ConstraintLayout
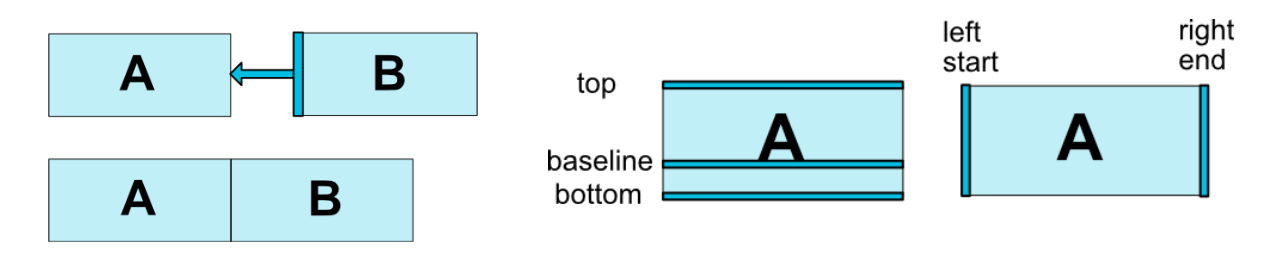
- ConstraintLayout is a ViewGroup which allows you to position and size widgets in a flexible way: Constraint
- Relative: with constraint & margin value & fixed width/height
- You should check all for horizontal & vertical
- constraint for start/end, width
- constraint for top/bottom, height
- To use 'match_constraint (= 0dp)', both side must be set
- If you use 'match_constraint (= 0dp)', constraint is not essential
- Check your view is properly positioned
Guideline
- You can use guidelines to set constraints properly
- Guideline can be Xdp from left/right/up/down
- or X% horizontally/vertically
- You can divide the screen into sections
- Scrollable View
- Only one component can be in
- Put ImageView and TextView inside the ScrollView
- Directly put two views is imporssible
- Put it inside LinearLayout inside of ScrollView
ListView
- Think about you are making listview manually
- You need to iterate with the number of items
- and construct the view by objectifying each element in each item
- With Inflater, you can make use of layout XML instead of making and adjusting all element
- With Adapter, you do not need to take care of iteration
- Just listView.adpater = myAdapterInstance do all!
- It is calling setter, listView.setAdapter(...)
Chatroom List
- MainActivity.kt
- ChatRoom.kt
- ChatRoomAdapter.kt
- activity_main.xml
- item_chatroom.xml
activity_main.xml
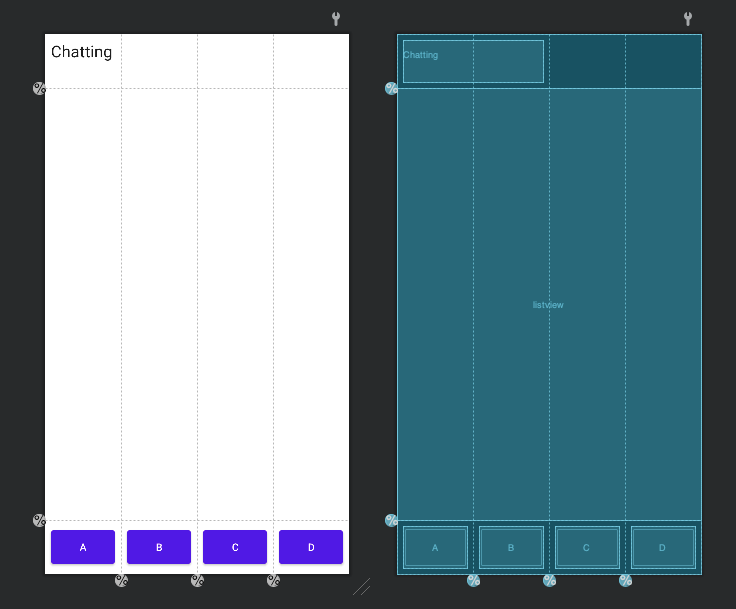
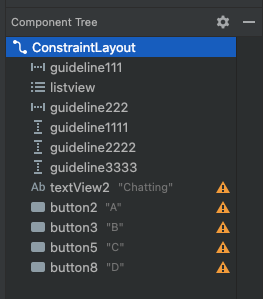
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<androidx.constraintlayout.widget.Guideline
android:id="@+id/guideline111"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal"
app:layout_constraintGuide_percent="0.1" />
<ListView
android:id="@+id/listview"
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintBottom_toTopOf="@+id/guideline222"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="@+id/guideline111" />
<androidx.constraintlayout.widget.Guideline
android:id="@+id/guideline222"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal"
app:layout_constraintGuide_percent="0.9" />
<androidx.constraintlayout.widget.Guideline
android:id="@+id/guideline1111"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
app:layout_constraintGuide_percent="0.25" />
<androidx.constraintlayout.widget.Guideline
android:id="@+id/guideline2222"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
app:layout_constraintGuide_percent="0.5" />
<androidx.constraintlayout.widget.Guideline
android:id="@+id/guideline3333"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
app:layout_constraintGuide_percent="0.75" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:text="Chatting"
android:textAppearance="@style/TextAppearance.AppCompat.Large"
app:layout_constraintBottom_toTopOf="@+id/guideline111"
app:layout_constraintEnd_toStartOf="@+id/guideline2222"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:text="A"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@+id/guideline1111"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="@+id/guideline222" />
