조합, 중복조합
function getCombinations(arr, selectNumber) {
const result = []
if (selectNumber === 1) return arr.map(v=>[v])
arr.forEach( (fixed, index,origin) => {
let rest = origin.slice(index+1)
let combinations = getCombinations(rest, selectNumber-1)
let attach = combinations.map(combination => [fixed, ...combination])
result.push(...attach)
});
return result
}


순열
function getCombinations(arr, selectNumber) {
const result = []
if (selectNumber === 1) return arr.map(v=>[v])
arr.forEach( (fixed, index,origin) => {
let rest = [...origin.slice(0,index),...origin.slice(index+1)]
let combinations = getCombinations(rest, selectNumber-1)
let attach = combinations.map(combination => [fixed, ...combination])
result.push(...attach)
});
return result
}

부분 수열
function getCombinations(arr, selectNumber) {
const result = []
if (selectNumber === 1) return arr.map(v=>[v])
arr.forEach( (fixed, index,origin) => {
let rest = [...origin.slice(0,index),...origin.slice(index+1)]
let combinations = getCombinations(rest, selectNumber-1)
result.push(...combinations)
let attach = combinations.map(combination => [fixed, ...combination])
result.push(...attach)
});
return result
}
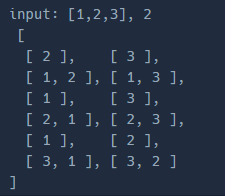
중복 순열
function getCombinations(arr, selectNumber) {
const result = []
if (selectNumber === 1) return arr.map(v=>[v])
arr.forEach( (fixed, index,origin) => {
let combinations = getCombinations(arr, selectNumber-1)
let attach = combinations.map(combination => [fixed, ...combination])
result.push(...attach)
});
return result
}
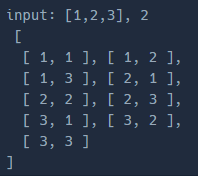
부분 집합
function getCombinations(arr){
let result = [];
for(let i = 1; i < (1 << arr.length); i++) {
result.push([]);
for(let j = 0; j < arr.length; j++) {
if(i & (1 << j)) result[i-1].push(arr[j])
}
}
return result
}

모든 경우의 수
function getCombinations(arr, selectNumber) {
const result = []
if (selectNumber === 1) return arr.map(v=>[v])
arr.forEach( (fixed, index,origin) => {
let rest = [...origin.slice(0,index),...origin.slice(index+1)]
let combinations = getCombinations(rest, selectNumber-1)
let attach = combinations.map(combination => [fixed, ...combination])
result.push(...attach)
});
return result
}
const getAllPermutations = (arr) => {
let results = [];
arr.forEach((value, index, origin) => {
results.push(...getCombinations(origin, index + 1));
});
return results;
}
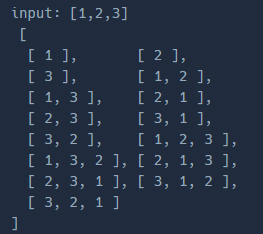