실습 문제 15
🚩 메서드명 : public void practice15 ( )
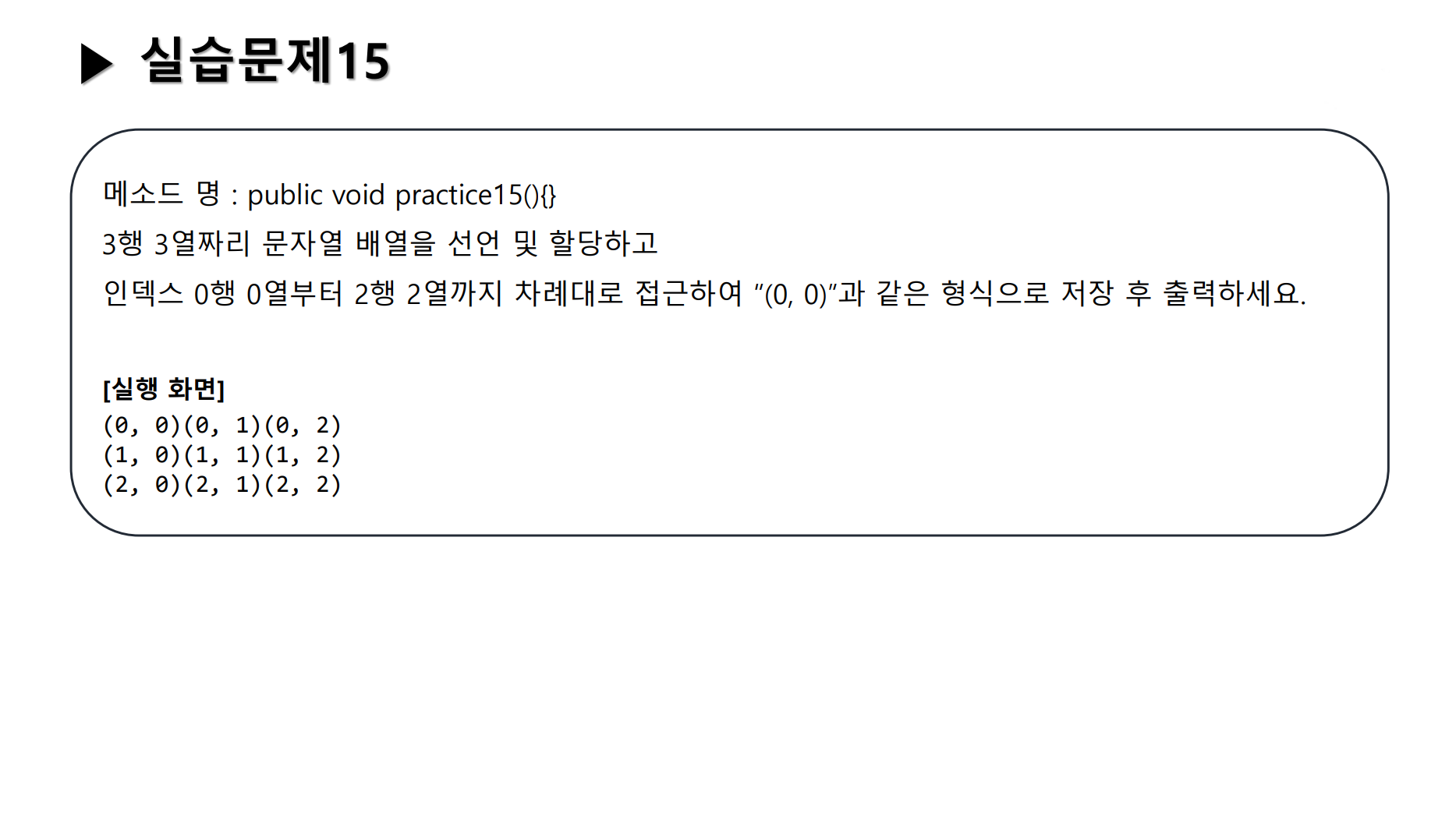
소스 코드
public void practice15() {
String[][] arr = new String[3][3];
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[i].length; j++) {
arr[i][j] = "(" + i + ", " + j + ")";
}
}
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[i].length; j++) {
System.out.print(arr[i][j]);
}
System.out.println();
}
}
실습 문제 16
🚩 메서드명 : public void practice16 ( )
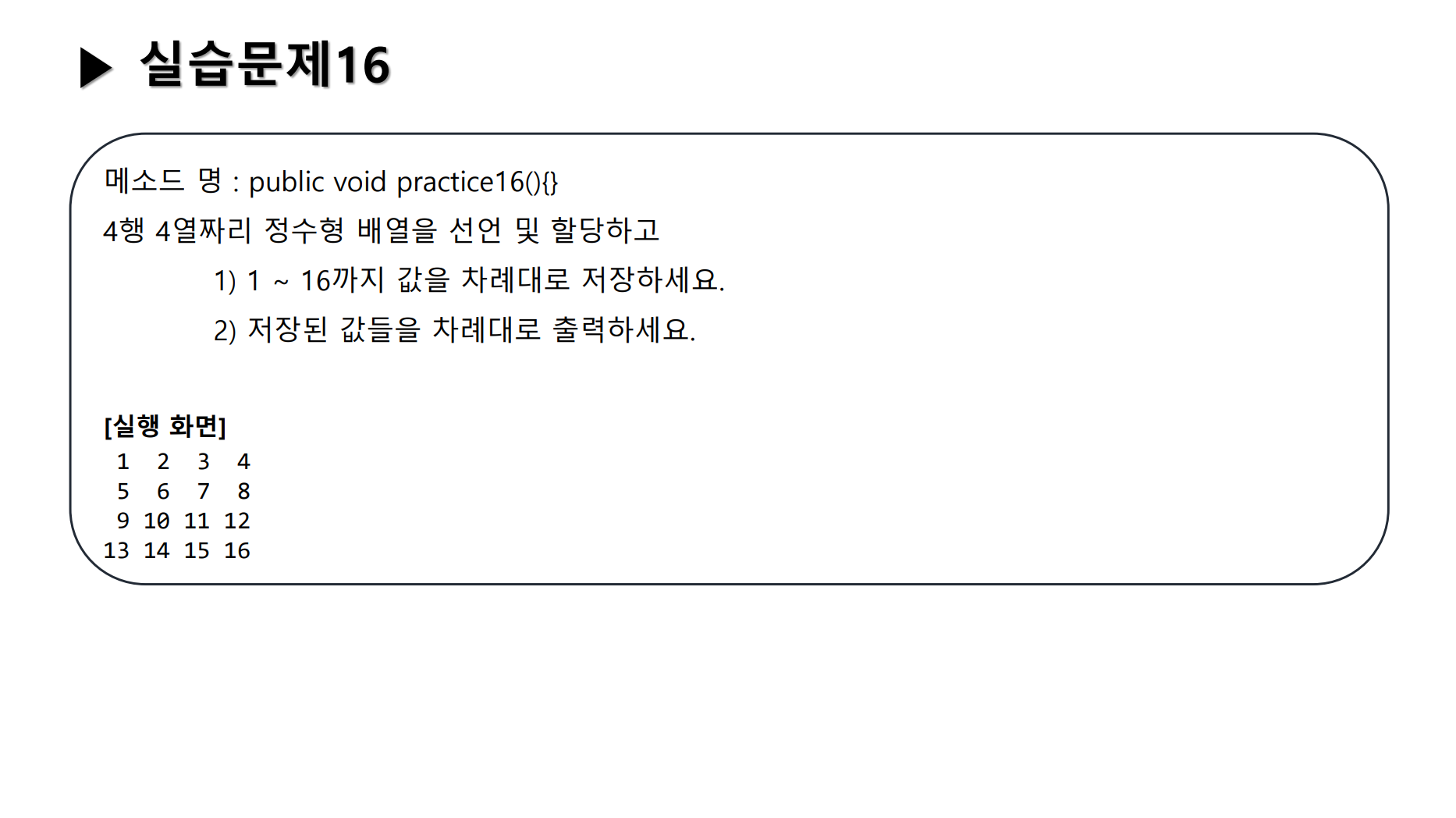
소스 코드
public void practice16() {
int[][] arr = new int[4][4];
int value = 1;
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[i].length; j++) {
arr[i][j] = value++;
}
}
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[i].length; j++) {
System.out.printf("%2d ", arr[i][j]);
}
System.out.println();
}
}
실습 문제 17
🚩 메서드명 : public void practice17 ( )
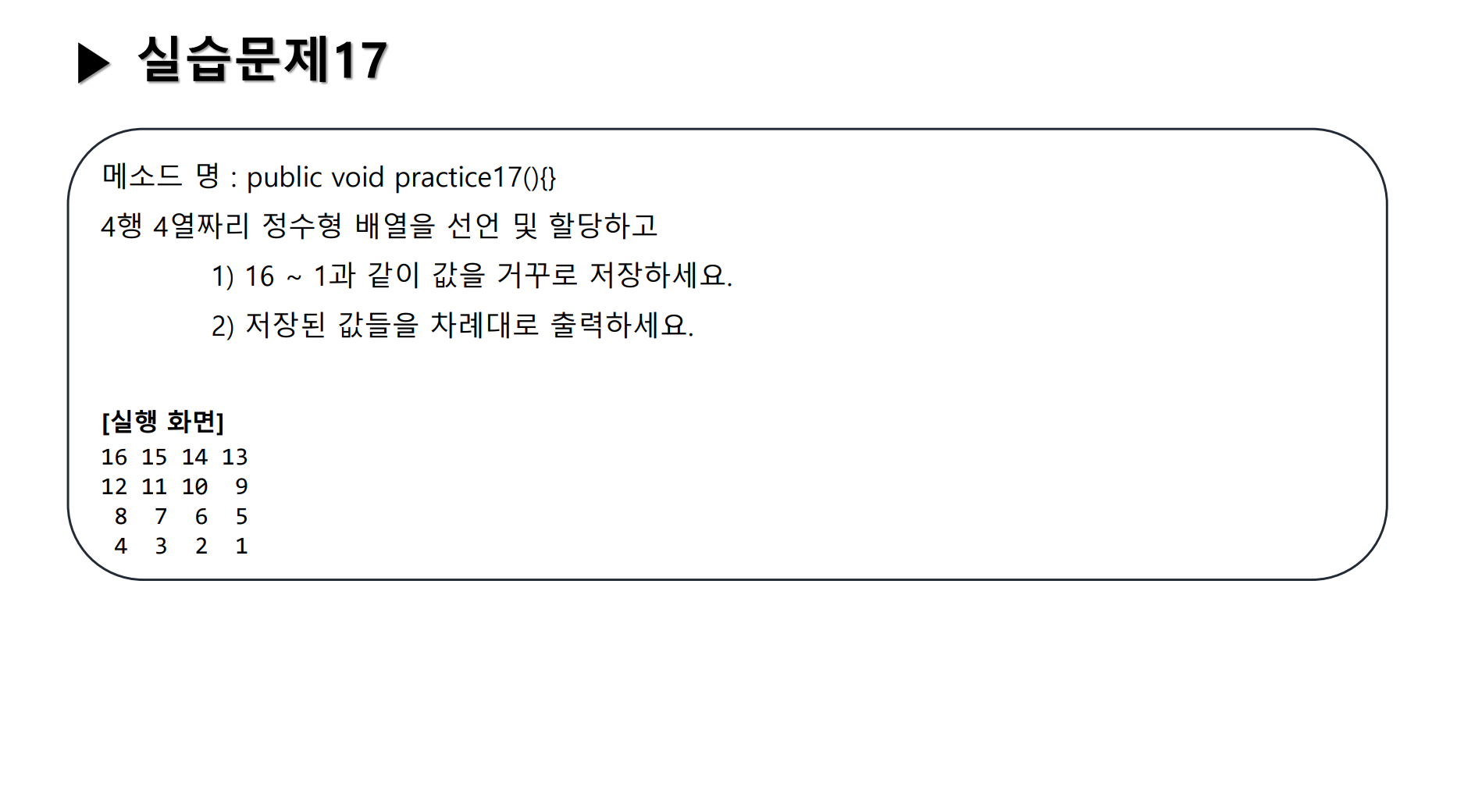
소스 코드
