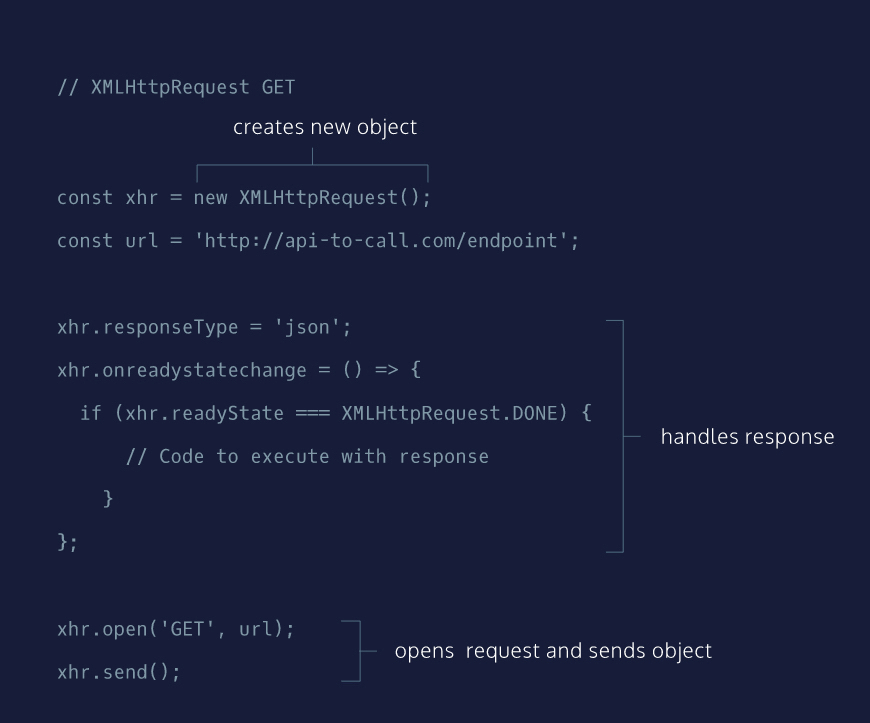
GET
const xhr = new XMLHttpRequest();
const url = 'https://api-to-call.com/endpoint';
xhr.responseType = 'json';
xhr.onreadystatechange = () => {
if (xhr.readyState === XMLHttpRequest.DONE) {
return xhr.response
}
}
xhr.open('GET', url);
xhr.send()
POST
const xhr = new XMLHttpRequest();
const url = 'https://api-to-call.com/endpoint';
const data = JSON.stringify({id:'200'})
xhr.responseType = 'json';
xhr.onreadystatechange = () => {
if (xhr.readyState === XMLHttpRequest.DONE) {
return xhr.response;
}
}
xhr.open('POST', url);
xhr.send(data)
const shortenUrl = () => {
const urlToShorten = inputField.value
const data = JSON.stringify({destination: urlToShorten});
const xhr = new XMLHttpRequest();
xhr.responseType = 'json';
xhr.onreadystatechange = () => {
if (xhr.readyState === XMLHttpRequest.DONE) {
renderResponse(xhr.response)
}
}
xhr.open('POST', url);
xhr.setRequestHeader('Content-type', 'application/json');
xhr.setRequestHeader('apikey', apiKey);
xhr.send(data);
}
FETCH
GET
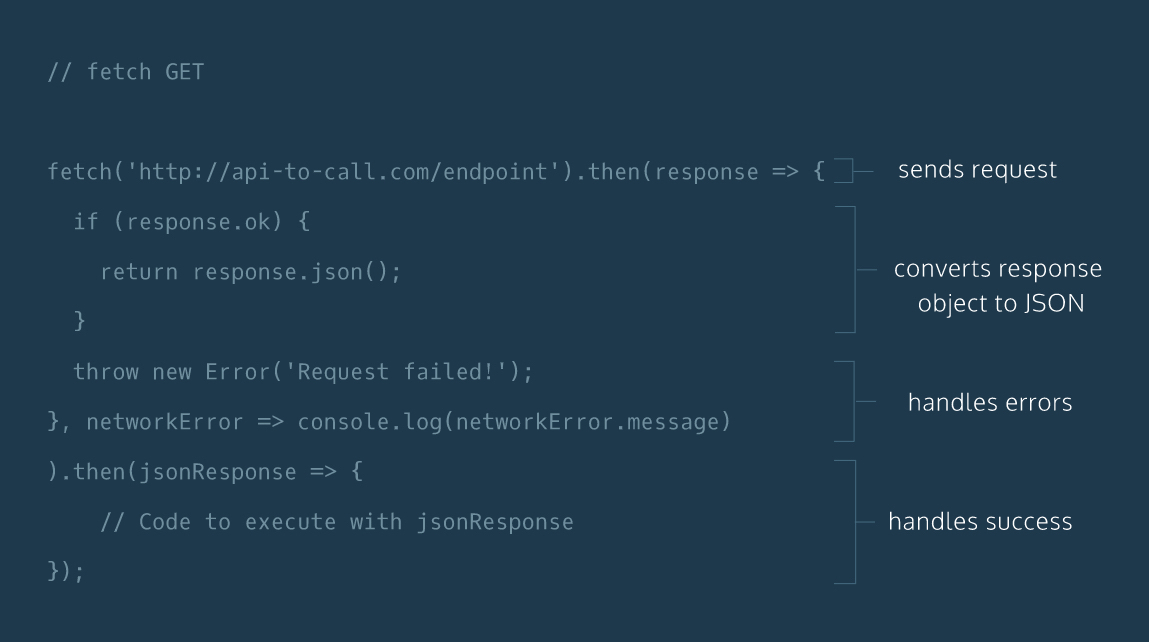
