사원관리 시스템
1. View의 역할을 하는 Main class
<style>
package empManage;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
Controller controller = new Controller();
System.out.println("────────▄█▀▄\r\n"
+ "──────▄██▀▀▀▀▄\r\n"
+ "────▄███▀▀▀▀▀▀▀▄\r\n"
+ "──▄████▀▀▀▀▀▀▀▀▀▀▄\r\n"
+ "▄█████▀▀▀▀▀▀▀▀▀▀▀▀▀▄\r\n" \
+ " 피라미드는 사람이 만들었다.\r\n"
+ " (주) 수드라");
while (true) {
System.out.println("0.사원목록 1.등록 2.삭제 3.수정 4.조회 5.휴가 6.종료");
int choice = scanner.nextInt();
if (choice == 0) {
controller.getEmpList();
} else if (choice == 1) {
System.out.println("========노예등록========");
controller.addEmp();
} else if (choice == 2) {
System.out.println("========노예해방========");
System.out.println("사번을 입력하세요 >> ");
controller.delEmp();
} else if (choice == 3) {
System.out.println("=======노예정보수정=======");
System.out.println("사번을 입력하세요 >> ");
controller.updateEmp();
} else if (choice == 4) {
System.out.println("========노예조회========");
System.out.println("사번을 입력하세요 >> ");
controller.searchEmp();
} else if (choice == 5) {
System.out.println("======도망간 노예조회======");
controller.searchVac();
} else if (choice == 6) {
System.out.println("========프로그램을 종료합니다.=======");
scanner.close();
break;
} else {
System.out.println("☠️☠️☠️☠️☠️잘못된 명령어 입니다.☠️☠️☠️☠️☠️");
}
}
}
}
</style>
2. 변수를 묶어주는 Model class 생성
<style>
package empManage;
public class Employee {
private String name;
private int empId;
private String eDate;
private String dpt;
private String rank;
private boolean vac;
private int salary;
public Employee() {
}
public Employee(String name, int empId, String eDate, String dpt, String rank, boolean vac, int salary) {
super();
this.name = name;
this.empId = empId;
this.eDate = eDate;
this.dpt = dpt;
this.rank = rank;
this.vac = vac;
this.salary = salary;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getEmpId() {
return empId;
}
public void setEmpId(int empId) {
this.empId = empId;
}
public String geteDate() {
return eDate;
}
public void seteDate(String eDate) {
this.eDate = eDate;
}
public String getDpt() {
return dpt;
}
public void setDpt(String dpt) {
this.dpt = dpt;
}
public String getRank() {
return rank;
}
public void setRank(String rank) {
this.rank = rank;
}
public boolean isVac() {
return vac;
}
public void setVac(boolean vac) {
this.vac = vac;
}
public int getSalary() {
return salary;
}
public void setSalary(int salary) {
this.salary = salary;
}
@Override
public String toString() {
return "Employee [name=" + name + ", empId=" + empId + ", eDate=" + eDate + ", dpt=" + dpt + ", rank=" + rank
+ ", vac=" + vac + ", salary=" + salary + "]";
}
}
</style>
3. 보이지 않는 곳에서 기능을 수행하는 Controller class 생성
<style>
package empManage;
import java.util.ArrayList;
import java.util.Scanner;
public class Controller {
ArrayList<Employee> empList = new ArrayList<Employee>();
Scanner sc = new Scanner(System.in);
public void getEmpList() {
if (empList.size() == 0) {
System.out.println("사원목록이 없습니다.");
} else {
for (int i = 0; i < empList.size(); i++) {
System.out.print(i + 1 + ". " + empList.get(i).getEmpId() + " ");
System.out.print(empList.get(i).getName() + " ( " + empList.get(i).getDpt());
System.out.println(empList.get(i).getRank() + " ) ");
