문제
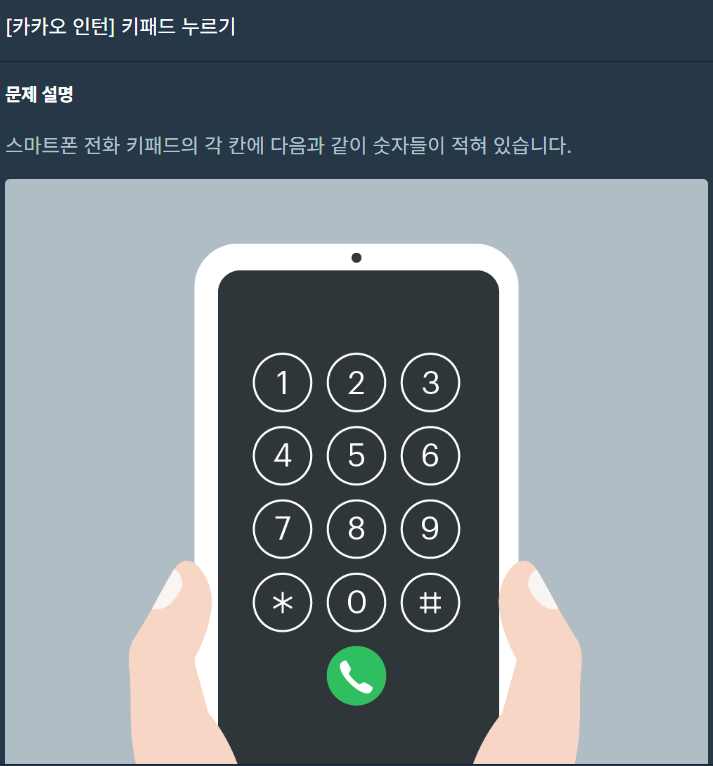
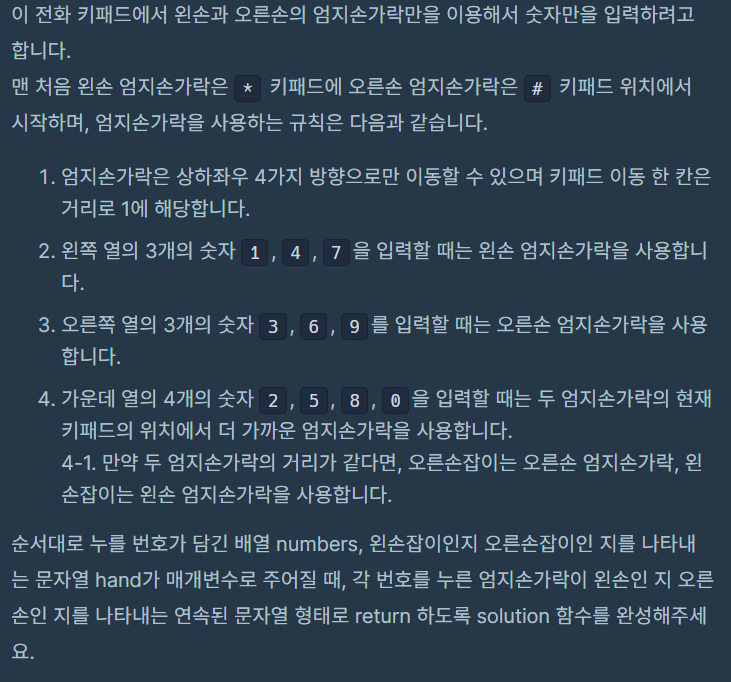
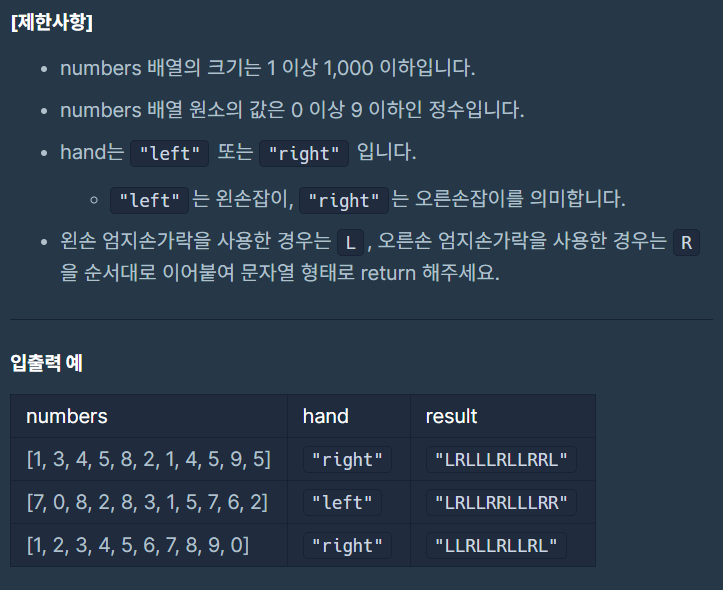
풀이 1
function solution(numbers, hand) {
var answer = '';
let key = {
'1' : [0,0],
'2' : [0,1],
'3' : [0,2],
'4' : [1,0],
'5' : [1,1],
'6' : [1,2],
'7' : [2,0],
'8' : [2,1],
'9' : [2,2],
'*' : [3,0],
'0' : [3,1],
'#' : [3,2]
}
let left = key['*']
let right = key['#']
numbers.map((v,i) => {
let target = key[v]
if (v===1 || v=== 4 || v=== 7) {
answer += 'L'
left = target
}
else if (v===3 || v=== 6 || v=== 9) {
answer += 'R'
right = target
}
else {
let leftCount = Math.abs(target[0] - left[0]) + Math.abs(target[1] - left[1])
let rightCount = Math.abs(target[0] - right[0]) + Math.abs(target[1] - right[1])
if (leftCount < rightCount) {
left = target
answer += 'L'
}
else if (rightCount < leftCount) {
right = target
answer += 'R'
}
else {
if (hand === 'left') {
left = target
answer += 'L'
}
else {
right =target
answer += 'R'
}
}
}
})
return answer;
}
풀이 2
function solution(numbers, hand) {
hand = hand[0] === "r" ? "R" : "L"
let position = [1, 4, 4, 4, 3, 3, 3, 2, 2, 2]
let h = { L: [1, 1], R: [1, 1] }
