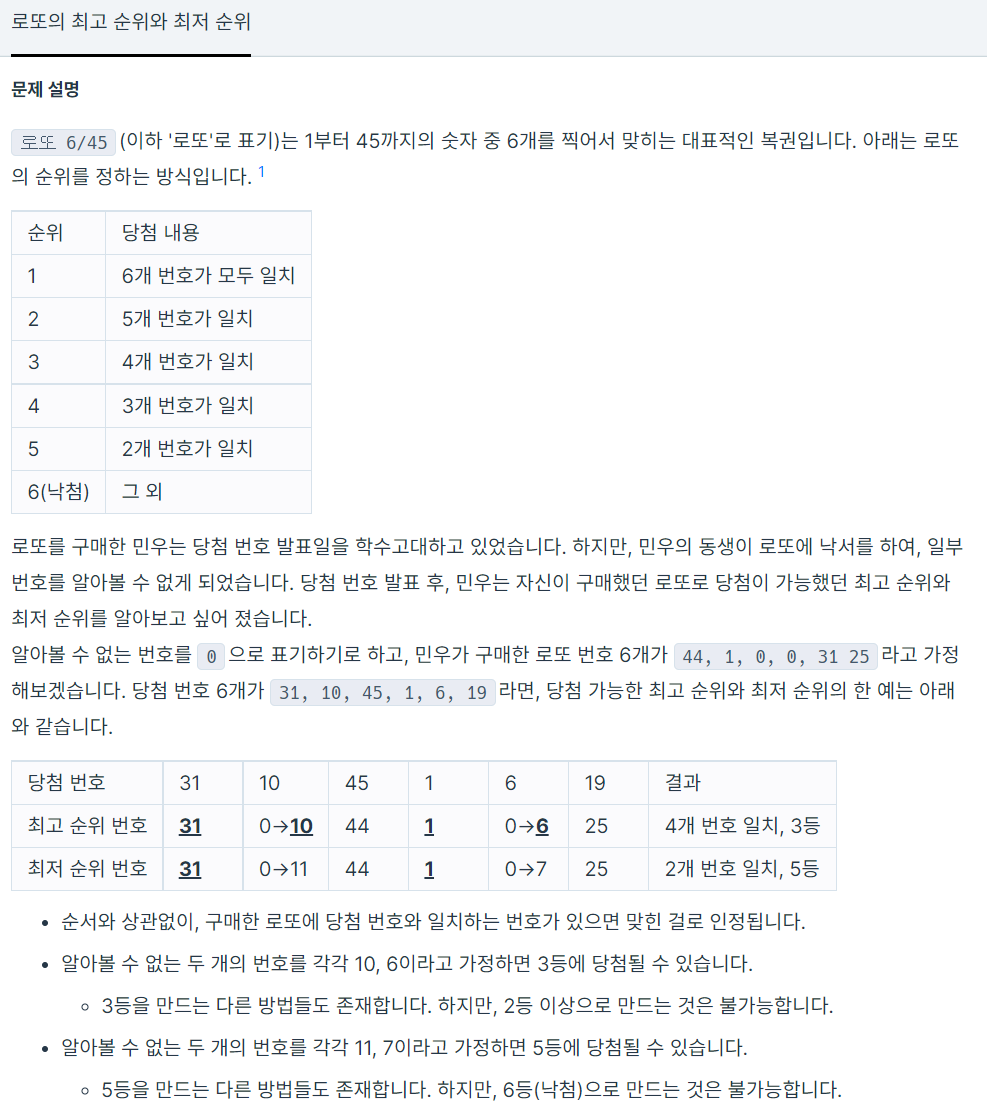
1. 접근
- 두 배열의 값이 같은지 확인하기 위해, 두 배열을 모두 오름차순 정렬한다.
- 단순하게 0이 아니면 6자리를 모두 돌아다니면서 값이 같은지 확인할 수 있다.
=> 더 큰 케이스의 경우를 위해, 반복문 실행을 최대한 줄이려면 내 로또번호가 당첨번호 자리보다 작아지면 그 숫자가 없는 것이므로 반복문을 탈출하도록 했다.
- 일치하는 번호의 개수(correct)와 0의 개수(zerocount)를 얻고나면, 그 값을 등수로 변환해준다.
=> 6개일치는 1등, 5개일치는 2등, ... , 1개 일치는 6등으로 등수+일치개수=7이다.
(여기서, 0개 일치도 6등인 점을 간과해서 오답이 나왔었다...)
- 따라서, 최대등수는 7에서 가장많이 맞은 개수를 빼준 값이고, 이 값은 1보다는 크거나 같아야 한다.
(동시에, 6보다 작거나 같아야 한다.)
- 등수 출력 과정을 단순하게 구현하려고 수식을 사용했는데 오히려 더 복잡해보이는 느낌이다.
2. 나의 풀이
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
vector<int> solution(vector<int> lottos, vector<int> win_nums) {
vector<int> answer;
sort(lottos.begin(),lottos.end());
sort(win_nums.begin(),win_nums.end());
int correct=0;
int zerocount=0;
for(int i=0,j=0;i<6;i++){
if(lottos[i]==0) {
zerocount++;
continue;
}
for(;j<6;j++){
if(lottos[i]==win_nums[j]) correct++;
else if(lottos[i]<win_nums[j]) break;
}
/* 단순한 반복문
for(int j=0;j<6;j++){
if(lottos[i]==win_nums[j]) correct++;
}
*/
}
answer.push_back(min(max(7-(correct+zerocount),1),6));
answer.push_back(min(7-(correct),6));
return answer;
}
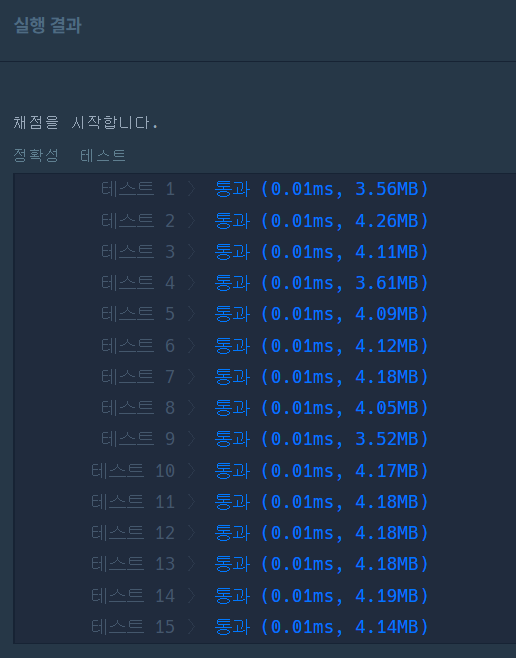