문제
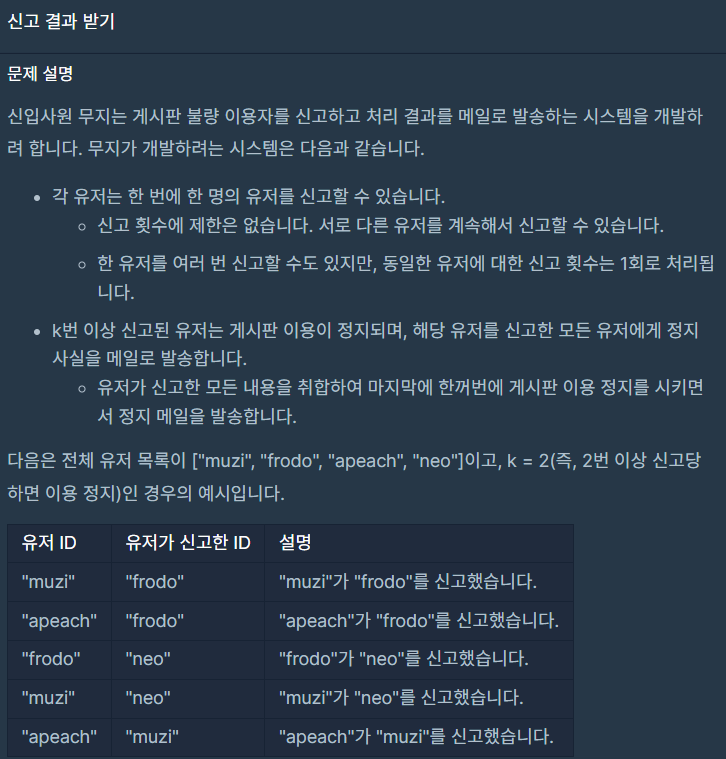
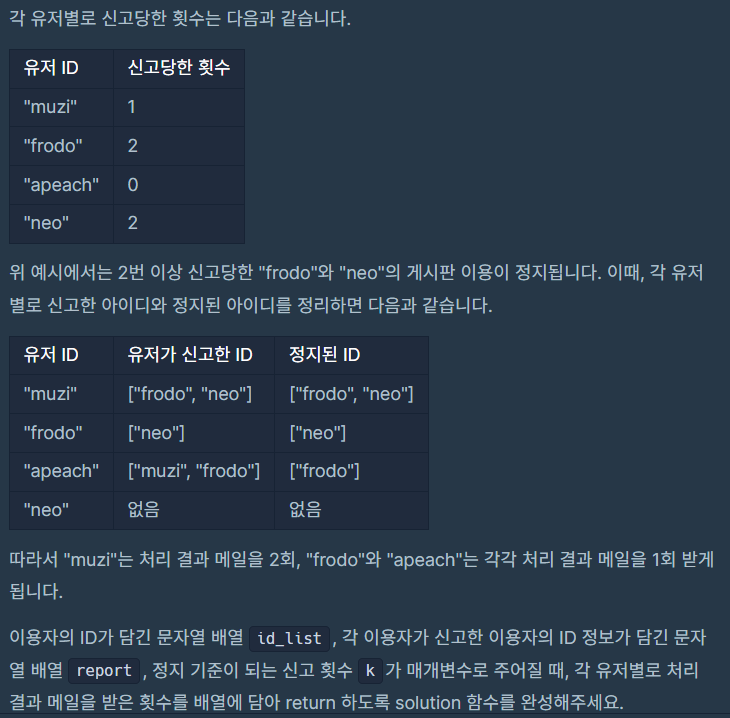
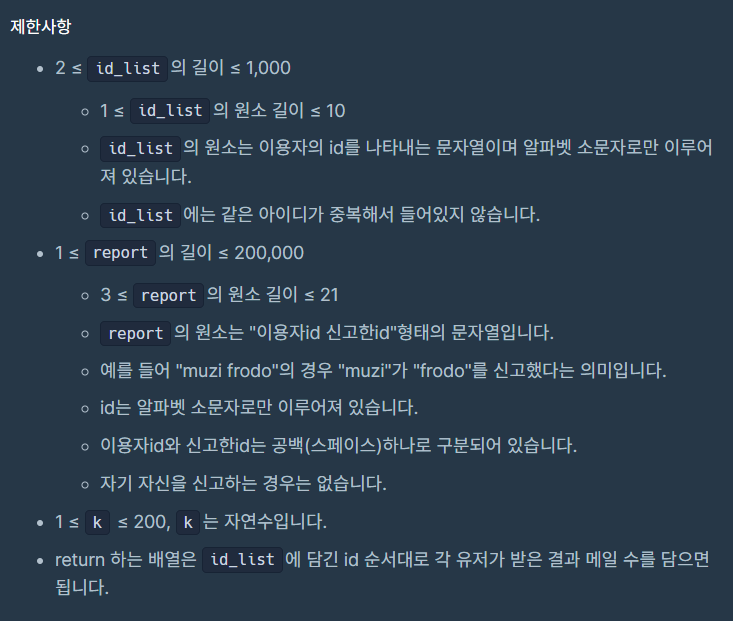
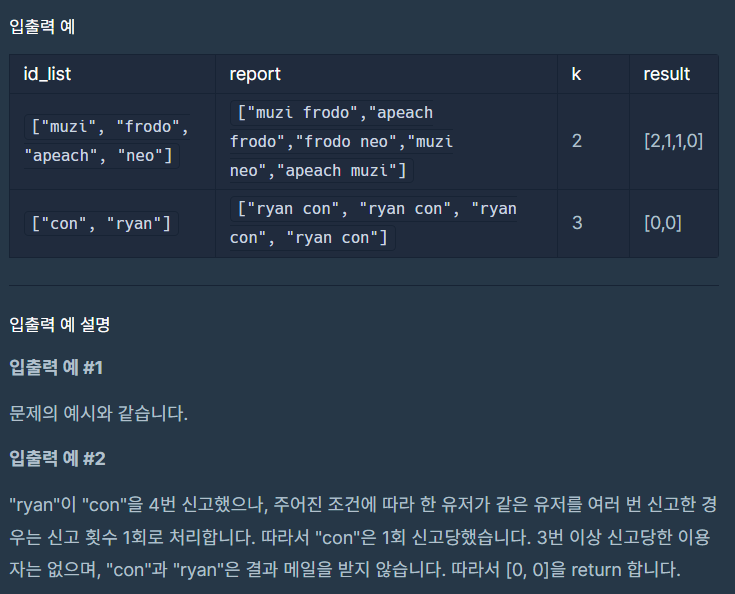
풀이 1
function solution(id_list, report, k) {
report = report.map(v => {
return v.split(' ')
})
let userList = {}
for (let id of id_list){
userList[id] = []
}
let userListclone = () => JSON.parse(JSON.stringify(userList))
let userReportingList = userListclone()
report.map(list => {
userReportingList[list[0]].push(list[1])
userReportingList[list[0]] = [...new Set(userReportingList[list[0]])]
})
let userReportedList = userListclone()
Object.values(userReportingList).map(reports => {
reports.map(reported => {
userReportedList[reported].push(reported)
})
})
let penaltyList = []
for (let [key,value] of Object.entries(userReportedList)){
if(value.length >= k) penaltyList.push(key)
}
let emailList = new Array(id_list.length).fill(0)
for (let i=0; i<id_list.length; i++) {
penaltyList.map(reported => {
if (userReportingList[id_list[i]].includes(reported)) emailList[i]++
})
}
return emailList
}
}
풀이 2
function solution(id_list, report, k) {
let reports = [...new Set(report)].map(a=>{return a.split(' ')});
