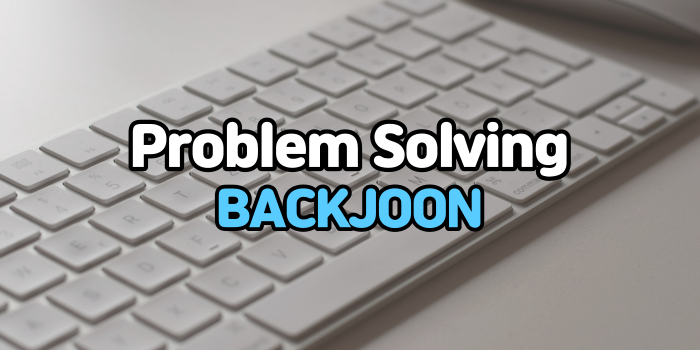
문제
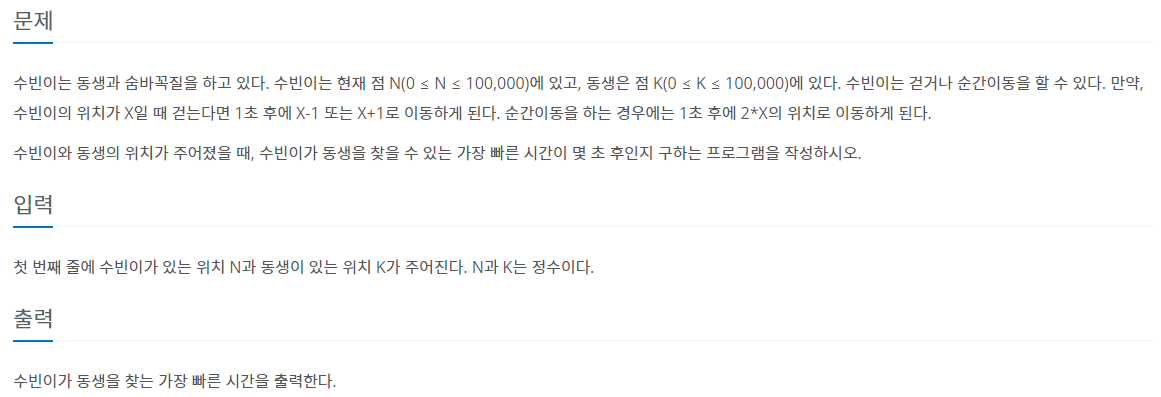
풀이
- 전형적인 BFS 문제이다.
- 한 위치에서 3가지 경우가 나올 수 있고 이 경우 중 100000이 넘어가는 위치는 빼고, 이미 그 위치를 방문했다면 빼고 큐에 push한다. 그 이유는 위치를 방문한 경우 더 빠른 경로가 계산되고 있다는 의미이기 때문이다.
- 런타임에러(OutofBounds)가 계속 발생했는 그 이유는 visited 배열이 100001로 큰 크기를 가지고 있는데 이 때 전역변수로 선언해야 힙 영역에 생성되어 큰 크기를 할당할 수 있고 main문 안에 지역변수로 선언하면 스택에 생성되어 크기가 제한이 걸릴 수 있기 때문이다.
코드
#include <iostream>
#include <queue>
using namespace std;
int visited[100001] = { 0 };
int main() {
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
queue<pair<int, int>> q;
int N, K;
cin >> N >> K;
visited[N] = 1;
q.push(make_pair(N, 0));
bool stop = false;
int result;
while (q.size() != 0 && stop == false) {
int node = q.front().first;
int depth = q.front().second;
q.pop();
if (node == K) {
result = depth;
break;
}
int a[3] = { node - 1, node + 1, node * 2 };
for (int i = 0; i < 3; i++) {
if (a[i] <= 100000) {
if (a[i] == K) {
stop = true;
result = depth + 1;
break;
}
else if (visited[a[i]] == 0) {
q.push(make_pair(a[i], depth + 1));
visited[a[i]] = 1;
}
}
}
}
cout << result << '\n';
return 0;
}