Object vs Data structure
JS에서의 자료구조
- 검색, 정렬, 삽입, 삭제
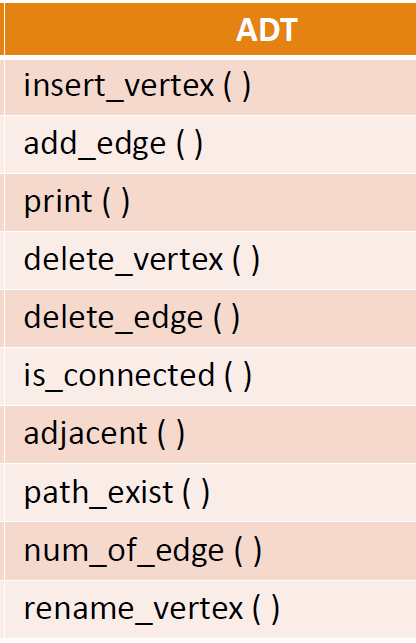
Declaration
const arr1 = new Array();
const arr2 = [1, 2];
Index position
const fruits = ['python', 'banana'];
console.log(fruits);
console.log(fruits.length);
console.log(fruits[0]);
console.log(fruits[fruits.length - 1]);
Looping over an array
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
for (let fruit of fruits) {
console.log(fruit);
}
fruits.forEach((fruit) => console.log(fruit));
Addition, deletion, copy
fruits.push('butter', 'comet');
console.log(fruits);
fruits.pop();
fruits.pop();
console.log(fruits);
fruits.unshift('butter', 'comet');
fruits.shift();
fruits.shift();
console.log(fruits);
fruits.push('airplane', 'coke', 'sleep');
console.log(fruits);
fruits.splice(1);
fruits.splice(1, 1);
fruits.splice(1, 2, 'js', 'node');
const fruits2 = ['pear', 'ship'];
const newFruits = fruits.concat(fruits2);
console.log(newFruits);
Searching
console.clear();
console.log(fruits);
console.log(fruits.indexOf('python'));
console.log(fruits.includes('JS'));
console.clear();
fruits.push('node');
console.log(fruits);
console.log(fruits.indexOf('node'));
console.log(fruits.lastIndexOf('node'));