인프런의 자바 알고리즘 문제풀이 강의를 공부합니다
1. 문자찾기
public class Main01 {
public static int solution(String str, char t){
int answer=0;
str = str.toUpperCase();
t = Character.toUpperCase(t);
for (int i=0; i<str.length(); i++){
if (str.charAt(i)==t)
answer++;
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.next();
char c = sc.next().charAt(0);
System.out.print(solution(str, c));
}
}
.charAt(인덱스번호)
: char타입으로 변환하여 문자 읽기
.toUpperCase()
: String 대문자변환
Character.toUpperCase(t)
: char타입 문자 대문자 변환
2. 대소문자 변환
public class Main02 {
public static String solution(String str){
String answer = "";
for (int i=0; i<str.length(); i++){
if (str.charAt(i) == str.toUpperCase().charAt(i))
answer += str.toLowerCase().charAt(i);
else
answer += str.toUpperCase().charAt(i);
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.nextLine();
System.out.println(solution(str));
}
}
강의 코드
public class reMain02 {
public static String solution(String str){
String answer = "";
for (char x : str.toCharArray()){
if (Character.isLowerCase(x))
answer += Character.toUpperCase(x);
else
answer += Character.toLowerCase(x);
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.next();
System.out.println(solution(str));
}
}
for(char(자료형) x(변수명) : array(배열명)) { 문장 }
향상된 for문
str.toCharArray()
: 문자를 charArray로 변환
3. 문장속 단어
방법1 : split
public class Main03 {
public static String solution(String str){
String answer = "";
int m = Integer.MIN_VALUE;
String[] s= str.split(" ");
for (String x: s){
int len= x.length();
if (len>m){
m=len;
answer=x;
}
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.nextLine();
System.out.println(solution(str));
}
}
.split(String 구분자)
: 구분자를 바탕으로 배열형식으로 문자열을 잘라준다
str.split(" ")
: 공백으로 분리 (, @ 등 활용가능)
.split(String 구분자, int limit)
: 구분자를 바탕으로 문자열을 자르지만 limit수만큼 잘라준다
방법2 : indexOf, subString
public class reMain03 {
public static String solution(String str){
String answer = "";
int m = Integer.MIN_VALUE, pos;
while ((pos = str.indexOf(' '))!= -1){
String tmp = str.substring(0, pos);
int len = tmp.length();
if (len>m){
m=len;
answer = tmp;
}