public void practice17() {
int[][] arr = new int[4][4];
int value = 16;
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[i].length; j++) {
arr[i][j] = value--;
}
}
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[i].length; j++) {
System.out.printf("%2d ", arr[i][j]);
}
System.out.println();
}
}
실습 문제 18
🚩 메서드명 : public void practice18 ( )
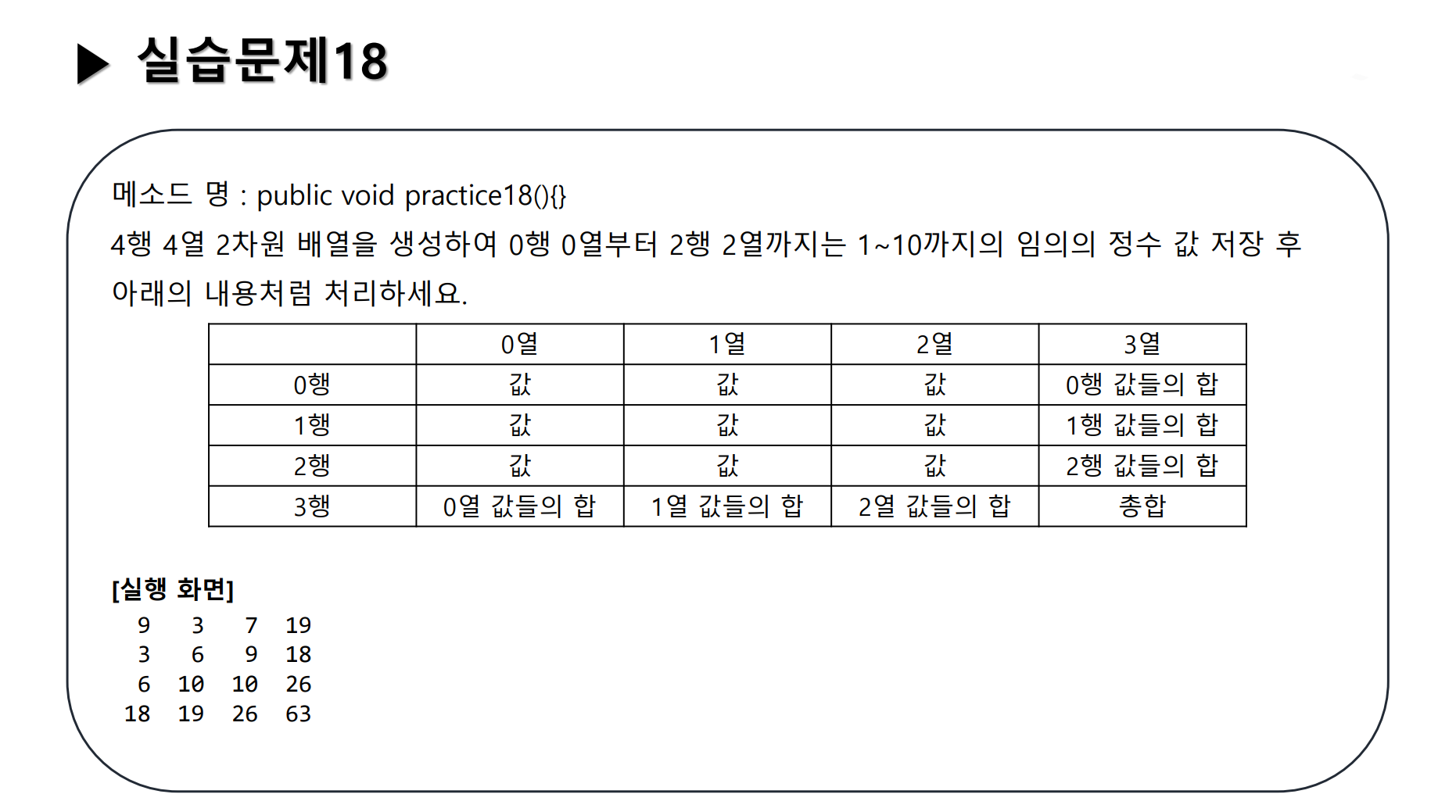
소스 코드
public void practice18() {
int[][] arr = new int[4][4];
final int LAST_ROW_INDEX = arr.length - 1;
final int LAST_COL_INDEX = arr[0].length - 1;
Random random = new Random();
for (int row = 0; row < LAST_ROW_INDEX; row++) {
for (int col = 0; col < LAST_COL_INDEX; col++) {
arr[row][col] = random.nextInt(10) + 1;
arr[LAST_ROW_INDEX][LAST_COL_INDEX] += arr[row][col];
arr[row][LAST_COL_INDEX] += arr[row][col];
arr[LAST_ROW_INDEX][col] += arr[row][col];
}
}
for (int row = 0; row <= LAST_ROW_INDEX; row++) {
