코드
import cv2
import mediapipe as mp
import numpy as np
from sklearn.neighbors import KNeighborsClassifier
spider = cv2.imread('./data/spider.jpg')
spider = cv2.resize(spider, (250,250))
mask2gray_spider = cv2.cvtColor(spider, cv2.COLOR_RGB2GRAY)
_, mask_b_spider = cv2.threshold(mask2gray_spider, 200, 255, cv2.THRESH_BINARY)
mask_b_inv_spider = cv2.bitwise_not(mask_b_spider)
img_fg_spider = cv2.bitwise_and(spider,spider,mask = mask_b_inv_spider)
mask = cv2.imread('./data/mask.jpg')
mask2gray_mask = cv2.cvtColor(mask, cv2.COLOR_RGB2GRAY)
_, mask_b_mask = cv2.threshold(mask2gray_mask, 250, 255, cv2.THRESH_BINARY)
mask_b_inv_mask = cv2.bitwise_not(mask_b_mask)
img_fg_mask = cv2.bitwise_and(mask,mask,mask = mask_b_inv_mask)
iron = cv2.imread('./data/ironman.jpg')
mask2gray_iron = cv2.cvtColor(iron, cv2.COLOR_RGB2GRAY)
_, mask_b_iron = cv2.threshold(mask2gray_iron, 200, 255, cv2.THRESH_BINARY)
mask_b_inv_iron = cv2.bitwise_not(mask_b_iron)
img_fg_iron = cv2.bitwise_and(iron,iron,mask = mask_b_inv_iron)
hulk = cv2.imread('./data/hulk.jpg')
mask2gray_hulk = cv2.cvtColor(hulk, cv2.COLOR_RGB2GRAY)
_, mask_b_hulk = cv2.threshold(mask2gray_hulk, 200, 255, cv2.THRESH_BINARY)
mask_b_inv_hulk = cv2.bitwise_not(mask_b_hulk)
img_fg_hulk = cv2.bitwise_and(hulk,hulk,mask = mask_b_inv_hulk)
sun = cv2.imread('./data/sunglass.jpg')
sun = cv2.resize(sun,(180,120))
mask2gray_sun = cv2.cvtColor(sun, cv2.COLOR_RGB2GRAY)
_, mask_b_sun = cv2.threshold(mask2gray_sun, 200, 255, cv2.THRESH_BINARY)
mask_b_inv_sun = cv2.bitwise_not(mask_b_sun)
img_fg_sun = cv2.bitwise_and(sun,sun,mask = mask_b_inv_sun)
video = cv2.VideoCapture(0)
cnt = 0
fps = 20
fcc = cv2.VideoWriter_fourcc(*'DIVX')
width = int(video.get(3))
height = int(video.get(4))
gesture = {
0:'fist', 1:'one', 2:'two', 3:'three', 4:'four', 5:'five',
6:'six', 7:'rock', 8:'spiderman', 9:'yeah', 10:'ok',
}
file = np.genfromtxt('./data/gesture_train.csv',delimiter = ',')
X = file[:, :-1].astype(np.float32)
y = file[:, -1].astype(np.float32)
knn = KNeighborsClassifier(n_neighbors = 3)
knn.fit(X,y)
mp_hands = mp.solutions.hands
mp_drawing = mp.solutions.drawing_utils
hands = mp_hands.Hands(
max_num_hands = 1,
min_detection_confidence = 0.5,
min_tracking_confidence = 0.5
)
mp_face = mp.solutions.face_mesh
face = mp_face.FaceMesh(
min_detection_confidence = 0.5,
min_tracking_confidence = 0.5
)
video = cv2.VideoCapture(0)
while video.isOpened():
ret, img = video.read()
img = cv2.flip(img,1)
img = cv2.cvtColor(img,cv2.COLOR_BGR2RGB)
result = hands.process(img)
img = cv2.cvtColor(img,cv2.COLOR_RGB2BGR)
if not ret:
break
if result.multi_hand_landmarks is not None:
for res in result.multi_hand_landmarks:
joint = np.zeros((21,3))
for j , lm in enumerate(res.landmark):
joint[j] = [lm.x, lm.y, lm.z]
v1 = joint[[0,1,2,3,0,5,6,7,0,9,10,11,0,13,14,15,0,17,18,19],:]
v2 = joint[[1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20],:]
v = v2-v1
v = v / np.linalg.norm(v, axis = 1)[:, np.newaxis]
angle = np.arccos(np.einsum('nt,nt->n',
v[[0,1,2,4,5,6,8,9,10,12,13,14,16,17,18],:],
v[[1,2,3,5,6,7,9,10,11,13,14,15,17,18,19],:]))
angle = np.degrees(angle)
X_pred = np.array([angle], dtype = np.float32)
results = knn.predict(X_pred)
idx = int(results)
img_x = img.shape[1]
img_y = img.shape[0]
hand_x = res.landmark[0].x