str = str.substring(pos+1);
}
if (str.length()>m)
answer = str;
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.nextLine();
System.out.println(solution(str));
}
}
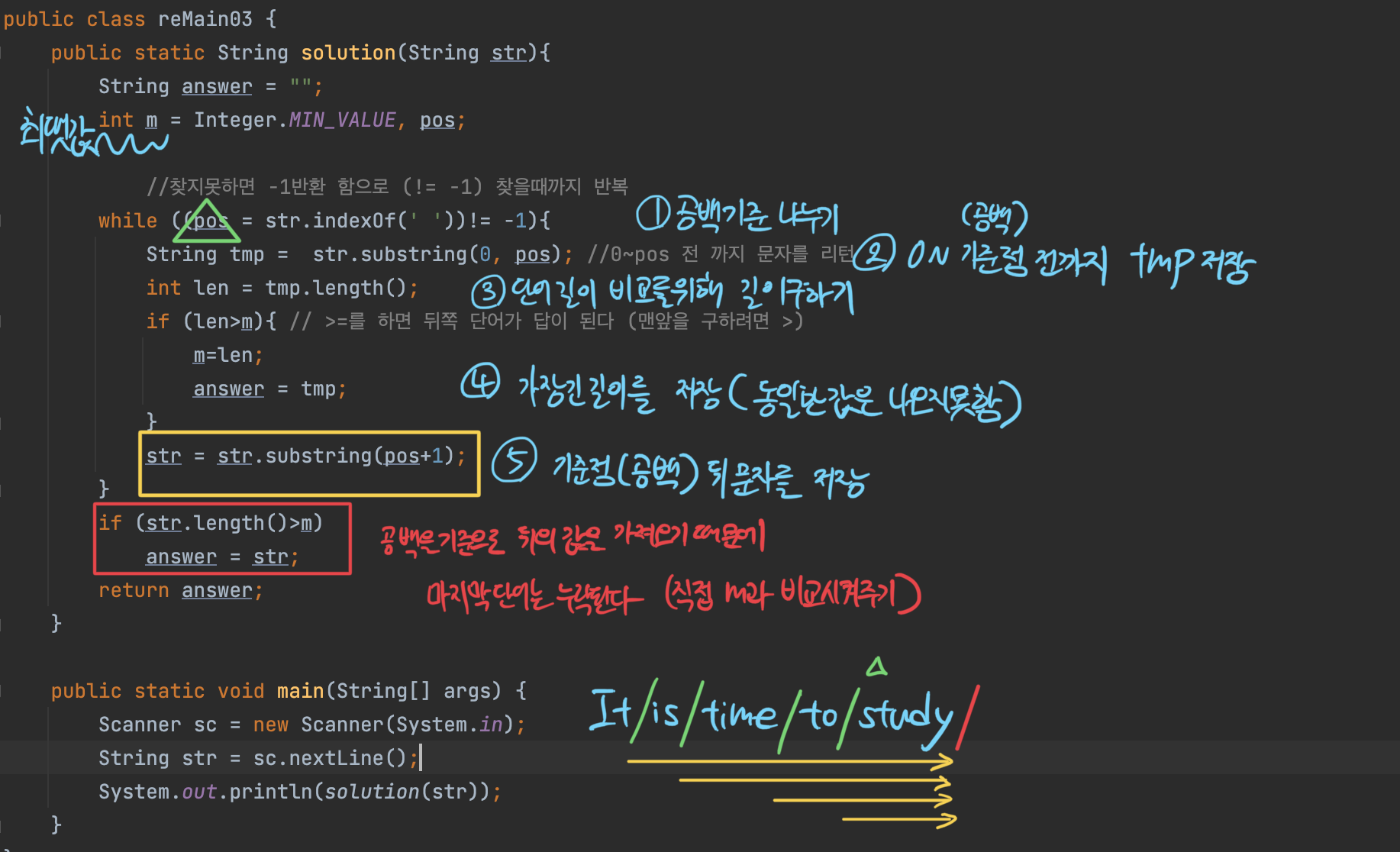
.indexOf()
: 문자열에서 특정 문 자열 위치를 찾아 인덱스idx값을 반환해준다. 찾지못하면 -1을 반환
str.indexOf(' ')
: 띄어쓰기 위치를 반환
.subString(int index)
: 인자를 하나만 받는 함수 ⇒ 해당위치를 포함하여 이후 모든 문자열 리턴
.subString(int be, int fe)
: 인자를 두개 받는 함수 ⇒ be~fe전 위치까지 값을 리턴
4. 단어 뒤집기
public class reMain04 {
public static ArrayList<String>solution(int n, String[]str){
ArrayList<String>answer = new ArrayList<>();
for (String x : str){
String tmp = new StringBuilder(x).reverse().toString();
answer.add(tmp);
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
String[] str = new String[n];
for (int i=0; i<n; i++){
str[i] = sc.next();
}
for (String x: solution(n, str)){
System.out.println(x);
}
}
}
StringBuilder
: string은 불변 객체임으로 더하면 그만큼 메모리할당이 되기 대문에 성능에 안좋음
StringBuilder는 문자열을 더할때 새로운 객체를 생성하는 것이 아닌 기존의 데이터에 더하는 방식 때문에 상대적으로 부하가 적다
StringBuilder().append()
: 문자열을 더한다
