package chapter20230817.Banking;
import java.util.Scanner;
class Account {
private int accID;
private int balance;
private String cusName;
public Account(int accID, int balance, String cusName) {
this.accID = accID;
this.balance = balance;
this.cusName = cusName;
}
public boolean checkID(int id) {
return this.accID == id;
}
public boolean checkBalance(int money) {
return this.balance < money;
}
public void deposit(int money) {
this.balance += money;
}
public void withdraw(int money) {
this.balance -= money;
}
public void showAccInfo() {
System.out.println("계좌ID: " + this.accID);
System.out.println("이 름: " + this.cusName);
System.out.println("잔 액: " + this.balance);
}
}
public class BankingSystem {
static Scanner scanner = new Scanner(System.in);
static Account[] accArr = new Account[100];
static int accNum=0;
public static void main(String[] args) {
int choice;
while(true) {
ShowMenu();
System.out.print("선택: ");
choice = Integer.parseInt(scanner.nextLine());
switch(choice) {
case 1:
makeAccount();
break;
case 2:
depositMoney();
break;
case 3:
withdrawMoney();
break;
case 4:
showAllAccInfo();
break;
case 5:
return;
default:
System.out.println("Illegal selection..");
}
}
}
static void ShowMenu() {
System.out.println(" -----Menu------");
System.out.println("1. 계좌개설");
System.out.println("2. 입 금");
System.out.println("3. 출 금");
System.out.println("4. 계좌정보 전체 출력");
System.out.println("5. 프로그램 종료");
}
static void makeAccount() {
int id;
String name;
int balance;
System.out.println("[계좌개설]");
System.out.print("계좌ID: ");
id = Integer.parseInt(scanner.nextLine());
System.out.print("이 름: ");
name = scanner.nextLine();
System.out.print("입금액: ");
balance = Integer.parseInt(scanner.nextLine());
System.out.println();
accArr[accNum] = new Account(id, balance, name);
accNum++;
}
static void depositMoney() {
int money;
int id;
System.out.println("[입 금]");
System.out.print("계좌ID: ");
id = Integer.parseInt(scanner.nextLine());
System.out.print("입금액: ");
money = Integer.parseInt(scanner.nextLine());
for(int i = 0; i < accNum; i++) {
if(accArr[i].checkID(id)) {
accArr[i].deposit(money);
System.out.println("입금완료");
return;
}
}
System.out.println("유효하지 않은 ID 입니다.");
}
static void withdrawMoney() {
int money;
int id;
System.out.println("[출 금]");
System.out.print("계좌ID: ");
id = Integer.parseInt(scanner.nextLine());
System.out.println();
System.out.print("출금액: ");
money = Integer.parseInt(scanner.nextLine());
for(int i = 0; i < accNum; i++) {
if(accArr[i].checkID(id)) {
if(accArr[i].checkBalance(money)) {
System.out.println("잔액부족");
return;
}
accArr[i].withdraw(money);
System.out.println("출금완료");
return;
}
}
System.out.println("유효하지 않은 ID 입니다.");
}
static void showAllAccInfo() {
for(int i = 0; i < accNum; i++) {
accArr[i].showAccInfo();
System.out.println();
}
}
}
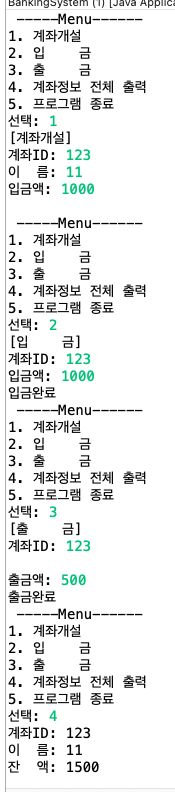