for (int col = 0; col <= LAST_COL_INDEX; col++) {
System.out.printf("%3d", arr[row][col]);
}
System.out.println();
}
}
실습 문제 19
🚩 메서드명 : public void practice19 ( )
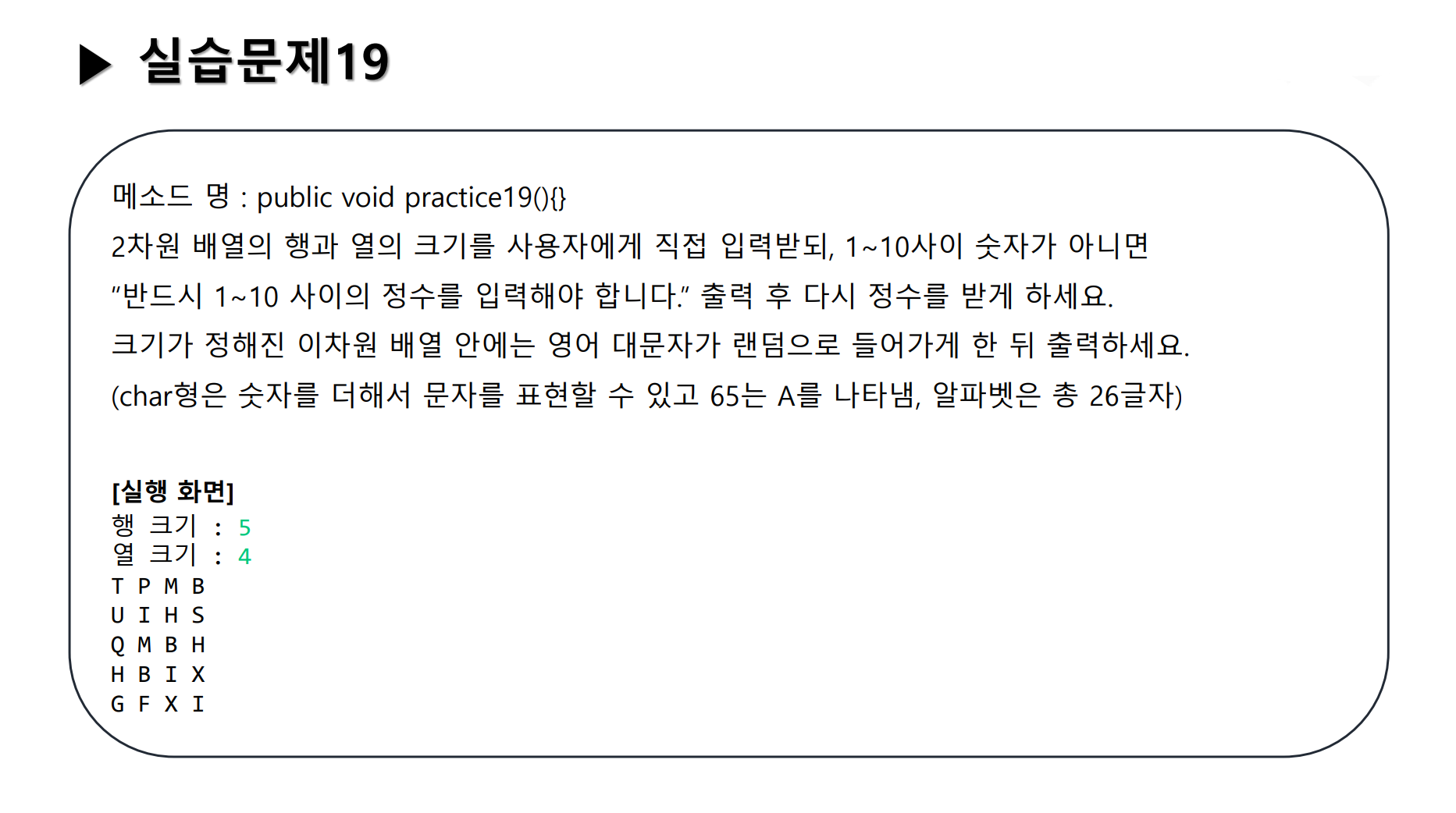
소스 코드
public void practice19() {
Scanner sc = new Scanner(System.in);
while (true) {
System.out.print("행 크기 : ");
int row = sc.nextInt();
System.out.print("열 크기 : ");
int col = sc.nextInt();
if ((row < 1 || row > 10) || (col < 1 || col > 10)) {
System.out.println("반드시 1~10 사이의 정수를 입력해야 합니다.");
} else {
char[][] arr = new char[row][col];
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[i].length; j++) {
arr[i][j] = (char)((int)(Math.random() * 26 + 65));
}
}
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[i].length; j++) {
System.out.print(arr[i][j] + " ");
}
System.out.println();
}
break;
}
}
}
실습 문제 20
🚩 메서드명 : public void practice20 ( )
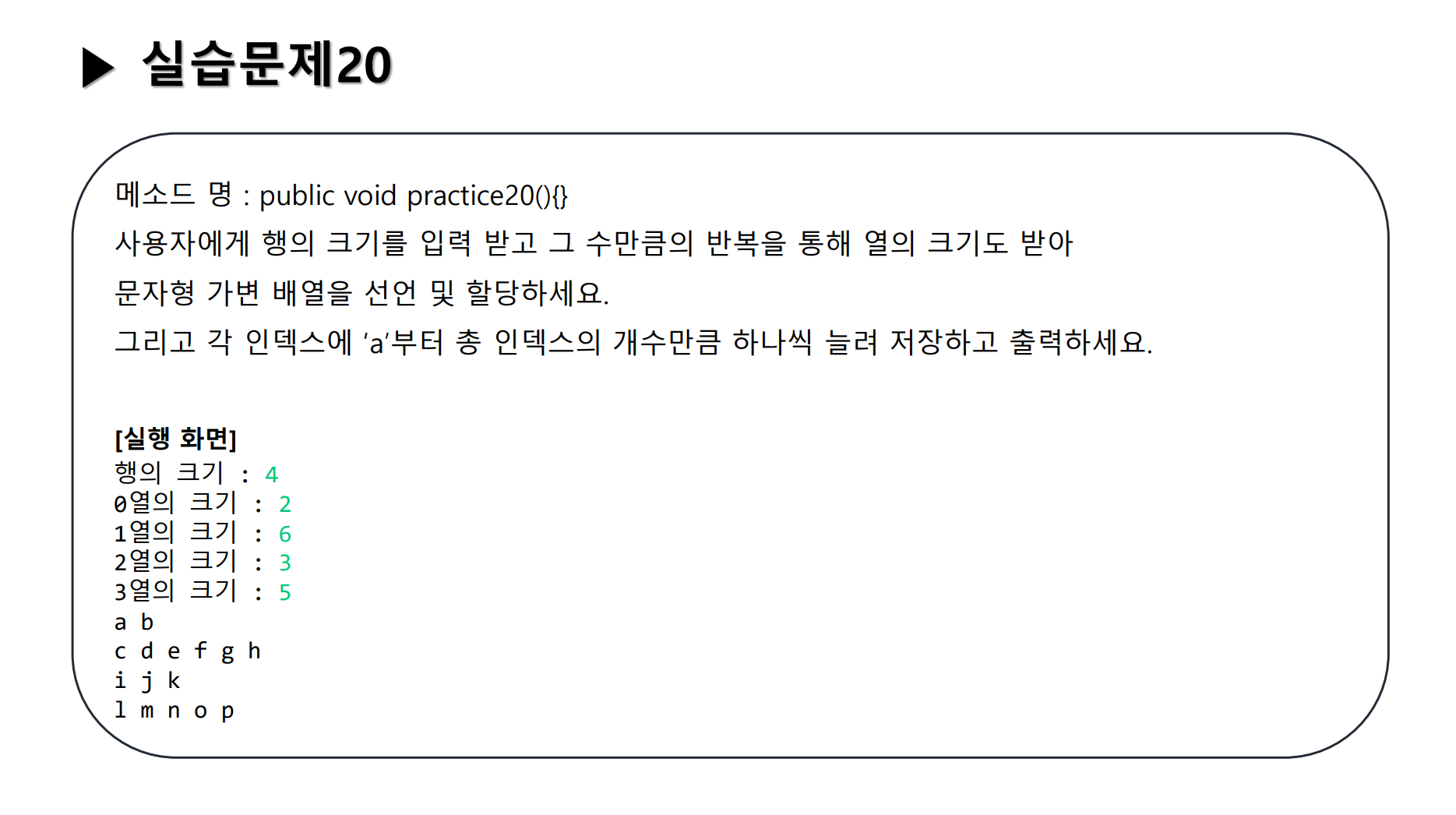