fetch('https://api-to-call.com/endpoint').then((response) => {
if (response.ok) {
return response.json();
}
throw new Error('Request failed!');
}, networkError => {
console.log(networkError.message)
}).then(jsonResponse => jsonResponse)
POST
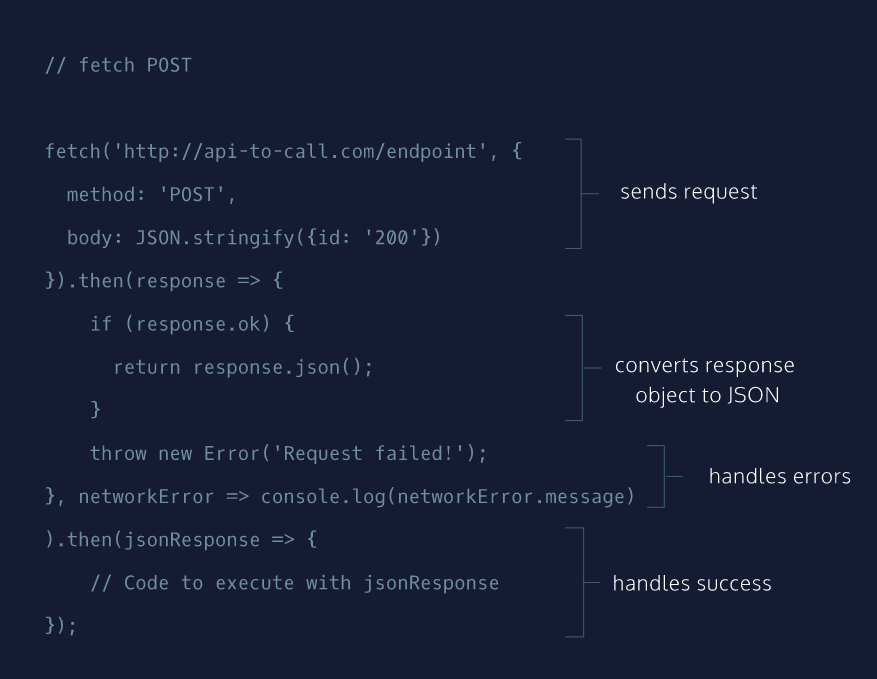
const shortenUrl = () => {
const urlToShorten = inputField.value;
const data = JSON.stringify({destination:urlToShorten});
fetch(url,{
method: 'POST',
headers : {
'Content-type': 'application/json',
'apikey':apiKey
},
body: data
}).then(response => {
if (response.ok) {
return response.json();
}
throw new Error('Request failed!');
}, networkError => {
console.log(networkError.message)
}).then(jsonResponse => {
renderResponse(jsonResponse)
})
}
async, await
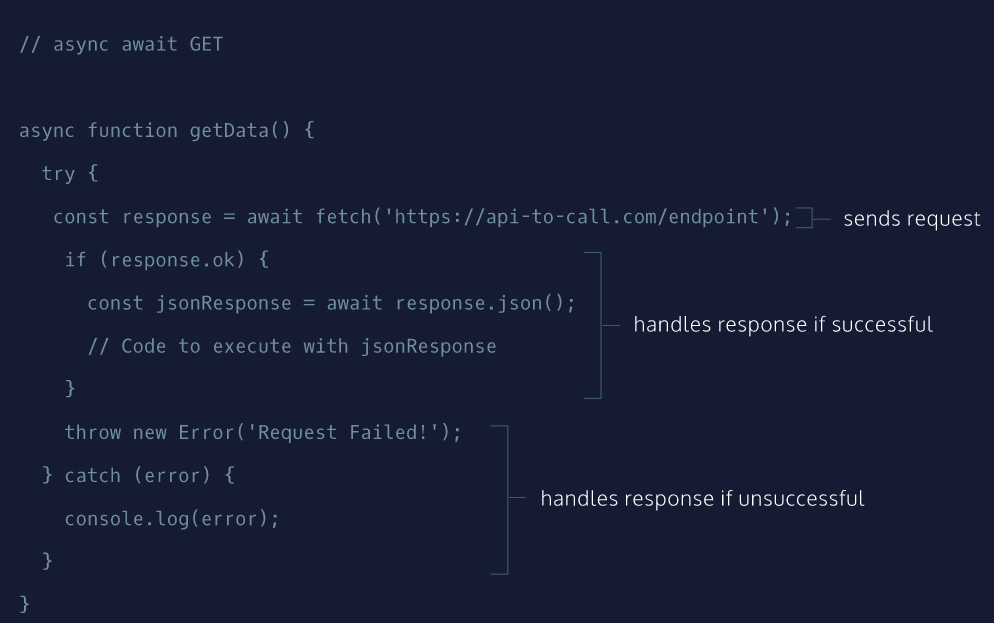
const getData = async () => {
try {
const response = await fetch('https://api-to-call.com/endpoint');
if (response.ok) {
const jsonResponse = await response.json();
return jsonResponse;
}
throw new Error('Request failed!')
} catch (error) {
console.log(error);
}
}