라우터 - 전체 애플리케이션의 성능 및 속도를 좌우함, 캐시에 저장됨
① component: HomeView
- 무조건적으로 실행해야 하는 첫 페이지
- 서버에 접근하는 순간 서버로 부터 app.js를 받아옴
② component: () => import(/ webpackChunkName: "about" /
- About 메뉴를 클릭하면 서버에 접근해서 about.js를 받아온 후, 브라우저 캐시에 저장함
- 해당 페이지에 접속 빈도가 적은 경우
- 파일 크기가 작아서 서버로 부터 받아오는데 무리가 없는 경우
③ component: () => import(/ webpackChunkName: "databinding", webpackPrefetch:true /
- 메뉴를 클릭하는 순간 서버에서 받아올 파일의 크기가 큰 경우, 미리 받아오면 유리한 경우
- 화면 열리자 마자 들어갈 빈도가 높은 경우
- databinding.js에서 관리를 함. 연관성이 있도록 그룹핑이 가능함
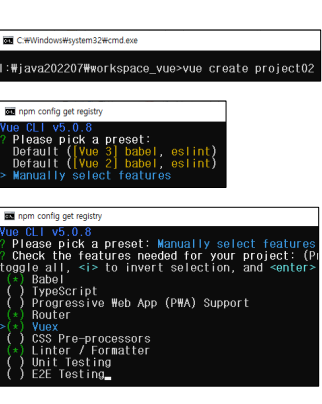
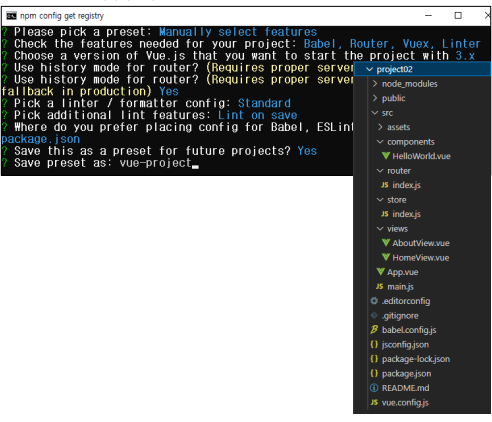
APPVUE
<template>
<nav>
<router-link to="/">Home</router-link> |
<router-link to="/about">About</router-link> |
<router-link to="/Welcome">Welcome</router-link> |
<router-link to="/databinding/String">String</router-link> |
<router-link to="/databinding/HTML">HTML</router-link> |
<router-link to="/databinding/Input">Input</router-link> |
<router-link to="/databinding/Select">Select</router-link> |
<router-link to="/databinding/CheckBox">CheckBox</router-link> |
<router-link to="/databinding/Radio">Radio</router-link> |
<router-link to="/databinding/Attri">Attri</router-link> |
<router-link to="/databinding/List">List</router-link> |
</nav>
<router-view/>
</template>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
}
nav {
padding: 30px;
}
nav a {
font-weight: bold;
color: #2c3e50;
}
nav a.router-link-exact-active {
color: #42b983;
}
</style>
1.데이터 바인딩
<template>