StringBuilder().toString()
: 문자열 출력
StringBuilder(x).reverse().toString()
: 문자 뒤집기
5. 특정 문자 뒤집기
public class Main05 {
public static String solution(String str){
String answer = "";
char[]s = str.toCharArray();
int lt=0, rt=str.length()-1;
while (lt<rt){
if (!Character.isAlphabetic(s[lt]))
lt++;
else if (!Character.isAlphabetic(s[rt]))
rt--;
else{
char tmp=s[lt];
s[lt] = s[rt];
s[rt] = tmp;
lt++;
rt--;
}
}
answer = String.valueOf(s);
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.next();
System.out.println(solution(str));
}
}
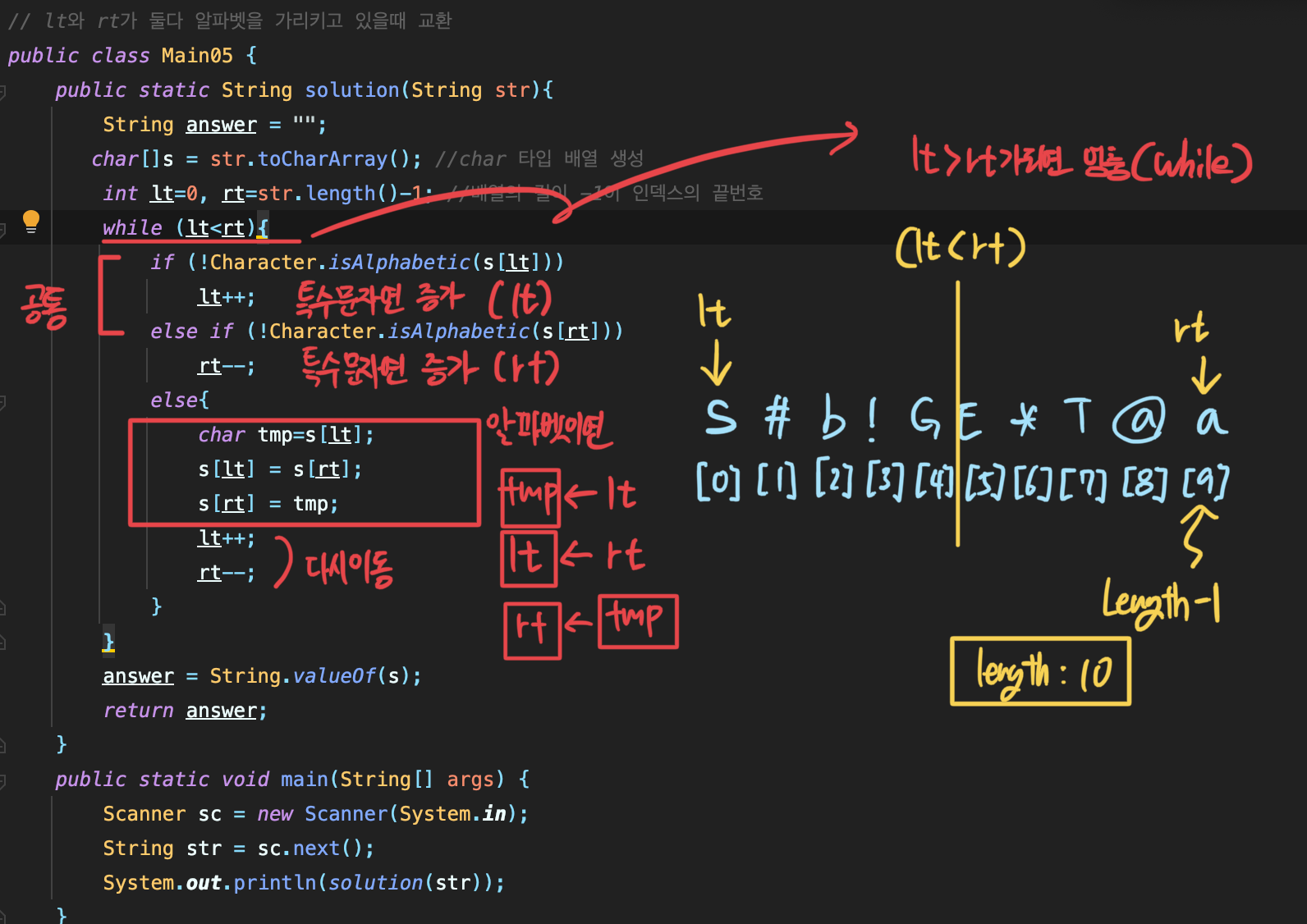
Character.isAlphabetic
: char타입 영문자 확인 메소드 (boolean리턴)
String.valueOf(n)
: 들어온 n의 값을 문자열로 변환
6. 중복 문자 제거
public class reMain06 {
public static String solution(String str){
String answer = "";
for (int i=0; i<str.length(); i++){
if (str.indexOf(str.charAt(i))==i){
answer += str.charAt(i);
}
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.next();
System.out.println(solution(str));
}
}
indexOf()
: 특정 문자나 문자열이 앞에서부터 처음 발견되는 인덱스를 반환하며 찾지 못할경우 -1를 반환한다
