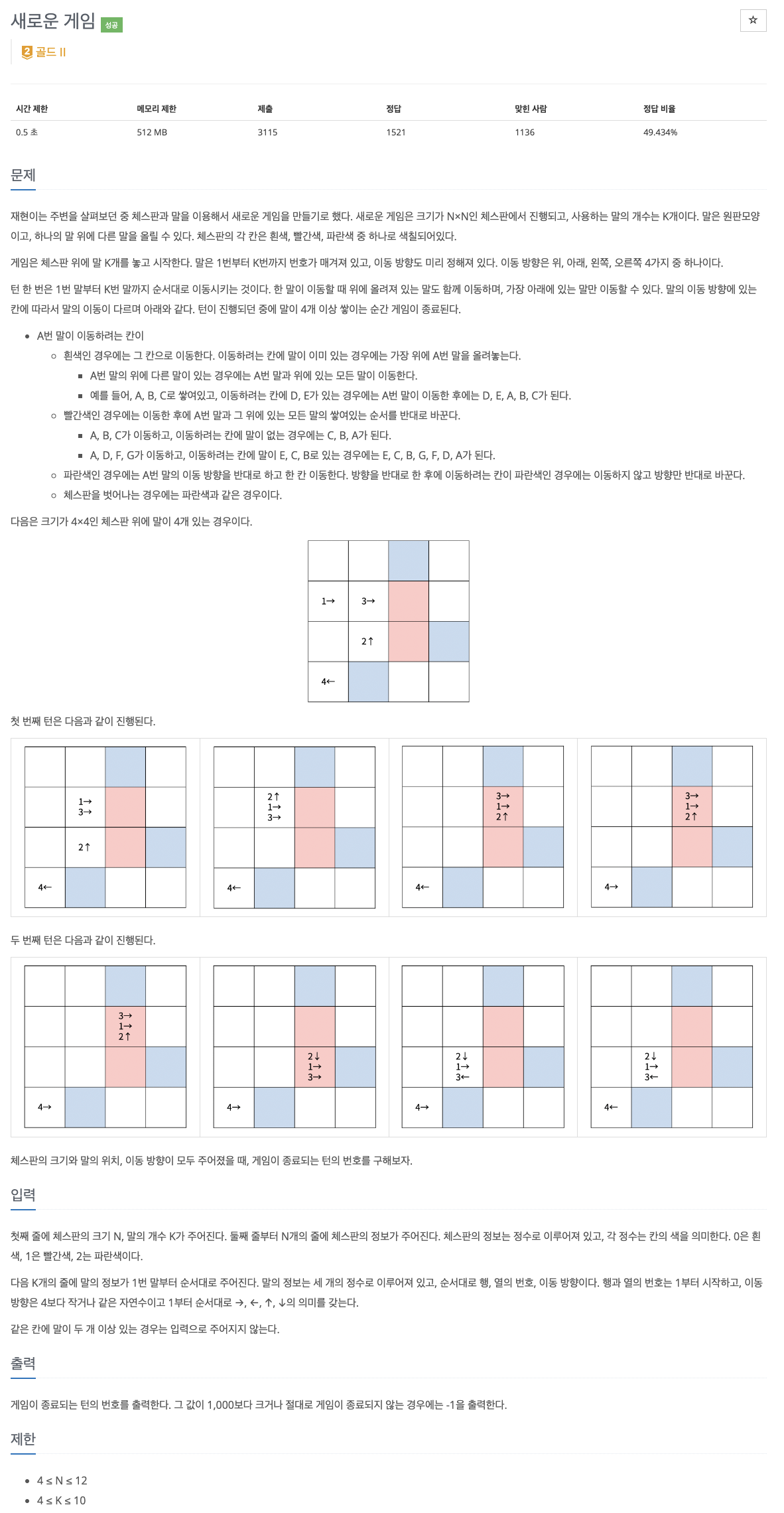
풀이 시간
구현 방식
- 설명대로 구현하면 되는 시뮬레이션 문제
- 메모리 제한이 시간 제한보다 널널해서 총 3개의 메인 변수를 선언하여 풀이했다
-> board: 2차원 리스트/ 각 체스판의 색깔 정보 저장
-> horses: 2차원 리스트/ 각 말의 번호당 x, y, direction 정보 저장
-> check: 3차원 리스트 (내부 원소는 deque)/ 해당 위치에 있는 말들의 정보 저장
- 매 turn마다 horses를 순서대로 순회하며 이동시켜줌. 이때 가장 아래에 있는 말만 이동할 수 있기 때문에 flag가 true로 바뀐 경우에만 이를 실행해줘야함
코드
import sys
from collections import deque
dx = [0, 0, -1, 1]
dy = [1, -1, 0, 0]
N, K = map(int, sys.stdin.readline()[:-1].split())
board = []
for n in range(N):
board.append(list(map(int, sys.stdin.readline()[:-1].split())))
horses = []; check = [[deque([]) for _ in range(N)] for _ in range(N)]
for k in range(K):
x, y, direction = map(int, sys.stdin.readline()[:-1].split())
horses.append([x-1, y-1, direction-1])
check[x-1][y-1].append(k)
turn = 0
while True:
turn += 1
if turn > 1000:
print(-1)
exit()
for i in range(len(horses)):
x, y, direction = horses[i]
flag = False
if i == check[x][y][0]: flag = True
if flag:
nx = x + dx[direction]; ny = y + dy[direction]
if 0 <= nx < N and 0 <= ny < N:
if board[nx][ny] == 0:
while check[x][y]:
horse_num = check[x][y].popleft()
horses[horse_num][0] = nx; horses[horse_num][1] = ny
check[nx][ny].append(horse_num)
elif board[nx][ny] == 1:
tmp_check = deque([])
while check[x][y]:
horse_num = check[x][y].popleft()
horses[horse_num][0] = nx; horses[horse_num][1] = ny
tmp_check.appendleft(horse_num)
check[nx][ny].extend(list(tmp_check))
elif board[nx][ny] == 2:
if direction == 0: direction = 1
elif direction == 1: direction = 0
elif direction == 2: direction = 3
elif direction == 3: direction = 2
horses[i][2] = direction
nx = x + dx[direction]; ny = y + dy[direction]
if 0 <= nx < N and 0 <= ny < N:
if board[nx][ny] == 0 :
while check[x][y]:
horse_num = check[x][y].popleft()
horses[horse_num][0] = nx; horses[horse_num][1] = ny
check[nx][ny].append(horse_num)
elif board[nx][ny] == 1:
tmp_check = deque([])
while check[x][y]:
horse_num = check[x][y].popleft()
horses[horse_num][0] = nx; horses[horse_num][1] = ny
tmp_check.appendleft(horse_num)
check[nx][ny].extend(list(tmp_check))
else:
if direction == 0: direction = 1
elif direction == 1: direction = 0
elif direction == 2: direction = 3
elif direction == 3: direction = 2
horses[i][2] = direction
nx = x + dx[direction]; ny = y + dy[direction]
if 0 <= nx < N and 0 <= ny < N:
if board[nx][ny] == 0 :
while check[x][y]:
horse_num = check[x][y].popleft()
horses[horse_num][0] = nx; horses[horse_num][1] = ny
check[nx][ny].append(horse_num)
elif board[nx][ny] == 1:
tmp_check = deque([])
while check[x][y]:
horse_num = check[x][y].popleft()
horses[horse_num][0] = nx; horses[horse_num][1] = ny
tmp_check.appendleft(horse_num)
check[nx][ny].extend(list(tmp_check))
for i in range(N):
for j in range(N):
if len(check[i][j]) >= 4:
print(turn)
exit()
결과
