interface LinkedList {
ListNode add(ListNode head, ListNode nodeToAdd, int position);
ListNode remove(ListNode head, int positionToRemove);
void printList(ListNode head);
boolean contains(ListNode head, ListNode nodeToCheck);
}
public class ListNode implements LinkedList{
private int data;
private ListNode next;
public ListNode(int data) {
this.data = data;
next = null;
}
public ListNode() {
}
@Override
public ListNode add(ListNode head, ListNode nodeToAdd, int position) {
if (position == 0 && head == null) {
head = nodeToAdd;
return head;
}
else if (position == 0){
nodeToAdd.next = head;
head = nodeToAdd;
return head;
}
ListNode node = head;
for (int i = 0; i < position - 1; i++) {
node = node.next;
}
nodeToAdd.next = node.next;
node.next = nodeToAdd;
return head;
}
@Override
public ListNode remove(ListNode head, int positionToRemove) {
if (positionToRemove == 0) {
head = head.next;
return head;
}
else {
ListNode node = head;
for(int i=0; i< positionToRemove; i++) {
node = node.next;
}
node.next = node.next.next;
return head;
}
}
@Override
public void printList(ListNode head) {
ListNode node = head;
while (node != null) {
System.out.print(node.data);
node = node.next;
}
System.out.println();
}
@Override
public boolean contains(ListNode head, ListNode nodeToCheck) {
ListNode node = head;
while(node != null) {
if (node.data == nodeToCheck.data) {
return true;
}
node = node.next;
}
return false;
}
public static void main(String[] args) {
LinkedList nums = new ListNode();
ListNode head = null;
for(int i=0; i<5; i++) {
head = nums.add(head, new ListNode(i), i);
}
nums.printList(head);
head = nums.remove(head, 0);
head = nums.remove(head, 0);
nums.printList(head);
System.out.println(nums.contains(head, new ListNode(3)));
System.out.println(nums.contains(head, new ListNode(1)));
}
}
- ListNode add(ListNode head, ListNode nodeToAdd, int position)
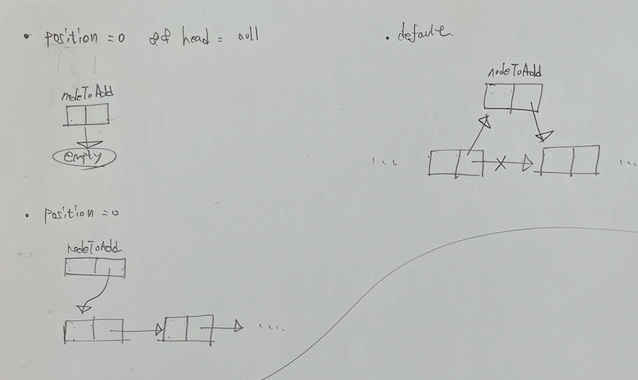
- ListNode remove(ListNode head, int positionToRemove);
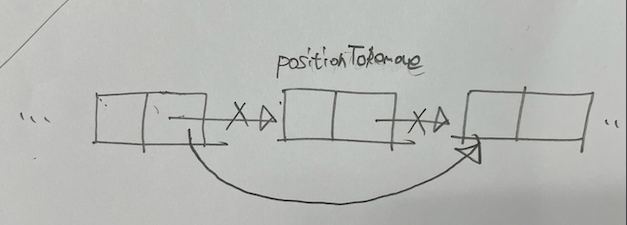