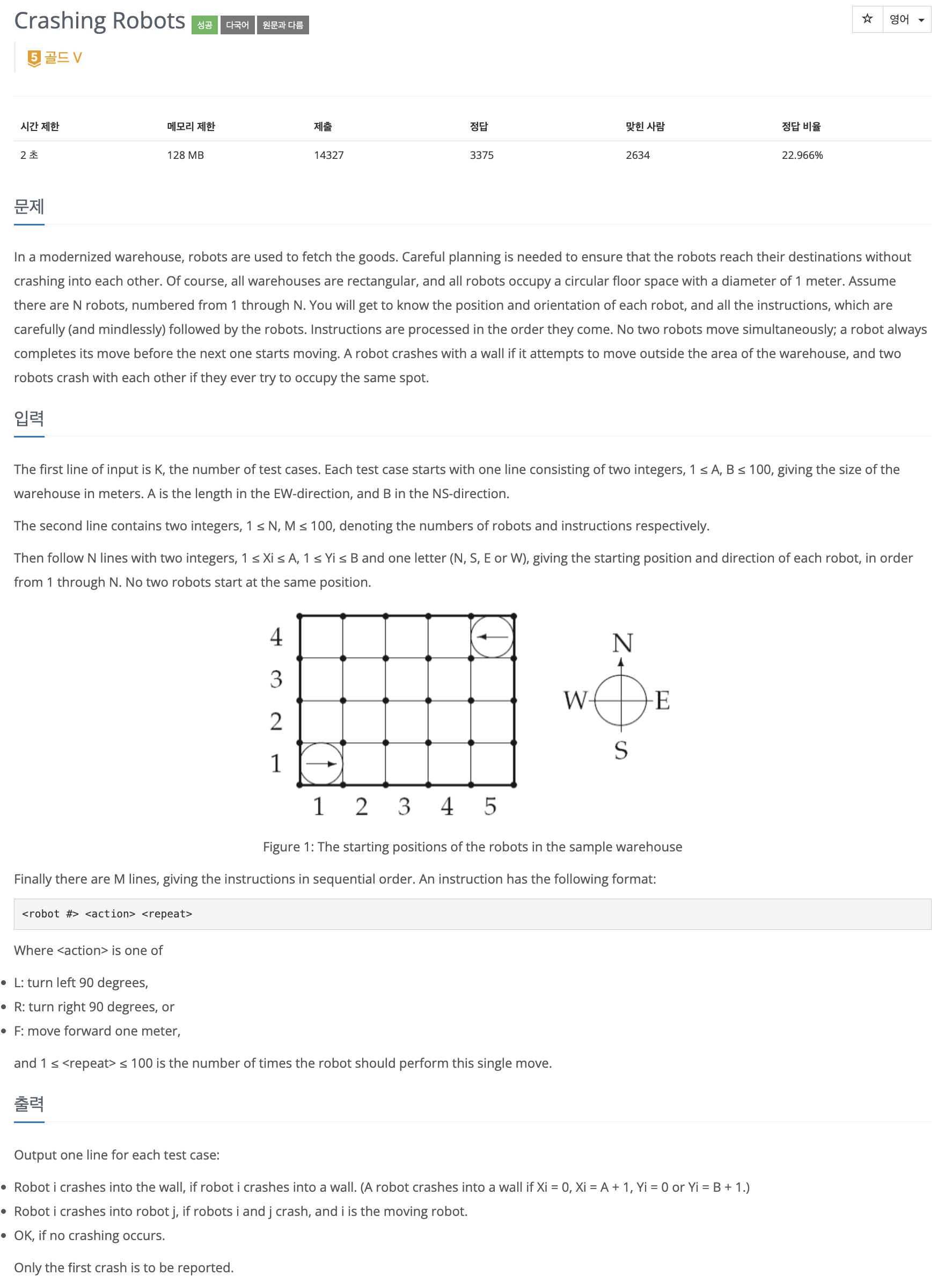
문제 링크
2174: Crashing Robots
구현 방식
- 2차원 list로 board를 선언해주고, 로봇이 없는 칸은 0, 로봇이 있는 칸은 로봇의 번호를 값으로 갖도록 해주었다
- robot이라는 hashMap을 이용해서 key는 로봇의 번호, value는 [x좌표, y좌표, 로봇의 현재 방향] 형식으로 데이터를 저장해주었다
- 그 외의 구현은 문제에서 안내한대로 해주면 된다
코드
#include <iostream>
#include <vector>
#include <map>
#include <string>
#include <array>
using namespace std;
#define MAX 100
int A, B, N, M;
int board[MAX][MAX] = {0, };
map<int, vector<int> > robot;
map<char, int> direction;
int dx[4] = {-1, 0, 0, 1};
int dy[4] = {0, -1, 1, 0};
int main() {
cin >> A >> B;
cin >> N >> M;
direction['N'] = 0; direction['W'] = 1; direction['E'] = 2; direction['S'] = 3;
for (int n=1; n<=N; n++){
int x, y;
char d;
cin >> y >> x >> d;
x = B - x; y--;
board[x][y] = n; robot[n] = vector<int> {x, y, direction[d]};
}
for (int m=0; m<M; m++){
int num, iteration;
char cmd;
cin >> num >> cmd >> iteration;
if (cmd == 'L'){
iteration %= 4;
for (int t=0; t<iteration; t++){
int d = robot[num][2];
if (d == 0) robot[num][2] = 1;
else if (d == 1) robot[num][2] = 3;
else if (d == 2) robot[num][2] = 0;
else if (d == 3) robot[num][2] = 2;
}
}
else if (cmd == 'R'){
iteration %= 4;
for (int t=0; t<iteration; t++){
int d = robot[num][2];
if (d == 0) robot[num][2] = 2;
else if (d == 1) robot[num][2] = 0;
else if (d == 2) robot[num][2] = 3;
else if (d == 3) robot[num][2] = 1;
}
}
else if (cmd == 'F'){
for (int t=0; t<iteration; t++){
int x = robot[num][0]; int y = robot[num][1]; int d = robot[num][2];
int nx = x + dx[d]; int ny = y + dy[d];
if (0 <= nx && nx < B && 0 <= ny && ny < A){
if (board[nx][ny] != 0){