indexOf(”찾을 특정문자”, “시작할 위치”)
: 시작할 위치는 생략가능, 생략일 경우 0부터 시작
7. 회문 문자열
방법1: charAt & UpperCase 사용 코드
public class Main07 {
public static String solution(String str){
String answer = "YES";
str = str.toUpperCase();
int len = str.length();
for (int i=0; i<len/2; i++){
if (str.charAt(i) != str.charAt(len-i-1)){
answer="NO";
}
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.next();
System.out.println(solution(str));
}
}
방법2: StringBuilder사용 코드
public class Main07_2 {
public static String solution(String str){
String answer = "NO";
String sb = new StringBuilder(str).reverse().toString();
if (str.equalsIgnoreCase(sb)){
answer="YES";
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.next();
System.out.println(solution(str));
}
}
.equalsIgnoreCase()
: 대소문자 구분없이 같은지 확인 true, false 리턴
.equals()
: 대소문자 구분하여 같은지 확인 true, false 리턴
8. 유효한 팰린드롬
public class Main08 {
public static String solution(String str){
String answer = "NO";
str = str.toUpperCase().replaceAll("[^A-Z]","");
String tmp = new StringBuilder(str).reverse().toString();
if (str.equals(tmp))
answer="YES";
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.nextLine();
System.out.println(solution(str));
}
}
.replaceAll("변환하고 싶은것", "변환할 문자값(정규식)")
: 대상 문자열을 원하는 문자값으로 변환하는 함수
- 정규 표현식 문법
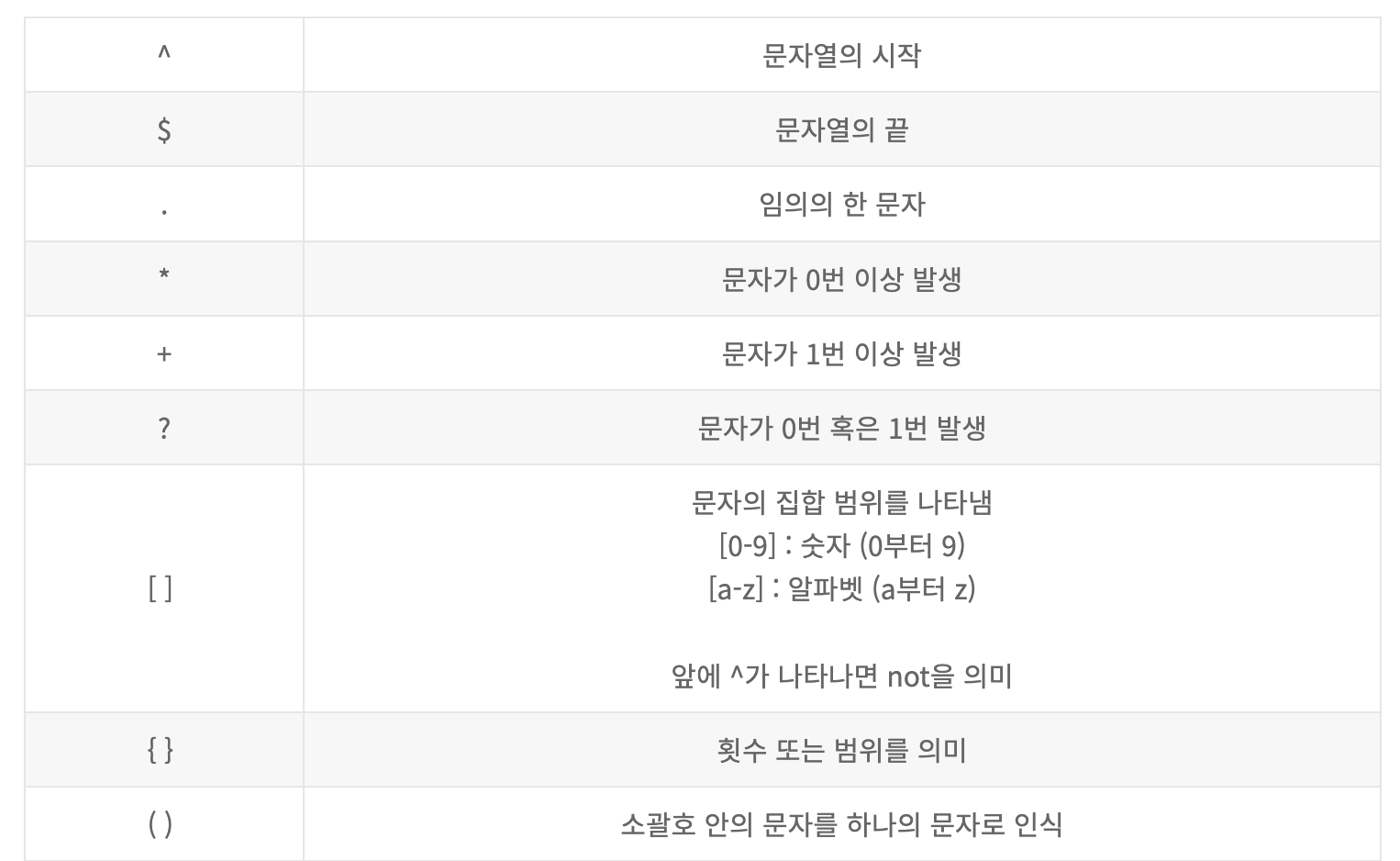
- 자주사용하는 정규 표현식
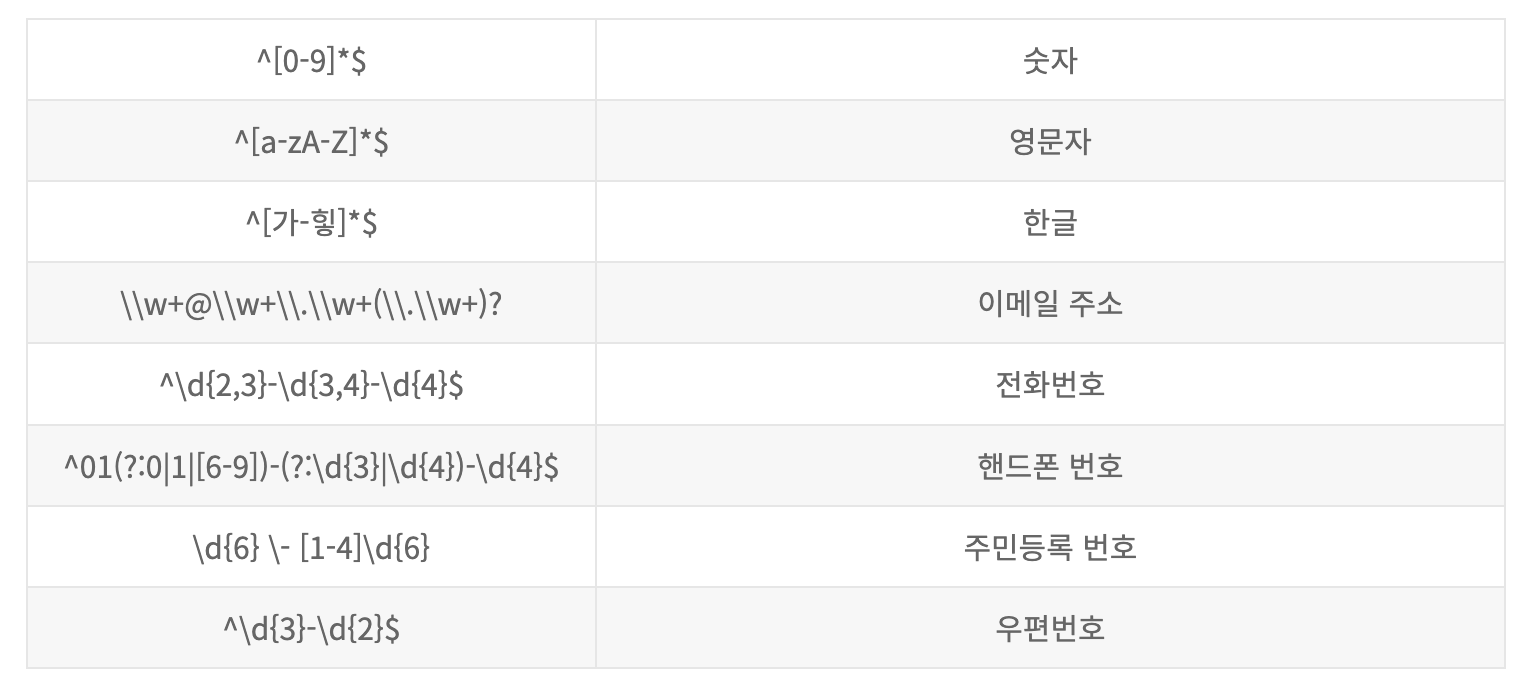
9. 숫자만 추출
방법1: 숫자(아스키코드)로 풀기
public class Main09 {
public static int solution(String str){
int answer =0;
for (char x : str.toCharArray()){
if (x>=48 && x<=57)
answer=answer*10+(x-48);
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.next();
System.out.println(solution(str));
}
}
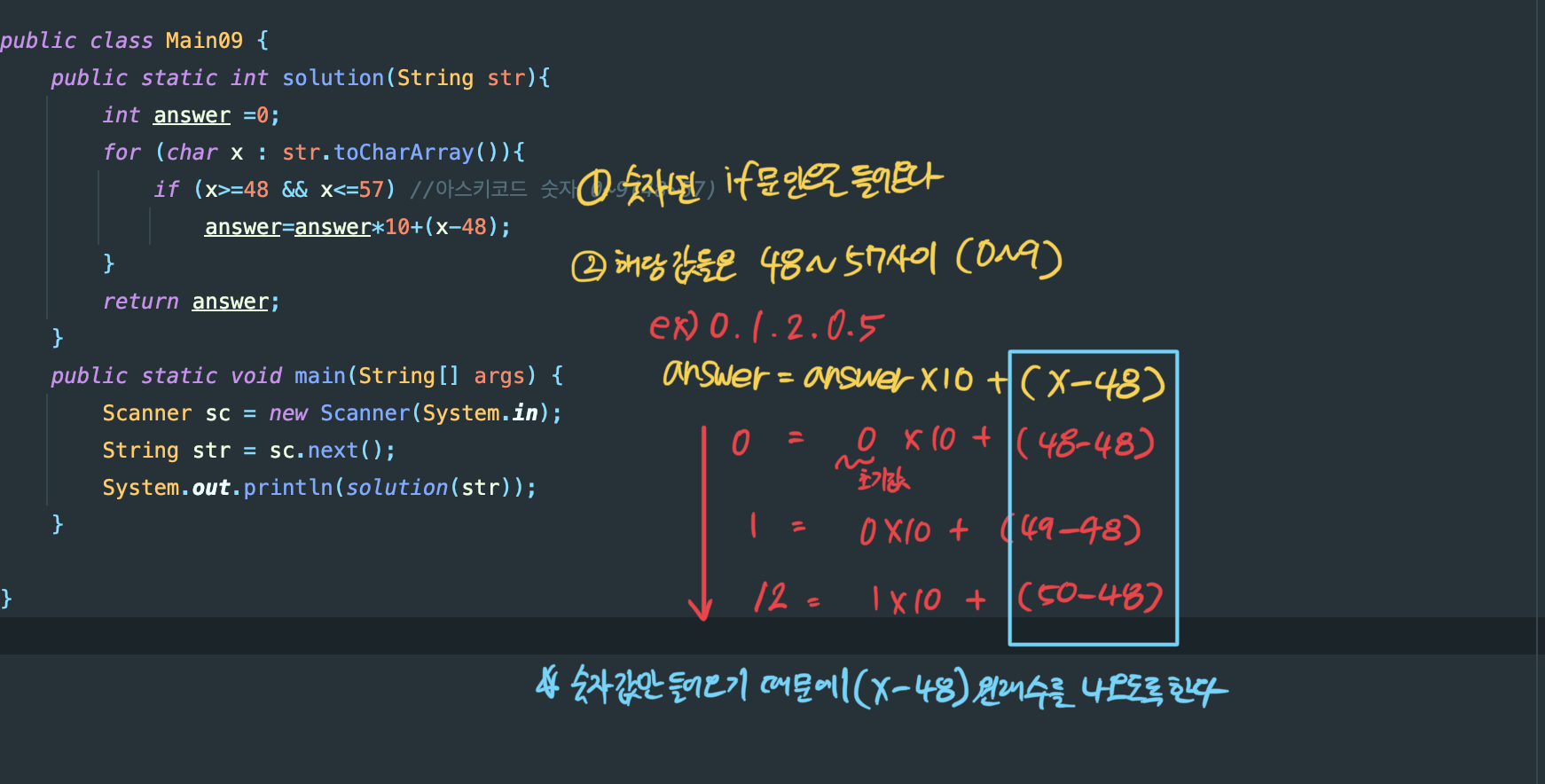
- 아스키코드(숫자, 대문자, 소문자 외우기!)
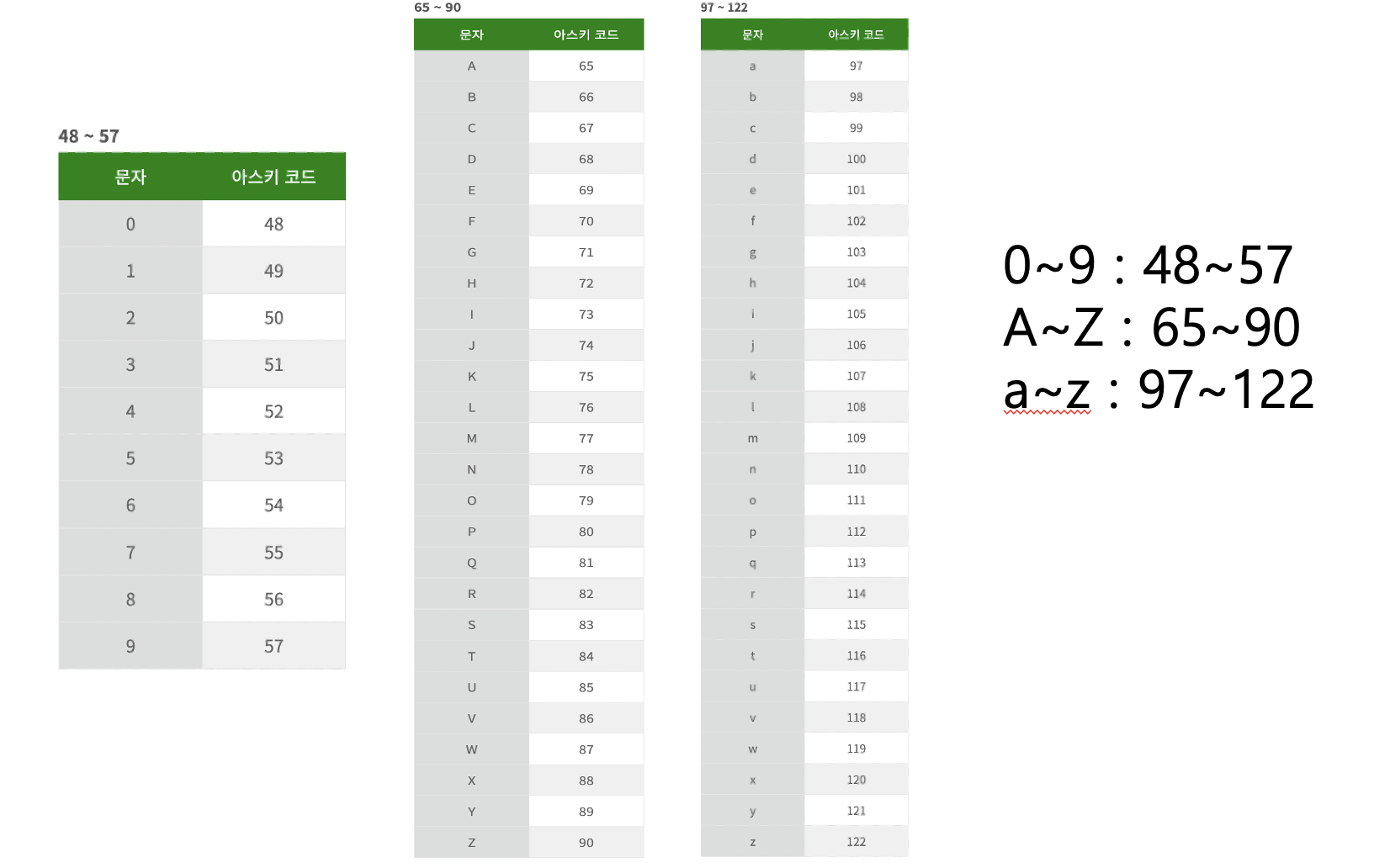
방법2: 문자열로 풀기
public class Main09_2 {
public static int solution(String str){
String answer ="";
for (char x : str.toCharArray()){
if (Character.isDigit(x)){
answer += x;
}
}
return Integer.parseInt(answer);
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.next();
System.out.println(solution(str));