return numbers.map(x => {
if (/[147]/.test(x)) {
h.L = [position[x], 1]
return "L"
}
if (/[369]/.test(x)) {
h.R = [position[x], 1]
return "R"
}
let distL = Math.abs(position[x] - h.L[0]) + h.L[1]
let distR = Math.abs(position[x] - h.R[0]) + h.R[1]
if (distL === distR) {
h[hand] = [position[x], 0]
return hand
}
if (distL < distR) {
h.L = [position[x], 0]
return "L"
}
h.R = [position[x], 0]
return "R"
}).join("")
}
풀이 3
function solution(numbers, hand) {
let answer = '';
const grid = [[0,-2], [-1,1], [0,1],
[1,1], [-1,0], [0,0],
[1,0], [-1,-1], [0,-1],
[1,-1], [-1,-2], [1,-2]];
let L = 10;
let R = 11;
let L_steps, R_steps;
hand = hand[0].toUpperCase();
numbers.forEach(el => {
switch (grid[el][0]){
case -1:
answer += "L";
L = el;
break;
case 1:
answer += "R";
R = el;
break;
case 0:
L_steps = Math.abs(grid[L][0] - grid[el][0]) + Math.abs(grid[L][1] - grid[el][1]);
R_steps = Math.abs(grid[R][0] - grid[el][0]) + Math.abs(grid[R][1] - grid[el][1]);
if(L_steps > R_steps){
answer += "R";
R = el;
} else if (L_steps < R_steps){
answer += "L";
L = el;
} else {
answer += hand;
eval(`${hand} = el`);
}
}
});
return answer;
}