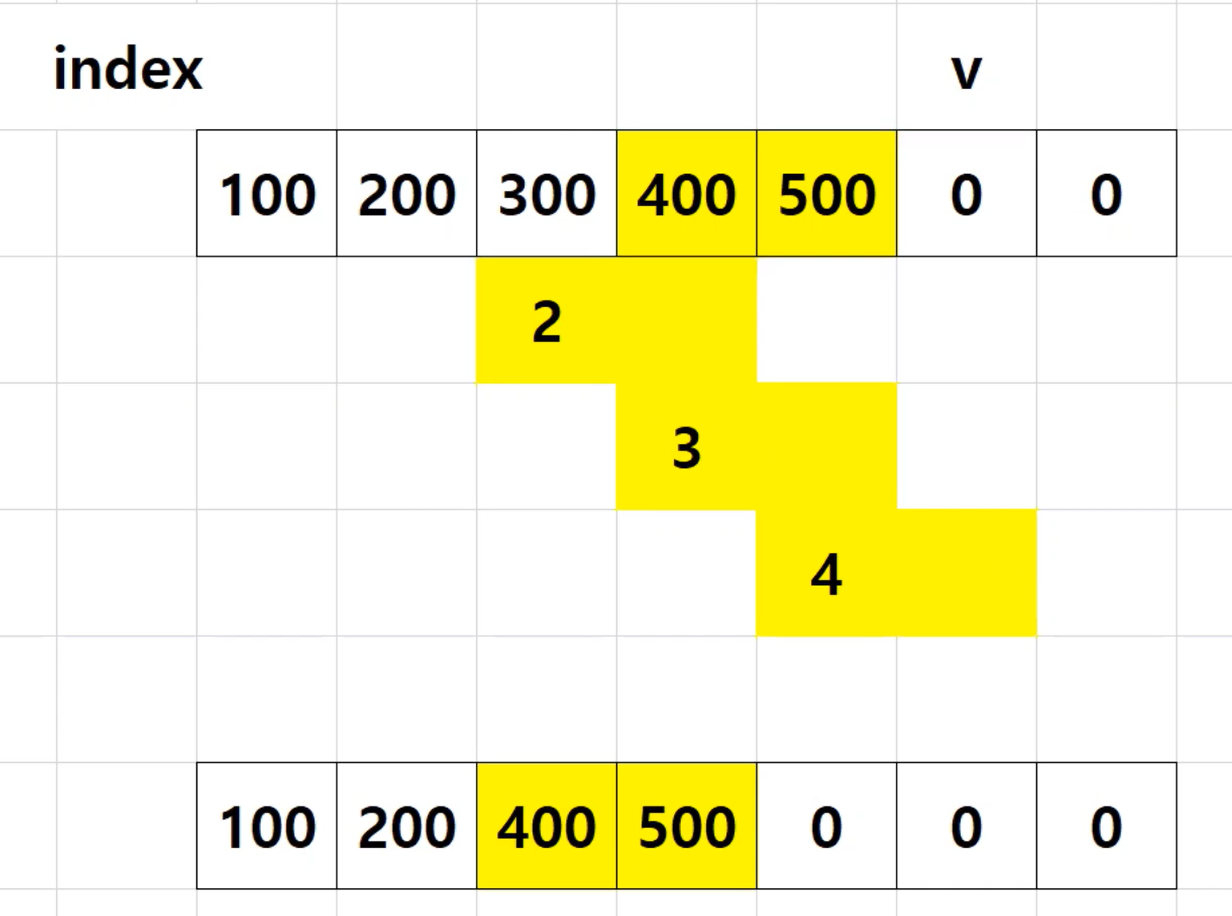
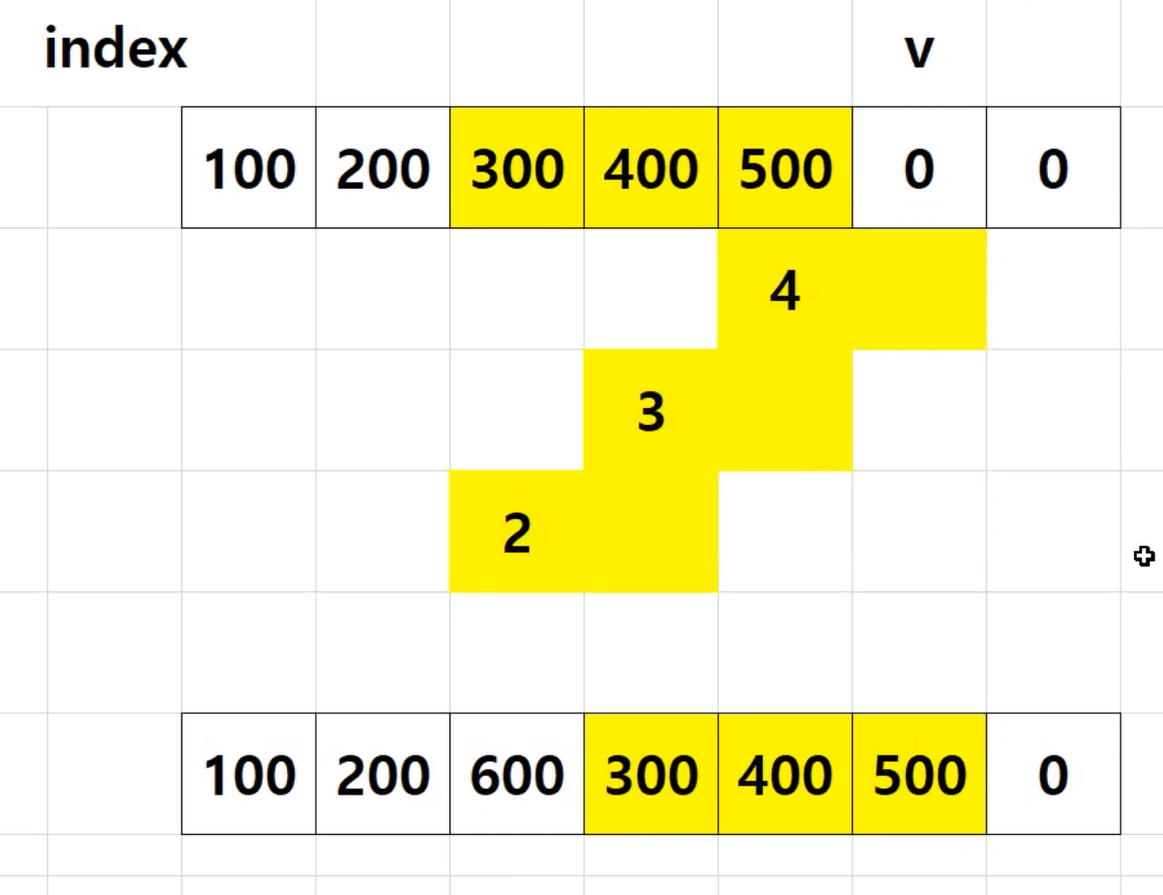
ArrayList vs HashMap
HashMap
- 방번호 없음 -> 요소의 순서가 없음
- 방이름 있음
- 사전 구조(Dictionary), 맵(Map), 연관 배열
- 키(key)와 값(Value)으로 요소 관리
- 루프 적용 불가능(단점x) -> 애초에 루프를 돌릴 목적으로 만드는 배열이 아니다.
- 가독성 때문에 사용하는 배열 -> 방의 이름이 명확!!! (이름보고 찾기 쉽다.)