let counts = new Map();
for (const bad of reports){
counts.set(bad[1],counts.get(bad[1])+1||1)
}
let good = new Map();
for(const report of reports){
if(counts.get(report[1])>=k){
good.set(report[0],good.get(report[0])+1||1)
}
}
let answer = id_list.map(a=>good.get(a)||0)
return answer;
}
풀이 3
function solution(id_list, report, k) {
report = [...new Set(report)];
const reported = report.map(el => el.split(' ')[1]);
const reported_final = [];
const count = new Array(id_list.length).fill(0);
reported.forEach(el => {
count[id_list.indexOf(el)]++;
});
count.forEach((el, i) => {
if(el >= k) {
reported_final.push(id_list[i])
}
});
count.fill(0);
report.forEach((el) => {
el = el.split(' ');
if(reported_final.includes(el[1])) {
count[id_list.indexOf(el[0])]++;
}
})
return count;
}
풀이 4
function solution(id_list, report, k) {
let userId = Array.from(Array(id_list.length), () => 0)
let target = [];
let result = {};
new Set(report).forEach(f => {
const r = f.split(' ');
const to = r[1];
target.push(r);
result[to] = (result[to] || 0) + 1;
})
Object.keys(result).forEach(f => {
if (result[f] >= k) {
target.filter(a => a[1] == f).forEach(m => userId[id_list.indexOf(m[0])]++)
}
})
return userId;
}