Node.js Introduction
Node.js의 특징
- Non-blocking
- Blocking I/O waits until the function in the program returns a result while Non-blocking I/O can execute other functions at the same time
- Single thread
- Uses one thread and can only execute another function at the same time
- Event loop
- A characteristic (logic) can that effectively execute functions to overcome the disadvantage of single thread
REPL
- Read, Evaluate, Print, Loop
- Read the inputted code and save it in memory
- Evaluate the value
- Print the value
- Loop until a certain signal is given
- Allows us to quickly confirm the value of a code and is appropriate for checking code syntax or output
Sync & Async (동기와 비동기)
- Sync: Waits until the running code outputs a result
- Async: Outputs a result regardless of the order of which the function executed
Blocking & Non-blocking Model
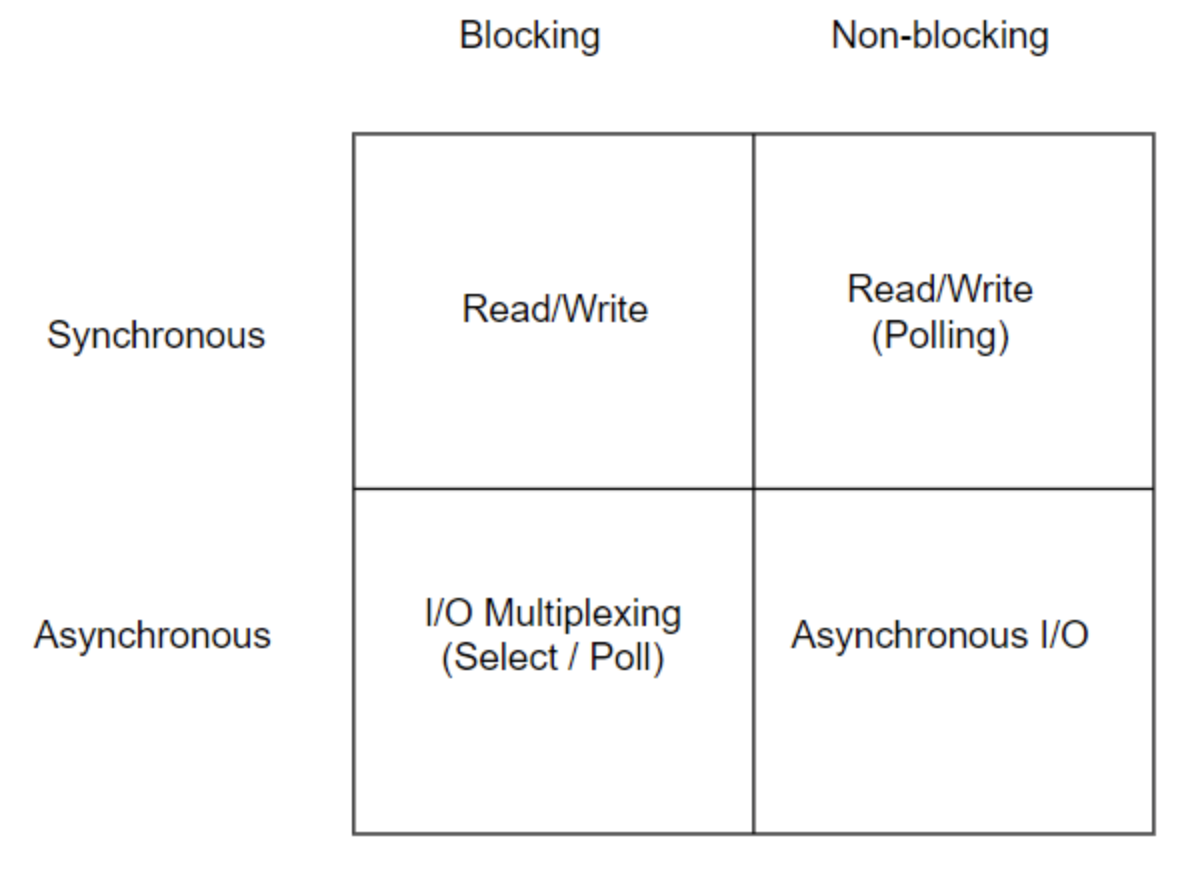
-
Blocking: Does not give control until the code is over and waits until it is over
-
Non-blocking: Gives control to another code so that the other code can execute even though the first code has not finished executing
-
Javascript uses Async + Non-blocking model to return the next code's result even though the current code has not finished executing
Promise
- Promise is an object type that helps execute in sync
- Composed of:
- executor: Only accepts functions. Automatically runs when the Promise is made. Must call either resolve or reject
- resolve: returned when the Promise is successfully run
- Runs
.then
when the function is resolved
- reject: returned when there is an error
- Runs
.catch
when the function is rejected
await
: Stops executing the function until the Promise is either fulfilled or rejected
new Promise(executor);
new Promise((resolve, reject) => {
});
- 3 types of Promise status:
- Pending (대기): Initial status, when the code hasn't run or hasn't been rejected
- Fulfilled (이행): The code has successfully run
- Rejected (거부): The code has failed
Object Literal
- Data types in Javascript can largely be categorized into primitive and object types
- Primitive type: only represents one value and cannot be changed
- Object type: represents various type values into one unit. Is a complex data structure. The value can be changed. Is made up of 0 or more properties and one property is made up of a key and value.
- Object Literal: A notational system to create an object
- Is a singleton, that means that when a change is made to an object made with object literal, it will change the object throughout the script
- As opposed to an object made with a constructor that will not change the object throughout the script if a change is made to the object
Error handling
- Use error handling to handle expected and unexpected errors
- There are usually more unexpected errors and a program must be ready to handle them
try/catch
Use try/catch to handle errors.
const users = ["Lee", "Kim", "Park", 2];
try {
for (const user of users) {
console.log(user.toUpperCase());
}
} catch (err) {
console.error(`Error: ${err.message}`);
}
throw
Throw an error on purpose when, for example, if an input is invalid.
If a user tries to withdraw more than the balance, throw an error:
function withdraw(amount, account) {
if (amount > account.balance)
throw new Error("잔고가 부족합니다.");
account.balance -= amount;
console.log(`현재 잔고가 ${account.balance}남았습니다.`);
}
const account = { balance: 1000 };
withdraw(2000, account);
finally
Executes regardless if an error has occurred or not