2022.03.24
스프링
@Autowired를 사용한 경우 bean이 주입되는 우선순위
- 인터페이스 구현이 우선순위
- type 기준 -> id체크
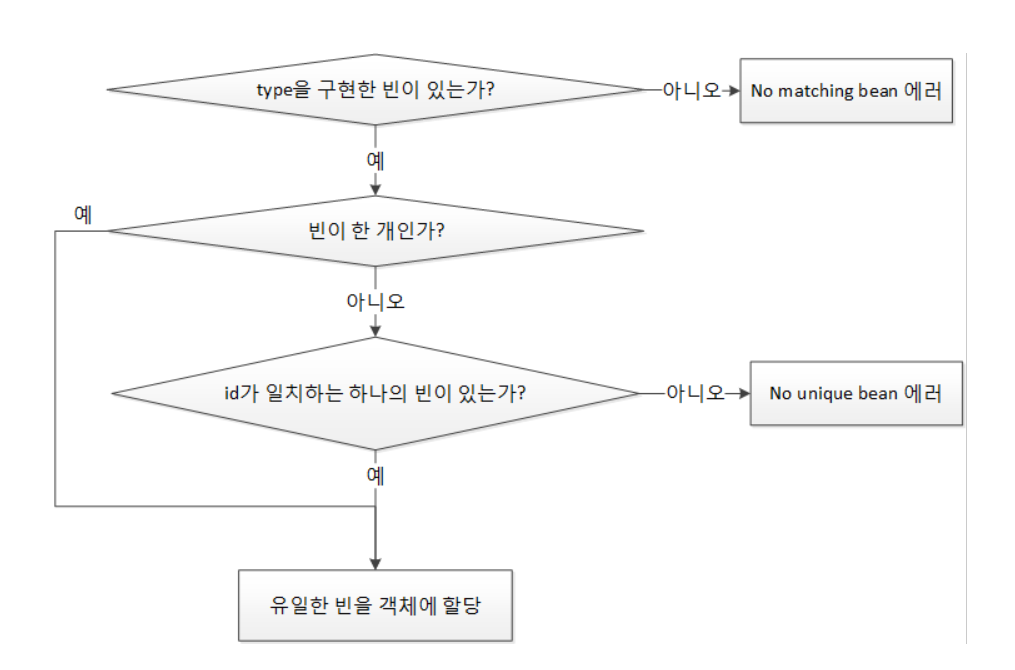
@Autowired
Tire tire
<bean class="exper004.KoreaTire"></bean>
<bean id="tire" class="expert004.Door"></bean>
스프링을 통한 의존성 주입 - @Resource를 통한 속성 주입
- @Autowired와 @Resource를 겉으로 보기에는 같은 기능을 하는 것 같다.
package com.heaven.mvc.expert005;
import javax.annotation.Resource;
public class Car {
@Resource
Tire tire;
public String getTireBrand() {
return "장착된 타이어: "+tire.getBrand();
}
}
- @Autowired vs @Resource
- @Autowired
- 스프링의 어노테이션
- type과 id중에 매칭 우선순위는 type
- @Resource
- 자바 표준 어노테이션
스프링을 사용하지 않아도 사용 할 수 있음
- type과 id중에 id가 우선순위가 높다.
| @Autowired | @Resource |
---|
출처 | 스프링 프레임워크 | 표준 자바 |
소속 패키지 | org.springframework.beans.factory.annotation.Autowired | javax.annotation.Resource |
빈 검색 방식 | byType 먼저, 못 찾으면 byName | byName 먼저, 못 찾으면 byType |
특이사항 | @Qualifier("") 협업 | name 어트리뷰트 |
byName 강제하기 | @Autowired @Qualifier("tire1") | @Resource(name="tire1") |
@Autowired vs @Resource vs < property>
package com.heaven.mvc.expert006;
import javax.annotation.Resource;
public class Car {
@Resource
Tire tire;
public String getTireBrand() {
return "장착된 타이어: "+tire.getBrand();
}
}
package com.heaven.mvc.expert006;
import javax.annotation.Resource;
public class Car {
@Autowired
Tire tire;
public String getTireBrand() {
return "장착된 타이어: "+tire.getBrand();
}
}
사례1. XML설정 - 한 개의 빈이 id없이 인터페이스를 구현한 경우
<?xml version="1.0" encoding="UTF-8"?>
<beans>
<context:annotation-config/>
<bean class="com.heaven.mvc.expert006.KoreaTire"></bean>
<bean id="car" class="com.heaven.mvc.expert006.Car"></bean>
</beans>
- @Autowired
타입이 일치하는게 딱 하나 있으므로 잘 동작
- @Resource
id가 일치하지는 않지만 타입이 일치하는게 있으므로 동작
사례2. XML설정 - 두 개의 빈이 id없이 인터페이스를 구현한 경우
<?xml version="1.0" encoding="UTF-8"?>
<beans>
<context:annotation-config/>
<bean class="com.heaven.mvc.expert006.KoreaTire"></bean>
<bean class="com.heaven.mvc.expert006.AmericaTire"></bean>
<bean id="car" class="com.heaven.mvc.expert006.Car"></bean>
</beans>
- @Autowired
타입 일치하는게 2개고 id가 없으므로 오류발생
- @Resource
id일치하는게 없고 타입이 일치하는게 2개이므로 오류발생
사례3. XML설정 - 두 개의 빈이 인터페이스를 구현하고 하나가 일치하는 id를 가진 경우
<?xml version="1.0" encoding="UTF-8"?>
<beans>
<context:annotation-config/>
<bean id="tire" class="com.heaven.mvc.expert006.KoreaTire"></bean>
<bean id="tire1" class="com.heaven.mvc.expert006.AmericaTire"></bean>
<bean id="car" class="com.heaven.mvc.expert006.Car"></bean>
</beans>
- @Autowired
타입 일치하는게 2개지만 id가 일치하는게 하나 있으므로 정상 동작
- @Resource
id일치하는게 하나 있으므로 정상 동작
사례4. XML설정 - 두 개의 빈이 인터페이스를 구현하고 일치하는 id가 없는 경우
<?xml version="1.0" encoding="UTF-8"?>
<beans>
<context:annotation-config/>
<bean id="tire1" class="com.heaven.mvc.expert006.KoreaTire"></bean>
<bean id="tire2" class="com.heaven.mvc.expert006.AmericaTire"></bean>
<bean id="car" class="com.heaven.mvc.expert006.Car"></bean>
</beans>
- @Autowired
타입 일치하는게 2개고 id도 일치하는게 없으므로 오류
- @Resource
id일치하는게 없고 타입이 일치하는게 2개이므로 오류발생
사례5. XML설정 - 일치하는 id가 하나 있지만 인터페이스를 구현하지 않은 경우
<?xml version="1.0" encoding="UTF-8"?>
<beans>
<context:annotation-config/>
<bean id="tire" class="com.heaven.mvc.expert006.Door"></bean>
<bean id="car" class="com.heaven.mvc.expert006.Car"></bean>
</beans>
- @Autowired
타입 일치하는게 없으므로 오류
- @Resource
id일치하는게 있지만 구현하지 않은 클래스이므로 오류
AOP - Aspect?관점?핵심 관심사?횡단 관심사?