}
}
Character.isDigit(x)
: 명시된 char값이 숫자인지 여부를 판단하여 true, false 리턴
Integer.parseInt()
: String타입의 숫자를 int 타입으로 변환해준다
- Byte.parseByte() , Short.parseShort(), Long.parseLong() 등 사용 가능
charAt()
: String으로 저장된 문자열 중에서 한 글자만 선택해서 char타입으로 변환
10. 가장 짧은 문자거리
public class Main10 {
public static int[] solution(String str, char t){
int[] answer = new int[str.length()];
int p=1000;
for (int i=0; i< str.length(); i++){
if (str.charAt(i)==t){
p=0;
answer[i]=p;
}
else{
p++;
answer[i]=p;
}
}
p=1000;
for (int i= str.length()-1; i>=0; i--){
if (str.charAt(i)==t)
p=0;
else{
p++;
answer[i]=Math.min(answer[i],p);
}
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.next();
char t = sc.next().charAt(0);
for (int x : solution(str, t)){
System.out.print(x + " ");
}
}
}
- `Math.min(answer[i],p)` : 두개의 인자를 비교하여 작은 값을 리턴
- 오로지 숫자(정수, 실수)만 비교가 가능하며 문자열은 비교가 불가능하다
- double, float, int, long 4개의 타입으로 입력이 가능하다
11. 문자열 압축
public class Main11 {
public static String solution(String str){
String answer = "";
str = str+" ";
int cnt=1;