<div>
<h1> Hello {{ userName }}</h1>
<p>{{ message }}</p>
</div>
</template>
<script>
export default {
data() {
return {
userName: 'John Doe',
message: '존 도우'
}
}
}
</script>
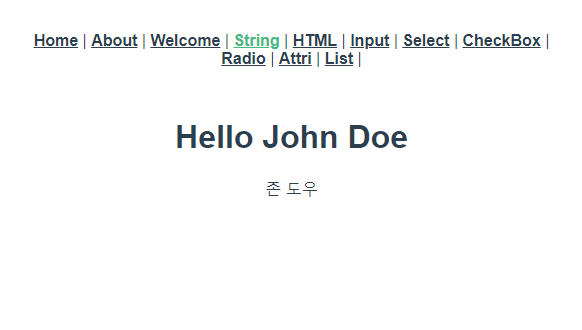
2.HTML 바인딩
<template>
<div>
<div>{{ htmlString }}</div>
<div v-html="htmlString"></div>
</div>
</template>
<script>
export default {
data() {
return {
htmlString: '<p style="color:red">빨간색 문자</p>'
}
}
}
</script>
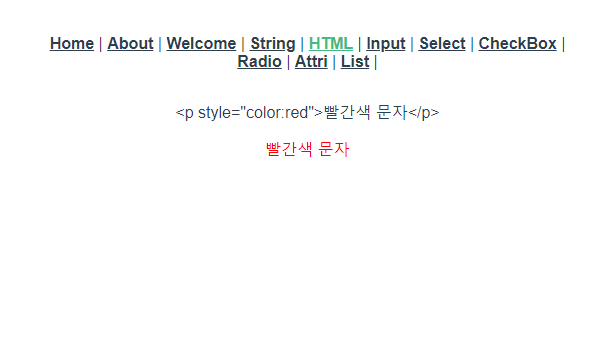
<template>
<div>
<h3>Input 바인딩 -양방향</h3>
<input type="text" v-model="userId">
<h3>이벤트</h3>
<button @click="myfunction">click</button>
<button @click="changeData">바꾸기</button>
<h3>숫자 바인딩</h3>
<input type="text" v-model="num1">
<input type="text" v-model="num2">
<span>{{ num1+num2 }}</span>
<hr>
<input type="text" v-model="num3">
<input type="text" v-model="num4">
<span>{{ num3+num4 }}</span>
</div>
</template>
<script>
export default {
data() {
return {
userId: 'itwill',
num1: 0,
num2: 0,
num3: 0,
num4: 0
}
},
methods: {
myfunction() {
alert(this.userId)
},
changeData() {
this.userId = 'KR'
}
}
}
</script>
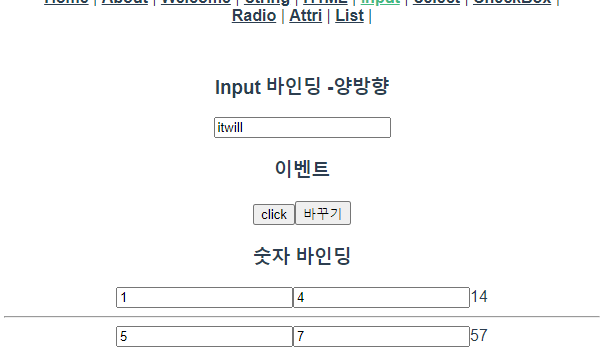
4.Select 바인딩
<template>
<div>
<h3>select 바인딩</h3>
<select name="" id="" v-model="selectedCity">
<option value=""></option>
<option value="02">서울</option>
<option value="054">제주</option>
<option value="064">부산</option>
</select>
</div>
</template>
<script>
export default {
data() {
return {
selectedCity: '02'
}
}
}
</script>
