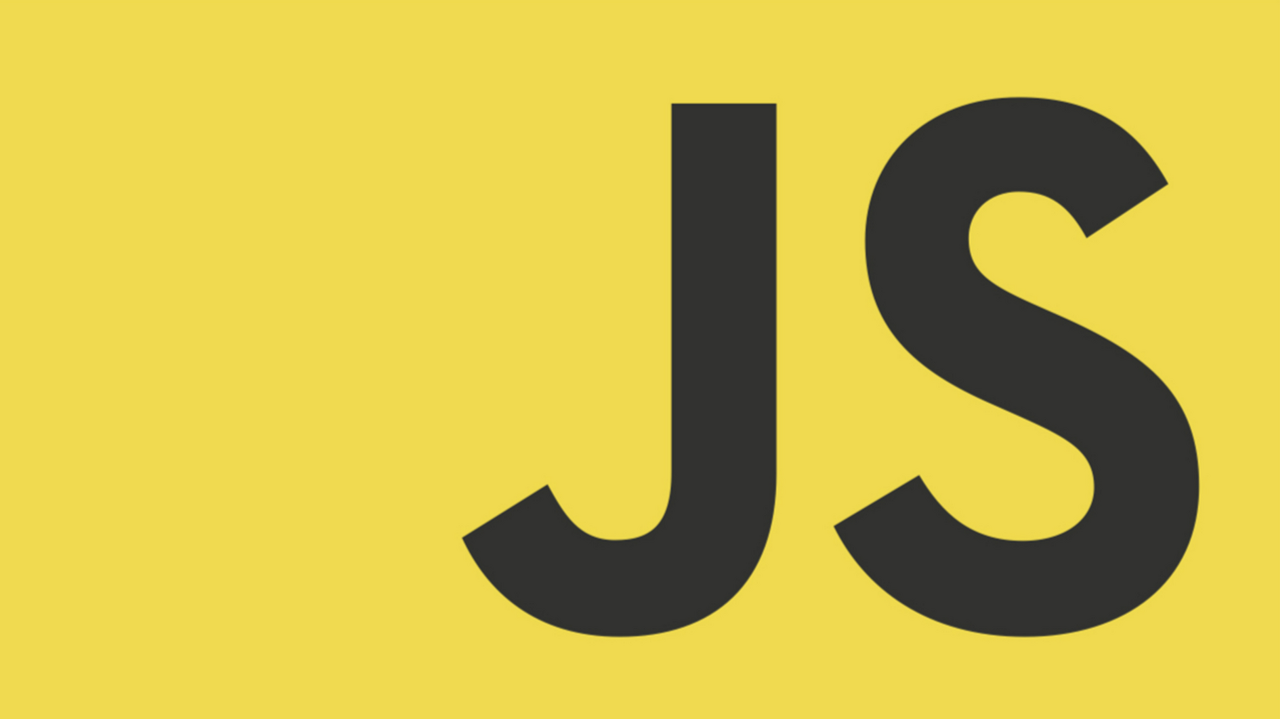
String
- 텍스트 길이에 상관없이 문자열 형태로 저장되는 자료형
- 자바스크립트에서는 글자 하나만 저장할 수 있는 Char 자료형이 없음
- 자바스크립트에서 문자열은 페이지 인코딩 방식과 상관없이 항상 UTF-16 형식을 따름
대표 속성(property)와 메서드(method)
- 문자열 길이 : String.length
- 문자열 접근 : String.CharAt(index), String.charCodeAt(index)
- 문자열 검색 : String.indexOf(), String.lastIndexOf(), String.includes()
- 문자열 변환 : String.toUpperCase(), String.toLowerCase()
- 문자열 치환 : String.replace()
- 문자열 추출 : String.slice(), String.substring(), String.substr()
- 문자열 분할 : String.split()
문자열 길이, 문자열 접근
<script>
let str = 'hello world !'