for (int i=0; i< str.length()-1; i++){
if (str.charAt(i) == str.charAt(i+1)){
cnt ++;
}else {
answer += str.charAt(i);
if (cnt>1)
answer += String.valueOf(cnt);
cnt=1;
}
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.next();
System.out.println(solution(str));
}
}
- `String.valueOf(num)` : Object 값을 String 형으로 변환 할 때 사용하는 메소드
toString()
: Object 값을 String 형으로 변환 할 때 사용하는 메소드
- null이 오면 “null”이라는 문자열을 출력
12. 암호
public class Main12 {
public static String solution(int n, String s){
String answer="";
for (int i=0; i<n; i++){
String tmp = s.substring(0, 7).replace('#','1').replace('*','0');
int num=Integer.parseInt(tmp, 2);
answer += (char)num;
s = s.substring(7);
}
return answer;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
String str = sc.next();
System.out.println(solution(n, str));
}
}
Integer.parseInt(String s)
: 문자열(s)을 10진수로 읽어서 int로 반환
Integer.parseInt(String s, int radix)
: 문자열(s)을 변환할 진수(radix)로 읽어서 int로 반환 (radix에 10을 넣으면 위의 메소드와 동일한 결과 도출)
String replace(찾을 문자열, 바꿀 문자열)
: 문자열 변경
자바코테.... 추억이군