Problem
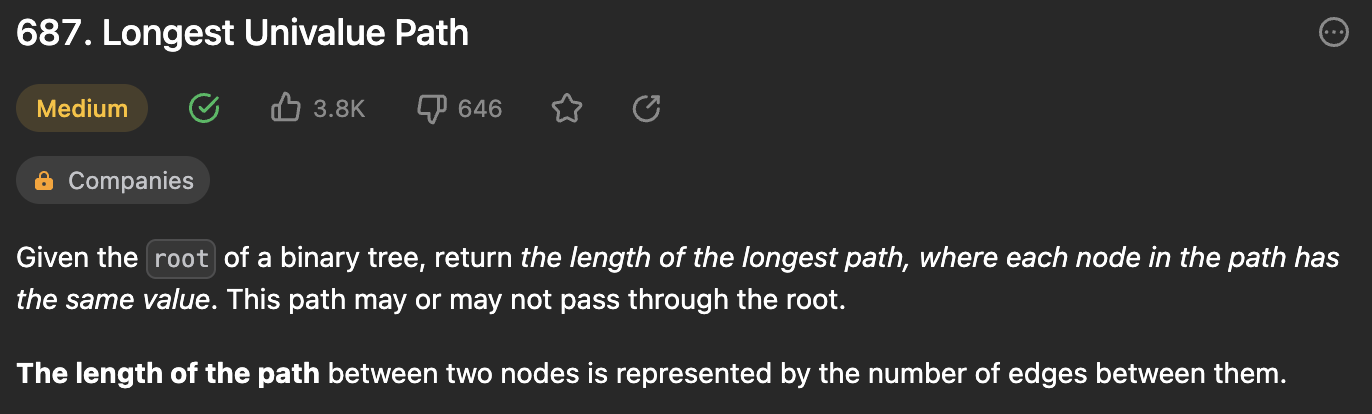
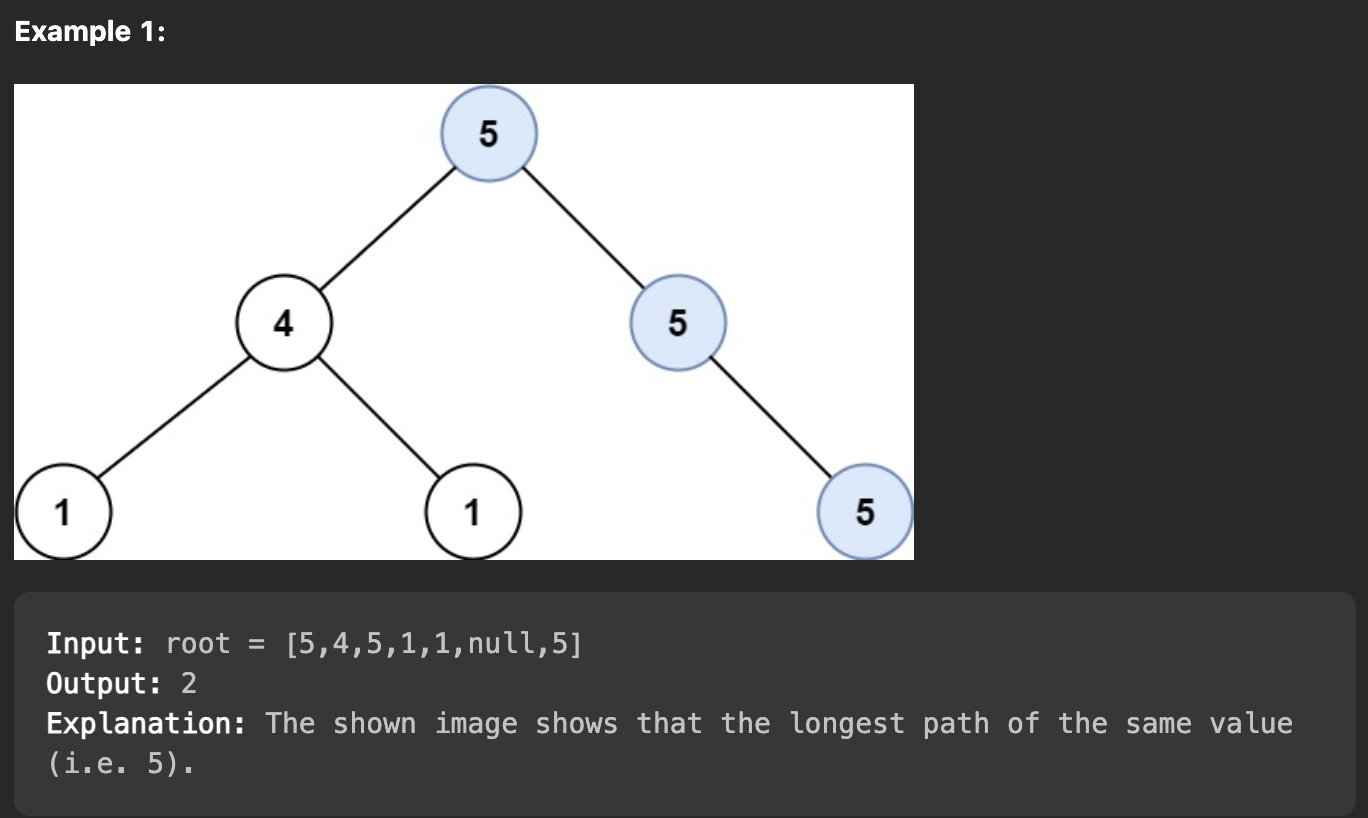
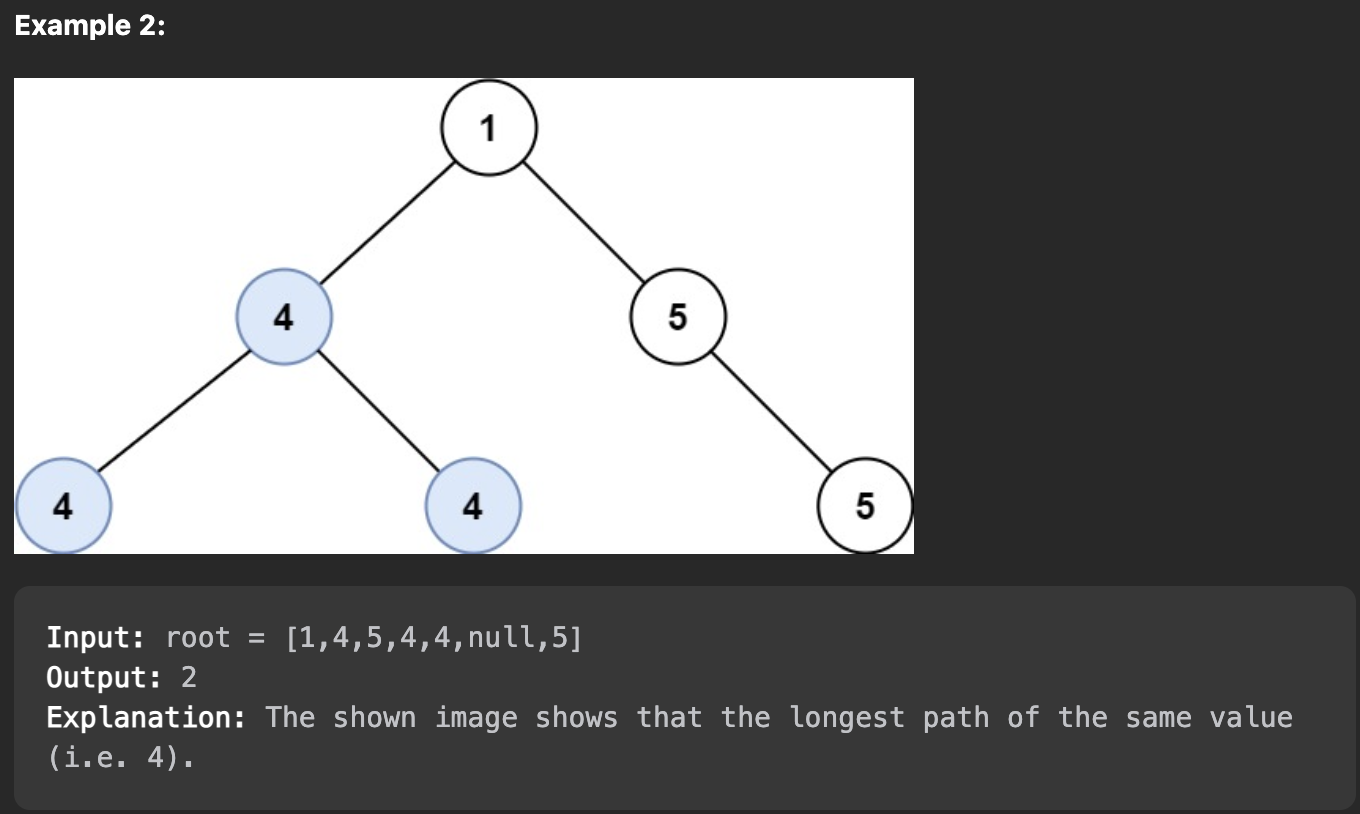
Solve
- 트리의 diameter 구하는 것처럼 재귀를 통해 자기 자신의 값과 자식이 있다면 각각 왼쪽 오른쪽 자식들의 값과 같은지 비교하여 각각의 diameter 를 증가시켜준다.
- 재귀에서의 반환값은 양쪽 diameter 이다.
