package chapter20230822.test10;
class Shape {
protected int x, y;
public void draw() {
System.out.println("Shape Draw");
}
}
class Rectangle extends Shape {
public int width, height;
@Override
public void draw() {
System.out.println("Rectangle Draw");
}
}
class Triangle extends Shape {
public int base, height;
@Override
public void draw() {
System.out.println("Triangle Draw");
}
}
class Circle extends Shape {
public int radius;
@Override
public void draw() {
System.out.println("Circle Draw");
}
}
public class test01 {
public static void main(String[] args) {
Shape shape = new Rectangle();
shape.draw();
shape.x = 0;
shape.y = 0;
}
}
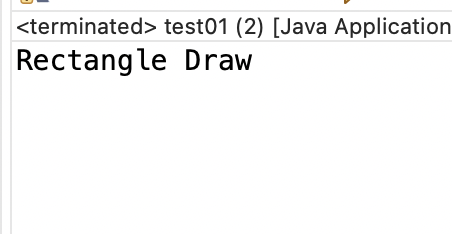
package chapter20230822.test10;
public class test02 {
public static void main(String[] args) {
Shape[] shapes = new Shape[3];
shapes[0] = new Rectangle();
shapes[1] = new Triangle();
shapes[2] = new Circle();
for(int i = 0; i < shapes.length; i++) {
shapes[i].draw();
}
}
}
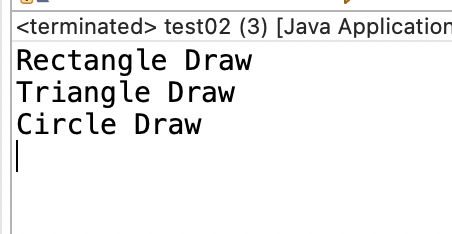
package chapter20230822.test10;
public class test03 {
public static void print(Shape shape) {
System.out.println("x=" + shape.x + " y=" + shape.y);
}
public static void main(String[] args) {
Rectangle shape1 = new Rectangle();
Triangle shape2 = new Triangle();
Circle shape3 = new Circle();
print(shape1);
print(shape2);
print(shape3);
}
}
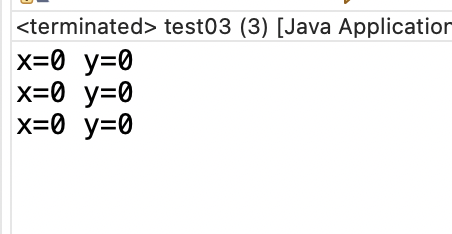