<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>08calc</title>
<script>
function validate() {
let op=document.getElementById("op").value;
op=op.trim();
if(!(op=='+' || op=='-' || op=='/'||op=='*'||op=='%')){
alert("연산기호는 + - / * % 만 입력 가능!");
return false;
}
return true;
}
</script>
</head>
<body>
<h3>계산기 연습</h3>
<form name="calcfrm" id="calcfrm" method="get" action="08_ok.jsp" onsubmit="return validate()">
첫번째 수 : <input type="number" name="num1" id="num1" min="0" max="100" required>
<hr>
연산자: <input type="text" name="op" id="op" size="3" maxlenght="1" required>
<hr>
두번째 수:<input type="number" name="num2" id="num2" min="0" max="100" required>
<hr>
<input type="submit" value="계산">
<input type="reset" value="취소">
</form>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<meta charset="UTF-8">
<title>08_ok.jsp</title>
</head>
<body>
<h3>계산 결과</h3>
<%
//한글 인코딩
request.setCharacterEncoding("utf-8");
int num1=Integer.parseInt(request.getParameter("num1").trim());
int num2=Integer.parseInt(request.getParameter("num2").trim());
String op=request.getParameter("op").trim();
int res1=0; //정수형 계산 결과
double res2=0.0;
if(op.equals("+")){
res1=num1+num2;
}else if(op.equals("-")){
res1=num1-num2;
}else if(op.equals("/")){
res2=(double)num1/num2;
}else if(op.equals("*")){
res1=num1*num2;
}else if(op.equals("%")){
res1=num1%num2;
}
%>
<table class="table table-striped">
<tr>
<td><%=num1%></td>
<td><%=op%></td>
<td><%=num2%></td>
<td>=</td>
<td>
<%
if(op.equals("/")){
out.print(String.format("%.1f", res2));
}else{
out.print(res1);
}
%>
</td>
</tr>
</table>
</body>
</html>
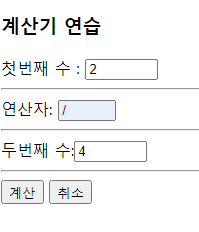