소스 코드
public void practice20() {
Scanner sc = new Scanner(System.in);
System.out.print("행의 크기 : ");
int row = sc.nextInt();
char[][] arr = new char[row][];
for (int i = 0; i < arr.length; i++) {
System.out.print(i + "행의 크기 : ");
int col = sc.nextInt();
arr[i] = new char[col];
}
char value = 'a';
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[i].length; j++) {
arr[i][j] = value++;
}
}
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[i].length; j++) {
System.out.print(arr[i][j] + " ");
}
System.out.println();
}
}
실습 문제 22
🚩 메서드명 : public void practice22 ( )
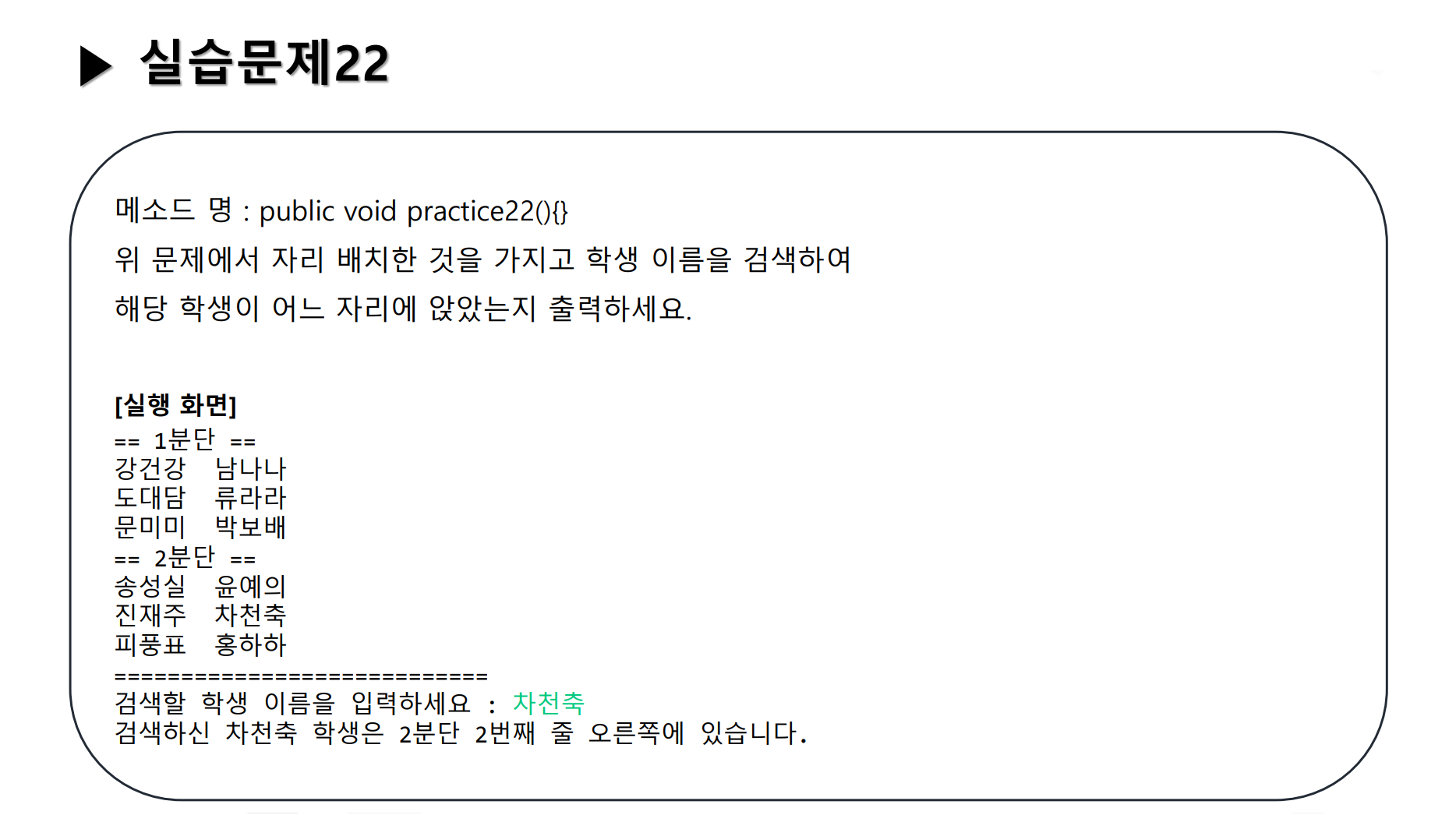
소스 코드
public void practice22() {
Scanner sc = new Scanner(System.in);
String[] students = { "강건강", "남나나", "도대담", "류라라", "문미미", "박보배", "송성실", "윤예의", "진재주", "차천축", "피풍표", "홍하하" };
String[][] seat1 = new String[3][2];
String[][] seat2 = new String[3][2];
int index = 0;
