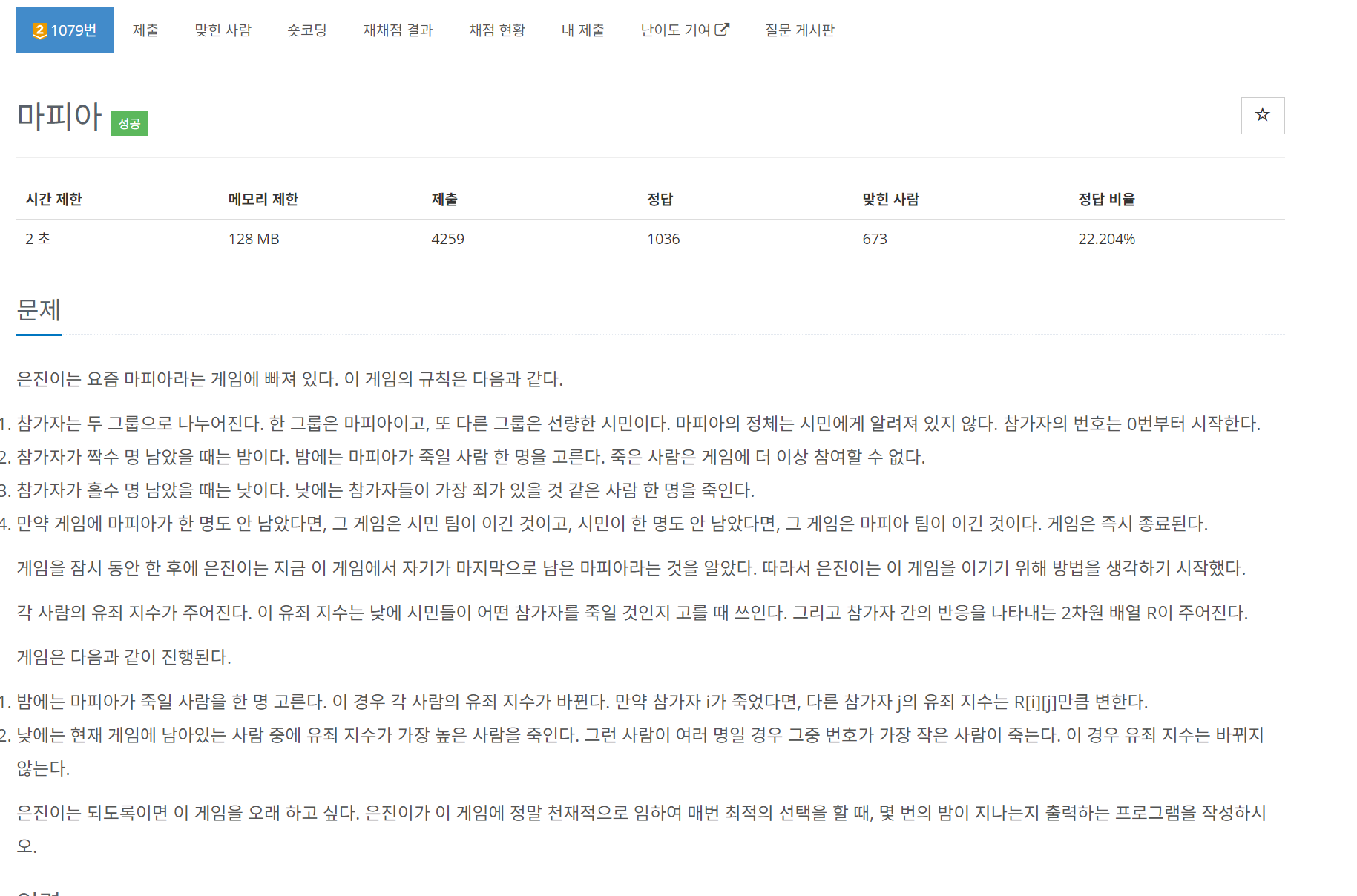
오답
#include <iostream>
#include <vector>
#include <cstring>
#include <algorithm>
using namespace std;
struct PLAYER {
int score;
int number;
int Dead = false;
bool operator<(const PLAYER& other) const {
return score > other.score;
}
};
int map[16][16];
int eunjin;
int n;
int result = 0;
vector<PLAYER> score;
void input() {
cin >> n;
for (int i = 0; i < n; i++) {
int a;
cin >> a;
score.push_back({ a,i });
}
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
cin >> map[i][j];
}
}
cin >> eunjin;
}
void solve(vector<PLAYER> _score, int day, int people) {
if (people % 2 == 0) {
for (int i = 0; i < n; i++) {
vector<PLAYER> scoreCopy = _score;
if (scoreCopy[i].number == eunjin) continue;
if (scoreCopy[i].Dead == true) continue;
int _day = day;
_day++;
result = max(_day, result);
scoreCopy[i].Dead = true;
for (int z = 0; z < n; z++) {
if (scoreCopy[z].Dead == true) continue;
scoreCopy[z].score += map[i][z];
}
solve(scoreCopy, _day, people - 1);
scoreCopy[i].Dead = false;
}
}
else {
vector<PLAYER> scoreCopy = _score;
sort(scoreCopy.begin(), scoreCopy.end());
if (scoreCopy[0].number != eunjin) {
scoreCopy[0].Dead = true;
solve(scoreCopy, day,people-1);
}
else {
return;
}
}
}
int main() {
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
input();
solve(score,0, n);
cout << result;
return 0;
}
#include<iostream>
#define endl "\n"
#define MAX 20
using namespace std;
struct INFO
{
int Score;
bool LIVE;
};
bool Flag;
int N, Mafia_Idx, Answer;
int Score_Board[MAX][MAX];
INFO Player[MAX];
