2021.04.06
자바 변수 종류
지역변수, Local Variable
- 변수는 자신의 영역이 존재한다.(자신이 활동할 수 있는 지역) > Scope
- 지역을 벗어나면 사용이 불가능(접근이 불가능)하다.
public class Ex16_Method {
public static void main(String[] args) {
int a = 10;
m1();
System.out.println(a);
String name = "아무개";
}
private static void m1() {
String name = "아무개";
int b = 20;
System.out.println(b);
}
}
변수의 생명 주기, Life Cycle
- 변수가 언제 태어나고, 언제 죽는지?
- 변수가 언제 메모리에 할당이 되고, 언제 메모리에서 해제가 되는지?
- 변수는 변수 선언문이 실행되는 시점에 태어난다.(메모리에 할당)
- 변수는 자신이 속한 블럭에서 제어가 벗어나는 순간 죽는다.(본인이 속한 메서드가 실행을 마칠때)(메모리에서 소멸된다.)
- 메인메서드에는 변수를 많이 안만드는 것이 좋다.(메인메서드안의 지역변수의 생명주기가 길기 때문에 메모리를 더 많이 잡아먹는다. 메서드의 지역변수의 생명주기가 더 쩗음.)
메소드 오버로딩, Method Overloading
- 같은 이름의 메소드를 여러개 만드는 기술
- 메소드가 인자 리스트의 형태를 다양하게 구성해서 동일한 메소드명을 가질 수 있게 하는 기술
- 프로그램 성능(x), 개발자에게 도움(o)
메서드 오버로딩의 조건 O
- 인자의 갯수
- 인자의 타입
메서드 오버로딩의 조건 X
- 인자의 이름
- 반환값의 타입
1. public static void test() {}
2. public static void test() {}
3. public static void test(int n) {}
4. public static void test(int m) {}
5. public static void test(String s) {}
6. public static void test(int n, int m) {}
7. public static void test(int n, String s) {}
8. public static void test(String s, int n) {}
9. public static String test(int n) {}
public class Ex17_Overloading {
public static void main(String[] args) {
test();
test(10);
test("홍길동");
}
public static void test(){
System.out.println("사과");
}
public static void test(int n){
}
public static void test(String s){
}
}
재귀 메소드, Recursive Method
- 메소드가 자신의 구현부에서(body) 자신을 호출하는 구문을 가지는 메소드
- 자기가 자기를 호출하는 메소드
public class Ex18_Recursive {
public static void main(String[] args) {
}
public static void test() {
System.out.println("테스트입니다.");
test();
}
}
public class Ex18_Recursive {
public static void main(String[] args) {
int n = 4;
int result = factorial(n);
System.out.printf("%d!= %d\n", n, result);
}
public static int factorial(int n) {
int temp = (n == 1) ? 1 : n * factorial(n-1);
return temp;
}
}
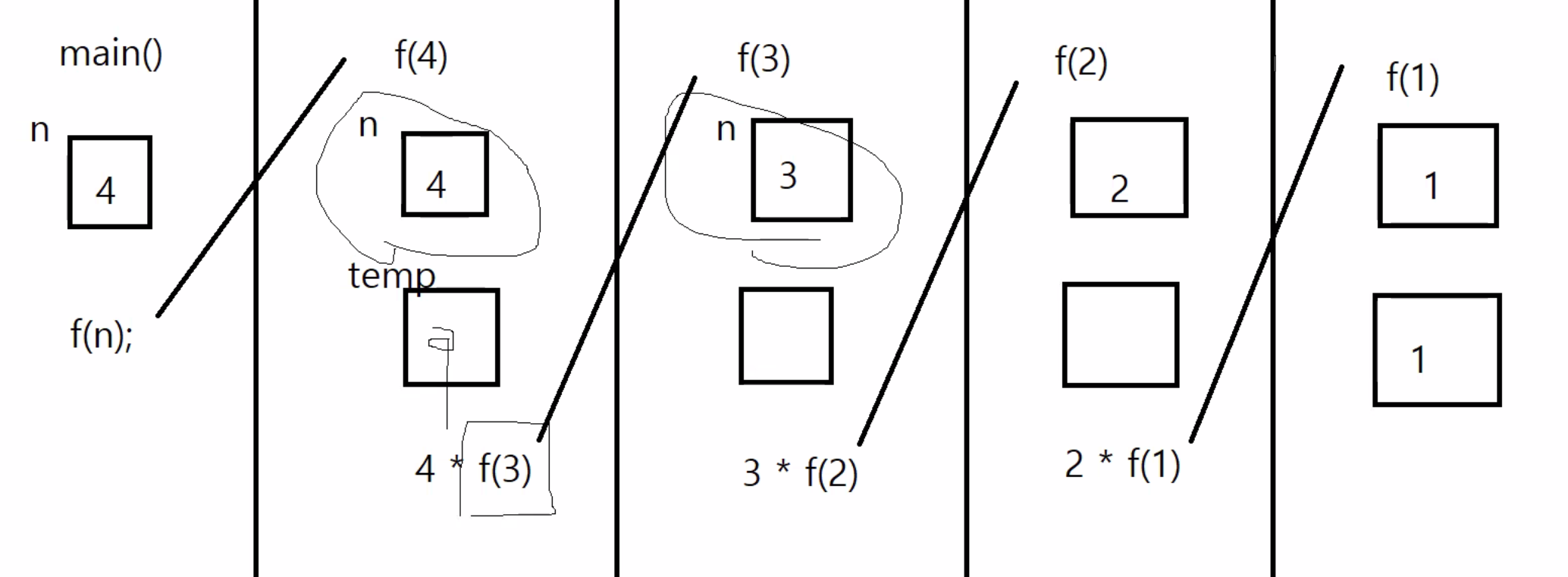