<Button
android:id="@+id/button3"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:text="B"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@+id/guideline2222"
app:layout_constraintStart_toStartOf="@+id/guideline1111"
app:layout_constraintTop_toTopOf="@+id/guideline222" />
<Button
android:id="@+id/button5"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:text="C"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@+id/guideline3333"
app:layout_constraintStart_toStartOf="@+id/guideline2222"
app:layout_constraintTop_toTopOf="@+id/guideline222" />
<Button
android:id="@+id/button8"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:text="D"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="@+id/guideline3333"
app:layout_constraintTop_toTopOf="@+id/guideline222" />
</androidx.constraintlayout.widget.ConstraintLayout>
item_chatroom.xml
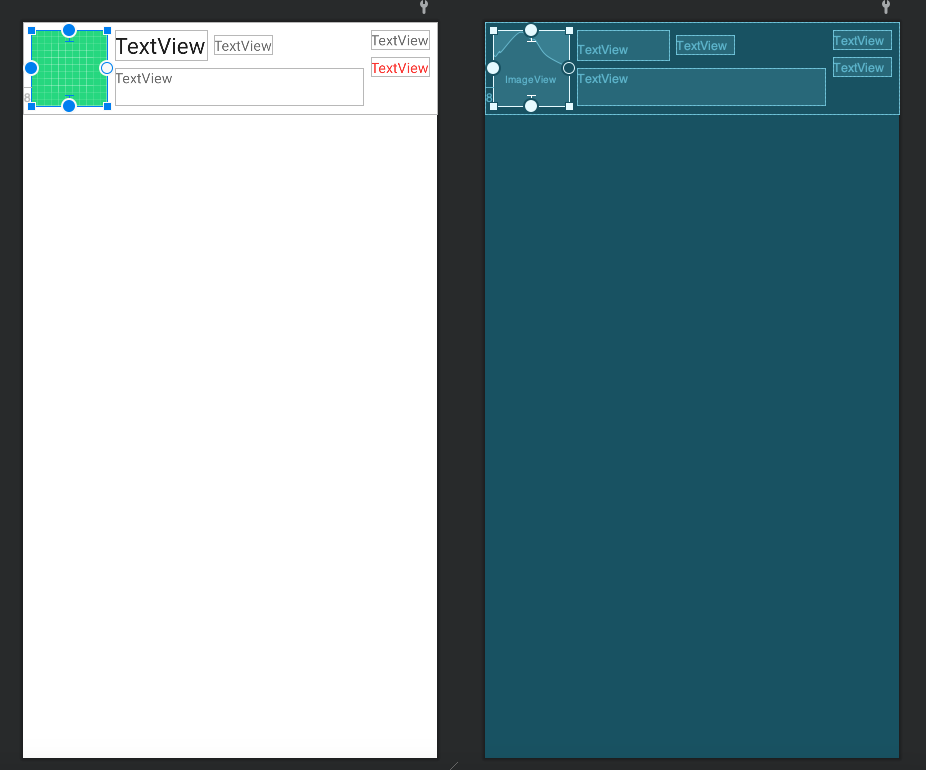
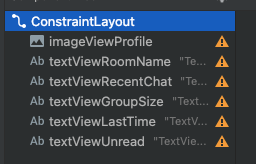
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/imageViewProfile"
android:layout_width="75dp"
android:layout_height="75dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginBottom="8dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:srcCompat="@drawable/ic_launcher_background" />
<TextView
android:id="@+id/textViewRoomName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:text="TextView"
android:textAppearance="@style/TextAppearance.AppCompat.Large"
app:layout_constraintStart_toEndOf="@+id/imageViewProfile"
app:layout_constraintTop_toTopOf="@+id/imageViewProfile" />
<TextView
android:id="@+id/textViewRecentChat"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:text="TextView"
app:layout_constraintBottom_toBottomOf="@+id/imageViewProfile"
app:layout_constraintEnd_toStartOf="@+id/textViewUnread"
app:layout_constraintStart_toEndOf="@+id/imageViewProfile"
app:layout_constraintTop_toBottomOf="@+id/textViewRoomName" />
<TextView
android:id="@+id/textViewGroupSize"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:text="TextView"
app:layout_constraintBottom_toBottomOf="@+id/textViewRoomName"
app:layout_constraintStart_toEndOf="@+id/textViewRoomName"
app:layout_constraintTop_toTopOf="@+id/textViewRoomName" />
<TextView
android:id="@+id/textViewLastTime"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:text="TextView"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textViewUnread"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:text="TextView"
android:textColor="#F44336"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textViewLastTime" />
</androidx.constraintlayout.widget.ConstraintLayout>
ChatRoom.kt
package com.example.week5
class ChatRoom (
val name: String,
val lastChat: String,
val thumbnail: Int,
val groupNumber: Int,
val lastTime: String,
val unread: Int
){
}
ChatRoomAdapter.kt
package com.example.week5
import android.content.Context