System.out.println("== 1분단 ==");
for (int i = 0; i < seat1.length; i++) {
for (int j = 0; j < seat1[i].length; j++) {
seat1[i][j] = students[index++];
System.out.print(seat1[i][j] + " ");
}
System.out.println();
}
System.out.println("== 2분단 ==");
for (int i = 0; i < seat2.length; i++) {
for (int j = 0; j < seat2[i].length; j++) {
seat2[i][j] = students[index++];
System.out.print(seat2[i][j] + " ");
}
System.out.println();
}
System.out.println("============================");
System.out.print("검색할 학생 이름을 입력하세요 : ");
String searchName = sc.next();
int seat = 0;
int row = 0;
String direction = null;
for (int i = 0; i < seat1.length; i++) {
for (int j = 0; j < seat1[i].length; j++) {
if (seat1[i][j].equals(searchName)) {
seat = 1;
row = i + 1;
direction = j == 0 ? "왼쪽" : "오른쪽";
} else if (seat2[i][j].equals(searchName)) {
seat = 2;
row = i + 1;
direction = j == 0 ? "왼쪽" : "오른쪽";
}
}
}
if (seat != 0) {
System.out.printf("검색하신 %s 학생은 %d분단 %d번째 줄 %s에 있습니다.\n", searchName, seat, row, direction);
} else {
System.out.println("검색한 학생이 존재하지 않습니다.");
}
}
실습 문제 24
🚩 메서드명 : public void practice24 ( )
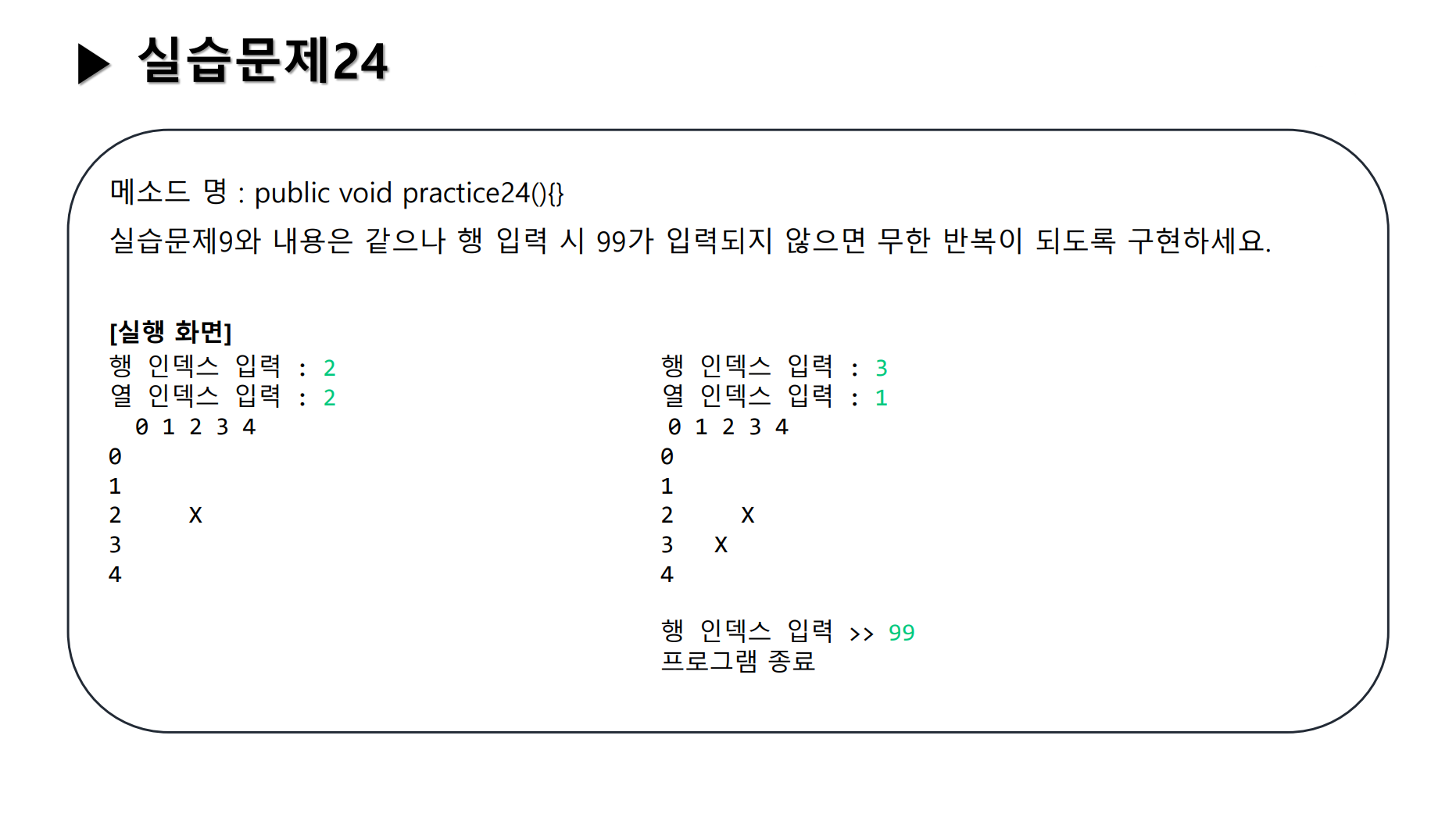
소스 코드
public void practice24() {
Scanner sc = new Scanner(System.in);
String[][] board = new String[6][6];
for (int i = 0; i < board.length - 1; i++) {
board[0][i + 1] = i + "";
board[i + 1][0] = i + "";
}
int rowIndex = 0;
int colIndex = 0;
while (true) {
while (true) {
System.out.print("행 인덱스 입력 : ");
rowIndex = sc.nextInt();
if (rowIndex == 99) {
break;
}
if (rowIndex < 0 || rowIndex > 4) {
System.out.println("0에서 4사이 인덱스를 입력해주세요.");
continue;
}
break;
}
if (rowIndex == 99) {
System.out.println("프로그램 종료");
break;
}
while (true) {
System.out.print("열 인덱스 입력 : ");
colIndex = sc.nextInt();
if (colIndex < 0 || colIndex > 4) {