1. console.log(str.length)
2. console.log(str.charAt(1))
3. console.log(str.charCodeAt(1))
4. console.log(str[1])
</script>
- str.length : 문자열의 길이를 반환합니다.(띄어쓰기, !도 포함한다.)
- str.charAt(index) : 문자열의 index 위치에 있는 문자를 반환합니다.
- str.charCodeAt(index) 메서드는 주어진 인덱스에 대한 UTF-16 코드를 나타내는 0부터 65535 사이의 정수를 반환합니다.
- str[index] : str 문자열의[index] 위치에 있는 문자를 반환합니다.
문자열 검색
<script>
let str = 'hello world !'
1. console.log(str.indexOf('o'))
2. console.log(str.indexOf('o', 5))
3. console.log(str.lastIndexOf('o'))
4. console.log(str.includes('hello'))
</script>
- str.indexof('o') : str 배열에서 요소의 값 'o'가 들어있는 첫 index를 반환한고 존재하지 않으면 -1을 반환한다.
- str.indexof('o' [, fromIndex]) : 1번과 마찬가지이지만, fromIndex의 위치부터 실행된다.
- str.lastIndexof('o') : 주어진 값과 일치하는 부분을 배열의 맨 뒷부분부터 역순으로 탐색하여 최초로 마주치는 인덱스를 반환한다. 일치하는 부분을 찾을 수 없으면 -1을 반환한다.
- str.includes('hello') : str 배열이 요소 'hello'를 포함 하고 있는지 판별한다. 포함 하고있으면 true, 그렇지 않으면 false를 반환한다.
문자열 변환, 문자열 치환
<script>
let str = 'hello world !'
1. console.log(str.toUpperCase())
2. console.log(str.toLowerCase())
3. console.log(str.replace('l', 'o'))
let str_change = str.replace('world', 'earth')
4. console.log(str_change)
5. console.log(str)
</script>
- str.toUpperCase() : str 문자열의 모든 요소를 대문자로 치환.
- str.toLowerCase() : str 문자열의 모든 요소를 소문자로 치환.
- str.replace('l', 'o') str 문자열의 요소 'l'을 찾아 첫번째만 'o'로 바꾼다.
- str.replace에서 실행한 결과를 담은 str_change은 'hello earth !'가 출력된다.
- str에는 원본 그대로인 'hello world !'가 출력된다.
문자열 추출, 문자열 분할
<script>
let str = 'hello world !'
1. console.log(str.slice(0, 5))
2. console.log(str.slice(0))
3. console.log(str.slice(-5,-3))
4. console.log(str.slice(-5))
</script>
- str.slice(0, 5) : str 문자열의 index 0번째부터 5 - 1번째 index까지의 요소를 반환한다.
- str.slice(0) : str 문자열의 index 0번째부터 마지막까지의 요소를 반환한다.
index -1의 위치는 요소의 마지막 위치이다.
- str.slice(-5, -3) : str 문자열의 index -5번째부터 -3번째까지의 요소를 반환한다.
- str.slice(-5) : str 문자열의 index -5번째부터 마지막까지의 요소를 반환한다.
<script>
let str = 'hello world !'
1. console.log(str.substring(0, 5));
console.log(str.substring(5, 0));
</script>
- str.substring(indexStart[, indexEnd]) : 문자열의 indexStart 지점부터 indexEnd - 1 지점까지의 요소를 반환한다.
indexEnd가 없을 시에는 indexStart지점부터 마지막까지 반환한다.
indexStart가 indexEnd보다 크면 서로의 위치를 바꿔서 진행한다.
<script>
let str = 'hello world !'
1. console.log(str.substr(3, 2));
</script>
- str.substr(start[, length]) : 문자열의 Start 지점부터 length 길이 만큼의 요소를 반환한다.
<script>
let fruit = 'apple banana melon'
result = fruit.split(' ')
console.log(result)
console.log(result[0])
console.log(result[1])
console.log(result[2])
</script>