ArrayList
- 요소 접근 -> 첨자(index) 사용 -> 요소의 순서가 있음
- 스칼라 배열(Scalar Array)
- 루프 적용 가능(장점)
HashMap 사용법
import java.util.HashMap;
public class Ex65_HashMap {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<String, Integer>();
map.put("국어",100);
map.put("영어",90);
map.put("수학",80);
System.out.println(map.size());
System.out.println(map.get("국어"));
System.out.println(map.get("영어"));
System.out.println(map.get("수학"));
map.put("국어", 60);
System.out.println(map.get("국어"));
HashMap<String, String> group = new HashMap<String, String>();
group.put("반장", "홍길동");
group.put("부반장", "아무개");
group.put("체육부장", "하하하");
System.out.println(map.get("반장"));
HashMap<String, Boolean> result = new HashMap<String, Boolean>();
result.put("홍길동", true);
result.put("아무개", false);
result.put("하하하", true);
System.out.println(result.get("홍길동"));
System.out.println(result.get("호호호"));
Scanner scan = new Scanner(System.in);
System.out.print("응시자명: ");
String name = scan.nextLine();
if(result.get(name) != null) {
if(result.get(name)) {
System.out.println("합격하셨습니다.");
} else {
System.out.println("불합격하셨습니다.");
}
} else {
System.out.println("응시자 명단에 없습니다.");
}
HashMap<Boolean, String> map2 = new HashMap<Boolean, String>();
map2.put(true, "홍길동");
map2.put(false, "아무개");
map2.put(true, "호호호");
HashMap<Integer, String> map3 = new HashMap<Integer, String>();
map3.put(1, "빨강");
map3.put(2, "노랑");
map3.put(3, "파랑");
}
}
순수배열, 클래스, ArrayList, HashMap 선택 기준
public class Ex65_HashMap {
public static void main(String[] args) {
Student2 s1 = new Student2();
s1.name = "홍길동";
s1.address = "서울시";
s1.tel = "010-1523-456";
s1.age = 20;
Student2 s2 = new Student2();
s2.name = "아무개";
s2.address = "서울시";
s2.tel = "010-5422-456";
s2.age = 25;
HashMap<String, String> s3 = new HashMap<String, String>();
s3.put("name", "하하하");
s3.put("address", "인천시");
s3.put("tel", "010-6589-6235");
s3.put("age", "21");
System.out.println(s2.name);
System.out.println(s3.get("name"));
HashMap<String, String> s4 = new HashMap<String, String>();
s4.put("name", "호호호");
s4.put("address", "인천시");
s4.put("tel", "010-3564-6584");
s4.put("age","24");
}
}
class Student2 {
public String name;
public String address;
public String tel;
public int age;
}
public class Ex65_HashMap {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<String, Integer>(10);
map.put("국어", 100);
map.put("영어", 90);
map.put("수학", 80);
System.out.println(map.size());
System.out.println(map.containsKey("국어"));
System.out.println(map.containsValue(100));
System.out.println(map.get("영어"));
System.out.println(map.isEmpty());
map.remove("국어");
System.out.println(map.size());
System.out.println(map.get("국어"));
}
}
HashMap - Q25문제 풀이
import java.util.Arrays;
public class Ex66_HashMap {
public static void main(String[] args) {
MyMap map = new MyMap();
System.out.println(map);
map.put("red", "빨강");
System.out.println(map);
map.put("blue", "파랑");
System.out.println(map);
map.put("yellow", "노랑");
map.put("green", "녹색");
map.put("white", "흰색");
System.out.println(map);
System.out.println(map.get("red"));
System.out.println(map.get("blue"));
System.out.println(map.get("yellow"));
System.out.println(map.get("black"));
map.put("white", "하양");
System.out.println(map);
}
}
class MyMap {
private Entry[] list;
private int index;
public MyMap() {
this.list = new Entry[4];
this.index = 0;
}
@Override
public String toString() {
return String.format("length: %d\nindex: %d\n%s\n"
, this.list.length
, this.index
, Arrays.toString(this.list));
}
public void put(String key, String value) {
doubling();
if (!containsKey(key)) {
Entry e = new Entry();
e.key = key;
e.value = value;
this.list[this.index] = e;
this.index++;
} else {
this.list[getIndex(key)].value = value;
}
}
private boolean containsKey(String key) {
for (int i=0; i<this.index; i++) {
if (this.list[i].key.equals(key)) {
return true;
}
}
return false;
}
private boolean containsValue(String value) {
for (int i=0; i<this.index; i++) {
if (this.list[i].value.equals(value)) {
return true;
}
}
return false;
}
private void doubling() {
if (this.index >= this.list.length) {
Entry[] temp = new Entry[this.list.length * 2];
for (int i=0; i<this.list.length; i++) {
temp[i] = this.list[i];
}
this.list = temp;
}
}
public String get(String key) {
int index = getIndex(key);
if (index > -1) {
return this.list[index].value;
} else {
return null;
}
}
public int getIndex(String key) {
for (int i=0; i<this.index; i++) {
if (this.list[i].key.equals(key)) {
return i;
}
}
return -1;
}
}
class Entry {
public String key;
public String value;
@Override
public String toString() {
return String.format("(%s,%s)", this.key, this.value);
}
}