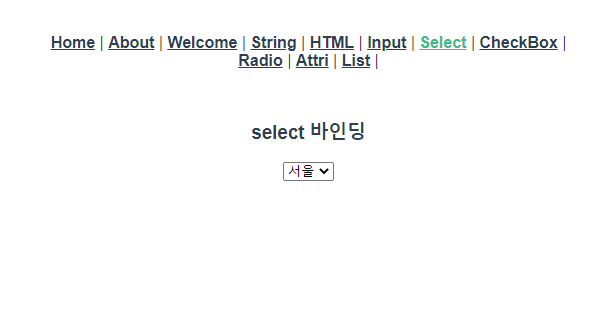
5.CheckBox 바인딩
<template>
<div>
<h1> CheckBox 바인딩</h1>
<div>
<input type="checkbox" id="html" value="html" v-model="favlang">
<label for="html">HTML</label>
</div>
<div>
<input type="checkbox" id="css" value="css" v-model="favlang">
<label for="css">CSS</label>
</div>
<div>
<input type="checkbox" id="js" value="js" v-model="favlang">
<label for="js">JS</label>
</div>
<div>
선택한 과목 : {{ favlang }}
</div>
</div>
</template>
<script>
export default {
data() {
return {
favlang: ['js']
}
}
}
</script>
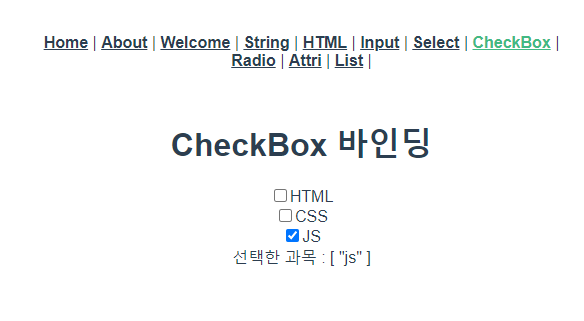
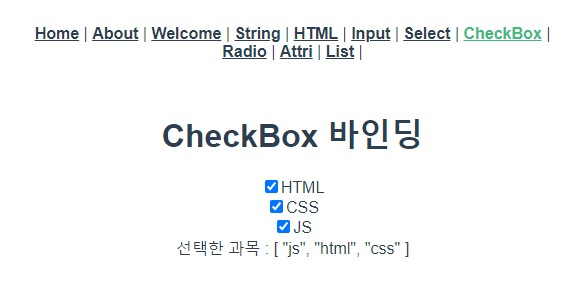
6.RADIO 바인딩
<template>
<div>
<h1> Radio 바인딩</h1>
<div>
<input type="radio" id="html" value="html" v-model="favlang">
<label for="html">HTML</label>
</div>
<div>
<input type="radio" id="css" value="css" v-model="favlang">
<label for="css">CSS</label>
</div>
<div>
<input type="radio" id="js" value="js" v-model="favlang">
<label for="js">JS</label>
</div>
<div>
선택한 과목 : {{ favlang }}
</div>
</div>
</template>
<script>
export default {
data() {
return {
favlang: ' '
}
}
}
</script>
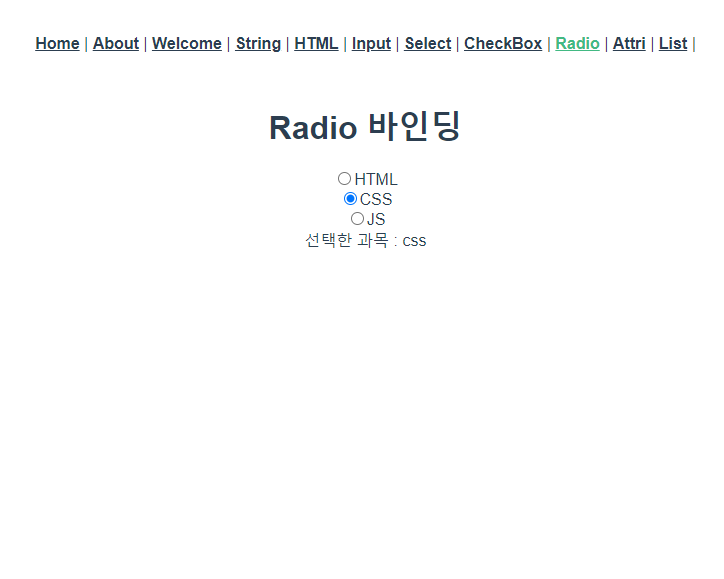
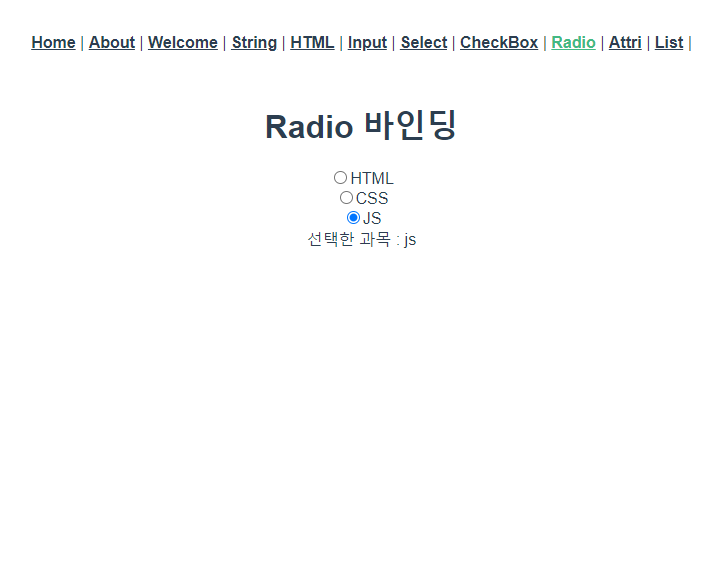
7.다양한 속성 바인딩
<template>
<div>
<h1>다양한 속성 바인딩</h1>
<hr>
<h3>readonly 속성</h3>
<input type="text" name="" id="" readonly v-model="userid">
<input type="text" name="" id="" v-bind:value="userid" readonly>
<input type="text" name="" id="" :value="userid" readonly>
<hr>
