1.문제
You are given an array coordinates, coordinates[i] = [x, y], where [x, y] represents the coordinate of a point. Check if these points make a straight line in the XY plane.
xy 평면상의 점들의 배열이 주어질 때 이 점들이 한 직선위에 위치하는지 묻는 문제이다.
Example 1
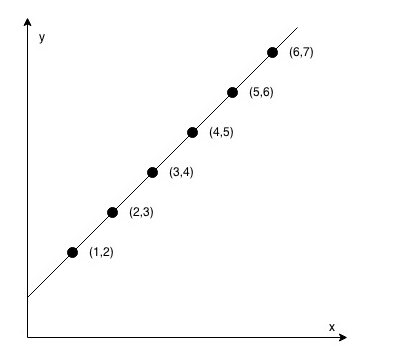
Input: coordinates = [[1,2],[2,3],[3,4],[4,5],[5,6],[6,7]]
Output: true
Example 2
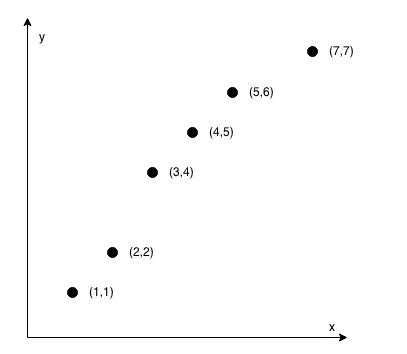
Input: coordinates = [[1,1],[2,2],[3,4],[4,5],[5,6],[7,7]]
Output: false
2.풀이
- 모든 점들이 한 직선 위에 있는지 체크하는 것이므로 임의의 두점을 잡아 기울기를 구한다.
- 그 다음 점들을 두개씩 잡아 순회하면서 구해둔 기울기와 현재 잡은 두 점의 기울기를 비교한다.
- 만약 기울기 값이 다르다면 false를 리턴
/**
* @param {number[][]} coordinates
* @return {boolean}
*/
const checkStraightLine = function (coordinates) {
let inclination =
(coordinates[0][1] - coordinates[1][1]) /
(coordinates[0][0] - coordinates[1][0]); // 처음 두 연속된 점의 기울기
for (let i = 0; i < coordinates.length - 1; i++) {
for (let j = i + 1; j < coordinates.length; j++) {
if (
// 각 이웃한 점의 기울기를 처음 두 연속된 점의 기울기와 비교
(coordinates[i][1] - coordinates[j][1]) /
(coordinates[i][0] - coordinates[j][0]) !==
inclination
) {
if (
//만약 기울기의 절댓값이 무한대라면 continue
Math.abs(inclination) === Infinity &&
Math.abs(
(coordinates[i][1] - coordinates[j][1]) /
(coordinates[i][0] - coordinates[j][0])
) === Infinity
) {
continue;
} else {
return false;
}
}
}
}
return true;
};
3.결과
