sheet 클래스
const Sheet = class{
constructor(sheet){
this._sheet = sheet;
this._rules = new Map();
}
add(selector){
const index = this._sheet.cssRules.length;
this._sheet.insertRule(`${selector}{}`,index);
const cssRule = this._sheet.cssRules[index];
const rule = new Rule(cssRule);
this._rules.set(selector,rule);
return rule;
}
remove(selector){
if(!this._rules.contains(selector)) return;
const rule = this._rules.get(selector)._rule;
Array.from(this._sheet.cssRules).some((cssRule,index)=>{
if(cssRule === rule._rule){
this._sheet.deleteRule(index);
return true;
}
})
}
get(selector){return this._rules.get(selector);}
}
전체코드
<html>
<head lang="en">
<meta charset="UTF-8">
<style>
</style>
<body>
<div class="test">a</div>
</body>
<script>
const Style = ((_) => {
const prop = new Map, prefix = 'webkt,moz,ms,chrome,o,khtml'.split(',');
const NONE = Symbol();
const BASE = document.body.style;
const getKey = (key) => {
if(prop.has(key)) return prop.get(key);
if(key in BASE) prop.set(key, key);
else if(!prefix.some(v =>{
const newKey = v + key[0].toUpperCase() + key.substr(1);
if(newKey in BASE){
prop.set(key, newKey);
key = newKey;
return true;
} else return false;
})){
prop.set(key, NONE);
key = NONE;
}
return key;
};
return class {
constructor(style) {
this._style = style;
}
get(key) {
key = getKey(key);
if (key === NONE) return null;
return this._style[key];
}
set(key, val) {
key = getKey(key);
if (key !== NONE) this._style[key] = val;
return this;
}
};
})();
const Rule = class{
constructor(rule){
this._rule = rule;
this._style = new Style(rule.style);
}
get(key){
return this._style.get(key);
}
set(key,val){
this._style.set(key,val);
return this;
}
}
const Sheet = class{
constructor(sheet){
this._sheet = sheet;
this._rules = new Map();
}
add(selector){
const index = this._sheet.cssRules.length;
this._sheet.insertRule(`${selector}{}`,index);
const cssRule = this._sheet.cssRules[index];
const rule = new Rule(cssRule);
this._rules.set(selector,rule);
return rule;
}
remove(selector){
if(!this._rules.contains(selector)) return;
const rule = this._rules.get(selector)._rule;
Array.from(this._sheet.cssRules).some((cssRule,index)=>{
if(cssRule === rule._rule){
this._sheet.deleteRule(index);
return true;
}
})
}
get(selector){return this._rules.get(selector);}
}
const sheet = new Sheet(document.styleSheets[0]);
sheet.add('body').set('background', '#f00');
sheet.add('.test').set(
'cssText',
`
width:200px;
border:1px solid #fff;
color: #000;
background: #fff
`
);
</script>
</html>
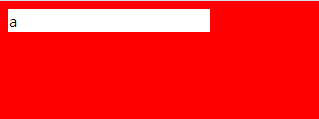