- AOP
로직 주입
이라고 할 수 있다.
- 횡단 관심사
다수의 모듈에 공통적으로 나타는 부분
- 코드 = 핵심 관심사 + 횡단 관심사
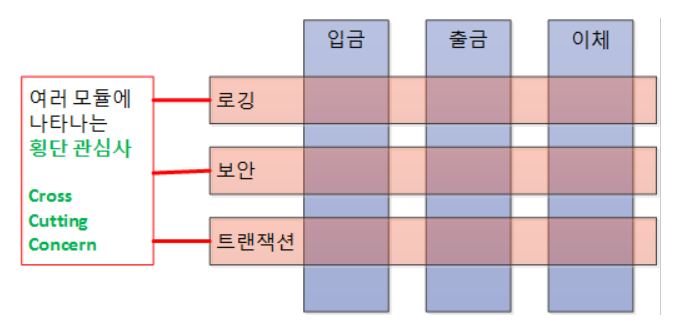
- 객체 지향에서 로직이 있는 곳은 당연히 메서드 안쪽이다. 따라서 메소드에서 코드를 주입할 수 있는 곳은
Around
,Before
,After
,AfterReturning
,AfterThrowing
총 5군데
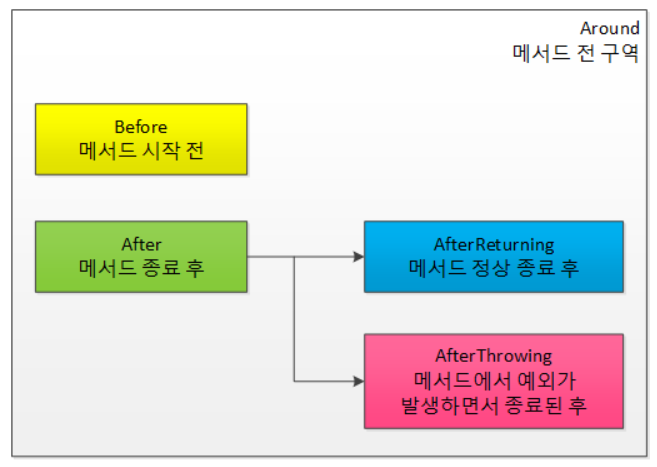
- 예제
package aop001;
public class Boy {
public void runSomething() {
System.out.println("열쇠로 문을 열고 집에 들어간다.");
try {
System.out.println("컴퓨터로 게임을 한다");
} catch (Exception e) {
if(e.getMessage().equals("집에 불남")) {
System.out.println("119에 신고한다.");
}
}finally {
System.out.println("소등하고 잔다.");
}
System.out.println("자물쇠를 잠그고 집을 나선다");
}
}
package aop001;
public class Girl {
public void runSomething() {
System.out.println("열쇠로 문을 열고 집에 들어간다.");
try {
System.out.println("요리를 한다");
} catch (Exception e) {
if(e.getMessage().equals("집에 불남")) {
System.out.println("119에 신고한다.");
}
}finally {
System.out.println("소등하고 잔다.");
}
System.out.println("자물쇠를 잠그고 집을 나선다");
}
}
package aop001;
public class Start {
public static void main(String[] args) {
Boy romeo = new Boy();
Girl juliet = new Girl();
romeo.runSomething();
juliet.runSomething();
}
}
AOP 실전편
package aop002;
public interface Person {
void runSomething();
}
package aop002;
public class Boy implements Person{
@Override
public void runSomething() {
System.out.println("컴퓨터로 게임을 한다");
}
}
package aop002;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Start {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("aop002/aop002.xml");
Person romeo = context.getBean("boy",Person.class);
romeo.runSomething();
}
}
package aop002;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
@Aspect
public class MyAspect {
@Before("execution(public void aop002.Boy.runSomething())")
public void before(JoinPoint joinpoint) {
System.out.println("얼굴 인식 확인 : 문을 개방하라");
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.1.xsd">
<aop:aspectj-autoproxy/>
<bean id="myAspect" class="aop002.MyAspect"/>
<bean id="boy" class="aop002.Boy"/>
</beans>