System.out.println("0에서 4사이 인덱스를 입력해주세요.");
continue;
}
break;
}
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[i].length; j++) {
if (i == rowIndex && j == colIndex) {
board[i + 1][j + 1] = "X";
}
if (board[i][j] == null) {
board[i][j] = " ";
}
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
}
실습 문제 25
🚩 메서드명 : public void practice25 ( )
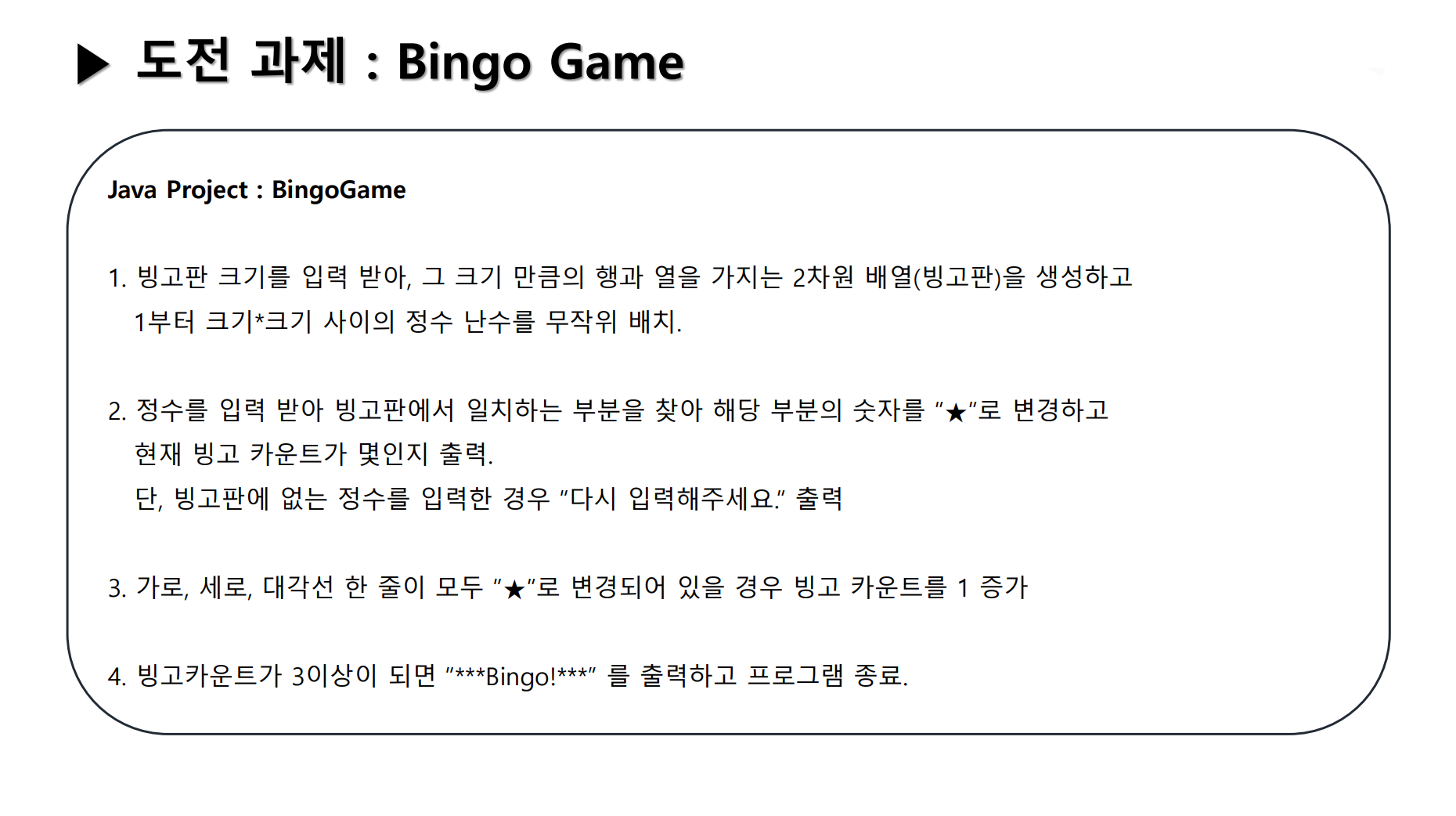
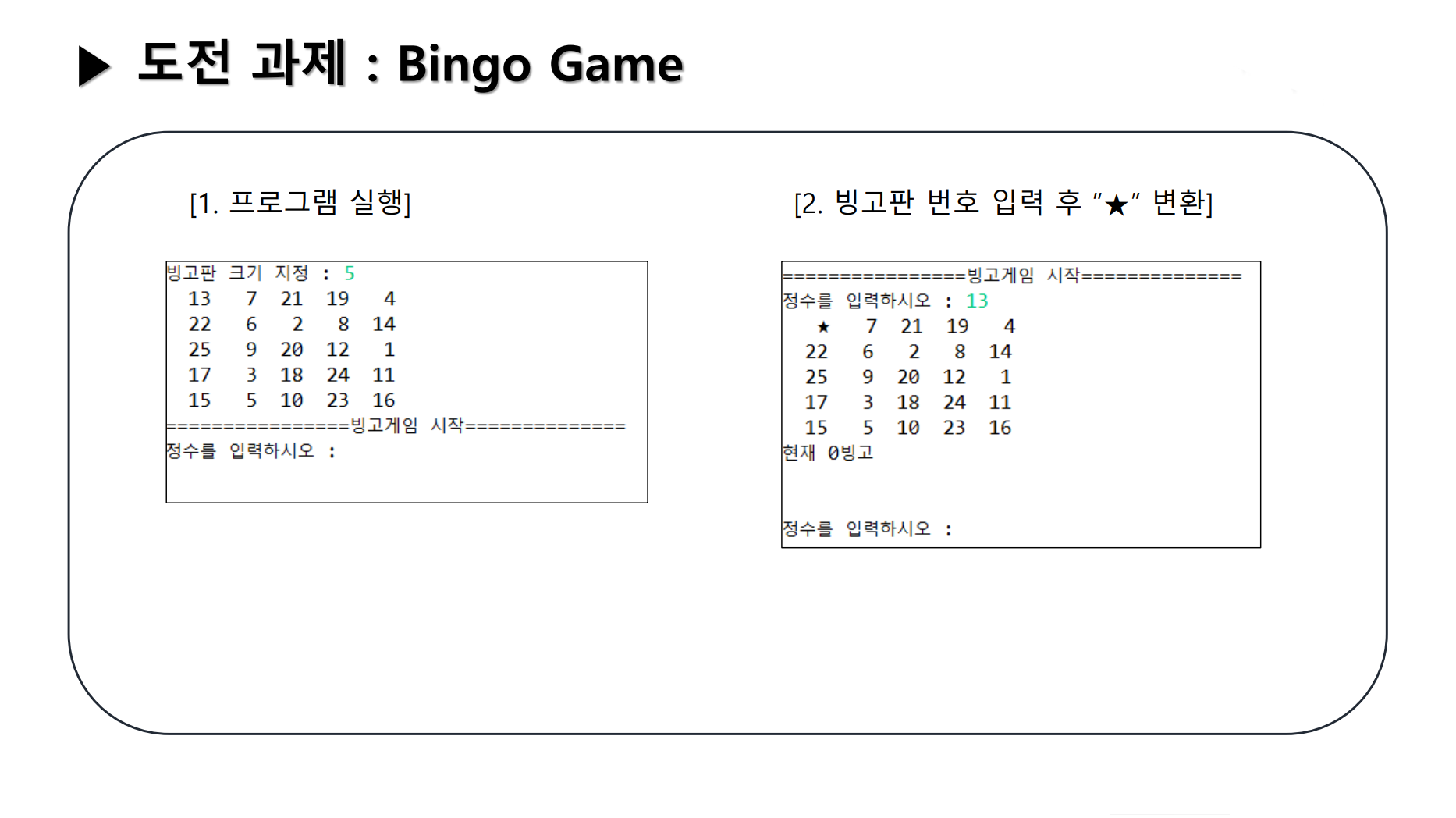
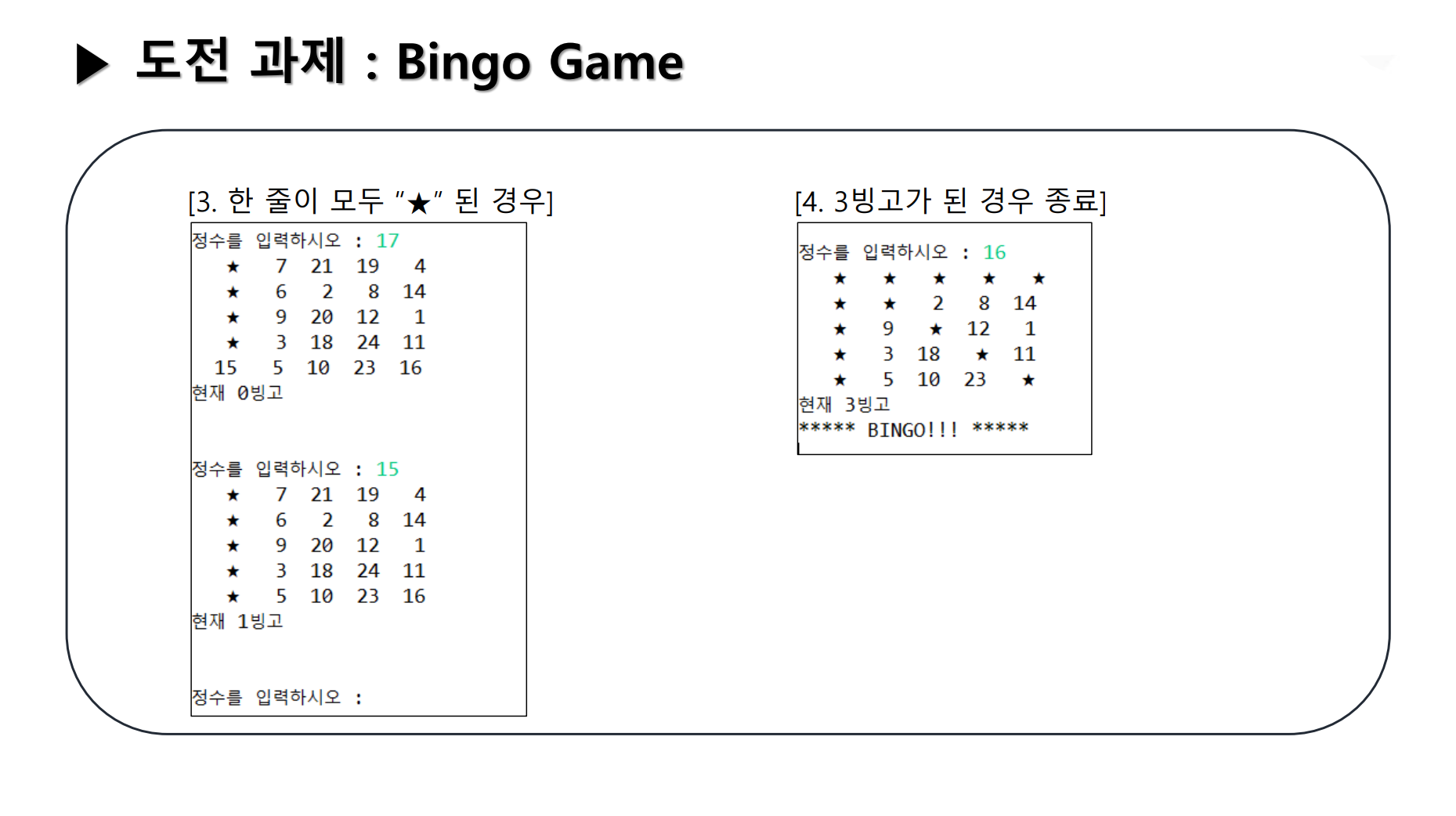
소스 코드
public void practice25() {
Scanner sc = new Scanner(System.in);
System.out.print("빙고판 크기 지정 : ");
int num = sc.nextInt();
int arr[] = new int[num * num];
for (int i = 0; i < arr.length; i++) {
arr[i] = (int)(Math.random() * arr.length) + 1;
for (int j = 0; j < i; j++) {
if (arr[i] == arr[j]) {
i--;
break;
}
}
}
int bingo[][] = new int[num][num];
int index = 0;
for (int i = 0; i < num; i++) {
for (int j = 0; j < num; j++) {
bingo[i][j] = arr[index++];
System.out.print(bingo[i][j] + "\t");
}
System.out.println();
}
System.out.println("========== 빙고 게임 시작 ==========");
while (true) {
System.out.print("정수를 입력하시오 : ");
int drop = sc.nextInt();
for (int i = 0; i < num; i++) {
for (int j = 0; j < num; j++) {
if (bingo[i][j] == drop)
bingo[i][j] = 0;
System.out.print(bingo[i][j] + "\t");
}
System.out.println();
}
int left = 0;
int right = 0;
int count = 0;
for (int i = 0; i < num; i++) {
int row = 0;
int col = 0;
for (int j = 0; j < num; j++) {
if (bingo[i][j] == 0) row++;
if (bingo[j][i] == 0) col++;
if (i == j && bingo[i][j] == 0) left++;
if ((i + j) == num - 1 && bingo[i][j] == 0) right++;
}
if (row == num) count++;
if (col == num) count++;
}
if (left == num) count++;
if (right == num) count++;
System.out.printf("현재 %d빙고\n\n", count);
if (count >= 3) break;
}
System.out.println("***** BINGO!!! *****");
}