우지코 보면서 빡세게 코딩하기
👩💻 배운것
Data Structure
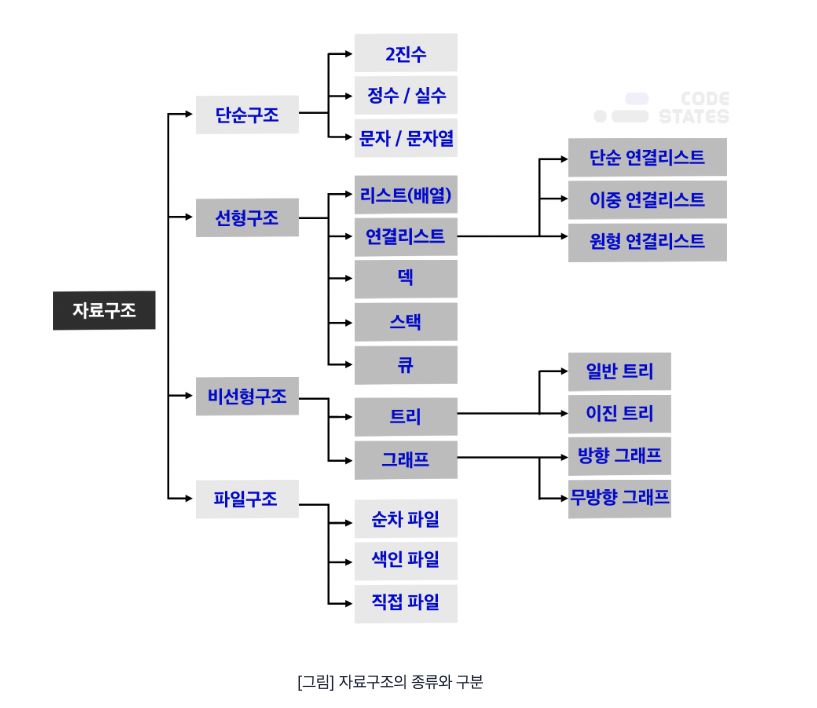
- 자주 등장하는 Stack, Queue, Tree, Graph
Stack
- LIFO (Last in First out)
- 프링글스같은거
- 데이터를 push, pop
class Stack {
constructor() {
this.storage = {};
this.top = 0; // 스택의 가장 상단을 가리키는 포인터 변수를 초기화 합니다.
}
size() {
return this.top;
}
// 스택에 데이터를 추가 할 수 있어야 합니다.
push(element) {
this.storage[this.top] = element;
this.top += 1;
}
// 가장 나중에 추가된 데이터가 가장 먼저 추출되어야 합니다.
pop() {
// 빈 스택에 pop 연산을 적용해도 에러가 발생하지 않아야 합니다
if (this.size()<=0) {
return;
}
const result = this.storage[this.top-1];
delete this.storage[this.top-1];
this.top -= 1;
return result;
}
}
Queue
- FIFO (First in First Out)
- enqueue, dequeue
class Queue {
//가장 앞에 있는 요소를 front,
//가장 뒤에 있는 요소를 rear 라고 했을 때
//queue constructor 생성
constructor() {
this.storage = {};
this.front = 0;
this.rear = 0;
}
size() {
return this.rear - this.front;
}
enqueue(element) {
this.storage[this.rear] = element;
this.rear += 1;
}
dequeue() {
if (this.size() <= 0) {
return;
}
const result = this.storage[this.front];
delete this.storage[this.front];
this.front += 1;
return result;
}
}
👫 페어프로그래밍
- 둘다 1,2번은 풀어놨어서 3번부터 풀기로 했다
function browserStack(actions, start) {
// TODO: 여기에 코드를 작성합니다.
if (typeof start != 'string'){
return false
}
let prev = [];
let next = [];
let curr = start;
for(let i=0; i<actions.length; i++){
//if action[i]=1일경우
//else if action[i]=-1일경울
//else if action[i]=스트링일경우
if (actions[i]===1 && next.length != 0){
prev.push(curr);
let n = next.pop();
curr = n;
} else if(actions[i]===-1 && prev.length != 0){
next.push(curr);
let p = prev.pop();
curr = p;
} else if (typeof actions[i] === 'string'){
prev.push(curr);
curr=actions[i];
next=[];
}
}
let output=[prev, curr, next]
return output
}
- 이문제에서 뒤로가기/앞으로가기 스택에 밸류가 없을경우를 찾아내는데 시간이 걸렸다
- 4번문제는 잘 모르겠어서 풀다가 레퍼런스를 봐야했다..
👍 후기
귀욤일 쭐 알았는데 불독이었넵 스택큐
Artist부터 지아코 앓았는데 이 새벽에 또 귀호강