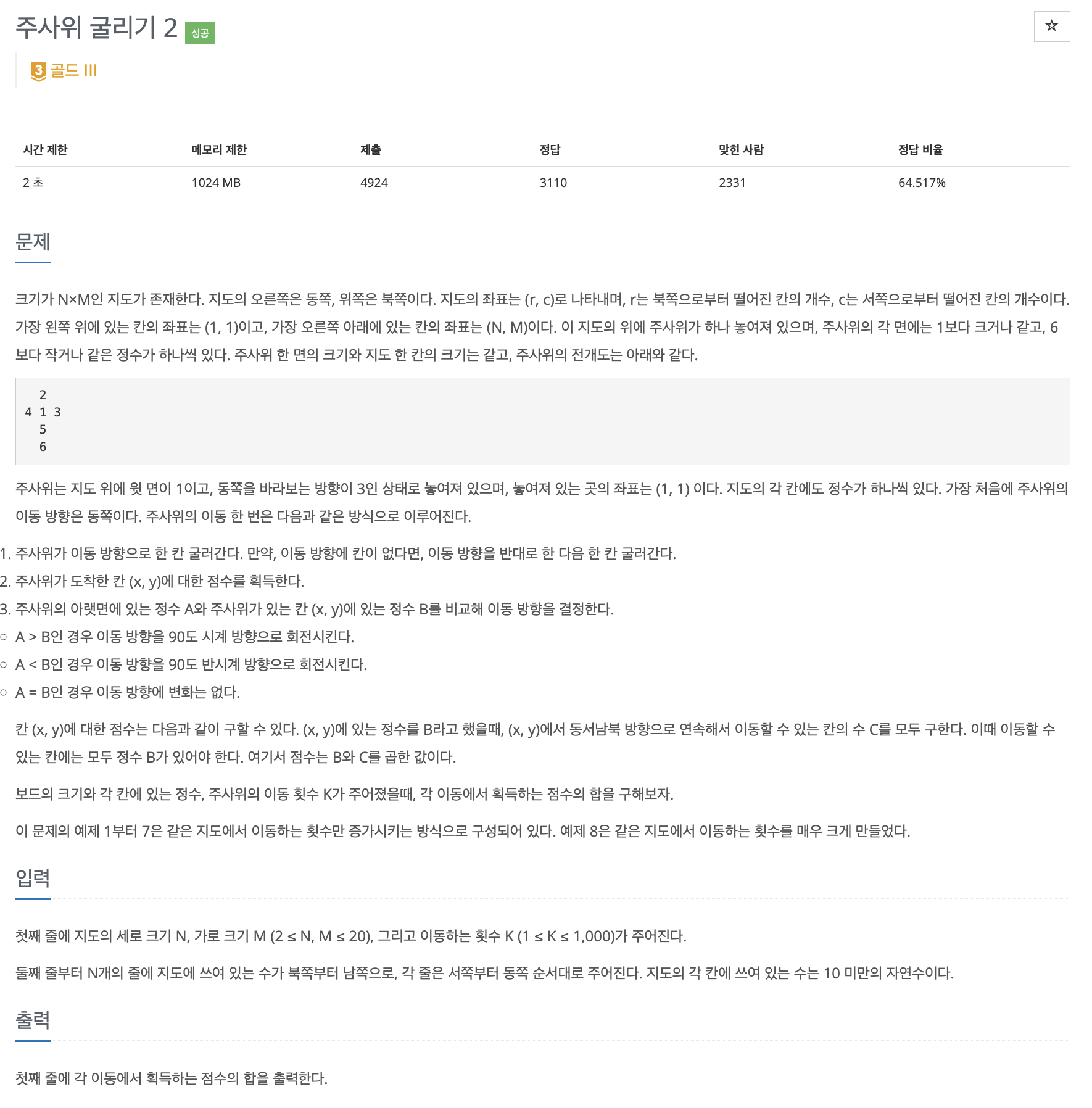
문제 링크
23288: 주사위 굴리기 2
구현 방식
- 전반적인 구현 흐름은 아래와 같다
K만큼 for문을 돌면서,
- 현재 위치에서 현재 방향으로 이동했을 때, 칸이 있는지 없는지 판별하여 없다면 방향을 반대로 바꿔준다
- 다음 위치로 주사위를 이동시킨다
- 해당 경우에 획득할 수 있는 점수를 bfs 탐색으로 구해준다
- change_dice 함수를 호출하여 주사위 전개도를 변경해준다
- 초기의 전개도를 dice = [1, 2, 3, 4, 5, 6]으로 설정해놓고 각 방향(동, 서, 남, 북)으로 이동했을 때 전개도가 어떻게 바뀌는 지 하드코딩 해줌
- B(칸 (x,y)에 있는 정수)와 변경된 전개도에 따른 A(주사위의 아랫면에 있는 정수)의 크기를 비교하여 방향을 변경해준다
코드
import sys
from collections import deque
dx = (0, 1, 0, -1)
dy = (1, 0, -1, 0)
N, M, K = map(int, sys.stdin.readline()[:-1].split())
board = [list(map(int, sys.stdin.readline()[:-1].split())) for _ in range(N)]
dice = [1, 2, 3, 4, 5, 6]
def change_dice(didx):
d_0 = dice[0]; d_1 = dice[1]; d_2 = dice[2]; d_3 = dice[3]; d_4 = dice[4]; d_5 = dice[5]
if didx == 0:
dice[0] = d_3; dice[1] = d_1; dice[2] = d_0; dice[3] = d_5; dice[4] = d_4; dice[5] = d_2
elif didx == 1:
dice[0] = d_1; dice[1] = d_5; dice[2] = d_2; dice[3] = d_3; dice[4] = d_0; dice[5] = d_4
elif didx == 2:
dice[0] = d_2; dice[1] = d_1; dice[2] = d_5; dice[3] = d_0; dice[4] = d_4; dice[5] = d_3
elif didx == 3:
dice[0] = d_4; dice[1] = d_0; dice[2] = d_2; dice[3] = d_3; dice[4] = d_5; dice[5] = d_1
def bfs(x, y, check):
q = deque([]); q.append((x, y))
visit = [[False]*M for n in range(N)]; visit[x][y] = True
cnt = 0
while q:
x, y = q.popleft()
cnt += 1
for i in range(4):
nx, ny = x + dx[i], y + dy[i]
if 0 <= nx < N and 0 <= ny < M:
if not visit[nx][ny] and board[nx][ny] == check:
visit[nx][ny] = True; q.append((nx, ny))
return cnt
x, y, didx, answer = 0, 0, 0, 0
for k in range(K):
if x+dx[didx] < 0 or x+dx[didx] >= N or y+dy[didx] < 0 or y+dy[didx] >= M: didx = (didx+2) % 4
x, y = x + dx[didx], y + dy[didx]
answer += (bfs(x, y, board[x][y]) * board[x][y])
change_dice(didx)
if dice[5] > board[x][y]: didx = (didx+1) % 4
elif dice[5] < board[x][y]: didx = (didx+3) % 4
print(answer)