<h3>src 속성</h3>
<img :src="imgSrc" alt="" style="width: 100px; height: auto;">
<hr>
<h3>disable 속성-검색어를 입력하지 않으면 조회버튼 비활성화</h3>
<input type="search" name="" id="" v-model="txt1">
<button :disabled="txt1 === ''">조회</button>
</div>
</template>
<script>
export default {
data() {
return {
userid: 'korea',
imgSrc: 'https://www.itwill.co.kr/css/wvtex/img/wvUser/logo.png',
txt1: ''
}
}
}
</script>
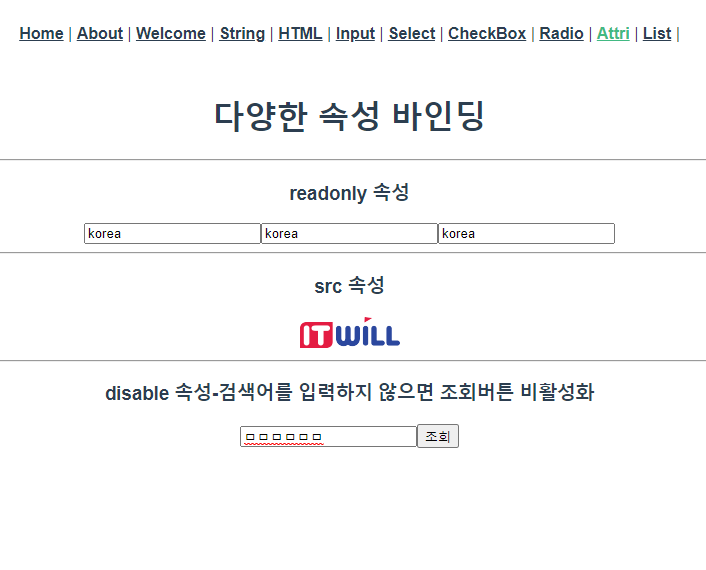
8.배열 데이터 바인딩
<template>
<div>
<h1> 배열 데이터 바인딩</h1>
<hr>
<h3>목록</h3>
<div>
<select name="" id="">
<option value=""></option>
<option v-for="city in cities" :value="city.code" :key="city.code">{{ city.title }}</option>
</select>
</div>
<div>
<h3>표 작성 후 목록 출력</h3>
<table border="3">
<thead>
<tr>
<th>제품번호</th>
<th>제품명</th>
<th>가격</th>
<th>주문수량</th>
<th>합계</th>
</tr>
</thead>
<tbody>
<tr v-for="drink in drinkList" :key="drink.drinkId">
<td>{{ drink.drinkId }}</td>
<td>{{ drink.drinkName }}</td>
<td>{{ drink.price}}</td>
<td><input type="number" name="" id="" v-model="drink.qty"></td>
<td>{{ drink.qty*drink.price }}</td>
</tr>
</tbody>
</table>
</div>
</div>
</template>
<script>
export default {
data() {
return {
cities: [
{ title: '서울', code: '02' },
{ title: '부산', code: '051' },
{ title: '제주', code: '064' }
],
drinkList: [
{ drinkId: '1', drinkName: '코카콜라', price: 700, qty: 1 },
{ drinkId: '2', drinkName: '오렌지주스', price: 1200, qty: 1 },
{ drinkId: '3', drinkName: '커피', price: 500, qty: 1 },
