Python 내장함수, 자료형, 정규표현식, 모듈...etc
sorted() vs list.sort()
>>> a = [234,35,1]
>>> sorted(a)
[1, 35, 234]
>>> a
[234, 35, 1]
>>> a.sort()
>>> a
[1, 35, 234]
Dictionary (key, value 형식)
>>> a = {}
>>> a[3] = [1,2,3]
>>> a[3][1]
2
>>> a[3][0]
1
>>> a[2] = {1: 'a'}
>>> a
{3: [1, 2, 3], 2: {1: 'a'}}
>>> a[2][1]
'a'
>>> del a[3]
>>> a
{2: {1: 'a'}}
Dictionary 내장함수 (keys(), values(), get(), clear())
>>> test = {1: 123, 2 : 2345, 3: 32435}
>>> test
{1: 123, 2: 2345, 3: 32435}
>>> test.keys()
dict_keys([1, 2, 3])
>>> len(test)
3
>>> for i in test.keys():
print(i)
1
2
3
>>> a = list(test.keys())
>>> a
[1, 2, 3]
>>> test.values()
dict_values([123, 2345, 32435])
>>> for i in test.values():
print(i)
123
2345
32435
>>> for i in test.items():
print(i)
type(i)
i[0]
i[1]
(1, 123)
<class 'tuple'>
1
123
(2, 2345)
<class 'tuple'>
2
2345
(3, 32435)
<class 'tuple'>
3
32435
>>> test.get(1)
123
Dictionary 값 추가 & 삭제 (update(), pop())
>>> test.update(age = 10, height = 100)
>>> test
{'age': 10, 'height': 100} # key 문자열로 인식
>>> test.update({2018: 2022})
>>> test.pop(2018)
2022
>>> test
{'age': 10, 'height': 100}
lamda 표현식
>>> a = lamda x:x+3
>>> a(1)
4
map(함수, list) and list comprehension
>>> a = [2,4,6,8,10]
>>> c = list(map((lambda x:x//2), a))
>>> c
[1, 2, 3, 4, 5]
>>> list(map(lambda x,y: x+y, a, c))
[140, 188, 236]
>>> c = [x//2 for x in a]
>>> c
[1, 2, 3, 4, 5]
#### 가독성이 떨어지지만 아래와 같이 표현도 가능하다
>>> d = list(map(lambda x:print(x) if x%2==0 else x+1, a))
2
4
6
8
10
>>> c = list(filter(lambda x:x>3, a))
>>> c
[4, 6, 8, 10]
>>> c = [x*23 for x in a if x>4]
>>> c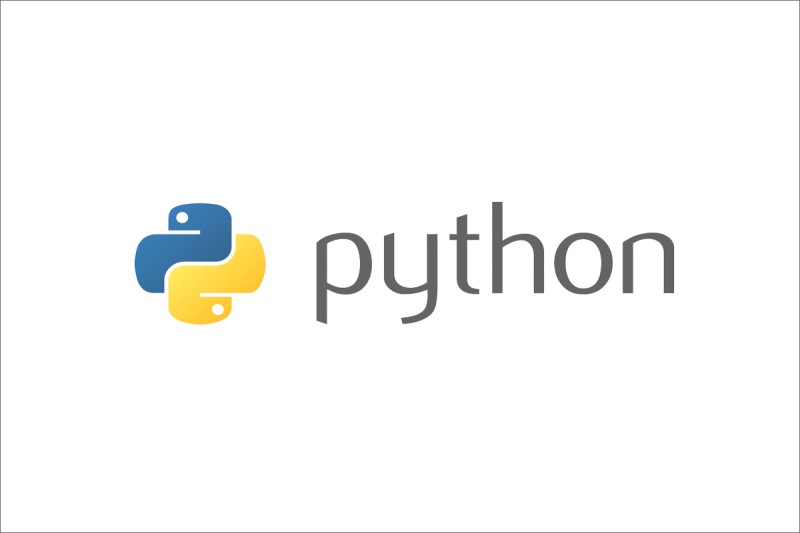
[138, 184, 230]
>>>
>>> from functools import reduce
>>> reduce(lambda x,y:x*y, a)
3840
>>> reduce(lambda x,y:x*y, a, 2)
7680
>>> reduce(lambda x,y:x*y, a, 0)
0
>>>
>>> c = [x*2 if x>4 else x*5 for x in range(1,10)]
>>> c
[5, 10, 15, 20, 10, 12, 14, 16, 18]