직렬화 (Serialization)
- 인스턴스의 상태를 그대로 저장하거나 네트웍으로 전송하고 이를 다시 복원 (Deserialization)하는 방식
- ObjectInputStream 과 ObjectOutputStream 사용
- 보조 스트림
Serialization 인터페이스
- 직렬화는 인스턴스의 내용이 외부(파일, 네트워크)로 유출되는 것이므로 프로그래머가 객체의 직렬화 가능 여부를 명시함
- 구현코드가 없는 mark interface
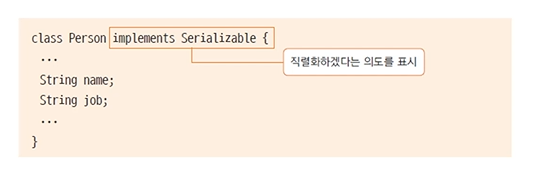
class Person implements Serializable{
String name;
String job;
public Person(String name, String job) {
this.name=name;
this.job=job;
}
public String toString() {
return name+","+job;
}
}
public class SerializationTest {
public static void main(String[] args) {
Person personLee=new Person("이순신","엔지니어");
Person personKim=new Person("김유신","선생님");
try(FileOutputStream fos= new FileOutputStream("serial.dat");
ObjectOutputStream oos= new ObjectOutputStream(fos)){
oos.writeObject(personLee);
oos.writeObject(personKim);
}catch(IOException e) {
System.out.println(e);
}
try(FileInputStream fis= new FileInputStream("serial.dat");
ObjectInputStream ois= new ObjectInputStream(fis)){
Person p1=(Person)ois.readObject();
Person p2=(Person)ois.readObject();
System.out.println(p1);
System.out.println(p2);
}catch(IOException e) {
System.out.println(e);
}catch(ClassNotFoundException e) {
System.out.println(e);
}
}
}