{ drinkId: '4', drinkName: '물', price: 700, qty: 1 },
{ drinkId: '5', drinkName: '보리차', price: 1200, qty: 1 },
{ drinkId: '6', drinkName: '포카리', price: 1000, qty: 1 },
{ drinkId: '7', drinkName: '뽀로로', price: 1300, qty: 1 }
]
}
}
}
</script>
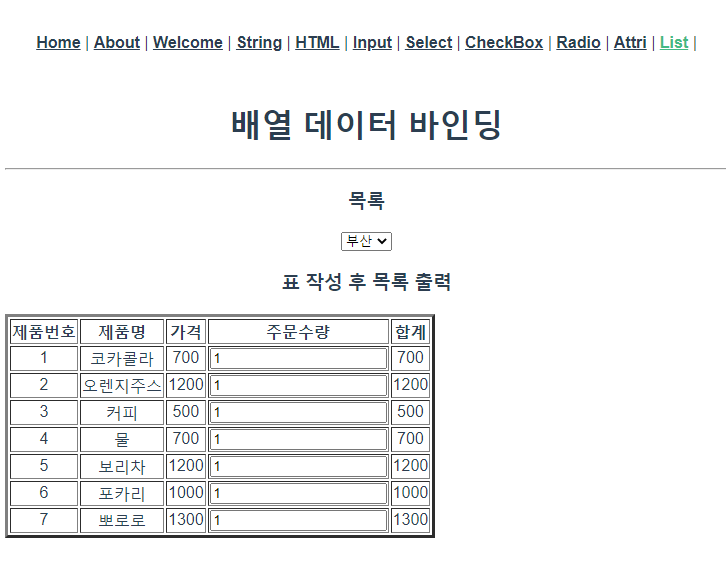
9.class 바인딩
<template>
<div>
<h1> Class 바인딩</h1>
<div style="color:red">클래스 바인딩1</div>
<div class="text-red">클래스 바인딩2</div>
<div :class="{'text-red': true}">클래스 바인딩3</div>
<div :class="{'text-red': false}">클래스 바인딩4</div>
<div :class="{'text-red': true, active:true}">클래스 바인딩5</div>
<div :class="{'text-red': false, 'active':true}">클래스 바인딩6</div>
<div :class="{'text-red': hasError, 'active':isActive}">클래스 바인딩7</div>
<div :class="class2">클래스 바인딩8</div>
</div>
</template>
<script>
export default {
data() {
return {
hasError: false,
isActive: true,
class2: ['active', 'text-red']
}
}
}
</script>
<style scoped>
.text-red{ color: red;}
.active {background-color: greenyellow; font-weight: bold;}
</style>
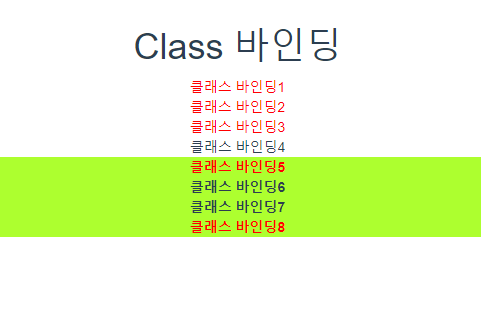
10.Style 속성 바인딩
<template>
<div>
<h1> Style 속성 바인딩</h1>
<div style="color:red; font-size: 20px">스타일 바인딩:글씨 red,폰트크기:20px</div>
<div :style="style1"> 스타일바인딩: 글씨 green, 폰트크기:30px</div>
<button @click="style1.color='blue'">색상바꾸기</button>
</div>
</template>
<script>
export default {
data() {
return {
style1: { color: 'green', fontSize: '30px' }
}
}
}
</script>
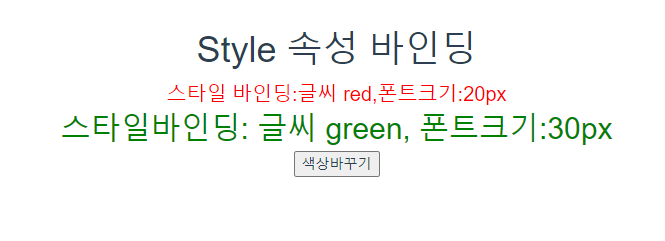
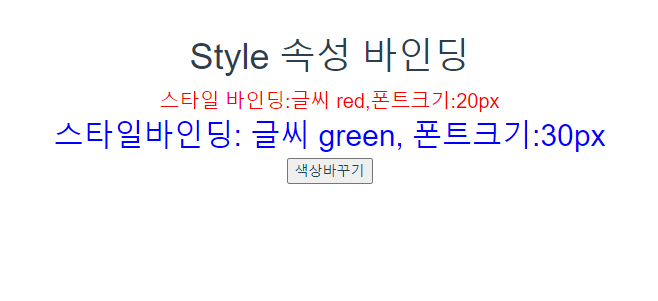