hand_y = res.landmark[0].y
if idx == 0:
cv2.putText(img, text = 'Mask',
org = ( int(hand_x * img_x) , int(hand_y * img_y)+20 ),
fontFace=cv2.FONT_HERSHEY_SIMPLEX, fontScale=1, color=(255, 255, 255), thickness=2
)
face_result = face.process(img)
if face_result.multi_face_landmarks is not None:
lip = face_result.multi_face_landmarks[0].landmark[0]
x_lip = int(lip.x * img.shape[1])
y_lip = int(lip.y * img.shape[0])
try :
roi = img[y_lip -112 :y_lip + 113, x_lip -112 : x_lip + 113]
img_bg = cv2.bitwise_and(roi,roi,mask=mask_b_mask)
bg_fg = cv2.add(img_bg, img_fg_mask)
img[y_lip -112 :y_lip + 113, x_lip -112 : x_lip + 113] = bg_fg
except :
pass
elif idx == 7 or idx == 8:
cv2.putText(img, text = 'SpiderMan',
org = ( int(hand_x * img_x) , int(hand_y * img_y)+20 ),
fontFace=cv2.FONT_HERSHEY_SIMPLEX, fontScale=1, color=(255, 255, 255), thickness=2
)
face_result = face.process(img)
if face_result.multi_face_landmarks is not None:
nose = face_result.multi_face_landmarks[0].landmark[4]
x_nose = int(nose.x * img.shape[1])
y_nose = int(nose.y * img.shape[0])
try :
roi = img[y_nose -125 :y_nose + 125, x_nose -125 : x_nose + 125]
img_bg = cv2.bitwise_and(roi,roi,mask=mask_b_spider)
bg_fg = cv2.add(img_bg, img_fg_spider)
img[y_nose -125 :y_nose + 125, x_nose -125 : x_nose + 125] = bg_fg
except :
pass
elif idx == 9:
cv2.putText(img, text = 'Sunglass',
org = ( int(hand_x * img_x) , int(hand_y * img_y)+20 ),
fontFace=cv2.FONT_HERSHEY_SIMPLEX, fontScale=1, color=(255, 255, 255), thickness=2
)
elif idx == 5:
cv2.putText(img, text = 'Iron Man',
org = ( int(hand_x * img_x) , int(hand_y * img_y)+20 ),
fontFace=cv2.FONT_HERSHEY_SIMPLEX, fontScale=1, color=(255, 255, 255), thickness=2
)
face_result = face.process(img)
if face_result.multi_face_landmarks is not None:
nose = face_result.multi_face_landmarks[0].landmark[4]
x_nose = int(nose.x * img.shape[1])
y_nose = int(nose.y * img.shape[0])
try :
roi = img[y_nose -112 :y_nose + 113, x_nose -112 : x_nose + 113]
img_bg = cv2.bitwise_and(roi,roi,mask=mask_b_iron)
bg_fg = cv2.add(img_bg, img_fg_iron)
img[y_nose -112 :y_nose + 113, x_nose -112 : x_nose + 113] = bg_fg
except :
pass
elif idx == 10:
cv2.putText(img, text = 'Hulk',
org = ( int(hand_x * img_x) , int(hand_y * img_y)+20 ),
fontFace=cv2.FONT_HERSHEY_SIMPLEX, fontScale=1, color=(255, 255, 255), thickness=2
)
face_result = face.process(img)
if face_result.multi_face_landmarks is not None:
nose = face_result.multi_face_landmarks[0].landmark[4]
x_nose = int(nose.x * img.shape[1])
y_nose = int(nose.y * img.shape[0])
try :
roi = img[y_nose -112 :y_nose + 113, x_nose -112 : x_nose + 113]
img_bg = cv2.bitwise_and(roi,roi,mask=mask_b_hulk)
bg_fg = cv2.add(img_bg, img_fg_hulk)
img[y_nose -112 :y_nose + 113, x_nose -112 : x_nose + 113] = bg_fg
except :
pass
mp_drawing.draw_landmarks(img, res, mp_hands.HAND_CONNECTIONS)
k = cv2.waitKey(30)
if k == 49:
break
elif k == 50:
cv2.imwrite(f'./data/cap_sun{cnt}.png', img, params=[cv2.IMWRITE_PNG_COMPRESSION, 0] )
cnt += 1
elif k == 51:
out = cv2.VideoWriter('./data/sun.avi', fcc, fps, (width, height))
record = True
elif k == 52:
record = False
out.release()
cv2.imshow('hand',img)
video.release()
cv2.destroyAllWindows()
결과
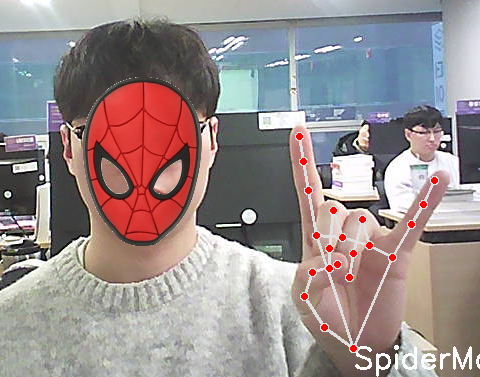
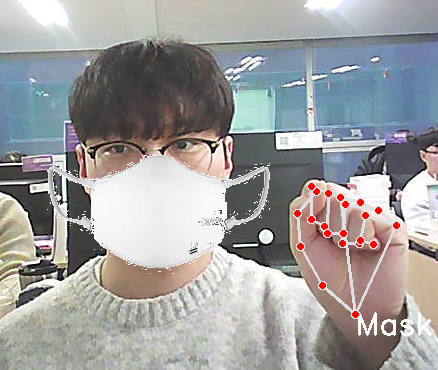