📍 Javascript를 공부했을 때 학습노트에 정리해둔 것을 옮겨왔다.
<navigator.geolocation.()>
geolocation은 객체(object)인데 두가지 함수를 가지고 있다.
navigator.geolocation.getCurrentPosition()
navigator.geolocation.watchPosition()
Step 1. User 의 location 좌표 정보를 읽기(가져오기)
const COORDS = 'coords';
/* getCurrentPosition( , )에서 좌표를 가져오는데 성공했을 때를 처리하는 함수 */
function handleGeoSucces(position) {
console.log(position);
}
/* getCurrentPosition( , )에서 좌표를 가져오는데 실패했을 때를 처리하는 함수 */
function handleGeoError(){
console.log("can't accese geo location");
}
/* 좌표를 요청하는 함수 */
function askForCoords() {
/* 위치 정보를 읽게 하는 navigator API 사용하기 */
navigator.geolocation.getCurrentPosition (handleGeoSucces, handleGeoError);
}
function loadCoords() {
const loadedCords = localStorage.getItem(COORDS);
// 만약 loadedCord 가 null 이면
if(loadedCords === null) {
// askForCoords() 함수를 호출한다.
askForCoords();
} else {
// getWeather() 함수를 호출한다.
}
}
function init() {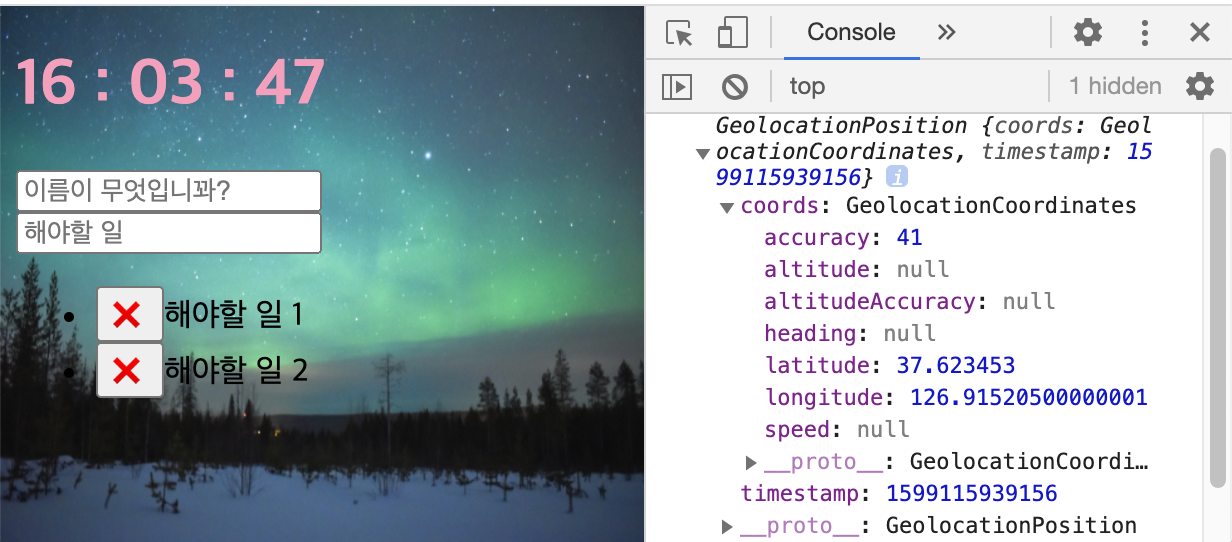
loadCoords();
}
init();
실행 화면 user의 위치를 요청하는 창이 뜬다. 위치 권한을 허용하겠다고 한다면
위치 권한을 차단(거절)한다면
Step 2. User 의 location 좌표 정보를 바탕으로 좌표 설정하기
/ * getCurrentPosition( , )에서 좌표를 가져오는데 성공했을 때를 처리하는 함수 */
function handleGeoSucces(position) {
// (1) position coords의 latitude(위도)를 설정
const latitude = position.coords.latitude;
// (2) position coords의 longitude(경도)를 설정
const longitude = position.coords.longitude;
// (3) 객체(object) 코드를 작성하자
const coordsObj = {
latitude : latitude,
longitude : longitude
};
}
Tip.
(3) 의 coordsObj 객체(object) 를 작성할 때, 변수의 이름과 coordsObj 객체(object)의 key 값의 이름을 같게 저장할 때는const coordsObj = { latitude, longitude };
로 작성할 수도 있다.
Step 3. User 의 location에서 가져온 좌표를 local storage에 저장하기
/* handleGeoSucces(position) 함수에서 가져온 좌표를 저장하는 함수 */
function saveCoods(coordsObj){
localStorage.setItem(COORDS, JSON.stringify(coordsObj));
/* localStorage.setItem로 저장하되, stringify를 이용해서 string으로 변환하는 JSON 인자를 실행 한다.*/
}
/ * getCurrentPosition( , )에서 좌표를 가져오는데 성공했을 때를 처리하는 함수 */
function handleGeoSucces(position) {
const latitude = position.coords.latitude;
const longitude = position.coords.longitude;
const coordsObj = {
latitude,
longitude
};
// 가져온 좌표를 저장하는 함수 실행
saveCoods(coordsObj);
}
실행 결과 (좌표 동의)