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.BaseAdapter
import android.widget.ImageView
import android.widget.TextView
class ChatRoomAdapter (val data: ArrayList<ChatRoom>, val context: Context): BaseAdapter() {
override fun getCount(): Int {
return data.size
}
override fun getItem(position: Int): Any {
return data[position]
}
override fun getItemId(position: Int): Long {
return 0
}
override fun getView(position: Int, convertView: View?, parent: ViewGroup?): View {
val inflater = context.getSystemService(Context.LAYOUT_INFLATER_SERVICE) as LayoutInflater
val generatedView = inflater.inflate(R.layout.item_chatroom, null)
val textViewName = generatedView.findViewById<TextView>(R.id.textViewRoomName)
val textViewChat = generatedView.findViewById<TextView>(R.id.textViewRecentChat)
val textViewGroupNumber = generatedView.findViewById<TextView>(R.id.textViewGroupSize)
val textViewTime = generatedView.findViewById<TextView>(R.id.textViewLastTime)
val imageViewThumbnail = generatedView.findViewById<ImageView>(R.id.imageViewProfile)
val textViewUnread = generatedView.findViewById<TextView>(R.id.textViewUnread)
textViewUnread.text = "" + data[position].unread
textViewName.text = data[position].name
textViewChat.text = data[position].lastChat
textViewTime.text = data[position].lastTime
//textViewGroupNumber.text = "" + data[position].groupNumber
textViewGroupNumber.text = if (data[position].groupNumber != 1) "" + data[position].groupNumber else ""
imageViewThumbnail.setImageResource(data[position].thumbnail)
return generatedView
}
}
- hiding the number of people in group when it is 1
MainActivity.kt
package com.example.week5
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.ListView
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val items = ArrayList<ChatRoom>()
items.add(ChatRoom("Kakao T", "Please leave review.", R.drawable.ic_launcher_background, 1, "8:24 p.m.", 1))
items.add(ChatRoom("SKKU Software", "Anybody taking MAP lecture? This week lab session is too hard. Can anybody give me the hint? I will be very happy if you help. If nobody help me, I will be very sad.", R.drawable.ic_launcher_background, 512, "7:15 p.m.", 128))
items.add(ChatRoom("Brother", "Hey.", R.drawable.ic_launcher_background, 1, "4:21 p.m.", 12))
items.add(ChatRoom("Family", "Emoji", R.drawable.ic_launcher_background, 4, "4:05 p.m.", 5))
items.add(ChatRoom("Study group", "See you tomorrow!", R.drawable.ic_launcher_background, 5, "4:01 p.m.", 2))
items.add(ChatRoom("Yogiyo", "How was the food?", R.drawable.ic_launcher_background, 1, "3:24 p.m.", 1))
items.add(ChatRoom("lorem ipsum", "dolor", R.drawable.ic_launcher_background, 6, "2:22 p.m.", 123))
items.add(ChatRoom("Placeholder", "Placeholder", R.drawable.ic_launcher_background, 12, "11:58 a.m.", 5))
val myAdapter = ChatRoomAdapter(items, applicationContext)
val listView = findViewById<ListView>(R.id.listview)
listView.adapter = myAdapter
}
}
- Implement ListView using ChatRoomAdapter