- 전역변수인 path 는 각각 자식들의 값과 현재 값이 같을 경우 증가시킨 각각의 leftPath, rightPath 를 더한 값으로 갱신시킨다.
- 본 함수에서는 같을 경우의 path이기 때문에 path 를 반환시킨다.
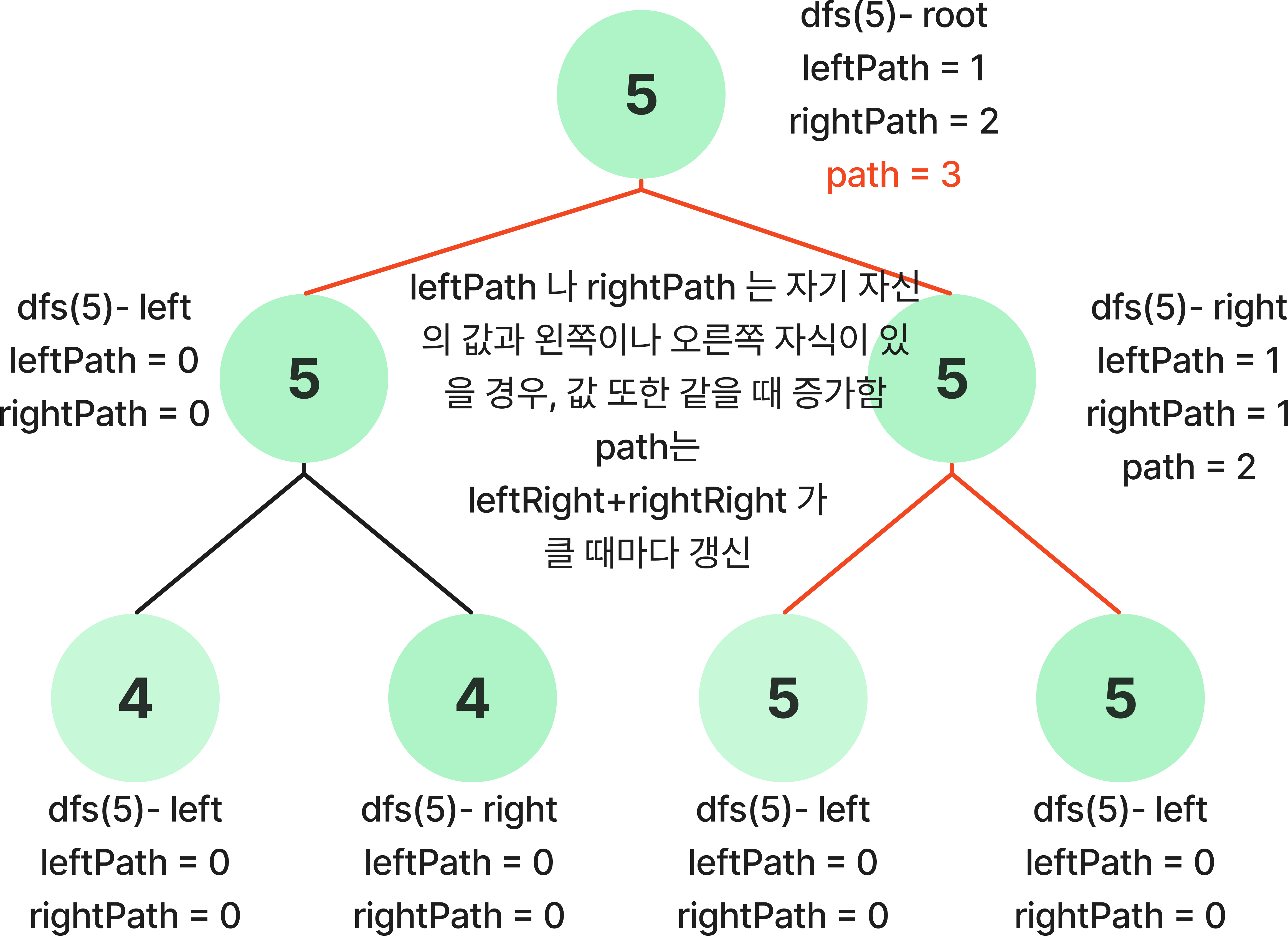
class Solution {
int path = 0;
public int dfs(TreeNode root){
if(root == null) return 0;
int left = dfs(root.left);
int right = dfs(root.right);
int leftPath = 0, rightPath = 0;
if(root.left != null && root.val == root.left.val){
leftPath += left+1;
}
if(root.right != null && root.val == root.right.val){
rightPath += right+1;
}
path = Math.max(path, leftPath + rightPath);
return Math.max(leftPath, rightPath);
}
public int longestUnivaluePath(TreeNode root) {
if(root == null) return 0;
dfs(root);
return path;
}
}
재귀는 항상 어렵다!