cout << "Robot " << num << " crashes into robot " << board[nx][ny] << endl;
return 0;
}
else{
board[x][y] = 0; board[nx][ny] = num;
robot[num] = vector<int> {nx, ny, d};
}
}
else{
cout << "Robot " << num << " crashes into the wall" << endl;
return 0;
}
}
}
}
cout << "OK" << endl;
return 0;
}
import sys
dx = (-1, 0, 0, 1)
dy = (0, -1, 1, 0)
direction = {'N':0, 'W':1, 'E':2, 'S':3}
A, B = map(int, sys.stdin.readline()[:-1].split()); N, M = map(int, sys.stdin.readline()[:-1].split())
board = [[0]*A for _ in range(B)]; robot = dict()
for n in range(1, N+1):
y, x, d = sys.stdin.readline()[:-1].split(); x = int(x); y = int(y); x = B-x; y -= 1
board[x][y] = n; robot[n] = [x, y, d]
for m in range(M):
num, cmd, iteration = sys.stdin.readline()[:-1].split(); num = int(num); iteration = int(iteration)
if cmd == 'L':
iteration %= 4
for _ in range(iteration):
d = robot[num][2]
if d == 'N': robot[num][2] = 'W'
elif d == 'W': robot[num][2] = 'S'
elif d == 'E': robot[num][2] = 'N'
elif d == 'S': robot[num][2] = 'E'
elif cmd == 'R':
iteration %= 4
for _ in range(iteration):
d = robot[num][2]
if d == 'N': robot[num][2] = 'E'
elif d == 'W': robot[num][2] = 'N'
elif d == 'E': robot[num][2] = 'S'
elif d == 'S': robot[num][2] = 'W'
elif cmd == 'F':
for _ in range(iteration):
x = robot[num][0]; y = robot[num][1]; d = robot[num][2]
nx = x + dx[direction[d]]; ny = y + dy[direction[d]]
if 0 <= nx < B and 0 <= ny < A:
if board[nx][ny] != 0:
print("Robot "+str(num)+" crashes into robot "+str(board[nx][ny])); exit()
else:
board[x][y] = 0; board[nx][ny] = num
robot[num] = [nx, ny, d]
else:
print("Robot "+str(num)+" crashes into the wall"); exit()
print("OK")