}
}
}
public void addEmp() {
Employee slave = new Employee();
System.out.println("이름입력 >> ");
slave.setName(sc.next());
System.out.println("사번입력 >> ");
slave.setEmpId(sc.nextInt());
System.out.println("입사일입력 >> ");
slave.seteDate(sc.next());
System.out.println("부서입력 >> ");
slave.setDpt(sc.next());
System.out.println("직급입력 >> ");
slave.setRank(sc.next());
System.out.println("휴가상태입력 >> ");
slave.setVac(sc.nextBoolean());
System.out.println("월급입력 >> ");
slave.setSalary(sc.nextInt());
empList.add(slave);
System.out.println("=======노예등록이 완료되었습니다.=======");
}
public void delEmp() {
int num = sc.nextInt();
for (int i = 0; i < empList.size(); i++) {
if (empList.get(i).getEmpId() == num) {
empList.remove(i);
System.out.println("=======노예가 해방되었습니다.=======");
break;
} else if (empList.get(i).getEmpId() != num) {
if (i == empList.size() - 1) {
System.out.println("=====일치하는 사원이 없습니다.=====");
}
}
}
}
public void updateEmp() {
int num = sc.nextInt();
updat : for (int i = 0; i < empList.size(); i++) {
if (empList.get(i).getEmpId() == num) {
System.out.println(empList.get(i).toString());
System.out.println("수정할 부분을 번호로 입력하세요.");
int cho = sc.nextInt();
switch (cho) {
case (1):
System.out.println("이름을 입력하세요.");
empList.get(i).setName(sc.next());
System.out.println("수정이 완료되었습니다.");
break updat;
case (2):
System.out.println("수정할 사번을 입력하세요.");
empList.get(i).setEmpId(sc.nextInt());
System.out.println("수정이 완료되었습니다.");
break updat;
case (3):
System.out.println("수정할 입사날짜를 입력하세요.");
empList.get(i).seteDate(sc.next());
System.out.println("수정이 완료되었습니다.");
break updat;
case (4):
System.out.println("수정할 부서를 입력하세요.");
empList.get(i).setDpt(sc.next());
System.out.println("수정이 완료되었습니다.");
break updat;
case (5):
System.out.println("수정할 직급을 입력하세요.");
empList.get(i).setRank(sc.next());
System.out.println("수정이 완료되었습니다.");
break updat;
case (6):
System.out.println("휴가중인지 입력하세요.");
empList.get(i).setVac(sc.nextBoolean());
System.out.println("수정이 완료되었습니다.");
break updat;
case (7):
System.out.println("수정할 연봉을 입력하세요.");
empList.get(i).setSalary(sc.nextInt());
System.out.println("수정이 완료되었습니다.");
break updat;
default:
System.out.println("잘못된 명령어입니다.");
}
} else if (empList.get(i).getEmpId() != num) {
if (i == empList.size() - 1) {
System.out.println("=====해당하는 사원이 존재하지 않습니다.=====");
}
}
}
}
public void searchEmp() {
int num = sc.nextInt();
for (int i = 0; i < empList.size(); i++) {
if (empList.get(i).getEmpId() == num) {
System.out.print(i + 1 + ". " + empList.get(i).getEmpId() + " ");
System.out.print(empList.get(i).getName() + " ( " + empList.get(i).getDpt());
System.out.println(empList.get(i).getRank() + " ) ");
break;
} else if (empList.get(i).getEmpId() != num) {
if (i == empList.size() - 1) {
System.out.println("=====해당하는 사원이 존재하지 않습니다.=====");
}
}
}
}
public void searchVac() {
for (int i = 0; i < empList.size(); i++) {
if (empList.get(i).isVac()) {
System.out.println(empList.get(i).toString());
}
}
}
}
</style>
- error 수집가
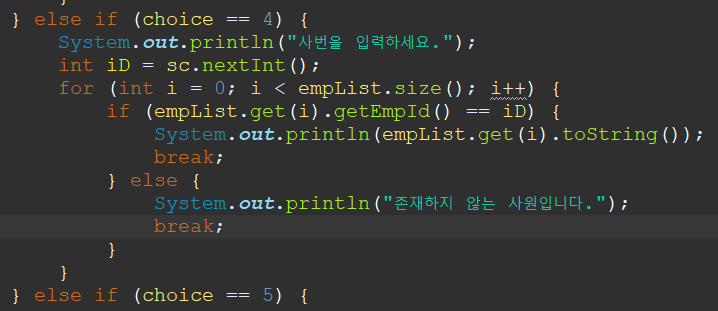
- 수정
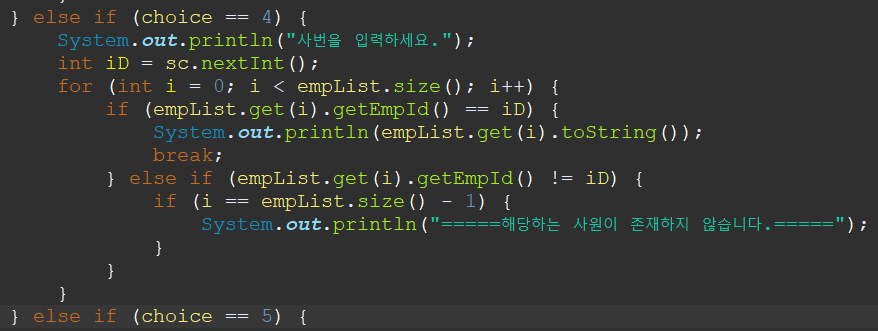
- 원인 error 첫 코드의 첫번째 for문 안의 if문에 조건이 맞지 않는다면 바로 else문으로 가서 break를 해버리기 때문에 1번 index를 제외한 2~나머지 사람들은 검색 안 되는 것이 당연한 것이었다.