💡 서버/클라이언트 소켓 연결 후 Stream 으로 데이터 보내기
✔️ 결과 화면
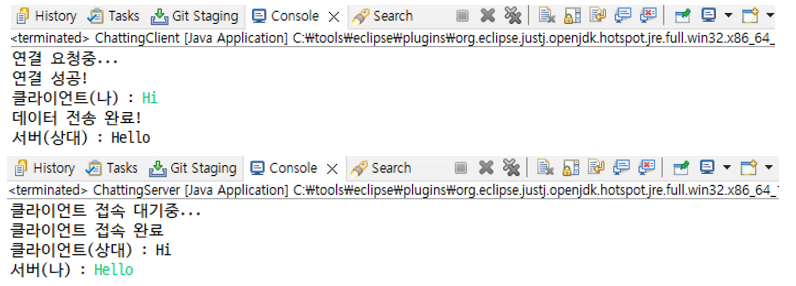
✔️ 서버 소켓(Server Socket) 소스코드
package com.kh.day16.network.exam3;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.Scanner;
public class ChattingServer {
public static void main(String[] args) {
ServerSocket serverSorket = null;
InputStream is = null;
OutputStream os = null;
Scanner sc = new Scanner(System.in);
try {
serverSorket = new ServerSocket(7777);
System.out.println("클라이언트 접속 대기중...");
Socket socket = serverSorket.accept();
System.out.println("클라이언트 접속 완료");
is = socket.getInputStream();
byte [] bytes = new byte[1024];
int readByteNo = is.read(bytes);
String message = new String(bytes, 0, readByteNo);
System.out.printf("클라이언트(상대) : %s\n", message);
os = socket.getOutputStream();
System.out.print("서버(나) : ");
message = sc.nextLine();
bytes = message.getBytes();
os.write(bytes);
os.flush();
System.out.println("데이터 전송 성공!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
✔️ 클라이언트 소켓(Client Socket) 소스코드
package com.kh.day16.network.exam3;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.Scanner;
public class ChattingClient {
public static void main(String[] args) {
String address = "127.0.0.1";
int port = 7777;
OutputStream os = null;
InputStream is = null;
Scanner sc = new Scanner(System.in);
try {
System.out.println("연결 요청중...");
Socket socket = new Socket(address, port);
System.out.println("연결 성공!");
os = socket.getOutputStream();
System.out.print("클라이언트(나) : ");
String message = sc.nextLine();
byte [] bytes = message.getBytes();
os.write(bytes);
os.flush();
System.out.println("데이터 전송 완료!");
is = socket.getInputStream();
bytes = new byte[1024];
int readByteNo = is.read(bytes);
message = new String(bytes, 0, readByteNo);
System.out.printf("서버(상대) : %s\n", message);
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
💡 1:1 채팅 프로그램 만들기
✔️ 결과 화면
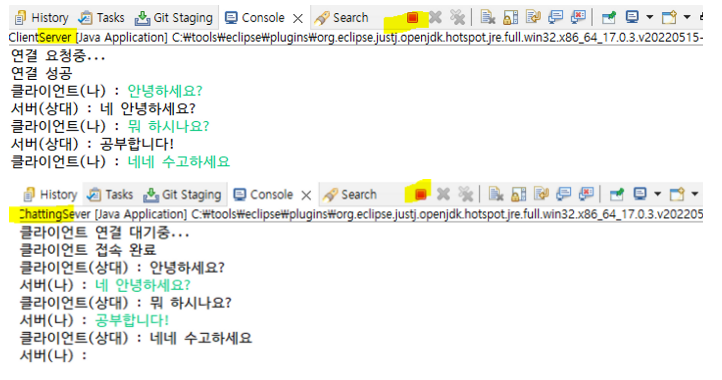
✔️ 서버 소켓(Server Socket) 소스코드
package com.kh.day16.network.exercise1;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.Scanner;
public class ChattingSever {
public static void main(String[] args) {
ServerSocket serverSocket = null;
OutputStream os = null;
InputStream is = null;
Scanner sc = new Scanner(System.in);
try {
serverSocket = new ServerSocket(7777);
System.out.println("클라이언트 연결 대기중...");
Socket socket = serverSocket.accept();
System.out.println("클라이언트 접속 완료");
is = socket.getInputStream();
os = socket.getOutputStream();
while(true) {
byte [] bytes = new byte[1024];
int readByteNo = is.read(bytes);
String message = new String(bytes, 0, readByteNo);
System.out.printf("클라이언트(상대) : %s\n", message);
System.out.print("서버(나) : ");
message = sc.nextLine();
bytes = message.getBytes();
os.write(bytes);
os.flush();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
✔️ 클라이언트 소켓(Client Socket) 소스코드
package com.kh.day16.network.exercise1;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.Scanner;
public class ClientServer {
public static void main(String[] args) {
String address = "192.168.60.217";
int port = 6312;
OutputStream os = null;
InputStream is = null;
Scanner sc = new Scanner(System.in);
try {
System.out.println("연결 요청중...");
Socket socket = new Socket(address, port);
System.out.println("연결 성공");
os = socket.getOutputStream();
is = socket.getInputStream();
while(true) {
System.out.print("클라이언트(나) : ");
String message = sc.nextLine();
byte [] bytes = message.getBytes();
os.write(bytes);
os.flush();
bytes = new byte[1024];
int readByteNo = is.read(bytes);
message = new String(bytes, 0, readByteNo);
System.out.printf("서버(상대) : %s\n", message);
}
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
정말 좋은 정보 감사합니다!