int Min(int A, int B) { if (A < B) return A; return B; }
int Bigger(int A, int B) { if (A > B) return A; return B; }
void Input()
{
cin >> N;
for (int i = 0; i < N; i++)
{
cin >> Player[i].Score;
Player[i].LIVE = true;
}
for (int i = 0; i < N; i++)
{
for (int j = 0; j < N; j++)
{
cin >> Score_Board[i][j];
}
}
cin >> Mafia_Idx;
}
void DFS(int PN, int Day)
{
if (Flag == true) return;
if (Player[Mafia_Idx].LIVE == false || PN == 1)
{
Answer = Bigger(Answer, Day);
if (PN == 1 && Player[Mafia_Idx].LIVE == true) Flag = true;
return;
}
if (PN % 2 == 0)
{
for (int i = 0; i < N; i++)
{
if (i == Mafia_Idx) continue;
if (Player[i].LIVE == false) continue;
Player[i].LIVE = false;
for (int j = 0; j < N; j++)
{
if (Player[j].LIVE == false) continue;
Player[j].Score = Player[j].Score + Score_Board[i][j];
}
DFS(PN - 1, Day + 1);
if (Flag == true) return;
for (int j = 0; j < N; j++)
{
if (Player[j].LIVE == false) continue;
Player[j].Score = Player[j].Score - Score_Board[i][j];
}
Player[i].LIVE = true;
}
}
else
{
int Max_Score = 0;
int Idx = 0;
for (int i = 0; i < N; i++)
{
if (Player[i].LIVE == false) continue;
if (Player[i].Score > Max_Score)
{
Max_Score = Player[i].Score;
Idx = i;
}
else if (Player[i].Score == Max_Score) Idx = Min(Idx, i);
}
Player[Idx].LIVE = false;
DFS(PN - 1, Day);
if (Flag == true) return;
Player[Idx].LIVE = true;
}
}
void Solution()
{
DFS(N, 0);
cout << Answer << endl;
}
void Solve()
{
Input();
Solution();
}
int main(void)
{
ios::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
Solve();
return 0;
}