Hibernate / JPA and JDBC
- Hibernate / JPA uses JDBC for all database communications
💎 Object-To-Relational Mapping(ORM)
- The developer defines mapping between Java class and database table
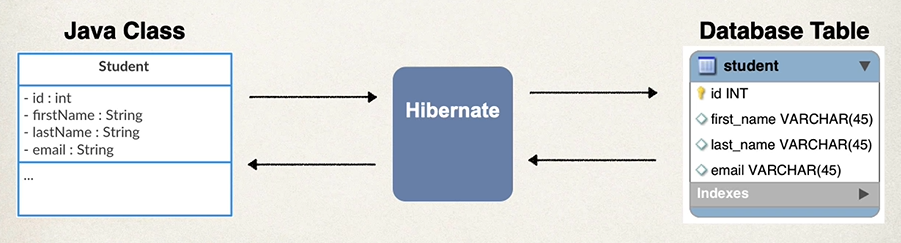
💎 What is JPA?
- Jakarta Persistence API
- Standard API for ORM
- Only a specification
- Defines a set of interfaces
⚾ Saving a Java Object with JPA
Student theStudent = new Student("abc, "xyz");
entityManager.persist(theStudent);
- entityManager: Special JPA helper object
- .persist: The data will be stored in the database SQL insert
⚾ Retrieving a Java Object with JPA
int theId = 1;
Student myStudent = entityManager.find(Student.class, theID);
- find: Query the database for given id or the pK
⚾ Querying for Java Objects
// gives a list of all of those student objects
TypedQuery<Student> theQuery = entityManager.createQuery("from student", Student.class);
List<Student> students = theQuery.getResultList();
- getResultList(): Returns a list of Student Objects from the database